stream-chat-react-native
💬 React-Native Chat SDK ➜ Stream Chat. Includes a tutorial on building your own chat app experience using React-Native, React-Navigation and Stream
Top Related Projects
💬 The most complete chat UI for React Native
🔥 A well-tested feature-rich modular Firebase implementation for React Native. Supports both iOS & Android platforms for all Firebase services.
React Native Notifications
React Native Local and Remote Notifications
A React Native wrapper around the Facebook SDKs for Android and iOS. Provides access to Facebook login, sharing, graph requests, app events etc.
Quick Overview
Stream Chat React Native is an official UI component library for building chat applications in React Native using the Stream Chat API. It provides a set of customizable components and hooks to easily implement real-time messaging features in mobile apps.
Pros
- Comprehensive set of pre-built UI components for chat functionality
- Highly customizable with theming and component overrides
- Built-in support for real-time updates and offline mode
- Extensive documentation and examples
Cons
- Learning curve for developers new to Stream Chat API
- Limited flexibility for complex custom layouts
- Dependency on Stream Chat backend services
- Potential performance issues with large message lists
Code Examples
- Initializing the Chat client:
import { StreamChat } from 'stream-chat';
import { Chat } from 'stream-chat-react-native';
const client = StreamChat.getInstance('YOUR_API_KEY');
// In your app component
<Chat client={client}>
{/* Your chat components */}
</Chat>
- Rendering a channel list:
import { ChannelList } from 'stream-chat-react-native';
const filters = { members: { $in: [userId] } };
const sort = { last_message_at: -1 };
<ChannelList
filters={filters}
sort={sort}
onSelect={(channel) => setSelectedChannel(channel)}
/>
- Displaying a message list and input:
import { Channel, MessageList, MessageInput } from 'stream-chat-react-native';
<Channel channel={selectedChannel}>
<MessageList />
<MessageInput />
</Channel>
Getting Started
-
Install the package:
npm install stream-chat-react-native
-
Initialize the Chat client in your app:
import { StreamChat } from 'stream-chat'; import { Chat } from 'stream-chat-react-native'; const client = StreamChat.getInstance('YOUR_API_KEY'); function App() { return ( <Chat client={client}> {/* Your chat components */} </Chat> ); }
-
Add chat components like ChannelList, Channel, MessageList, and MessageInput to your app structure.
-
Customize the appearance and behavior of components using props and theming options as needed.
Competitor Comparisons
💬 The most complete chat UI for React Native
Pros of react-native-gifted-chat
- Simpler setup and integration for basic chat functionality
- Lightweight and focused solely on chat UI components
- More customizable out-of-the-box with easier theming options
Cons of react-native-gifted-chat
- Limited built-in features compared to stream-chat-react-native
- Lacks advanced functionality like real-time presence and typing indicators
- No built-in support for file attachments or rich media messages
Code Comparison
react-native-gifted-chat:
import { GiftedChat } from 'react-native-gifted-chat'
<GiftedChat
messages={this.state.messages}
onSend={messages => this.onSend(messages)}
user={{
_id: 1,
}}
/>
stream-chat-react-native:
import { Chat, Channel, MessageList, MessageInput } from 'stream-chat-react-native'
<Chat client={chatClient}>
<Channel channel={channel}>
<MessageList />
<MessageInput />
</Channel>
</Chat>
The code comparison shows that react-native-gifted-chat offers a more compact implementation for basic chat functionality, while stream-chat-react-native provides a more structured approach with separate components for different chat elements, allowing for greater flexibility and feature-rich implementations.
🔥 A well-tested feature-rich modular Firebase implementation for React Native. Supports both iOS & Android platforms for all Firebase services.
Pros of react-native-firebase
- Comprehensive Firebase integration: Offers a wide range of Firebase services, including authentication, real-time database, cloud functions, and more
- Active community and frequent updates: Regularly maintained with contributions from a large developer base
- Native implementation: Provides better performance and deeper integration with device-specific features
Cons of react-native-firebase
- Steeper learning curve: Requires understanding of Firebase ecosystem and its various services
- Potentially higher resource usage: Full Firebase integration may lead to larger app size and increased battery consumption
- Less focused on chat functionality: Requires additional work to implement chat features compared to stream-chat-react-native
Code Comparison
stream-chat-react-native (Chat-specific implementation):
import { StreamChat } from 'stream-chat';
import { Chat, Channel, MessageList, MessageInput } from 'stream-chat-react-native';
const client = StreamChat.getInstance('API_KEY');
client.connectUser({ id: 'user_id' }, 'user_token');
<Chat client={client}>
<Channel channel={channel}>
<MessageList />
<MessageInput />
</Channel>
</Chat>
react-native-firebase (Firebase Realtime Database for chat):
import database from '@react-native-firebase/database';
const reference = database().ref('/messages');
reference.on('value', snapshot => {
const messages = snapshot.val();
// Handle messages
});
reference.push({
text: 'New message',
user: 'user_id',
timestamp: database.ServerValue.TIMESTAMP,
});
React Native Notifications
Pros of react-native-notifications
- Focused specifically on push notifications, providing a more specialized solution
- Offers deeper integration with native iOS and Android notification systems
- Supports advanced notification features like custom sounds and actions
Cons of react-native-notifications
- Limited to notification functionality, lacking chat-specific features
- Requires more setup and configuration for basic notification handling
- May need additional libraries for complete chat implementation
Code Comparison
react-native-notifications:
import NotificationsIOS from 'react-native-notifications';
NotificationsIOS.addEventListener('notificationReceived', (notification) => {
console.log("Notification received:", notification);
});
stream-chat-react-native:
import { StreamChat } from 'stream-chat';
import { Chat, Channel, MessageList } from 'stream-chat-react-native';
const client = StreamChat.getInstance('API_KEY');
client.connectUser({ id: 'user_id' }, 'user_token');
Summary
react-native-notifications is a specialized library for handling push notifications in React Native apps, offering deep integration with native notification systems. It's ideal for projects that require advanced notification features but may need additional libraries for full chat functionality.
stream-chat-react-native, on the other hand, provides a comprehensive solution for building chat interfaces, including built-in components and notification handling. It's more suitable for projects that need a complete chat experience out of the box, but may be overkill for simple notification requirements.
The choice between these libraries depends on the specific needs of your project, whether you're focusing solely on notifications or require a full-featured chat solution.
React Native Local and Remote Notifications
Pros of react-native-push-notification
- Lightweight and focused solely on push notifications
- Supports both iOS and Android platforms
- Easier to integrate for simple notification requirements
Cons of react-native-push-notification
- Limited to push notifications, lacking chat functionality
- Requires additional setup for advanced features
- Less frequent updates and maintenance
Code Comparison
react-native-push-notification:
PushNotification.configure({
onNotification: function (notification) {
console.log("NOTIFICATION:", notification);
},
permissions: {
alert: true,
badge: true,
sound: true,
},
});
stream-chat-react-native:
import { StreamChat } from 'stream-chat';
import { Chat, Channel, MessageList } from 'stream-chat-react-native';
const client = StreamChat.getInstance('API_KEY');
client.connectUser({ id: 'user_id' }, 'user_token');
<Chat client={client}>
<Channel channel={channel}>
<MessageList />
</Channel>
</Chat>
stream-chat-react-native offers a comprehensive chat solution with built-in UI components and real-time messaging capabilities. It provides a more robust and feature-rich environment for developing chat applications, including message threading, reactions, and file attachments. However, it may be overkill for projects that only require simple push notifications.
react-native-push-notification is better suited for applications that need basic notification functionality without the overhead of a full chat system. It's more lightweight and easier to implement for simple use cases, but lacks the advanced features and pre-built UI components offered by stream-chat-react-native.
A React Native wrapper around the Facebook SDKs for Android and iOS. Provides access to Facebook login, sharing, graph requests, app events etc.
Pros of react-native-fbsdk
- Officially supported by Facebook, ensuring compatibility with the latest Facebook SDK features
- Comprehensive integration with Facebook's ecosystem, including login, sharing, and analytics
- Well-documented and widely adopted in the React Native community
Cons of react-native-fbsdk
- Limited to Facebook-specific functionality, not suitable for general chat applications
- Requires more setup and configuration compared to stream-chat-react-native
- Less frequent updates and maintenance as it's now archived
Code Comparison
stream-chat-react-native:
import { StreamChat } from 'stream-chat';
import { Chat, Channel, MessageList, MessageInput } from 'stream-chat-react-native';
const client = StreamChat.getInstance('API_KEY');
react-native-fbsdk:
import { LoginButton, AccessToken } from 'react-native-fbsdk';
<LoginButton
onLoginFinished={(error, result) => {
if (error) {
console.log("login has error: " + result.error);
} else if (result.isCancelled) {
console.log("login is cancelled.");
} else {
AccessToken.getCurrentAccessToken().then(
(data) => {
console.log(data.accessToken.toString())
}
)
}
}}
/>
The code snippets demonstrate the different focus of each library. stream-chat-react-native provides a ready-to-use chat interface, while react-native-fbsdk focuses on Facebook-specific features like login functionality.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Official React Native SDK for Stream Chat
The official React Native and Expo components for Stream Chat, a service for building chat applications.
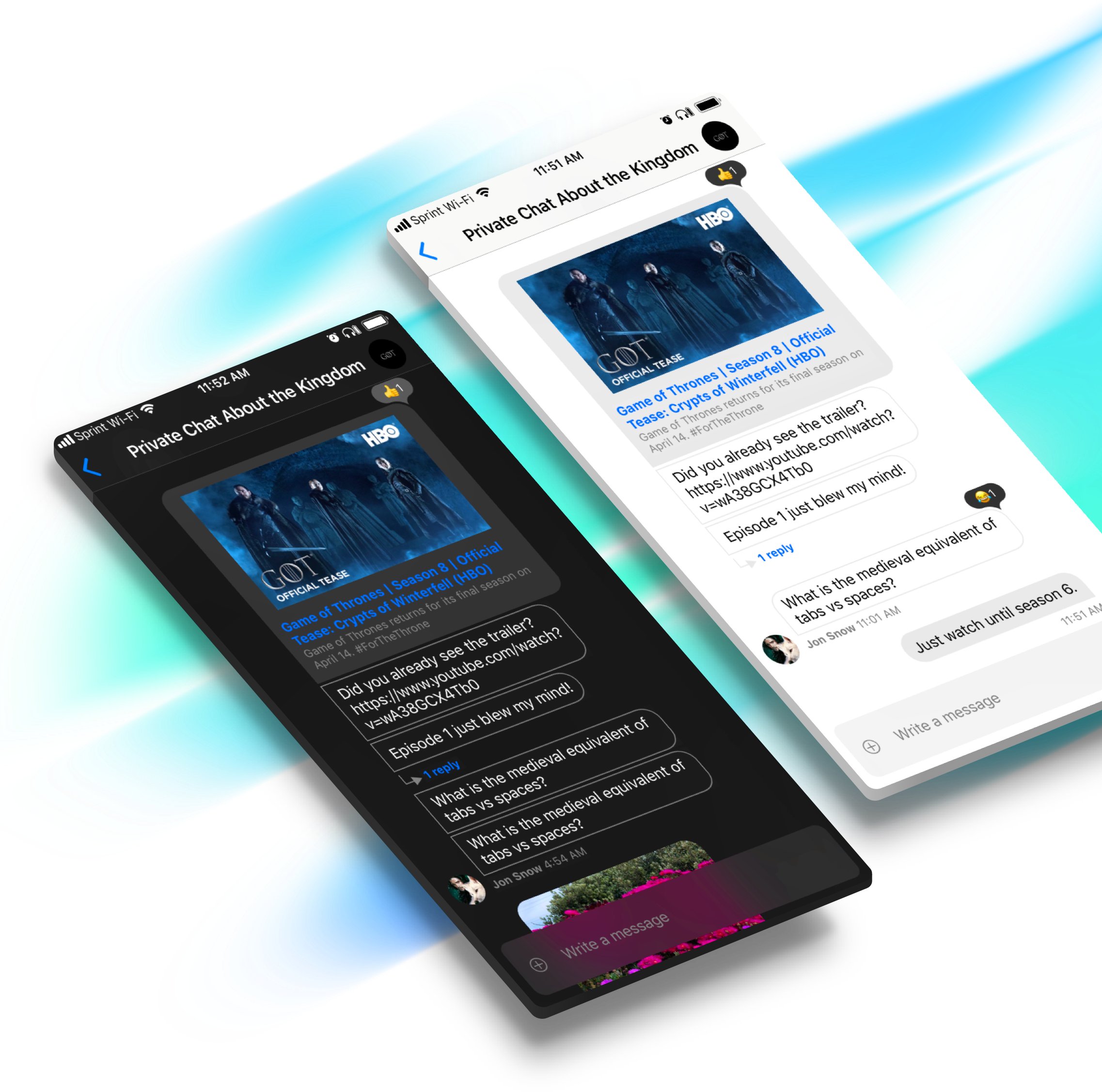
Quick Links
- Stream Chat API product overview
- Register to get an API key for Stream Chat
- React Native Chat Tutorial
- Chat UI Kit
- Documentation
- Release Notes
Contents
ð React Native Chat Tutorial
The best place to start is the React Native Chat Tutorial. It teaches you how to use this SDK and also shows how to make frequently required changes.
Free for Makers
Stream is free for most side and hobby projects. To qualify your project/company needs to have < 5 team members and < $10k in monthly revenue. For complete pricing details visit our Chat Pricing Page
ð® Example Apps
This repo includes 3 example apps. One made with Expo, two in TypeScript. One TypeScript app is a simple implementation for reference, the other is a more full featured app example.
Besides, our team maintains a dedicated repository for fully-fledged sample applications and demos at GetStream/react-native-samples. Please consider checking following sample applications:
ð¬ Keep in mind
-
Navigation between different components is something we expect consumers to implement. You can check out the example given in this repository
-
Minor releases may come with some breaking changes, so always check the release notes before upgrading the minor version.
You can see detailed documentation about the components at https://getstream.io/chat/docs/sdk/reactnative/
ð Contributing
We welcome code changes that improve this library or fix a problem, and please make sure to follow all best practices and test all the changes. Please check our dev setup docs to get you started. We are pleased to merge your code into the official repository. Make sure to sign our Contributor License Agreement (CLA) first. See our license file for more details.
Git flow & Release process
We enforce conventional commits and have an automated releasing process using workspaces and semantic-release. Read our git flow & release process guide for more information
We are hiring
We've recently closed a $38 million Series B funding round and we keep actively growing. Our APIs are used by more than a billion end-users, and you'll have a chance to make a huge impact on the product within a team of the strongest engineers all over the world.
Check out our current openings and apply via Stream's website.
Top Related Projects
💬 The most complete chat UI for React Native
🔥 A well-tested feature-rich modular Firebase implementation for React Native. Supports both iOS & Android platforms for all Firebase services.
React Native Notifications
React Native Local and Remote Notifications
A React Native wrapper around the Facebook SDKs for Android and iOS. Provides access to Facebook login, sharing, graph requests, app events etc.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot