Top Related Projects
A safe, extensible ORM and Query Builder for Rust
Next-generation ORM for Node.js & TypeScript | PostgreSQL, MySQL, MariaDB, SQL Server, SQLite, MongoDB and CockroachDB
🧰 The Rust SQL Toolkit. An async, pure Rust SQL crate featuring compile-time checked queries without a DSL. Supports PostgreSQL, MySQL, and SQLite.
Rust Compile Time ORM with Async Dynamic SQL
Powerful SQL migration toolkit for Rust.
Quick Overview
SeaORM is an async, dynamic, and lightweight Object-Relational Mapping (ORM) library for Rust. It provides a high-level abstraction for database operations, supporting multiple database backends and offering features like query building, migrations, and connection pooling.
Pros
- Asynchronous by design, providing efficient database operations
- Supports multiple database backends (MySQL, PostgreSQL, SQLite)
- Offers both active record and data mapper patterns
- Provides a powerful query builder and migration system
Cons
- Learning curve for developers new to Rust or ORMs
- Limited documentation compared to more established ORMs
- May have performance overhead compared to raw SQL in some cases
- Still relatively young, with potential for breaking changes in future versions
Code Examples
- Defining an entity:
use sea_orm::entity::prelude::*;
#[derive(Clone, Debug, PartialEq, DeriveEntityModel)]
#[sea_orm(table_name = "posts")]
pub struct Model {
#[sea_orm(primary_key)]
pub id: i32,
pub title: String,
pub text: String,
}
#[derive(Copy, Clone, Debug, EnumIter, DeriveRelation)]
pub enum Relation {}
impl ActiveModelBehavior for ActiveModel {}
- Performing a simple query:
use sea_orm::{DatabaseConnection, EntityTrait};
async fn find_post_by_id(db: &DatabaseConnection, id: i32) -> Result<Option<post::Model>, DbErr> {
post::Entity::find_by_id(id).one(db).await
}
- Inserting a new record:
use sea_orm::{ActiveModelTrait, Set};
async fn create_post(db: &DatabaseConnection, title: &str, text: &str) -> Result<post::Model, DbErr> {
let new_post = post::ActiveModel {
title: Set(title.to_owned()),
text: Set(text.to_owned()),
..Default::default()
};
new_post.insert(db).await
}
Getting Started
To start using SeaORM, add it to your Cargo.toml
:
[dependencies]
sea-orm = { version = "0.12", features = [ "sqlx-postgres", "runtime-tokio-native-tls", "macros" ] }
Then, in your Rust code:
use sea_orm::{Database, DbErr};
#[tokio::main]
async fn main() -> Result<(), DbErr> {
let db = Database::connect("postgres://username:password@localhost/database").await?;
// Use the database connection for queries
Ok(())
}
This sets up a basic connection to a PostgreSQL database. Adjust the connection string and features as needed for your specific database backend.
Competitor Comparisons
A safe, extensible ORM and Query Builder for Rust
Pros of Diesel
- More mature and battle-tested, with a larger community and ecosystem
- Offers compile-time checking of SQL queries, reducing runtime errors
- Provides a powerful query builder with type-safe expressions
Cons of Diesel
- Steeper learning curve due to its macro-heavy approach
- Limited support for async operations, primarily focused on synchronous APIs
- Requires manual schema definition and migration management
Code Comparison
Diesel query example:
let results = users
.filter(published.eq(true))
.limit(5)
.load::<Post>(&mut conn)?;
Sea-ORM query example:
let results = Post::find()
.filter(post::Column::Published.eq(true))
.limit(5)
.all(&db).await?;
Both Diesel and Sea-ORM are Rust ORMs for database operations. Diesel is more established and offers strong compile-time guarantees, while Sea-ORM provides a more familiar ActiveRecord-like API with built-in async support. Diesel's query syntax is more concise, but Sea-ORM's approach may be more intuitive for developers coming from other ORMs. The choice between them often depends on specific project requirements and developer preferences.
Next-generation ORM for Node.js & TypeScript | PostgreSQL, MySQL, MariaDB, SQL Server, SQLite, MongoDB and CockroachDB
Pros of Prisma
- More mature and widely adopted, with a larger community and ecosystem
- Supports multiple programming languages (JavaScript, TypeScript, Go)
- Offers a powerful migration system and schema versioning
Cons of Prisma
- Requires a separate schema file, which can lead to duplication
- Less flexible for complex queries and raw SQL operations
- Steeper learning curve for developers familiar with traditional ORMs
Code Comparison
Prisma:
const user = await prisma.user.create({
data: {
name: 'Alice',
email: 'alice@example.com',
},
})
Sea-ORM:
let user = User::insert(ActiveModel {
name: Set("Alice".to_owned()),
email: Set("alice@example.com".to_owned()),
..Default::default()
})
.exec(db)
.await?;
Both ORMs provide a clean and intuitive API for database operations. Prisma uses a more declarative approach, while Sea-ORM follows Rust's idiomatic patterns.
Sea-ORM is specifically designed for Rust, offering better integration with the language's features and ecosystem. It provides more fine-grained control over database operations and is generally more performant due to Rust's zero-cost abstractions.
Prisma, on the other hand, offers a more unified experience across different programming languages and has a larger set of features and integrations available out-of-the-box.
🧰 The Rust SQL Toolkit. An async, pure Rust SQL crate featuring compile-time checked queries without a DSL. Supports PostgreSQL, MySQL, and SQLite.
Pros of sqlx
- Lower-level API, offering more control and flexibility
- Supports both synchronous and asynchronous operations
- Lighter weight and potentially faster for simple queries
Cons of sqlx
- Requires more manual work for complex queries and relationships
- Less abstraction, which can lead to more boilerplate code
- Limited ORM-like features compared to Sea-ORM
Code Comparison
sqlx:
let users = sqlx::query!("SELECT * FROM users WHERE active = ?", true)
.fetch_all(&pool)
.await?;
Sea-ORM:
let users = User::find()
.filter(user::Column::Active.eq(true))
.all(&db)
.await?;
Summary
sqlx is a lower-level database library that provides more direct control over SQL queries and database operations. It's suitable for developers who prefer writing raw SQL and want fine-grained control over their database interactions.
Sea-ORM, on the other hand, is a full-featured ORM that provides a higher level of abstraction. It offers more convenience for complex queries and relationships but may have a steeper learning curve and potentially more overhead for simple operations.
The choice between the two depends on the project's requirements, the developer's preferences, and the complexity of the database interactions needed in the application.
Rust Compile Time ORM with Async Dynamic SQL
Pros of rbatis
- Written in Rust, offering better performance and memory safety
- Supports multiple databases including MySQL, PostgreSQL, and SQLite
- Provides both ORM and SQL builder functionalities
Cons of rbatis
- Less mature ecosystem compared to Sea-ORM
- Documentation may be less comprehensive or up-to-date
- Smaller community and fewer third-party integrations
Code Comparison
rbatis:
#[crud_table]
#[derive(Clone, Debug)]
pub struct User {
pub id: Option<u64>,
pub name: Option<String>,
pub age: Option<i32>,
}
let rb = Rbatis::new();
rb.link("mysql://localhost:3306/test").await?;
let user = User::select_by_column(&rb, "id", 1).await?;
Sea-ORM:
#[derive(Clone, Debug, PartialEq, DeriveEntityModel)]
#[sea_orm(table_name = "users")]
pub struct Model {
#[sea_orm(primary_key)]
pub id: i32,
pub name: String,
pub age: i32,
}
let db = Database::connect("mysql://localhost:3306/test").await?;
let user = Users::find_by_id(1).one(&db).await?;
Both ORMs provide similar functionality for defining models and querying databases. rbatis uses attributes like #[crud_table]
, while Sea-ORM uses #[sea_orm(...)]
. The syntax for connecting to databases and performing queries is slightly different, but both aim to provide a type-safe and ergonomic API for database operations in Rust.
Powerful SQL migration toolkit for Rust.
Pros of refinery
- Focused solely on database migrations, providing a lightweight and specialized solution
- Supports both embedded and CLI-based migration management
- Offers a simple and intuitive API for defining and running migrations
Cons of refinery
- Limited to migration functionality, lacking ORM features
- Smaller community and ecosystem compared to Sea-ORM
- Less comprehensive documentation and fewer examples available
Code Comparison
refinery:
use refinery::embed_migrations;
embed_migrations!("./migrations");
fn main() {
let mut conn = establish_connection();
embedded_migrations::run(&mut conn).unwrap();
}
Sea-ORM:
use sea_orm_migration::prelude::*;
#[async_std::main]
async fn main() {
let db = Database::connect("database_url").await?;
Migrator::up(&db, None).await?;
}
Sea-ORM is a full-featured ORM with migration support, while refinery focuses exclusively on migrations. Sea-ORM offers a more comprehensive solution for database interactions, including entity management and query building. refinery, being more specialized, provides a simpler API for migration-specific tasks.
Sea-ORM has a larger community, more extensive documentation, and a wider range of features. However, refinery's lightweight nature may be preferable for projects that only require migration functionality without the overhead of a full ORM.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
SeaORM
SeaORM is a relational ORM to help you build web services in Rust with the familiarity of dynamic languages.
If you like what we do, consider starring, sharing and contributing!
Please help us with maintaining SeaORM by completing the SeaQL Community Survey 2024!
Join our Discord server to chat with other members of the SeaQL community!
Getting Started
Integration examples:
- Actix v4 Example
- Axum Example
- GraphQL Example
- jsonrpsee Example
- Loco TODO Example / Loco REST Starter
- Poem Example
- Rocket Example / Rocket OpenAPI Example
- Salvo Example
- Tonic Example
- Seaography Example
Features
-
Async
Relying on SQLx, SeaORM is a new library with async support from day 1.
-
Dynamic
Built upon SeaQuery, SeaORM allows you to build complex dynamic queries.
-
Testable
Use mock connections and/or SQLite to write tests for your application logic.
-
Service Oriented
Quickly build services that join, filter, sort and paginate data in REST, GraphQL and gRPC APIs.
A quick taste of SeaORM
Entity
use sea_orm::entity::prelude::*;
#[derive(Clone, Debug, PartialEq, DeriveEntityModel)]
#[sea_orm(table_name = "cake")]
pub struct Model {
#[sea_orm(primary_key)]
pub id: i32,
pub name: String,
}
#[derive(Copy, Clone, Debug, EnumIter, DeriveRelation)]
pub enum Relation {
#[sea_orm(has_many = "super::fruit::Entity")]
Fruit,
}
impl Related<super::fruit::Entity> for Entity {
fn to() -> RelationDef {
Relation::Fruit.def()
}
}
Select
// find all models
let cakes: Vec<cake::Model> = Cake::find().all(db).await?;
// find and filter
let chocolate: Vec<cake::Model> = Cake::find()
.filter(cake::Column::Name.contains("chocolate"))
.all(db)
.await?;
// find one model
let cheese: Option<cake::Model> = Cake::find_by_id(1).one(db).await?;
let cheese: cake::Model = cheese.unwrap();
// find related models (lazy)
let fruits: Vec<fruit::Model> = cheese.find_related(Fruit).all(db).await?;
// find related models (eager)
let cake_with_fruits: Vec<(cake::Model, Vec<fruit::Model>)> =
Cake::find().find_with_related(Fruit).all(db).await?;
Insert
let apple = fruit::ActiveModel {
name: Set("Apple".to_owned()),
..Default::default() // no need to set primary key
};
let pear = fruit::ActiveModel {
name: Set("Pear".to_owned()),
..Default::default()
};
// insert one
let pear = pear.insert(db).await?;
// insert many
Fruit::insert_many([apple, pear]).exec(db).await?;
Update
use sea_orm::sea_query::{Expr, Value};
let pear: Option<fruit::Model> = Fruit::find_by_id(1).one(db).await?;
let mut pear: fruit::ActiveModel = pear.unwrap().into();
pear.name = Set("Sweet pear".to_owned());
// update one
let pear: fruit::Model = pear.update(db).await?;
// update many: UPDATE "fruit" SET "cake_id" = NULL WHERE "fruit"."name" LIKE '%Apple%'
Fruit::update_many()
.col_expr(fruit::Column::CakeId, Expr::value(Value::Int(None)))
.filter(fruit::Column::Name.contains("Apple"))
.exec(db)
.await?;
Save
let banana = fruit::ActiveModel {
id: NotSet,
name: Set("Banana".to_owned()),
..Default::default()
};
// create, because primary key `id` is `NotSet`
let mut banana = banana.save(db).await?;
banana.name = Set("Banana Mongo".to_owned());
// update, because primary key `id` is `Set`
let banana = banana.save(db).await?;
Delete
// delete one
let orange: Option<fruit::Model> = Fruit::find_by_id(1).one(db).await?;
let orange: fruit::Model = orange.unwrap();
fruit::Entity::delete(orange.into_active_model())
.exec(db)
.await?;
// or simply
let orange: Option<fruit::Model> = Fruit::find_by_id(1).one(db).await?;
let orange: fruit::Model = orange.unwrap();
orange.delete(db).await?;
// delete many: DELETE FROM "fruit" WHERE "fruit"."name" LIKE 'Orange'
fruit::Entity::delete_many()
.filter(fruit::Column::Name.contains("Orange"))
.exec(db)
.await?;
ð§ Seaography: instant GraphQL API
Seaography is a GraphQL framework built on top of SeaORM. Seaography allows you to build GraphQL resolvers quickly. With just a few commands, you can launch a GraphQL server from SeaORM entities!
Look at the Seaography Example to learn more.
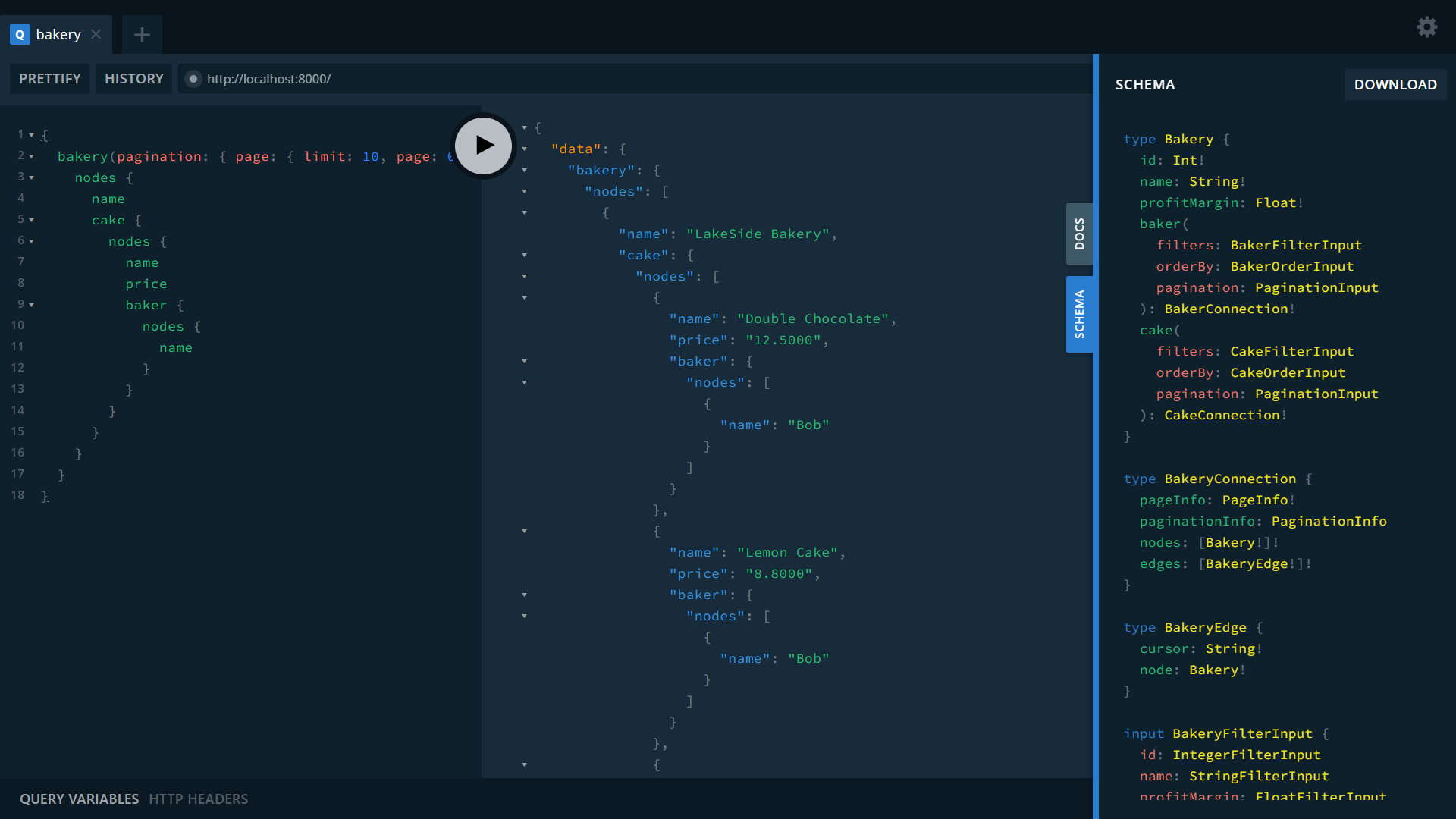
ð¥ï¸ Admin Dashboard
Making use of Seaography, it will be a breeze to create admin dashboard with your favourite frontend framework. Check out our examples and tutorials:
- React Admin Example
- Adding GraphQL Support to Loco with Seaography
- GraphQL based Admin Dashboard with Loco and Seaography
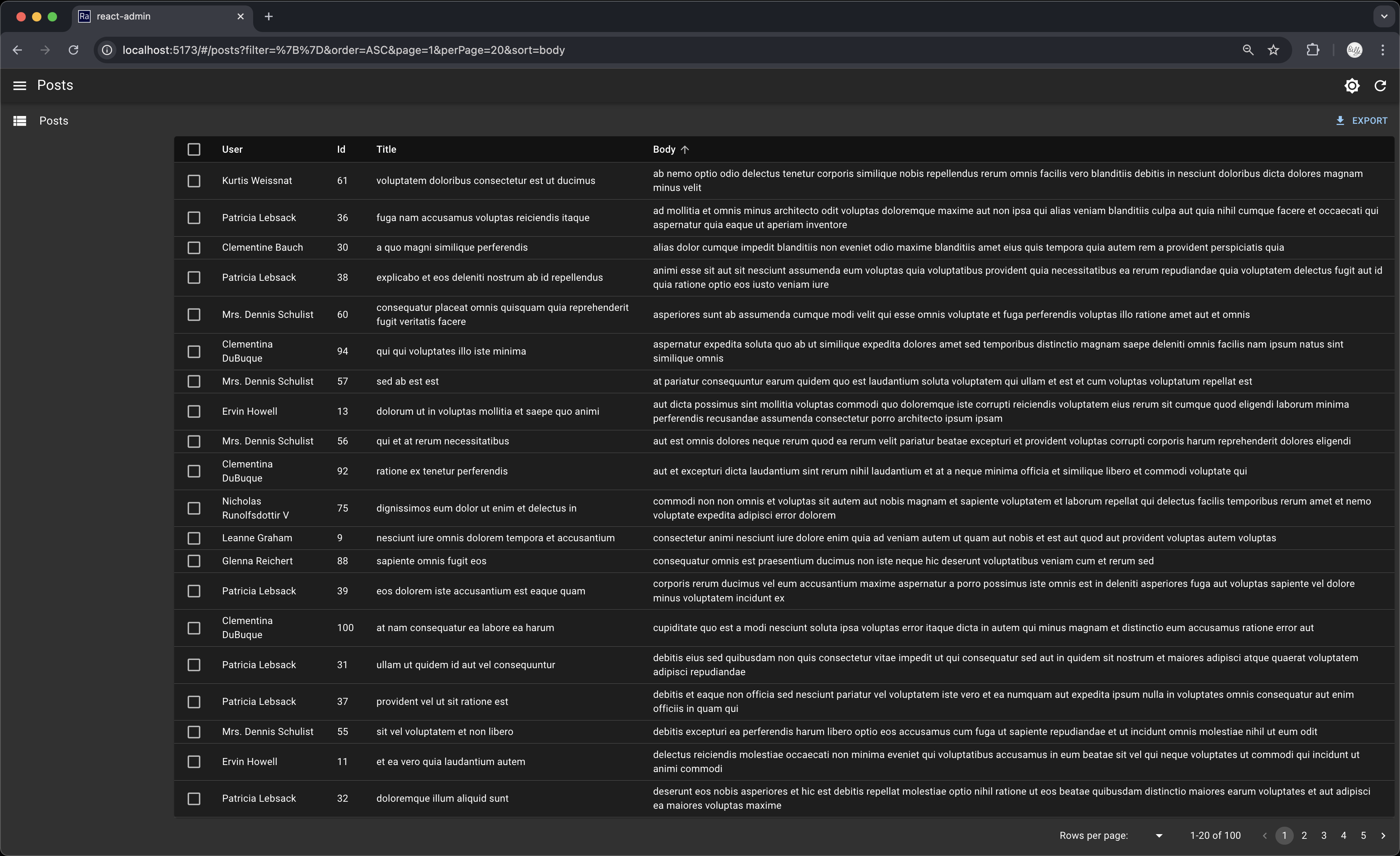
Learn More
Who's using SeaORM?
See Built with SeaORM. Feel free to submit yours!
License
Licensed under either of
- Apache License, Version 2.0 (LICENSE-APACHE or http://www.apache.org/licenses/LICENSE-2.0)
- MIT license (LICENSE-MIT or http://opensource.org/licenses/MIT)
at your option.
Contribution
Unless you explicitly state otherwise, any contribution intentionally submitted for inclusion in the work by you, as defined in the Apache-2.0 license, shall be dual licensed as above, without any additional terms or conditions.
We invite you to participate, contribute and together help build Rust's future.
A big shout out to our contributors!
Sponsorship
SeaQL.org is an independent open-source organization run by passionate developers. If you enjoy using our libraries, please star and share our repositories. If you feel generous, a small donation via GitHub Sponsor will be greatly appreciated, and goes a long way towards sustaining the organization.
Gold Sponsors
Silver Sponsors
|
|
Weâre immensely grateful to our sponsors: Osmos, for their gold-tier sponsorship and trust in the tools we develop. Digital Ocean, for sponsoring our servers. And JetBrains, for sponsoring our IDE.
Mascot
A friend of Ferris, Terres the hermit crab is the official mascot of SeaORM. His hobby is collecting shells.
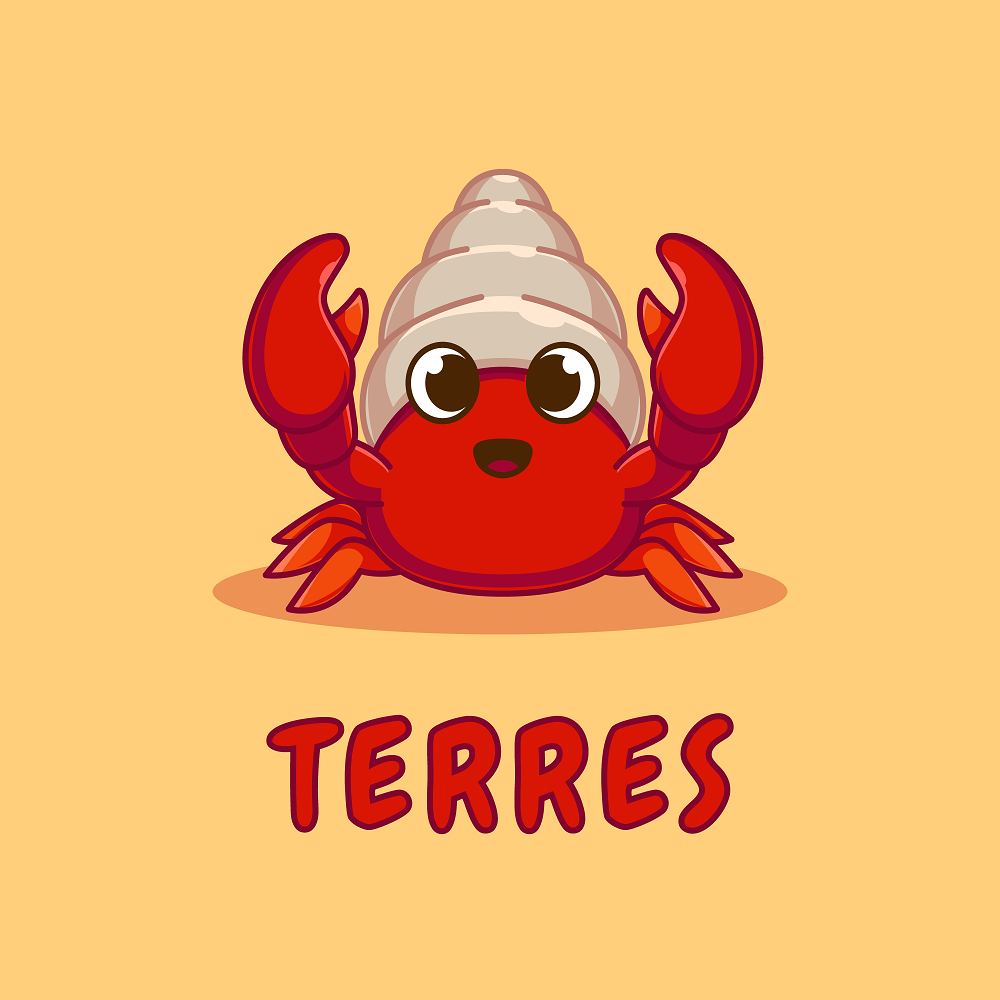
Top Related Projects
A safe, extensible ORM and Query Builder for Rust
Next-generation ORM for Node.js & TypeScript | PostgreSQL, MySQL, MariaDB, SQL Server, SQLite, MongoDB and CockroachDB
🧰 The Rust SQL Toolkit. An async, pure Rust SQL crate featuring compile-time checked queries without a DSL. Supports PostgreSQL, MySQL, and SQLite.
Rust Compile Time ORM with Async Dynamic SQL
Powerful SQL migration toolkit for Rust.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot