Top Related Projects
A complete computer science study plan to become a software engineer.
💯 Curated coding interview preparation materials for busy software engineers
Learn how to design large-scale systems. Prep for the system design interview. Includes Anki flashcards.
Interactive roadmaps, guides and other educational content to help developers grow in their careers.
📝 Algorithms and data structures implemented in JavaScript with explanations and links to further readings
:books: Freely available programming books
Quick Overview
CS-Notes is a comprehensive collection of study notes and resources for computer science and technology topics. It covers a wide range of subjects including data structures, algorithms, operating systems, network protocols, and more. The repository serves as a valuable reference for students, developers, and professionals in the field of computer science.
Pros
- Extensive coverage of various computer science topics in one place
- Well-organized content with clear categorization
- Regular updates and contributions from the community
- Includes both theoretical concepts and practical examples
Cons
- Primarily written in Chinese, which may limit accessibility for non-Chinese speakers
- Some topics may not be covered in as much depth as dedicated textbooks or courses
- The sheer volume of information can be overwhelming for beginners
- Lacks interactive elements or exercises for hands-on learning
Note: As this is not a code library, the code example and quick start sections have been omitted as per the instructions.
Competitor Comparisons
A complete computer science study plan to become a software engineer.
Pros of coding-interview-university
- More comprehensive coverage of computer science fundamentals
- Structured learning path with a clear roadmap
- Includes video resources and links to external learning materials
Cons of coding-interview-university
- Primarily in English, which may limit accessibility for non-English speakers
- Less focus on practical coding examples and problem-solving
- May be overwhelming for beginners due to its extensive content
Code comparison
CS-Notes:
public class Solution {
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode curr = head;
while (curr != null) {
ListNode nextTemp = curr.next;
curr.next = prev;
prev = curr;
curr = nextTemp;
}
return prev;
}
}
coding-interview-university:
def reverse(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
Both repositories provide valuable resources for computer science and coding interview preparation. CS-Notes offers more concise, topic-focused content with practical examples, while coding-interview-university provides a broader, more structured approach to learning computer science fundamentals. The choice between the two depends on individual learning preferences and goals.
💯 Curated coding interview preparation materials for busy software engineers
Pros of tech-interview-handbook
- More comprehensive coverage of non-technical aspects of interviews (e.g., behavioral questions, negotiation)
- Better organized structure with clear sections for different interview stages
- Includes a curated list of external resources and study materials
Cons of tech-interview-handbook
- Less in-depth coverage of specific computer science topics compared to CS-Notes
- Primarily focused on web development and front-end technologies, which may not be suitable for all roles
Code comparison
CS-Notes example (Java):
public class BinarySearch {
public int binarySearch(int[] nums, int target) {
int left = 0, right = nums.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (nums[mid] == target) return mid;
if (nums[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
}
tech-interview-handbook example (JavaScript):
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
Learn how to design large-scale systems. Prep for the system design interview. Includes Anki flashcards.
Pros of system-design-primer
- Focuses specifically on system design, providing in-depth coverage of this crucial topic
- Includes visual aids and diagrams to illustrate complex concepts
- Offers interactive coding exercises and real-world examples
Cons of system-design-primer
- Narrower scope, primarily covering system design rather than a broad range of CS topics
- Less comprehensive coverage of fundamental CS concepts and algorithms
- May be more challenging for beginners due to its focus on advanced topics
Code comparison
While both repositories primarily focus on explanations rather than code, system-design-primer does include some code snippets for illustration. CS-Notes tends to have more algorithm implementations. Here's a brief comparison:
system-design-primer:
def get_user(user_id):
user = memcache.get("user.{0}", user_id)
if user is None:
user = db.query("SELECT * FROM users WHERE user_id = {0}", user_id)
memcache.set("user.{0}", user, 30)
return user
CS-Notes:
public class QuickSort {
public static void sort(int[] arr) {
quickSort(arr, 0, arr.length - 1);
}
private static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int partitionIndex = partition(arr, low, high);
quickSort(arr, low, partitionIndex - 1);
quickSort(arr, partitionIndex + 1, high);
}
}
// ... (partition method omitted for brevity)
}
Interactive roadmaps, guides and other educational content to help developers grow in their careers.
Pros of developer-roadmap
- Visual representation of learning paths for various tech roles
- Regularly updated with modern technologies and practices
- Community-driven content with contributions from developers worldwide
Cons of developer-roadmap
- Less in-depth technical content compared to CS-Notes
- May overwhelm beginners with the sheer amount of information presented
- Focuses more on web development, potentially lacking coverage in other CS areas
Code comparison
While both repositories primarily focus on educational content rather than code, developer-roadmap does include some code snippets in its explanations. CS-Notes, on the other hand, is more text-based. Here's a brief example from developer-roadmap:
// Example from developer-roadmap
const greeting = 'Hello, World!';
console.log(greeting);
CS-Notes doesn't typically include code snippets, instead focusing on theoretical concepts and explanations.
Summary
developer-roadmap offers a comprehensive visual guide for aspiring developers, covering various technologies and career paths. It's particularly useful for those looking to understand the broader landscape of software development. CS-Notes, in contrast, provides more in-depth technical content, focusing on computer science fundamentals and interview preparation. The choice between the two depends on whether you're seeking a broad overview of development paths or deep dives into CS concepts.
📝 Algorithms and data structures implemented in JavaScript with explanations and links to further readings
Pros of javascript-algorithms
- Focused specifically on JavaScript implementations of algorithms and data structures
- Includes explanations and examples for each algorithm
- Actively maintained with frequent updates
Cons of javascript-algorithms
- Limited to JavaScript, while CS-Notes covers a broader range of topics
- Less comprehensive in terms of general computer science concepts
- May not be as suitable for interview preparation across multiple languages
Code Comparison
CS-Notes (Java):
public class BinarySearch {
public int binarySearch(int[] nums, int target) {
int left = 0, right = nums.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (nums[mid] == target) return mid;
if (nums[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
}
javascript-algorithms (JavaScript):
function binarySearch(array, target) {
let left = 0;
let right = array.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (array[mid] === target) return mid;
if (array[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
Both repositories provide implementations of common algorithms, but CS-Notes offers a broader range of computer science topics in multiple languages, while javascript-algorithms focuses solely on JavaScript implementations with detailed explanations.
:books: Freely available programming books
Pros of free-programming-books
- Extensive collection of free programming resources across multiple languages and topics
- Community-driven project with frequent updates and contributions
- Well-organized structure, making it easy to find relevant resources
Cons of free-programming-books
- Lacks original content, primarily serving as a curated list of external resources
- May include outdated or deprecated resources if not regularly maintained
- No standardized format or quality control for listed resources
Code comparison
Not applicable, as both repositories primarily contain documentation and resource lists rather than code.
Summary
CS-Notes is a comprehensive collection of computer science and programming notes in Chinese, focusing on interview preparation and fundamental concepts. It offers original, curated content but is limited to a single language.
free-programming-books, on the other hand, is a multilingual directory of free learning resources for various programming languages and computer science topics. It provides a wider range of resources but relies on external content.
Both repositories serve different purposes and cater to different audiences. CS-Notes is ideal for Chinese-speaking developers seeking in-depth study materials, while free-programming-books is better suited for those looking for a diverse collection of free learning resources across multiple languages and topics.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
ç®æ³ | æä½ç³»ç» | ç½ç» | é¢å对象 | æ°æ®åº | Java | ç³»ç»è®¾è®¡ | å·¥å · | ç¼ç å®è·µ | åè®° |
---|---|---|---|---|---|---|---|---|---|
:pencil2: | :computer: | :cloud: | :art: | :floppy_disk: | :coffee: | :bulb: | :wrench: | :watermelon: | :memo: |
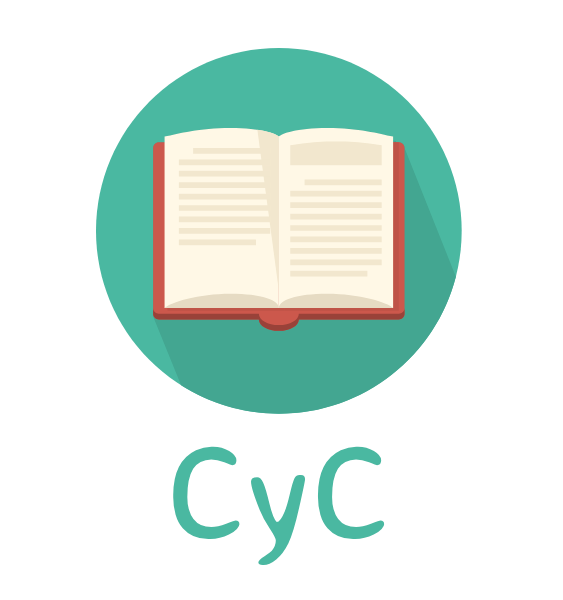
:pencil2: ç®æ³
:computer: æä½ç³»ç»
:cloud: ç½ç»
:floppy_disk: æ°æ®åº
:coffee: Java
:bulb: ç³»ç»è®¾è®¡
:art: é¢å对象
:wrench: å·¥å ·
:watermelon: ç¼ç å®è·µ
:memo: åè®°
æç
ç¬è®°å 容æç § ä¸æææ¡æçæå è¿è¡æçï¼ä»¥ä¿è¯å 容çå¯è¯»æ§ã
ä¸ä½¿ç¨ ![]()
è¿ç§æ¹å¼æ¥å¼ç¨å¾çï¼èæ¯ç¨ <img>
æ ç¾ã䏿¹é¢æ¯ä¸ºäºè½å¤æ§å¶å¾ç以åéç大尿¾ç¤ºï¼å¦ä¸æ¹é¢æ¯å 为 GFM 䏿¯æ <center> ![]() </center>
è¿ç§æ¹æ³è®©å¾çå±
䏿¾ç¤ºï¼åªè½ä½¿ç¨ <div align="center"> <img src=""/> </div>
è¾¾å°å±
ä¸çææã
å¨çº¿æçå·¥å ·ï¼Text-Typesettingã
License
æ¬ä»åºçå 容䏿¯å°ç½ä¸çèµæéææ¼åèæ¥ï¼é¤äºå°é¨åå¼ç¨ä¹¦ä¸åææ¯ææ¡£çåæï¼è¿é¨åå 容é½å¨æ«å°¾çåè龿¥ä¸å äºåºå¤ï¼ï¼å ¶ä½é½æ¯æçååã卿¨å¼ç¨æ¬ä»åºå 容æè 对å 容è¿è¡ä¿®æ¹æ¼ç»æ¶ï¼è¯·ç½²å并以ç¸åæ¹å¼å ±äº«ï¼è°¢è°¢ã
转载æç« 请å¨å¼å¤´ææ¾å¤æ æè¯¥é¡µé¢å°åï¼å ¬ä¼å·çå ¶å®è½¬è½½è¯·èç³» zhengyc101@163.comã
Logoï¼logomakr
è´è°¢
æè°¢ä»¥ä¸äººå对æ¬ä»åºååºçè´¡ç®ï¼å½ç¶ä¸ä» ä» åªæè¿äºè´¡ç®è ï¼è¿éå°±ä¸ä¸ä¸å举äºãå¦æä½ å¸æè¢«æ·»å å°è¿ä¸ªååä¸ï¼å¹¶ä¸æäº¤è¿ Issue æè PRï¼è¯·ä¸æèç³»ã
Top Related Projects
A complete computer science study plan to become a software engineer.
💯 Curated coding interview preparation materials for busy software engineers
Learn how to design large-scale systems. Prep for the system design interview. Includes Anki flashcards.
Interactive roadmaps, guides and other educational content to help developers grow in their careers.
📝 Algorithms and data structures implemented in JavaScript with explanations and links to further readings
:books: Freely available programming books
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot