Top Related Projects
Getting Started with Spring Boot 3:
about learning Spring Boot via examples. Spring Boot 教程、技术栈示例代码,快速简单上手教程。
《Spring Boot基础教程》,2.x版本持续连载中!点击下方链接直达教程目录!
🚀一个用来深入学习并实战 Spring Boot 的项目。
Understand and love the power of Spring Boot - All its features are illustrated developing a web application managing todos and a basic API for survey questionnaire. Also covers unit testing, mocking and integration testing.
Quick Overview
The springboot-learning-example
repository is a comprehensive collection of Spring Boot-based projects and examples, created by Jeff Li. It serves as a learning resource for developers who want to explore and understand the capabilities of the Spring Boot framework.
Pros
- Extensive Coverage: The repository covers a wide range of Spring Boot-related topics, including web development, security, data access, messaging, and more.
- Beginner-Friendly: The examples are well-documented and provide a step-by-step approach, making it easier for beginners to understand and learn Spring Boot.
- Active Maintenance: The repository is actively maintained, with regular updates and bug fixes, ensuring the examples remain relevant and up-to-date.
- Diverse Functionality: The projects within the repository demonstrate various use cases and best practices for Spring Boot, catering to different development needs.
Cons
- Overwhelming for Beginners: The sheer number of projects and examples in the repository may be overwhelming for complete beginners, who might find it challenging to navigate and prioritize their learning.
- Potential Outdated Versions: While the repository is actively maintained, some of the examples may use older versions of Spring Boot or related technologies, which could require additional effort to update.
- Lack of Comprehensive Tutorials: While the examples are well-documented, the repository may lack more in-depth, step-by-step tutorials that guide users through the entire development process.
- Limited Project-Specific Guidance: The repository focuses on individual examples, and may not provide comprehensive guidance on how to integrate these examples into a larger, real-world application.
Code Examples
Since this repository is a collection of Spring Boot-based projects and examples, it does not contain a single, cohesive code library. However, here are a few examples of the types of code you might find in the repository:
Example 1: Simple Web Application
@SpringBootApplication
public class SimpleWebApplication {
public static void main(String[] args) {
SpringApplication.run(SimpleWebApplication.class, args);
}
@RestController
public class HelloController {
@GetMapping("/")
public String hello() {
return "Hello, Spring Boot!";
}
}
}
This example demonstrates a basic Spring Boot web application that exposes a single REST endpoint, returning a "Hello, Spring Boot!" message.
Example 2: Security Configuration
@Configuration
@EnableWebSecurity
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.logout();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}password").roles("ADMIN");
}
}
This example demonstrates a basic Spring Security configuration, where the application has two roles (ADMIN
and USER
) and the access to different endpoints is controlled based on the user's role.
Getting Started
To get started with the springboot-learning-example
repository, follow these steps:
-
Clone the repository to your local machine:
git clone https://github.com/JeffLi1993/springboot-learning-example.git
-
Navigate to the project directory:
cd springboot-learning-example
-
Explore the different submodules and projects within the repository, each of which demonstrates a specific aspect of Spring Boot development.
-
Choose a project that interests you and navigate to its directory:
cd project-directory
-
Review the project's README file, which should provide detailed instructions on how to build, run, and explore the example.
-
Follow the instructions in the README to set up the project, which may involve installing dependencies, configuring the database, or running the application.
-
Once
Competitor Comparisons
Getting Started with Spring Boot 3:
Pros of tutorials
- Covers a wider range of topics beyond Spring Boot, including other Java frameworks and libraries
- More comprehensive and regularly updated with new content
- Larger community and more contributors, leading to diverse examples and use cases
Cons of tutorials
- Can be overwhelming due to the sheer volume of content
- May lack the focused, step-by-step approach of springboot-learning-example
- Some examples might be more complex or advanced for beginners
Code Comparison
springboot-learning-example:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
tutorials:
@SpringBootApplication
@EnableJpaRepositories("com.baeldung.repository")
@EntityScan("com.baeldung.entity")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
The tutorials example includes additional annotations for JPA configuration, demonstrating a more complex setup compared to the simpler springboot-learning-example.
about learning Spring Boot via examples. Spring Boot 教程、技术栈示例代码,快速简单上手教程。
Pros of spring-boot-examples
- More comprehensive coverage of Spring Boot topics, including advanced concepts
- Better organized structure with clear categorization of examples
- More frequent updates and active maintenance
Cons of spring-boot-examples
- May be overwhelming for beginners due to the large number of examples
- Some examples lack detailed explanations or comments
Code Comparison
springboot-learning-example:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
spring-boot-examples:
@SpringBootApplication
@EnableScheduling
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
The main difference in the code snippets is that spring-boot-examples includes the @EnableScheduling
annotation, demonstrating more advanced features from the start.
Both repositories provide valuable resources for learning Spring Boot, but spring-boot-examples offers a more extensive and up-to-date collection of examples. However, springboot-learning-example might be more suitable for beginners due to its simpler structure and focused examples.
《Spring Boot基础教程》,2.x版本持续连载中!点击下方链接直达教程目录!
Pros of SpringBoot-Learning
- More comprehensive coverage of Spring Boot topics, including advanced concepts
- Better organized structure with clear categorization of examples
- More frequent updates and active maintenance
Cons of SpringBoot-Learning
- Less beginner-friendly, with fewer explanations for basic concepts
- Larger codebase may be overwhelming for newcomers to Spring Boot
Code Comparison
SpringBoot-Learning:
@SpringBootApplication
@EnableScheduling
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
springboot-learning-example:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
The main difference in the code snippets is that SpringBoot-Learning includes the @EnableScheduling
annotation, indicating more advanced features are covered in this repository.
Both repositories provide valuable resources for learning Spring Boot, but SpringBoot-Learning offers a more comprehensive and up-to-date learning experience, while springboot-learning-example may be more suitable for beginners due to its simpler structure and explanations.
🚀一个用来深入学习并实战 Spring Boot 的项目。
Pros of spring-boot-demo
- More comprehensive coverage of Spring Boot features and integrations
- Better organized structure with clear module separation
- More frequent updates and active maintenance
Cons of spring-boot-demo
- Potentially overwhelming for beginners due to its extensive scope
- Less focused on step-by-step learning compared to springboot-learning-example
Code Comparison
spring-boot-demo:
@SpringBootApplication
@EnableScheduling
public class SpringBootDemoTaskApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootDemoTaskApplication.class, args);
}
}
springboot-learning-example:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
The spring-boot-demo example includes additional annotations like @EnableScheduling
, demonstrating more advanced configurations out of the box.
Both repositories serve as valuable resources for learning Spring Boot, with spring-boot-demo offering a more extensive range of examples and integrations, while springboot-learning-example provides a more gradual learning curve for beginners. The choice between them depends on the user's experience level and specific learning goals.
Understand and love the power of Spring Boot - All its features are illustrated developing a web application managing todos and a basic API for survey questionnaire. Also covers unit testing, mocking and integration testing.
Pros of spring-boot-master-class
- More comprehensive coverage of Spring Boot topics, including microservices and cloud deployment
- Better organized structure with clear sections for different skill levels
- Includes video course materials and additional resources for learning
Cons of spring-boot-master-class
- Less focus on specific Spring Boot features and configurations
- Fewer code examples for immediate implementation
- May be overwhelming for absolute beginners due to its breadth of content
Code Comparison
springboot-learning-example:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
spring-boot-master-class:
@RestController
@SpringBootApplication
public class CourseApiApp {
public static void main(String[] args) {
SpringApplication.run(CourseApiApp.class, args);
}
}
The spring-boot-master-class example includes the @RestController
annotation, indicating a focus on RESTful web services, while the springboot-learning-example keeps it simpler with just the basic @SpringBootApplication
annotation.
Both repositories offer valuable resources for learning Spring Boot, but they cater to different learning styles and depth of coverage. springboot-learning-example provides more targeted examples, while spring-boot-master-class offers a broader curriculum with additional learning materials.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
ä¸ãæ¯ææ³¥ç¦å
å ³æ³¨æ³¥ç¦å 个人åå®¢çæ´æ°ï¼æçå客 - å享å¦ä¹ å¯è½å°çææ¯åæ
Spring Boot 2.x ç³»åæç¨ï¼spring boot å®è·µå¦ä¹ æ¡ä¾ï¼æ¯åå¦è åæ ¸å¿ææ¯å·©åºçæä½³å®è·µã
- æ¿èµ·å¾®ä¿¡ï¼å ³æ³¨å ¬ä¼å·ï¼ãç¨åºåæ³¥ç¦å ã
- ç»æç¨ç弿ºä»£ç ä»åºç¹ä¸ª Star å§
- 帮å¿å享该系åæç« 龿¥ç»æ´å¤çæå
äºãç³»åæç« ç®å½
ã Spring Boot 2 å¿«éæç¨ ã
- Spring Boot 2 å¿«éæç¨ï¼WebFlux éæ Thymeleafï¼äºï¼
- Spring Boot 2 å¿«éæç¨ï¼WebFlux éæ Mongodbï¼åï¼
- Spring Boot 2 å¿«éæç¨ï¼WebFlux Restful CRUD å®è·µï¼ä¸ï¼
- Spring Boot 2 å¿«éæç¨ï¼WebFlux å¿«éå ¥é¨ï¼äºï¼
- Spring Boot 2 å¿«éæç¨ï¼WebFlux REST API å ¨å±å¼å¸¸å¤ç Error Handling
- Spring Boot 2 å¿«éæç¨ï¼WebFlux ç³»åæç¨å¤§çº²ï¼ä¸ï¼
ã åºç¡ - å ¥é¨ç¯ ã
- Spring Boot 2.0 é ç½®å¾ææç¨
- Spring Boot 2.0 çå¿«éå ¥é¨ï¼å¾ææç¨ï¼
- Spring Boot ä¹ HelloWorld 详解
- Spring Boot ä¹é ç½®æä»¶è¯¦è§£
ã åºç¡ - Web ä¸å¡å¼åç¯ ã
- Spring Boot Web å¼å注解ç¯
- Spring Boot 表åéªè¯ç¯
- Spring Boot 2.x å°æ°åè½ â Spring Data Web configuration
- Spring Boot å®ç° Restful æå¡ï¼åºäº HTTP / JSON ä¼ è¾
- Spring Boot ä¹ RESRful API æéæ§å¶
- Spring Boot éæ FreeMarker
- Spring Boot HTTP over JSON çé误ç å¼å¸¸å¤ç
- Spring Boot ä½¿ç¨ Swagger2 æå»º RESRful API ææ¡£
- Spring Boot éæ JSP
- Spring Boot éæ Thymeleaf
- Spring Boot åå æµè¯ç使ç¨
- Spring Boot çæ´æ°é¨ç½²
ã åºç¡ â æ°æ®åå¨ç¯ ã
- Spring Boot æ´å Mybatis ç宿´ Web æ¡ä¾
- Spring Boot æ´å Mybatis Annotation 注解æ¡ä¾
- Spring Boot æ´å Mybatis å®ç° Druid 夿°æ®æºé ç½®
- Spring Boot æ´åä½¿ç¨ JdbcTemplate
- Spring Boot æ´å Spring-data-jpa
- Spring Boot 声æå¼äºå¡ç®¡ç
ã åºç¡ â æ°æ®ç¼åç¯ ã
- Spring Boot æ´å Redis å®ç°ç¼åæä½
- Spring Boot æ´å Redis Annotation å®ç°ç¼åæä½
- Spring Boot æ´å MongoDB å®ç°ç¼åæä½
- Spring Boot æ´å EhCache å®ç°ç¼åæä½
ã åºç¡ â æ¥å¿ç®¡çç¯ ã
- Spring Boot é»è®¤æ¥å¿ logback é 置解æ
- Spring Boot ä½¿ç¨ log4j è®°å½æ¥å¿
- Spring Boot 对 log4j è¿è¡å¤ç¯å¢ä¸åæ¥å¿çº§å«çæ§å¶
- Spring Boot ä½¿ç¨ log4j è®°å½æ¥å¿å° MongoDB
- Spring Boot 1.5.x å¨æä¿®æ¹æ¥å¿çº§å«
ã åºç¡ â åºç¨ç¯ ã
- Spring Boot Actuator çæ§
- Spring Boot Web åºç¨é¨ç½²
ã æå â å®å ¨æ§å¶åæéç¯ ã
- Spring Boot æ´å Spring Security
- Spring Boot æ´å Shiro
- Spring Boot æ´å Spring Session
ã æå â ä¸é´ä»¶ç¯ ã
- Spring Boot 2.x ï¼éè¿ spring-boot-starter-hbase éæ HBase
- Spring Boot æ´å RabbitMQ
- Spring Boot æ´å Quartz
ã æå â æºç ç¯ ã
- Spring Boot å¯å¨åçè§£æ
ã Elasticsearch ç¯ ã
- Spring Boot æ´å Elasticsearch
- æ·±å ¥æµ åº spring-data-elasticsearch ä¹ ElasticSearch æ¶æåæ¢ï¼ä¸ï¼
- æ·±å ¥æµ åº spring-data-elasticsearch ç³»å â æ¦è¿°åå ¥é¨ï¼äºï¼
- æ·±å ¥æµ åº spring-data-elasticsearch â åºæ¬æ¡ä¾è¯¦è§£ï¼ä¸ï¼
- æ·±å ¥æµ åº spring-data-elasticsearch â 宿æ¡ä¾è¯¦è§£ï¼åï¼
ã Dubbo ç¯ ã
- Spring Boot æ´å Dubbo/ZooKeeper 详解 SOA æ¡ä¾
- Spring Boot ä¸å¦ä½ä½¿ç¨ Dubbo Activate æ©å±ç¹
- Spring Boot Dubbo applications.properties é ç½®æ¸ å
ä¸ãæåæ¨è
- æçå客ï¼å享å¦ä¹ å¯è½å°çææ¯åæ
- æçGitHubï¼Follow ä¸å
- æçGiteeï¼Follow ä¸å
- Springé®ç社åºï¼å¦ææ¨æä»ä¹é®é¢ï¼å¯ä»¥å»è¿éåå¸
åãæçå ¬å·
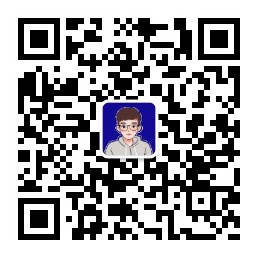
Top Related Projects
Getting Started with Spring Boot 3:
about learning Spring Boot via examples. Spring Boot 教程、技术栈示例代码,快速简单上手教程。
《Spring Boot基础教程》,2.x版本持续连载中!点击下方链接直达教程目录!
🚀一个用来深入学习并实战 Spring Boot 的项目。
Understand and love the power of Spring Boot - All its features are illustrated developing a web application managing todos and a basic API for survey questionnaire. Also covers unit testing, mocking and integration testing.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot