SPlisHSPlasH
SPlisHSPlasH is an open-source library for the physically-based simulation of fluids.
Top Related Projects
PositionBasedDynamics is a library for the physically-based simulation of rigid bodies, deformable solids and fluids.
The FLIP Fluids addon is a tool that helps you set up, run, and render high quality liquid fluid effects all within Blender, the free and open source 3D creation suite.
Fluid simulation engine for computer graphics applications
Quick Overview
SPlisHSPlasH is an open-source library for physically-based simulation of fluids and related phenomena. It implements various Smoothed Particle Hydrodynamics (SPH) methods for simulating incompressible and compressible fluids, as well as additional techniques for rigid body coupling, surface tension, and more. The library is designed to be efficient and extensible, making it suitable for both research and practical applications in computer graphics and animation.
Pros
- Comprehensive implementation of multiple SPH methods and related techniques
- Efficient C++ codebase with CUDA acceleration for improved performance
- Extensible architecture allowing for easy integration of new simulation methods
- Includes a GUI for visualization and interaction with simulations
Cons
- Steep learning curve for users unfamiliar with SPH methods and fluid simulation
- Limited documentation for some advanced features and customization options
- Requires significant computational resources for large-scale simulations
- Dependency on external libraries may complicate setup and cross-platform compatibility
Code Examples
- Creating a fluid simulation scene:
SimulationBase *sim = new SimulationBase();
FluidModel *fluid = sim->addFluidModel("Fluid", 2.0);
fluid->setViscosity(0.01);
fluid->setDensity0(1000);
- Adding boundary objects to the simulation:
BoundaryModel_Akinci2012 *boundary = new BoundaryModel_Akinci2012();
boundary->loadObj("boundary.obj");
sim->addBoundaryModel(boundary);
- Performing a simulation step:
TimeStep *timeStep = new TimeStep();
timeStep->step(sim);
- Accessing particle positions:
const std::vector<Vector3r>& positions = fluid->getPositions();
for (const auto& pos : positions) {
// Process particle positions
}
Getting Started
To get started with SPlisHSPlasH:
-
Clone the repository:
git clone https://github.com/InteractiveComputerGraphics/SPlisHSPlasH.git
-
Install dependencies (CMake, Eigen3, etc.) as specified in the documentation.
-
Build the project:
mkdir build cd build cmake .. make
-
Run an example simulation:
./bin/SPHSimulator ../data/scenes/DamBreak.json
For more detailed instructions and advanced usage, refer to the project's documentation and examples in the repository.
Competitor Comparisons
PositionBasedDynamics is a library for the physically-based simulation of rigid bodies, deformable solids and fluids.
Pros of PositionBasedDynamics
- More versatile, handling various simulation types (cloth, deformables, fluids)
- Simpler implementation for certain physics scenarios
- Potentially faster for non-fluid simulations
Cons of PositionBasedDynamics
- Less specialized for fluid dynamics compared to SPlisHSPlasH
- May require more manual tuning for fluid-like behavior
- Potentially less accurate for complex fluid interactions
Code Comparison
PositionBasedDynamics (cloth simulation):
Vector3r corr = stiffness * (restLength - (p1 - p2).norm()) * (p1 - p2).normalized();
p1 += invMass1 * corr;
p2 -= invMass2 * corr;
SPlisHSPlasH (SPH fluid simulation):
Vector3r gradW = sim->gradW(xi - xj);
Vector3r a = -m_mass * (pi / (density_i * density_i) + pj / (density_j * density_j)) * gradW;
ai += a;
aj -= a;
Both libraries offer physics-based simulations, but SPlisHSPlasH focuses on fluid dynamics using Smoothed Particle Hydrodynamics (SPH), while PositionBasedDynamics provides a broader range of simulations. SPlisHSPlasH is likely more accurate and efficient for fluid simulations, whereas PositionBasedDynamics offers greater flexibility for various physics scenarios. The code snippets demonstrate the different approaches: PositionBasedDynamics uses position-based constraints, while SPlisHSPlasH employs SPH calculations for fluid behavior.
The FLIP Fluids addon is a tool that helps you set up, run, and render high quality liquid fluid effects all within Blender, the free and open source 3D creation suite.
Pros of Blender-FLIP-Fluids
- Seamless integration with Blender, providing a user-friendly interface for fluid simulations
- Extensive documentation and tutorials, making it accessible for beginners
- Optimized for GPU acceleration, resulting in faster simulation times
Cons of Blender-FLIP-Fluids
- Limited to Blender environment, reducing flexibility for use in other applications
- Less customizable than SPlisHSPlasH, which offers more control over simulation parameters
- Focuses primarily on FLIP method, while SPlisHSPlasH supports multiple fluid simulation techniques
Code Comparison
SPlisHSPlasH:
SimulationDataDFSPH *sim = new SimulationDataDFSPH();
sim->init();
TimeStep *ts = new TimeStepDFSPH();
ts->step(sim);
Blender-FLIP-Fluids:
bpy.ops.flip_fluid_operators.add_domain()
domain = bpy.context.scene.objects.active
domain.flip_fluid.domain.resolution = 100
bpy.ops.flip_fluid_operators.bake_fluid()
The code snippets demonstrate the different approaches: SPlisHSPlasH uses C++ for low-level control, while Blender-FLIP-Fluids leverages Blender's Python API for a more user-friendly experience.
Fluid simulation engine for computer graphics applications
Pros of fluid-engine-dev
- More comprehensive, covering a wider range of fluid simulation techniques
- Extensive documentation and educational resources, including a companion book
- Supports both 2D and 3D simulations
Cons of fluid-engine-dev
- Less focused on real-time performance compared to SPlisHSPlasH
- May have a steeper learning curve due to its broader scope
- Not as specialized in SPH (Smoothed Particle Hydrodynamics) methods
Code Comparison
SPlisHSPlasH (C++):
Vector3r Simulation::computeXSPHViscosity(const unsigned int i)
{
const Real h = m_supportRadius;
const Real h2 = h*h;
const Real m = m_masses[i];
Vector3r sum = Vector3r::Zero();
for (unsigned int j = 0; j < m_neighbors[i].size(); j++)
{
const unsigned int neighborIndex = m_neighbors[i][j];
// ... (calculation continues)
}
return sum;
}
fluid-engine-dev (C++):
Vector3D SphSystemData3::smoothedVelocity(size_t i) const {
Vector3D sum = Vector3D();
SphStdKernel3 kernel(kernelRadius());
const auto& neighbors = neighborLists()[i];
for (size_t j : neighbors) {
Vector3D dist = positions()[j] - positions()[i];
// ... (calculation continues)
}
return sum;
}
Both repositories implement fluid simulation algorithms, but fluid-engine-dev offers a more diverse set of tools and techniques, while SPlisHSPlasH focuses on optimized SPH methods for real-time applications.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
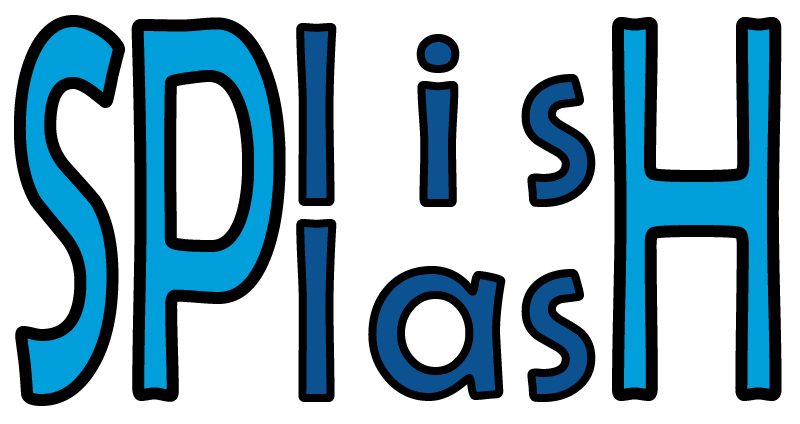
SPlisHSPlasH is an open-source library for the physically-based simulation of fluids. The simulation in this library is based on the Smoothed Particle Hydrodynamics (SPH) method which is a popular meshless Lagrangian approach to simulate complex fluid effects. The SPH formalism allows an efficient computation of a certain quantity of a fluid particle by considering only a finite set of neighboring particles. One of the most important research topics in the field of SPH methods is the simulation of incompressible fluids. SPlisHSPlasH implements current state-of-the-art pressure solvers (WCSPH, PCISPH, PBF, IISPH, DFSPH, PF) to simulate incompressibility. Moreover, the library provides different methods to simulate viscosity, surface tension and vorticity.
The library uses the following external libraries: Eigen, json, partio, zlib, cxxopts, tinyexpr, toojpeg, pybind, glfw, hapPLY, nfd, and imgui. All external dependencies are included.
Furthermore we use our own libraries:
- PositionBasedDynamics to simulate dynamic rigid bodies
- Discregrid to detect collisions between rigid bodies
- CompactNSearch to perform the neighborhood search
- cuNSearch to perform the neighborhood search on the GPU
- GenericParameters to handle generic parameters
SPlisHSPlasH can export the particle data in the partio and vtk format. If you want to import partio files in Maya or Blender, try out our plugins:
Author: Jan Bender
License
The SPlisHSPlasH library code is licensed under the MIT license. See LICENSE for details.
External dependencies are covered by separate licensing terms. See the extern folder for the code and respective licensing terms of each dependency.
Documentation
Forum
On our GitHub discussions page you can ask questions, discuss about simulation topics, and share ideas.
Build Instructions
This project is based on CMake. Simply generate project, Makefiles, etc. using CMake and compile the project with a compiler of your choice that supports C++11. The code was tested with the following configurations:
- Windows 10 64-bit, CMake 3.18.3, Visual Studio 2019
- Debian 11.5 64-bit, CMake 3.18.4, GCC 10.2.1.
Note: Please use a 64-bit target on a 64-bit operating system. 32-bit builds on a 64-bit OS are not supported.
Python Installation Instruction
For Windows and Linux targets there exists prebuilt python wheel files which can be installed using
pip install pysplishsplash
These are available for Python versions 3.6-3.10. See also here: pySPlisHSPlasH. If you do not meet these conditions please refer to the build instructions and to the python binding Getting started guide.
The command line simulator is available by running one of the following
splash
splash --help
Features
SPlisHSPlasH implements:
- an open-source SPH fluid simulation (2D & 3D)
- neighborhood search on CPU or GPU
- supports vectorization using AVX
- Python binding (thanks to Stefan Jeske)
- supports embedded Python scripts
- several implicit pressure solvers (WCSPH, PCISPH, PBF, IISPH, DFSPH, PF)
- explicit and implicit viscosity methods
- current surface tension approaches
- different vorticity methods
- computation of drag forces
- support for multi-phase simulations
- simulation of deformable solids
- rigid-fluid coupling with static and dynamic bodies
- two-way coupling with deformable solids
- XSPH velocity filter
- fluid emitters
- scripted animation fields
- a json-based scene file importer
- automatic surface sampling
- a tool for volume sampling of closed geometries
- a tool to generate spray, foam and bubble particles in a postprocessing step
- a tool to skin a visual mesh to the moving particles of an elastic solid in a postprocessing step
- partio file export of all particle data
- VTK file export of all particle data (enables the data import in ParaView)
- rigid body export
- a Maya plugin to model and generate scene files
- a ParaView plugin to import particle data
A list of all implemented simulation methods can be found here: https://splishsplash.physics-simulation.org/features
Screenshots & Videos
https://splishsplash.physics-simulation.org/gallery
Citation
To cite SPlisHSPlasH you can use this BibTeX entry:
@software{SPlisHSPlasH_Library,
author = {Bender, Jan and others},
license = {MIT},
title = {{SPlisHSPlasH Library}},
url = {https://github.com/InteractiveComputerGraphics/SPlisHSPlasH},
}
Top Related Projects
PositionBasedDynamics is a library for the physically-based simulation of rigid bodies, deformable solids and fluids.
The FLIP Fluids addon is a tool that helps you set up, run, and render high quality liquid fluid effects all within Blender, the free and open source 3D creation suite.
Fluid simulation engine for computer graphics applications
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot