Top Related Projects
Fast persistent recoverable log and key-value store + cache, in C# and C++.
A library that provides an embeddable, persistent key-value store for fast storage.
LevelDB is a fast key-value storage library written at Google that provides an ordered mapping from string keys to string values.
FoundationDB - the open source, distributed, transactional key-value store
RocksDB/LevelDB inspired key-value database in Go
Core database component for the Realm Mobile Database SDKs
Quick Overview
WCDB is a high-performance, cross-platform database framework developed by Tencent. It's built on top of SQLite and provides an efficient, complete, and easy-to-use ORM for iOS, macOS, and Android. WCDB aims to provide a unified interface for database operations across different platforms.
Pros
- High performance with optimized SQL execution and multi-threading support
- Cross-platform compatibility (iOS, macOS, Android)
- Built-in encryption and full-text search capabilities
- Comprehensive ORM support with a simple and intuitive API
Cons
- Steeper learning curve compared to simpler SQLite wrappers
- Limited community support compared to more widely-used database solutions
- Potential overhead for simpler applications that don't require advanced features
- Dependency on SQLite, which may not be suitable for all use cases
Code Examples
- Creating a table and inserting data (iOS/Swift):
class Sample: TableCodable {
var identifier: Int = 0
var description: String? = nil
enum CodingKeys: String, CodingTableKey {
typealias Root = Sample
static let objectRelationalMapping = TableBinding(CodingKeys.self)
case identifier
case description
}
}
try database.create(table: Sample.self)
let object = Sample()
object.identifier = 1
object.description = "Sample"
try database.insert(objects: object, intoTable: Sample.self)
- Querying data (iOS/Swift):
let objects: [Sample] = try database.getObjects(fromTable: Sample.self,
where: Sample.Properties.identifier == 1)
- Updating data (iOS/Swift):
try database.update(table: Sample.self,
on: Sample.Properties.description,
with: "Updated Sample",
where: Sample.Properties.identifier == 1)
Getting Started
To use WCDB in your iOS project:
-
Add WCDB to your project using CocoaPods:
pod 'WCDB.swift'
-
Import WCDB in your Swift file:
import WCDBSwift
-
Create a database instance:
let database = Database(withPath: "path/to/your/database.db")
-
Define your model classes conforming to
TableCodable
protocol and start using WCDB's APIs for database operations.
Competitor Comparisons
Fast persistent recoverable log and key-value store + cache, in C# and C++.
Pros of FASTER
- Designed for larger-than-memory data, offering better scalability for big data scenarios
- Supports both key-value and log storage, providing more flexibility in data models
- Offers hybrid log-structured storage for improved performance in certain workloads
Cons of FASTER
- Less focus on mobile platforms, potentially limiting its use in mobile app development
- May have a steeper learning curve due to its more complex architecture
- Lacks built-in encryption features, which are important for sensitive data handling
Code Comparison
WCDB (SQL-based):
INSERT INTO users (name, age) VALUES ('John', 30);
SELECT * FROM users WHERE age > 25;
FASTER (Key-Value based):
faster.Upsert(key, value);
faster.Read(key, out value);
Summary
WCDB is a SQLite-based database optimized for mobile platforms, offering strong encryption and ACID compliance. FASTER, on the other hand, is a high-performance key-value store and log designed for larger-than-memory data scenarios. While WCDB excels in mobile app development with its SQL interface and encryption features, FASTER provides better scalability and flexibility for big data applications. The choice between them depends on the specific requirements of the project, such as platform, data size, and query complexity.
A library that provides an embeddable, persistent key-value store for fast storage.
Pros of RocksDB
- More mature and widely adopted, with extensive documentation and community support
- Highly optimized for SSDs and offers better performance for large-scale data storage
- Supports a wider range of storage engines and customization options
Cons of RocksDB
- Higher complexity and steeper learning curve compared to WCDB
- Larger memory footprint and potentially slower for small-scale applications
- Less focus on mobile-specific optimizations
Code Comparison
WCDB (C++):
WCDB::Database database("path/to/database");
WCDB::Table<Person> table = database.getTable<Person>("person");
table.insert(person);
RocksDB (C++):
rocksdb::DB* db;
rocksdb::Options options;
rocksdb::Status status = rocksdb::DB::Open(options, "path/to/database", &db);
db->Put(rocksdb::WriteOptions(), "key", "value");
Key Differences
- WCDB is specifically designed for mobile platforms, while RocksDB is more general-purpose
- WCDB offers a higher-level API with ORM-like features, whereas RocksDB provides a lower-level key-value interface
- RocksDB focuses on performance and scalability for large datasets, while WCDB prioritizes ease of use and mobile-specific optimizations
LevelDB is a fast key-value storage library written at Google that provides an ordered mapping from string keys to string values.
Pros of LevelDB
- Lightweight and fast key-value storage engine
- Supports ordered mapping from string keys to string values
- Widely used and battle-tested in various applications
Cons of LevelDB
- Limited support for complex queries and indexing
- Lacks built-in multi-threaded support
- No native SQL interface
Code Comparison
LevelDB:
leveldb::DB* db;
leveldb::Options options;
options.create_if_missing = true;
leveldb::Status status = leveldb::DB::Open(options, "/tmp/testdb", &db);
WCDB:
WCTDatabase *database = [[WCTDatabase alloc] initWithPath:@"/tmp/testdb"];
[database createTableAndIndexesOfName:@"table_name" withClass:YourClass.class];
Key Differences
- WCDB is specifically designed for mobile platforms (iOS and Android), while LevelDB is more general-purpose
- WCDB provides ORM capabilities and supports complex SQL queries, whereas LevelDB is a simpler key-value store
- WCDB offers built-in encryption and multi-threaded operations, features not natively available in LevelDB
- LevelDB has a simpler API and is easier to integrate into projects that don't require complex database operations
- WCDB is based on SQLite and extends its functionality, while LevelDB uses its own storage format
FoundationDB - the open source, distributed, transactional key-value store
Pros of FoundationDB
- Distributed architecture for high scalability and fault tolerance
- Multi-model database supporting key-value, document, and relational models
- Strong ACID guarantees with serializable isolation
Cons of FoundationDB
- Steeper learning curve due to its distributed nature
- Limited mobile-specific optimizations compared to WCDB
- Requires more resources for deployment and management
Code Comparison
WCDB (SQLite-based):
WCDB::Database database("path/to/database");
WCDB::Table<Person> table = database.getTable<Person>("person");
table.insert(person);
FoundationDB:
@fdb.transactional
def insert_person(tr, person):
tr['person/' + person.id] = fdb.tuple.pack((person.name, person.age))
db.run(insert_person, person)
Key Differences
- WCDB is optimized for mobile platforms, while FoundationDB is designed for distributed systems
- WCDB uses SQLite as its core, whereas FoundationDB has a custom storage engine
- FoundationDB offers stronger consistency guarantees in distributed environments
- WCDB provides better performance for local, mobile-centric use cases
Both databases have their strengths, with WCDB excelling in mobile applications and FoundationDB shining in distributed, scalable environments.
RocksDB/LevelDB inspired key-value database in Go
Pros of Pebble
- Designed for high-performance distributed databases, optimized for CockroachDB
- Written in Go, offering better cross-platform compatibility
- Actively maintained with frequent updates and improvements
Cons of Pebble
- Less focused on mobile platforms compared to WCDB
- May have a steeper learning curve for developers not familiar with Go
- Lacks some specific optimizations for SQLite that WCDB provides
Code Comparison
WCDB (C++):
WCDB::Database database("path/to/database");
WCDB::Table table = database[@"tableName"];
auto objects = table.getObjects<MyObject>(where("key" == "value"));
Pebble (Go):
db, _ := pebble.Open("path/to/database", &pebble.Options{})
defer db.Close()
value, closer, _ := db.Get([]byte("key"))
defer closer.Close()
Key Differences
- WCDB is primarily designed for mobile platforms, while Pebble targets distributed systems
- WCDB offers tighter integration with SQLite, whereas Pebble is a standalone key-value store
- WCDB provides an ORM-like interface, while Pebble offers a lower-level key-value API
- Pebble is written in Go, making it more suitable for server-side applications, while WCDB (C++) is optimized for mobile devices
Core database component for the Realm Mobile Database SDKs
Pros of realm-core
- Cross-platform support with native implementations for multiple languages
- Advanced features like real-time synchronization and offline-first capabilities
- Extensive documentation and active community support
Cons of realm-core
- Steeper learning curve due to its unique object-oriented data model
- Larger file size and memory footprint compared to some lightweight alternatives
Code Comparison
realm-core:
auto realm = Realm::get_shared_realm(config);
auto table = realm->read_group().get_table("Person");
auto obj = table->create_object_with_primary_key("Alice");
obj.set("age", 30);
wcdb:
WCDB::Database database("path/to/database");
WCDB::Table<Person> table(database);
Person person;
person.name = "Alice";
person.age = 30;
table.insert(person);
Key Differences
- realm-core uses a unique object-oriented data model, while wcdb follows a more traditional SQL-like approach
- realm-core offers built-in synchronization features, whereas wcdb focuses on local database operations
- wcdb is optimized for mobile platforms, particularly iOS and Android, while realm-core targets a broader range of platforms
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
WCDB
ä¸æçæ¬è¯·åçè¿é
WCDB is an efficient, complete, easy-to-use mobile database framework used in the WeChat application. It's based on SQLite and SQLCipher, and supports five languages: C++, Java, Kotlin, Swift and Objective-C.
Feature
Easy-to-use
- ORM (Object Relational Mapping): WCDB provides a flexible, easy-to-use ORM for creating tables, indices and constraints, as well as CRUD through C++/Java/Kotlin/Swift/Objc objects.
- WINQ (WCDB language integrated query): WINQ is a native data querying capability which frees developers from writing glue code to concatenate SQL query strings.
With ORM and WINQ, you can insert, update, query and delete objects from database in one line code:
// C++
database.insertObjects<Sample>(Sample(1, "text"), myTable);
database.updateRow("text2", WCDB_FIELD(Sample::content), myTable, WCDB_FIELD(Sample::id) == 1);
auto objects = database.getAllObjects<Sample>(myTable, WCDB_FIELD(Sample::id) > 0);
database.deleteObjects(myTable, WCDB_FIELD(Sample::id) == 1);
// Java
database.insertObject(new Sample(1, "text"), DBSample.allFields(), myTable);
database.updateValue("text2", DBSample.content, myTable, DBSample.id.eq(1));
List<Sample> objects = database.getAllObjects(DBSample.allFields(), myTable, DBSample.id.gt(0));
database.deleteObjects(myTable, DBSample.id.eq(1));
// Kotlin
database.insertObject<Sample>(Sample(1, "text"), DBSample.allFields(), myTable)
database.updateValue("text2", DBSample.content, myTable, DBSample.id.eq(1))
val objects = database.getAllObjects<Sample>(DBSample.allFields(), myTable, DBSample.id.gt(0))
database.deleteObjects(myTable, DBSample.id.eq(1))
// Swift
try database.insert(Sample(id:1, content:"text"), intoTable: myTable)
try database.update(table: myTable,
on: Sample.Properties.content,
with: "text2"
where:Sample.Properties.id == 1)
let objects: [Sample] = try database.getObjects(fromTable: myTable,
where: Sample.Properties.id > 0)
try database.delete(fromTable: myTable where: Sample.Properties.id == 1)
// Objc
[database insertObject:sample intoTable:myTable];
[database updateTable:myTable
setProperty:Sample.content
toValue:@"text2"
where:Sample.id == 1];
NSArray* objects = [database getObjectsOfClass:Sample.class
fromTable:myTable
where:Sample.id > 0];
[database deleteFromTable:myTable where:Sample.id == 1];
Efficient
Through the framework layer and sqlcipher source optimization, WCDB have more efficient performance.
- Multi-threaded concurrency: WCDB supports concurrent read-read and read-write access via connection pooling.
- Deeply optimized: WCDB has deeply optimized the source code and configuration of SQLite to adapt to the development scenarios of mobile terminals. At the same time, WCDB has also been optimized for common time-consuming scenarios, such as writing data in batches.
Complete
WCDB summarizes common problems in practice to provide a more complete development experience for database development:
- Encryption Support: WCDB supports database encryption via SQLCipher.
- Corruption recovery: WCDB provides a built-in repair kit for database corruption recovery.
- Anti-injection: WCDB provides a built-in protection from SQL injection.
- Database model upgrade: The database model is bound to the class definition, so that the addition, deletion and modification of database fields are consistent with the definition of class variables.
- Full-text search: WCDB provides an easy-to-use full-text search interface and includes tokenizers for multiple languages.
- Data Migration: WCDB supports to migrate data from one databasse to another with simple configuration. And developers don't need to care about the intermediate status and progress of the migration.
- Data Compression: WCDB supports to compress content via Zstd within specific fields of a database table through a simple configuration. Once configured, the details of data compression and decompression become transparent to developers, and WCDB can automatically compress existing data.
Compatibility
WCDB has interfaces in five languages: C++, Java, Kotlin, Swift, and Objc. Interfaces in different languages share the same underlying logic. The code structure of WCDB is shown in the figure below:
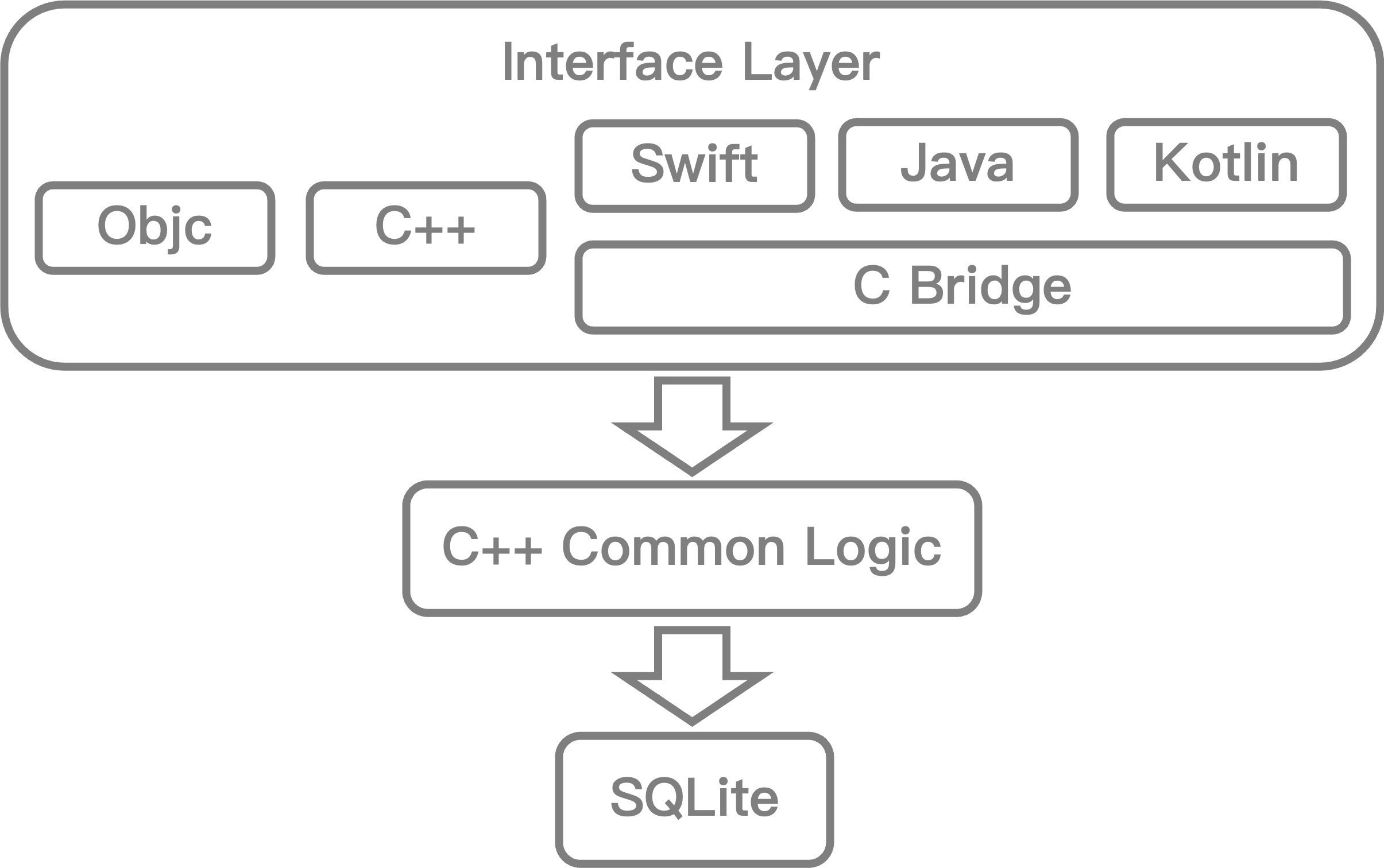
Under such architecture, WCDB in different languages can have the same interface structure and interface capabilities. In one project, you can write database code in different languages with one WCDB. Database logic in different languages will not conflict. Some global interfaces such as error monitoring can work on database logic in different languages at the same time.
Build and Install
Following wikies contain the detailed instructions about building and installing of WCDB.
- Building and Installing of WCDB C++
- Building and Installing of WCDB Java/Kotlin
- Building and Installing of WCDB Swift
- Building and Installing of WCDB Objc
Tutorials
Tutorials of different languages can be found below:
- Tutorials for WCDB C++
- Tutorials for WCDB Java/Kotlin
- Tutorials for WCDB Swift
- Tutorials for WCDB Objc
Contributing
If you are interested in contributing, check out the [CONTRIBUTING.md], also join our Tencent OpenSource Plan.
ä¿¡æ¯å ¬ç¤º
-
å¼åè : æ·±å³å¸è ¾è®¯è®¡ç®æºç³»ç»æéå ¬å¸
Top Related Projects
Fast persistent recoverable log and key-value store + cache, in C# and C++.
A library that provides an embeddable, persistent key-value store for fast storage.
LevelDB is a fast key-value storage library written at Google that provides an ordered mapping from string keys to string values.
FoundationDB - the open source, distributed, transactional key-value store
RocksDB/LevelDB inspired key-value database in Go
Core database component for the Realm Mobile Database SDKs
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot