Top Related Projects
FNA - Accuracy-focused XNA4 reimplementation for open platforms
A simple and easy-to-use library to enjoy videogames programming
Godot Engine – Multi-platform 2D and 3D game engine
Desktop/Android/HTML5/iOS Java game development framework
Simple and Fast Multimedia Library
Cocos2d-x is a suite of open-source, cross-platform, game-development tools utilized by millions of developers across the globe. Its core has evolved to serve as the foundation for Cocos Creator 1.x & 2.x.
Quick Overview
MonoGame is an open-source implementation of the Microsoft XNA 4 Framework. It allows developers to create cross-platform games using C# and other .NET languages. MonoGame supports a wide range of platforms, including Windows, macOS, Linux, iOS, Android, and various gaming consoles.
Pros
- Cross-platform development: Create games that run on multiple platforms with a single codebase
- Familiar XNA-like API: Easy transition for developers with XNA experience
- Active community and ongoing development: Regular updates and improvements
- Extensive documentation and resources available
Cons
- Steeper learning curve compared to some other game engines
- Limited built-in visual tools for level design and asset management
- Performance may not be as optimized as native game engines for specific platforms
- Some platforms require additional setup or licenses for deployment
Code Examples
- Initializing a basic MonoGame project:
public class Game1 : Game
{
private GraphicsDeviceManager _graphics;
private SpriteBatch _spriteBatch;
public Game1()
{
_graphics = new GraphicsDeviceManager(this);
Content.RootDirectory = "Content";
}
protected override void Initialize()
{
// TODO: Add your initialization logic here
base.Initialize();
}
}
- Loading and drawing a texture:
private Texture2D _texture;
protected override void LoadContent()
{
_spriteBatch = new SpriteBatch(GraphicsDevice);
_texture = Content.Load<Texture2D>("myTexture");
}
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.CornflowerBlue);
_spriteBatch.Begin();
_spriteBatch.Draw(_texture, new Vector2(100, 100), Color.White);
_spriteBatch.End();
base.Draw(gameTime);
}
- Handling input:
protected override void Update(GameTime gameTime)
{
if (GamePad.GetState(PlayerIndex.One).Buttons.Back == ButtonState.Pressed || Keyboard.GetState().IsKeyDown(Keys.Escape))
Exit();
// TODO: Add your update logic here
base.Update(gameTime);
}
Getting Started
- Install Visual Studio or your preferred IDE with .NET support
- Install the MonoGame extension for Visual Studio or the MonoGame SDK
- Create a new MonoGame project using the provided templates
- Add your game logic to the
Update
method and rendering code to theDraw
method - Use the Content Pipeline to import and manage game assets
- Build and run your game on your desired platform
For detailed instructions, refer to the official MonoGame documentation at https://docs.monogame.net/
Competitor Comparisons
FNA - Accuracy-focused XNA4 reimplementation for open platforms
Pros of FNA
- More accurate XNA implementation, leading to better compatibility with existing XNA games
- Simpler codebase, making it easier to maintain and debug
- Better performance in some scenarios due to its lightweight nature
Cons of FNA
- Smaller community and ecosystem compared to MonoGame
- Less extensive platform support, focusing mainly on desktop platforms
- Fewer built-in tools and content pipeline options
Code Comparison
MonoGame:
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.CornflowerBlue);
spriteBatch.Begin();
spriteBatch.Draw(texture, position, Color.White);
spriteBatch.End();
base.Draw(gameTime);
}
FNA:
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.CornflowerBlue);
spriteBatch.Begin();
spriteBatch.Draw(texture, position, Color.White);
spriteBatch.End();
}
The code structure is very similar between MonoGame and FNA, as both aim to replicate the XNA framework. The main difference in this example is that MonoGame calls base.Draw(gameTime)
at the end, while FNA doesn't require this call.
A simple and easy-to-use library to enjoy videogames programming
Pros of raylib
- Lightweight and easy to learn, with a simple C API
- Cross-platform support for desktop, web, and mobile
- Built-in support for 3D rendering and physics
Cons of raylib
- Less extensive ecosystem and community compared to MonoGame
- Limited support for advanced graphics features and shaders
- Fewer tools and editors available for game development
Code Comparison
MonoGame:
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.CornflowerBlue);
spriteBatch.Begin();
spriteBatch.Draw(texture, position, Color.White);
spriteBatch.End();
base.Draw(gameTime);
}
raylib:
void DrawGame(void)
{
BeginDrawing();
ClearBackground(RAYWHITE);
DrawTexture(texture, position.x, position.y, WHITE);
EndDrawing();
}
Both MonoGame and raylib are popular game development frameworks, but they cater to different audiences. MonoGame is more suited for C# developers and offers a larger ecosystem, while raylib provides a simpler, lightweight alternative with a focus on ease of use and cross-platform support. The choice between the two depends on the developer's preferences, programming language familiarity, and project requirements.
Godot Engine – Multi-platform 2D and 3D game engine
Pros of Godot
- Complete game engine with built-in editor, scene system, and asset management
- Supports multiple programming languages (GDScript, C#, C++)
- More extensive built-in features for 2D and 3D game development
Cons of Godot
- Steeper learning curve due to its comprehensive nature
- Less flexibility for low-level engine customization
- Potentially higher resource usage for simpler projects
Code Comparison
MonoGame (C#):
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.CornflowerBlue);
spriteBatch.Begin();
spriteBatch.Draw(texture, position, Color.White);
spriteBatch.End();
base.Draw(gameTime);
}
Godot (GDScript):
func _draw():
draw_texture(texture, position)
MonoGame is a framework that provides a lower-level API for game development, requiring more manual setup but offering greater control. Godot is a full-featured game engine with a visual editor and scene system, making it easier to create complex games quickly but with less low-level control. MonoGame uses C# exclusively, while Godot supports multiple languages, with GDScript being its primary scripting language.
Desktop/Android/HTML5/iOS Java game development framework
Pros of libGDX
- Cross-platform development for desktop, Android, iOS, and web
- Rich ecosystem with numerous extensions and third-party libraries
- Flexible and lightweight, allowing low-level access to the hardware
Cons of libGDX
- Steeper learning curve, especially for beginners
- Less suitable for 3D game development compared to MonoGame
- Limited built-in UI tools, often requiring third-party solutions
Code Comparison
MonoGame (C#):
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.CornflowerBlue);
spriteBatch.Begin();
spriteBatch.Draw(texture, position, Color.White);
spriteBatch.End();
base.Draw(gameTime);
}
libGDX (Java):
@Override
public void render() {
Gdx.gl.glClearColor(0, 0, 0.2f, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
batch.begin();
batch.draw(texture, position.x, position.y);
batch.end();
}
Both frameworks offer similar functionality for 2D game development, but MonoGame is more focused on C# and XNA-style development, while libGDX provides a more flexible, Java-based approach with broader platform support.
Simple and Fast Multimedia Library
Pros of SFML
- Lower-level API, offering more control over graphics and audio
- Lighter weight and faster performance for simple 2D games
- Supports multiple programming languages (C++, C#, Python, etc.)
Cons of SFML
- Less comprehensive than MonoGame, lacking built-in game-specific features
- Smaller community and ecosystem compared to MonoGame
- Limited 3D support, primarily focused on 2D graphics
Code Comparison
SFML (C++):
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Window");
while (window.isOpen()) {
window.clear();
window.display();
}
return 0;
}
MonoGame (C#):
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
public class Game1 : Game {
protected override void Draw(GameTime gameTime) {
GraphicsDevice.Clear(Color.CornflowerBlue);
base.Draw(gameTime);
}
}
Both SFML and MonoGame are popular game development frameworks, but they cater to different needs. SFML provides a more low-level approach, giving developers greater control over graphics and audio, while MonoGame offers a higher-level API with more built-in game-specific features. SFML is generally lighter and faster for simple 2D games, while MonoGame provides better support for complex games and 3D graphics.
Cocos2d-x is a suite of open-source, cross-platform, game-development tools utilized by millions of developers across the globe. Its core has evolved to serve as the foundation for Cocos Creator 1.x & 2.x.
Pros of cocos2d-x
- Multi-platform support: iOS, Android, Windows, macOS, and more
- Rich set of built-in UI controls and effects
- Extensive documentation and large community support
Cons of cocos2d-x
- Steeper learning curve, especially for C++ beginners
- Larger project size and potentially slower compile times
- Less integration with modern game development tools
Code Comparison
MonoGame (C#):
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.CornflowerBlue);
spriteBatch.Begin();
spriteBatch.Draw(texture, position, Color.White);
spriteBatch.End();
base.Draw(gameTime);
}
cocos2d-x (C++):
void HelloWorld::draw(Renderer *renderer, const Mat4 &transform, uint32_t flags)
{
Director::getInstance()->pushMatrix(MATRIX_STACK_TYPE::MATRIX_STACK_MODELVIEW);
Director::getInstance()->loadMatrix(MATRIX_STACK_TYPE::MATRIX_STACK_MODELVIEW, transform);
sprite->draw(renderer, transform, flags);
Director::getInstance()->popMatrix(MATRIX_STACK_TYPE::MATRIX_STACK_MODELVIEW);
}
Both frameworks offer robust game development capabilities, but MonoGame provides a more streamlined experience for C# developers, while cocos2d-x offers greater flexibility and performance optimization options for C++ developers.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
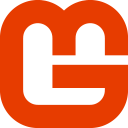
MonoGame
One framework for creating powerful cross-platfrom games
Supported Platforms ⢠Resources ⢠Samples ⢠Support and Contributions ⢠Source Code ⢠Helpful Links ⢠License
Overview
MonoGame is a simple and powerful .NET framework for creating games for desktop PCs, video game consoles, and mobile devices using the C# programming language. It has been successfully used to create games such as Streets of Rage 4, Carrion, Celeste, Stardew Valley, and many others.
It is an open-source re-implementation of the discontinued Microsoft's XNA Framework.
Supported Platforms
We support a growing list of platforms across the desktop, mobile, and console space. If there is a platform we don't support, please make a request or come help us add it.
- Desktop PCs
- Windows 8.1 and up (OpenGL & DirectX)
- Linux (OpenGL)
- macOS 10.15 and up (OpenGL)
- Mobile/Tablet Devices
- Android 6.0 and up (OpenGL)
- iPhone/iPad 10.0 and up (OpenGL)
- Consoles (for registered developers)
- PlayStation 4
- PlayStation 5
- Xbox One (XDK only) (GDK coming soon)
- Nintendo Switch
Resources
- Getting started â
- "How To" Guides â
- Documentation Hub â
- API Reference â
- Community Tutorials â
Samples
Check out the awesome game samples maintained by the MonoGame team:
Platformer 2D Sample | NeonShooter |
---|---|
Supported on all platforms | Supported on all platforms |
![]() | ![]() |
The Platformer 2D sample is a basic 2D platformer pulled from the original XNA samples and upgraded for MonoGame. | Neon Shooter Is a graphically intensive twin-stick shooter with particle effects and save data from Michael Hoffman |
Auto Pong Sample | Ship Game 3D |
---|---|
Supported on all platforms | GL / DX / iOS / Android |
![]() | ![]() |
A short sample project showing you how to make the classic game of pong, with generated soundfx, in 300 lines of code. | 3D Ship Game (Descent clone) sample, pulled from the XNA archives and updated for MonoGame |
Support and Contributions
If you think you have found a bug or have a feature request, use our issue tracker. Before opening a new issue, please search to see if your problem has already been reported. Try to be as detailed as possible in your issue reports.
If you need help using MonoGame or have other questions we suggest you post on GitHub discussions page or Discord server. Please do not use the issue tracker for personal support requests.
If you are interested in contributing fixes or features to MonoGame, please read our contributors guide first.
Subscription
If you'd like to help the project by supporting us financially, consider supporting us via a subscription for the price of a monthly coffee.
Money goes towards hosting, new hardware and if enough people subscribe a dedicated developer.
There are several options on our Donation Page.
Source Code
The full source code is available here from GitHub:
- Clone the source:
git clone https://github.com/MonoGame/MonoGame.git
- Set up the submodules:
git submodule update --init
- Open the solution for your target platform to build the game framework.
- Open the Tools solution for your development platform to build the pipeline and content tools.
For the prerequisites for building from source, please look at the Requirements file.
A high level breakdown of the components of the framework:
- The game framework is found in MonoGame.Framework.
- The content pipeline is located in MonoGame.Framework.Content.Pipeline.
- Project templates are in Templates.
- See Tests for the framework unit tests.
- See Tools/Tests for the content pipeline and other tool tests.
- The mgcb is a command line tool for content processing.
- The mgfxc is a command line effect compiler tool.
- The mgcb-editor tool is a GUI frontend for content processing.
Helpful Links
- The official website is monogame.net.
- Our issue tracker is on GitHub.
- You can join the Discord server and chat live with the core developers and other users.
- The official documentation is on our website.
- Download release and development packages.
- Follow @MonoGameTeam on Twitter.
- Get premium content on Patreon (coming soon)
License
The MonoGame project is under the Microsoft Public License except for a few portions of the code. See the LICENSE.txt file for more details. Third-party libraries used by MonoGame are under their own licenses. Please refer to those libraries for details on the license they use.
Top Related Projects
FNA - Accuracy-focused XNA4 reimplementation for open platforms
A simple and easy-to-use library to enjoy videogames programming
Godot Engine – Multi-platform 2D and 3D game engine
Desktop/Android/HTML5/iOS Java game development framework
Simple and Fast Multimedia Library
Cocos2d-x is a suite of open-source, cross-platform, game-development tools utilized by millions of developers across the globe. Its core has evolved to serve as the foundation for Cocos Creator 1.x & 2.x.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot