Top Related Projects
Tool for producing high quality forecasts for time series data that has multiple seasonality with linear or non-linear growth.
A unified framework for machine learning with time series
A python library for user-friendly forecasting and anomaly detection on time series.
Time series forecasting with PyTorch
A Python package for Bayesian forecasting with object-oriented design and probabilistic models under the hood.
Quick Overview
Nixtla/neuralforecast is an open-source Python library for time series forecasting using neural networks. It provides a collection of state-of-the-art deep learning models specifically designed for time series prediction, along with utilities for data preprocessing, model evaluation, and result visualization.
Pros
- Offers a wide range of advanced neural network models for time series forecasting
- Provides a consistent API for easy model comparison and experimentation
- Includes built-in support for probabilistic forecasting and uncertainty quantification
- Integrates well with popular data science libraries like pandas and scikit-learn
Cons
- Requires a good understanding of deep learning concepts for optimal use
- May have a steeper learning curve compared to traditional statistical forecasting methods
- Can be computationally intensive, especially for large datasets or complex models
- Documentation might be less comprehensive compared to more established forecasting libraries
Code Examples
- Basic usage with TemporalFusionTransformer:
from neuralforecast import NeuralForecast
from neuralforecast.models import TemporalFusionTransformer
model = NeuralForecast(
models=[TemporalFusionTransformer(h=24, input_size=7)],
freq='H'
)
model.fit(df)
forecast = model.predict()
- Using multiple models for ensemble forecasting:
from neuralforecast import NeuralForecast
from neuralforecast.models import NHITS, NBEATS
model = NeuralForecast(
models=[NHITS(h=24), NBEATS(h=24)],
freq='H'
)
model.fit(df)
forecast = model.predict()
- Probabilistic forecasting with DeepAR:
from neuralforecast import NeuralForecast
from neuralforecast.models import DeepAR
model = NeuralForecast(
models=[DeepAR(h=24, input_size=7, quantiles=[0.1, 0.5, 0.9])],
freq='H'
)
model.fit(df)
forecast = model.predict()
Getting Started
To get started with Nixtla/neuralforecast:
- Install the library:
pip install neuralforecast
- Import required modules and prepare your data:
import pandas as pd
from neuralforecast import NeuralForecast
from neuralforecast.models import NHITS
# Load your time series data into a pandas DataFrame
df = pd.read_csv('your_data.csv')
- Create and fit a model:
model = NeuralForecast(
models=[NHITS(h=24, input_size=7)],
freq='H'
)
model.fit(df)
- Generate forecasts:
forecast = model.predict()
print(forecast)
Competitor Comparisons
Tool for producing high quality forecasts for time series data that has multiple seasonality with linear or non-linear growth.
Pros of Prophet
- Widely adopted and battle-tested in production environments
- Extensive documentation and community support
- Handles holidays and seasonal effects out-of-the-box
Cons of Prophet
- Less flexible for custom model architectures
- Can be slower for large-scale forecasting tasks
- Limited support for external regressors
Code Comparison
Prophet:
from fbprophet import Prophet
m = Prophet()
m.fit(df)
future = m.make_future_dataframe(periods=365)
forecast = m.predict(future)
NeuralForecast:
from neuralforecast import NeuralForecast
from neuralforecast.models import NBEATS
model = NeuralForecast(models=[NBEATS(h=24, input_size=24)])
model.fit(df)
forecast = model.predict()
NeuralForecast offers a more flexible approach to time series forecasting, leveraging neural network architectures. It provides better performance for large-scale forecasting tasks and allows for easier customization of model architectures. However, Prophet remains a solid choice for simpler forecasting tasks and benefits from its extensive documentation and community support.
A unified framework for machine learning with time series
Pros of sktime
- Broader scope covering various time series tasks beyond forecasting
- More extensive documentation and tutorials
- Larger community and ecosystem of extensions
Cons of sktime
- Steeper learning curve due to more complex architecture
- Slower performance for some tasks compared to specialized libraries
Code Comparison
sktime:
from sktime.forecasting.naive import NaiveForecaster
from sktime.datasets import load_airline
y = load_airline()
forecaster = NaiveForecaster(strategy="mean")
forecaster.fit(y)
y_pred = forecaster.predict(fh=[1,2,3])
neuralforecast:
from neuralforecast import NeuralForecast
from neuralforecast.models import NBEATS
model = NeuralForecast(models=[NBEATS(input_size=30, h=5)])
model.fit(df)
forecast = model.predict()
The code examples highlight the different approaches:
- sktime focuses on a unified interface for various forecasting methods
- neuralforecast specializes in neural network-based forecasting models
Both libraries offer powerful forecasting capabilities, but sktime provides a more comprehensive toolkit for time series analysis, while neuralforecast focuses on state-of-the-art neural network models for forecasting.
A python library for user-friendly forecasting and anomaly detection on time series.
Pros of darts
- Broader range of models and algorithms, including classical statistical methods
- More extensive documentation and tutorials
- Supports both univariate and multivariate time series forecasting
Cons of darts
- Generally slower performance, especially for large datasets
- Less focus on deep learning models compared to neuralforecast
- May require more manual feature engineering for some models
Code Comparison
darts:
from darts import TimeSeries
from darts.models import Prophet
series = TimeSeries.from_dataframe(df, 'date', 'value')
model = Prophet()
model.fit(series)
forecast = model.predict(n=30)
neuralforecast:
from neuralforecast import NeuralForecast
from neuralforecast.models import NBEATS
model = NeuralForecast(models=[NBEATS(input_size=30, h=7)])
model.fit(df)
forecast = model.predict()
Both libraries offer intuitive APIs for time series forecasting, but neuralforecast focuses more on deep learning models and provides a more streamlined approach for neural network-based forecasting. darts offers a wider variety of traditional and machine learning models, making it more versatile for different types of time series problems.
Time series forecasting with PyTorch
Pros of pytorch-forecasting
- More extensive documentation and examples
- Wider range of models and architectures available
- Better integration with PyTorch ecosystem
Cons of pytorch-forecasting
- Steeper learning curve for beginners
- Less focus on traditional statistical methods
- May be overkill for simpler forecasting tasks
Code Comparison
neuralforecast:
from neuralforecast import NeuralForecast
from neuralforecast.models import NBEATS
model = NeuralForecast(models=[NBEATS(input_size=7, h=1, max_epochs=50)])
model.fit(df)
forecast = model.predict()
pytorch-forecasting:
from pytorch_forecasting import TemporalFusionTransformer, TimeSeriesDataSet
training = TimeSeriesDataSet(
data,
time_idx="timestamp",
target="target",
group_ids=["id"],
static_categoricals=["category"],
time_varying_known_reals=["price"],
time_varying_unknown_reals=["weather"],
)
model = TemporalFusionTransformer.from_dataset(training)
model.fit(train_dataloader, val_dataloader)
predictions = model.predict(test_dataloader)
The code comparison shows that pytorch-forecasting requires more setup and configuration, but offers greater flexibility in defining the dataset and model architecture. neuralforecast provides a simpler API for quick implementation of common forecasting tasks.
A Python package for Bayesian forecasting with object-oriented design and probabilistic models under the hood.
Pros of Orbit
- Offers a wider range of probabilistic time series models, including Bayesian structural time series
- Provides built-in diagnostics and model selection tools
- Supports Bayesian inference for parameter estimation and uncertainty quantification
Cons of Orbit
- Less focus on deep learning models compared to NeuralForecast
- May have a steeper learning curve for users unfamiliar with Bayesian methods
- Potentially slower training and inference times for large-scale forecasting tasks
Code Comparison
NeuralForecast:
from neuralforecast import NeuralForecast
from neuralforecast.models import NBEATS
model = NeuralForecast(models=[NBEATS(h=24, input_size=72)])
model.fit(df)
forecast = model.predict()
Orbit:
from orbit.models import DLT
model = DLT(response_col='y', date_col='ds', seasonality=52)
model.fit(df)
prediction = model.predict(df)
Both libraries offer concise APIs for model creation, fitting, and prediction. NeuralForecast focuses on neural network-based models, while Orbit provides a broader range of traditional and Bayesian time series models. The choice between them depends on the specific forecasting needs and the user's familiarity with different modeling approaches.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Nixtla

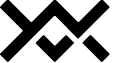
Neural ð§ Forecast
User friendly state-of-the-art neural forecasting models
NeuralForecast offers a large collection of neural forecasting models focusing on their performance, usability, and robustness. The models range from classic networks like RNNs to the latest transformers: MLP
, LSTM
, GRU
, RNN
, TCN
, TimesNet
, BiTCN
, DeepAR
, NBEATS
, NBEATSx
, NHITS
, TiDE
, DeepNPTS
, TSMixer
, TSMixerx
, MLPMultivariate
, DLinear
, NLinear
, TFT
, Informer
, AutoFormer
, FedFormer
, PatchTST
, iTransformer
, StemGNN
, and TimeLLM
.
Installation
You can install NeuralForecast
with:
pip install neuralforecast
or
conda install -c conda-forge neuralforecast
Vist our Installation Guide for further details.
Quick Start
Minimal Example
from neuralforecast import NeuralForecast
from neuralforecast.models import NBEATS
from neuralforecast.utils import AirPassengersDF
nf = NeuralForecast(
models = [NBEATS(input_size=24, h=12, max_steps=100)],
freq = 'M'
)
nf.fit(df=AirPassengersDF)
nf.predict()
Get Started with this quick guide.
Why?
There is a shared belief in Neural forecasting methods' capacity to improve forecasting pipeline's accuracy and efficiency.
Unfortunately, available implementations and published research are yet to realize neural networks' potential. They are hard to use and continuously fail to improve over statistical methods while being computationally prohibitive. For this reason, we created NeuralForecast
, a library favoring proven accurate and efficient models focusing on their usability.
Features
- Fast and accurate implementations of more than 30 state-of-the-art models. See the entire collection here.
- Support for exogenous variables and static covariates.
- Interpretability methods for trend, seasonality and exogenous components.
- Probabilistic Forecasting with adapters for quantile losses and parametric distributions.
- Train and Evaluation Losses with scale-dependent, percentage and scale independent errors, and parametric likelihoods.
- Automatic Model Selection with distributed automatic hyperparameter tuning.
- Familiar sklearn syntax:
.fit
and.predict
.
Highlights
- Official
NHITS
implementation, published at AAAI 2023. See paper and experiments. - Official
NBEATSx
implementation, published at the International Journal of Forecasting. See paper. - Unified with
StatsForecast
,MLForecast
, andHierarchicalForecast
interfaceNeuralForecast().fit(Y_df).predict()
, inputs and outputs. - Built-in integrations with
utilsforecast
andcoreforecast
for visualization and data-wrangling efficient methods. - Integrations with
Ray
andOptuna
for automatic hyperparameter optimization. - Predict with little to no history using Transfer learning. Check the experiments here.
Missing something? Please open an issue or write us in
Examples and Guides
The documentation page contains all the examples and tutorials.
ð Automatic Hyperparameter Optimization: Easy and Scalable Automatic Hyperparameter Optimization with Auto
models on Ray
or Optuna
.
ð¡ï¸ Exogenous Regressors: How to incorporate static or temporal exogenous covariates like weather or prices.
ð Transformer Models: Learn how to forecast with many state-of-the-art Transformers models.
ð Hierarchical Forecasting: forecast series with very few non-zero observations.
ð©âð¬ Add Your Own Model: Learn how to add a new model to the library.
Models
See the entire collection here.
Missing a model? Please open an issue or write us in
How to contribute
If you wish to contribute to the project, please refer to our contribution guidelines.
References
This work is highly influenced by the fantastic work of previous contributors and other scholars on the neural forecasting methods presented here. We want to highlight the work of Boris Oreshkin, Slawek Smyl, Bryan Lim, and David Salinas. We refer to Benidis et al. for a comprehensive survey of neural forecasting methods.
Contributors â¨
Thanks goes to these wonderful people (emoji key):
fede ð» ð§ |
Cristian Challu ð» ð§ |
José Morales ð» ð§ |
mergenthaler ð ð» |
Kin ð» ð ð£ |
Greg DeVos ð¤ |
Alejandro ð» |
stefanialvs ð¨ |
Ikko Ashimine ð |
vglaucus ð |
Pietro Monticone ð |
This project follows the all-contributors specification. Contributions of any kind welcome!
Top Related Projects
Tool for producing high quality forecasts for time series data that has multiple seasonality with linear or non-linear growth.
A unified framework for machine learning with time series
A python library for user-friendly forecasting and anomaly detection on time series.
Time series forecasting with PyTorch
A Python package for Bayesian forecasting with object-oriented design and probabilistic models under the hood.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot