Top Related Projects
It's online. It's offline. It's a Service Worker!
✈️ Easily create sites that work offline as well as online
https://github.com/facebookincubator/create-react-app + Progressive Web App goodness
Webpack plugin that generates a service worker using sw-precache that will cache webpack's bundles' emitted assets. You can optionally pass sw-precache configuration options to webpack through this plugin.
Offline plugin (ServiceWorker, AppCache) for webpack (https://webpack.js.org/)
IndexedDB, but with promises
Quick Overview
Workbox is a set of JavaScript libraries for adding offline support to web apps. It provides a collection of tools and strategies for caching assets, handling background sync, and managing service workers, making it easier for developers to create Progressive Web Apps (PWAs) with robust offline capabilities.
Pros
- Easy to use and integrate into existing web projects
- Provides a variety of caching strategies to suit different use cases
- Offers a modular approach, allowing developers to use only the features they need
- Regularly updated and maintained by Google, ensuring compatibility with modern web standards
Cons
- Learning curve for developers new to service workers and PWAs
- May add unnecessary complexity for simple websites that don't require extensive offline functionality
- Some advanced features might require additional configuration and understanding of browser behavior
- Limited browser support for older versions of Internet Explorer and other legacy browsers
Code Examples
- Registering a service worker:
import { Workbox } from 'workbox-window';
if ('serviceWorker' in navigator) {
const wb = new Workbox('/service-worker.js');
wb.register();
}
- Caching static assets:
import { precacheAndRoute } from 'workbox-precaching';
precacheAndRoute([
{ url: '/styles/main.css', revision: '1' },
{ url: '/images/logo.png', revision: null }
]);
- Implementing a network-first caching strategy:
import { registerRoute } from 'workbox-routing';
import { NetworkFirst } from 'workbox-strategies';
registerRoute(
({ request }) => request.destination === 'image',
new NetworkFirst()
);
Getting Started
To get started with Workbox, follow these steps:
-
Install Workbox via npm:
npm install workbox-cli --global
-
Generate a service worker configuration:
workbox wizard
-
Build your service worker:
workbox generateSW workbox-config.js
-
Register the service worker in your main JavaScript file:
if ('serviceWorker' in navigator) { window.addEventListener('load', () => { navigator.serviceWorker.register('/service-worker.js'); }); }
-
Customize your service worker as needed using Workbox modules and strategies.
Competitor Comparisons
It's online. It's offline. It's a Service Worker!
Pros of serviceworker-cookbook
- Provides a wide range of practical examples and recipes for different Service Worker use cases
- Focuses on educational content, making it easier for developers to learn and understand Service Worker concepts
- Maintained by Mozilla Developer Network (MDN), a trusted source for web development documentation
Cons of serviceworker-cookbook
- Less comprehensive tooling compared to Workbox
- May require more manual implementation and configuration for complex use cases
- Not as actively maintained or updated as Workbox
Code Comparison
serviceworker-cookbook example (basic caching):
self.addEventListener('fetch', function(event) {
event.respondWith(
caches.match(event.request).then(function(response) {
return response || fetch(event.request);
})
);
});
Workbox example (basic caching):
importScripts('https://storage.googleapis.com/workbox-cdn/releases/6.1.5/workbox-sw.js');
workbox.routing.registerRoute(
({request}) => request.destination === 'image',
new workbox.strategies.CacheFirst()
);
The serviceworker-cookbook provides more basic examples, while Workbox offers a higher-level API with built-in strategies and easier configuration for common use cases.
✈️ Easily create sites that work offline as well as online
Pros of UpUp
- Simpler and more lightweight, focusing specifically on offline functionality
- Easier to set up and use for basic offline capabilities
- Better suited for smaller projects or those with minimal offline requirements
Cons of UpUp
- Less feature-rich compared to Workbox's comprehensive service worker toolkit
- Limited customization options for advanced caching strategies
- Less active development and community support
Code Comparison
UpUp basic setup:
UpUp.start({
'content-url': 'offline.html',
'assets': ['images/logo.png', 'css/style.css']
});
Workbox basic setup:
importScripts('https://storage.googleapis.com/workbox-cdn/releases/6.1.5/workbox-sw.js');
workbox.routing.registerRoute(
({request}) => request.destination === 'image',
new workbox.strategies.CacheFirst()
);
UpUp provides a simpler API for basic offline functionality, while Workbox offers more granular control over caching strategies and service worker behavior. Workbox is better suited for larger, more complex applications requiring advanced offline capabilities, while UpUp is ideal for quickly adding basic offline support to smaller projects.
https://github.com/facebookincubator/create-react-app + Progressive Web App goodness
Pros of create-react-pwa
- Specifically tailored for React applications, providing a streamlined setup process
- Includes a pre-configured service worker and manifest file for PWA functionality
- Offers a simpler learning curve for developers already familiar with Create React App
Cons of create-react-pwa
- Less flexible compared to Workbox, with fewer customization options
- Limited to React projects, while Workbox can be used with various frameworks
- May not receive as frequent updates or have as large a community as Workbox
Code Comparison
create-react-pwa:
import { precacheAndRoute } from 'workbox-precaching';
import { registerRoute } from 'workbox-routing';
import { StaleWhileRevalidate } from 'workbox-strategies';
precacheAndRoute(self.__WB_MANIFEST);
registerRoute(
({request}) => request.destination === 'image',
new StaleWhileRevalidate()
);
Workbox:
importScripts('https://storage.googleapis.com/workbox-cdn/releases/6.1.5/workbox-sw.js');
workbox.routing.registerRoute(
({request}) => request.destination === 'image',
new workbox.strategies.CacheFirst()
);
workbox.precaching.precacheAndRoute(self.__WB_MANIFEST);
Both examples demonstrate similar functionality, but Workbox offers more flexibility in configuration and strategy selection.
Webpack plugin that generates a service worker using sw-precache that will cache webpack's bundles' emitted assets. You can optionally pass sw-precache configuration options to webpack through this plugin.
Pros of sw-precache-webpack-plugin
- Specifically designed for webpack integration, making it easier to use in webpack-based projects
- Simpler configuration for basic service worker caching needs
- Lightweight and focused on a single task (precaching)
Cons of sw-precache-webpack-plugin
- Less flexible and feature-rich compared to Workbox
- Limited to precaching, lacking advanced caching strategies and runtime caching options
- No longer actively maintained, with the last update in 2018
Code Comparison
sw-precache-webpack-plugin:
new SWPrecacheWebpackPlugin({
cacheId: 'my-app-cache',
filename: 'service-worker.js',
staticFileGlobs: ['dist/**/*.{js,html,css,png,jpg,gif}'],
minify: true,
});
Workbox:
new WorkboxWebpackPlugin.GenerateSW({
clientsClaim: true,
skipWaiting: true,
runtimeCaching: [{
urlPattern: /\.(?:png|jpg|jpeg|svg)$/,
handler: 'CacheFirst',
}],
});
The sw-precache-webpack-plugin is simpler to configure for basic precaching needs, while Workbox offers more advanced features like runtime caching strategies. Workbox is actively maintained and provides a more comprehensive solution for service worker management in modern web applications.
Offline plugin (ServiceWorker, AppCache) for webpack (https://webpack.js.org/)
Pros of offline-plugin
- Simpler setup and configuration for basic use cases
- Tighter integration with webpack, making it easier to use in webpack-based projects
- Automatic generation of ServiceWorker and AppCache files
Cons of offline-plugin
- Less flexible and customizable compared to Workbox
- Limited to webpack projects, while Workbox is framework-agnostic
- Fewer advanced features and caching strategies available
Code Comparison
offline-plugin configuration:
new OfflinePlugin({
safeToUseOptionalCaches: true,
caches: {
main: ['index.html', 'app.js', 'app.css'],
additional: ['*.chunk.js']
}
})
Workbox configuration:
workbox.routing.registerRoute(
/\.(?:js|css)$/,
new workbox.strategies.StaleWhileRevalidate({
cacheName: 'static-resources',
})
);
Both offline-plugin and Workbox aim to simplify the process of adding offline capabilities to web applications. offline-plugin is more focused on webpack integration and offers a simpler setup for basic use cases. Workbox, on the other hand, provides more flexibility, advanced features, and can be used with various build tools and frameworks. The choice between the two depends on the project's specific requirements and the desired level of customization.
IndexedDB, but with promises
Pros of idb
- Focused specifically on IndexedDB, providing a simpler and more intuitive API
- Lightweight and minimal, with a smaller bundle size
- Promises-based API, making it easier to work with asynchronous operations
Cons of idb
- Limited scope compared to Workbox's comprehensive service worker toolset
- Lacks built-in caching strategies and offline support features
- May require additional libraries or custom code for full PWA functionality
Code Comparison
idb:
import { openDB } from 'idb';
const db = await openDB('myDatabase', 1, {
upgrade(db) {
db.createObjectStore('myStore');
},
});
Workbox:
import { registerRoute } from 'workbox-routing';
import { CacheFirst } from 'workbox-strategies';
registerRoute(
({ request }) => request.destination === 'image',
new CacheFirst()
);
Summary
idb is a focused library for working with IndexedDB, offering a simpler API and smaller footprint. Workbox, on the other hand, provides a comprehensive set of tools for service workers and PWAs, including caching strategies and offline support. The choice between the two depends on the specific needs of your project and the level of PWA functionality required.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
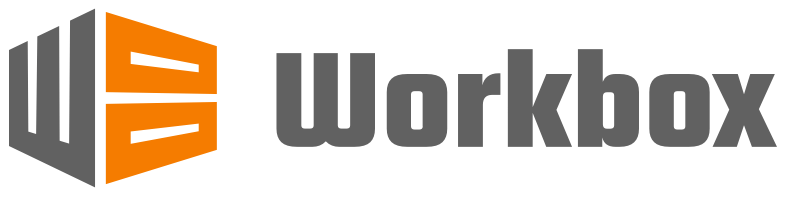
Welcome to Workbox!
Workbox is a collection of JavaScript libraries for Progressive Web Apps.
Documentation
Maintenance update
Workbox is a powerful library originally developed by members of Chrome's developer relations team to facilitate the creation of Progressive Web Apps and to improve the offline experience of web applications. It offers a suite of tools and strategies for efficiently caching and serving web assets, managing service workers, and handling offline scenarios. Workbox simplifies the implementation of common caching patterns and provides developers with a comprehensive toolkit to build robust, resilient web applications. From now on, Chrome's Aurora team will be the new owners of Workbox.
Contributing
Development happens in the open on GitHub. We're thankful to the community for contributing any improvements.
Please read the guide to contributing for information about setting up your environment and other requirements prior to filing any pull requests.
License
MIT. See LICENSE for details.
Top Related Projects
It's online. It's offline. It's a Service Worker!
✈️ Easily create sites that work offline as well as online
https://github.com/facebookincubator/create-react-app + Progressive Web App goodness
Webpack plugin that generates a service worker using sw-precache that will cache webpack's bundles' emitted assets. You can optionally pass sw-precache configuration options to webpack through this plugin.
Offline plugin (ServiceWorker, AppCache) for webpack (https://webpack.js.org/)
IndexedDB, but with promises
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot