Top Related Projects
Implementation of ImageView for Android that supports zooming, by various touch gestures.
Android library (AAR). Highly configurable, easily extendable deep zoom view for displaying huge images without loss of detail. Perfect for photo galleries, maps, building plans etc.
Adds touch functionality to Android ImageView.
Customizable Android full screen image viewer for Fresco library supporting "pinch to zoom" and "swipe to dismiss" gestures. Made by Stfalcon
Big image viewer supporting pan and zoom, with very little memory usage and full featured image loading choices. Powered by Subsampling Scale Image View, Fresco, Glide, and Picasso. Even with gif and webp support! 🍻
Quick Overview
GestureViews is an Android library that provides a set of custom views and utilities for implementing gesture-based interactions in Android applications. It focuses on image viewing and manipulation, offering features like zooming, panning, and smooth transitions between different image states.
Pros
- Easy integration with existing Android projects
- Smooth and natural gesture animations
- Supports both single and multiple image viewing scenarios
- Customizable behavior and appearance
Cons
- Limited to image-based interactions
- May require additional setup for complex use cases
- Documentation could be more comprehensive
- Potential performance issues with very large images
Code Examples
- Basic image viewer setup:
val gestureImageView = findViewById<GestureImageView>(R.id.gesture_image_view)
gestureImageView.controller.settings
.setMaxZoom(2f)
.setDoubleTapZoom(-1f) // Will zoom to max zoom level
.setPanEnabled(true)
.setZoomEnabled(true)
.setDoubleTapEnabled(true)
.setRotationEnabled(true)
.setRestrictRotation(false)
.setOverscrollDistance(0f, 0f)
.setOverzoomFactor(2f)
.setFillViewport(false)
.setImage(R.drawable.image)
- Implementing a custom state animator:
class CustomStateAnimator : StateAnimator {
override fun enterImage(view: GestureImageView) {
view.animate()
.scaleX(1f)
.scaleY(1f)
.alpha(1f)
.setDuration(300)
.start()
}
override fun exitImage(view: GestureImageView) {
view.animate()
.scaleX(0.5f)
.scaleY(0.5f)
.alpha(0f)
.setDuration(300)
.start()
}
}
gestureImageView.controller.stateAnimator = CustomStateAnimator()
- Using GestureImageView with Glide:
Glide.with(this)
.load("https://example.com/image.jpg")
.into(object : CustomViewTarget<GestureImageView, Drawable>(gestureImageView) {
override fun onLoadFailed(errorDrawable: Drawable?) {
// Handle error
}
override fun onResourceReady(resource: Drawable, transition: Transition<in Drawable>?) {
view.setImageDrawable(resource)
}
override fun onResourceCleared(placeholder: Drawable?) {
view.setImageDrawable(null)
}
})
Getting Started
- Add the dependency to your app's
build.gradle
:
dependencies {
implementation 'com.alexvasilkov:gesture-views:2.8.3'
}
- Add GestureImageView to your layout:
<com.alexvasilkov.gestures.views.GestureImageView
android:id="@+id/gesture_image_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
- Initialize and configure the GestureImageView in your Activity or Fragment:
val gestureImageView = findViewById<GestureImageView>(R.id.gesture_image_view)
gestureImageView.controller.settings
.setMaxZoom(3f)
.setPanEnabled(true)
.setZoomEnabled(true)
gestureImageView.setImageResource(R.drawable.your_image)
Competitor Comparisons
Implementation of ImageView for Android that supports zooming, by various touch gestures.
Pros of PhotoView
- Simpler implementation with fewer dependencies
- Better performance for basic image viewing and zooming
- More widely adopted and maintained
Cons of PhotoView
- Limited customization options compared to GestureViews
- Lacks advanced features like image transitions and animations
- May not handle complex gesture scenarios as smoothly
Code Comparison
PhotoView implementation:
val photoView = findViewById<PhotoView>(R.id.photo_view)
photoView.setImageResource(R.drawable.image)
GestureViews implementation:
val gestureView = findViewById<GestureImageView>(R.id.gesture_view)
gestureView.controller.settings
.setMaxZoom(3f)
.setDoubleTapZoom(2f)
gestureView.setImageResource(R.drawable.image)
PhotoView offers a more straightforward implementation for basic image viewing and zooming, while GestureViews provides more control over gesture settings and advanced features. The choice between the two libraries depends on the specific requirements of your project, with PhotoView being suitable for simpler use cases and GestureViews offering more flexibility for complex image interactions.
Android library (AAR). Highly configurable, easily extendable deep zoom view for displaying huge images without loss of detail. Perfect for photo galleries, maps, building plans etc.
Pros of Subsampling-Scale-Image-View
- Specialized for handling very large images efficiently
- Supports tiling and subsampling for optimal memory usage
- Provides smooth scrolling and zooming for high-resolution images
Cons of Subsampling-Scale-Image-View
- Limited to image viewing functionality
- Lacks advanced gesture support beyond basic zooming and panning
- May require more setup for complex image manipulation scenarios
Code Comparison
Subsampling-Scale-Image-View:
SubsamplingScaleImageView imageView = new SubsamplingScaleImageView(context);
imageView.setImage(ImageSource.resource(R.drawable.large_image));
imageView.setMaxScale(10f);
GestureViews:
GestureImageView imageView = new GestureImageView(context);
imageView.getController().getSettings()
.setMaxZoom(10f)
.setDoubleTapZoom(3f);
imageView.setImageResource(R.drawable.image);
Both libraries offer easy-to-use image viewing capabilities, but GestureViews provides a more comprehensive set of gesture controls and animations. Subsampling-Scale-Image-View excels at handling very large images with efficient memory usage, while GestureViews offers a wider range of interactive features for general image manipulation tasks.
The code examples demonstrate that both libraries have a straightforward setup process, with Subsampling-Scale-Image-View focusing on image loading and scaling, while GestureViews offers more granular control over gesture settings.
Adds touch functionality to Android ImageView.
Pros of TouchImageView
- Simpler implementation with fewer dependencies
- Lightweight and focused specifically on image viewing functionality
- Easier to integrate into existing projects due to its simplicity
Cons of TouchImageView
- Less feature-rich compared to GestureViews
- Limited to single image viewing, lacking support for image galleries or transitions
- May require additional customization for advanced use cases
Code Comparison
TouchImageView:
TouchImageView touchImageView = findViewById(R.id.touch_image_view);
touchImageView.setImageResource(R.drawable.my_image);
touchImageView.setMaxZoom(4f);
GestureViews:
GestureImageView gestureImageView = findViewById(R.id.gesture_image_view);
gestureImageView.getController().getSettings()
.setMaxZoom(3f)
.setDoubleTapZoom(2f);
gestureImageView.setImageResource(R.drawable.my_image);
Both libraries offer easy-to-use image viewing capabilities with zooming and panning. TouchImageView provides a more straightforward approach, while GestureViews offers more advanced features and customization options. The choice between the two depends on the specific requirements of your project and the level of functionality needed.
Customizable Android full screen image viewer for Fresco library supporting "pinch to zoom" and "swipe to dismiss" gestures. Made by Stfalcon
Pros of FrescoImageViewer
- Built on top of Fresco library, providing efficient image loading and caching
- Supports zooming and panning of images out of the box
- Easy integration with existing Fresco-based projects
Cons of FrescoImageViewer
- Limited to Fresco library, which may not be suitable for all projects
- Less flexible for custom gesture implementations
- Fewer animation options compared to GestureViews
Code Comparison
GestureViews:
GestureImageView imageView = findViewById(R.id.image);
imageView.getController().enableScrollInViewPager();
imageView.getController().setOnGesturesListener(new GestureController.OnGestureListener() {
@Override
public void onDown() {
// Handle touch down event
}
});
FrescoImageViewer:
new ImageViewer.Builder<>(context, images)
.setStartPosition(startPosition)
.setOnDismissListener(onDismissListener)
.show();
GestureViews offers more granular control over gestures and animations, while FrescoImageViewer provides a simpler API for basic image viewing functionality. GestureViews is more versatile but may require more setup, whereas FrescoImageViewer is easier to implement but tied to the Fresco ecosystem.
Big image viewer supporting pan and zoom, with very little memory usage and full featured image loading choices. Powered by Subsampling Scale Image View, Fresco, Glide, and Picasso. Even with gif and webp support! 🍻
Pros of BigImageViewer
- Supports multiple image loading engines (Fresco, Glide, and custom implementations)
- Offers progressive image loading for better performance with large images
- Provides a simpler API for basic image viewing functionality
Cons of BigImageViewer
- Less comprehensive gesture support compared to GestureViews
- Fewer customization options for transitions and animations
- Limited documentation and examples compared to GestureViews
Code Comparison
GestureViews:
GestureImageView imageView = findViewById(R.id.image);
imageView.getController()
.setSettings(GestureController.getSettingsBuilder()
.setMaxZoom(3f)
.setDoubleTapZoom(2f)
.build())
.enableScrollInViewPager(viewPager);
BigImageViewer:
BigImageView bigImageView = findViewById(R.id.image);
bigImageView.setOptimizeDisplay(true);
bigImageView.showImage(Uri.parse("https://example.com/image.jpg"));
The code comparison shows that GestureViews offers more granular control over gesture settings, while BigImageViewer provides a simpler API for basic image viewing functionality. GestureViews allows for detailed customization of zoom levels and integration with ViewPager, whereas BigImageViewer focuses on optimized display and straightforward image loading.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
GestureViews
ImageView and FrameLayout with gestures control and position animation.
Main goal of this library is to make images viewing process as smooth as possible and to make it easier for developers to integrate it into their apps.
Features
- Gestures support: pan, zoom, quick scale, fling, double tap, rotation.
- Seamless integration with ViewPager (panning smoothly turns into ViewPager flipping and vise versa).
- View position animation ("opening" animation). Useful to animate into full image view mode.
- Advanced animation from RecyclerView (or ListView) into ViewPager.
- Exit full image mode by scroll and scale gestures.
- Rounded images with animations support.
- Image cropping (supports rotation).
- Lots of settings.
- Gestures listener: down (touch), up (touch), single tap, double tap, long press.
- Custom state animation (animating position, zoom, rotation).
- Supports both ImageView and FrameLayout out of the box, also supports custom views.
Sample app
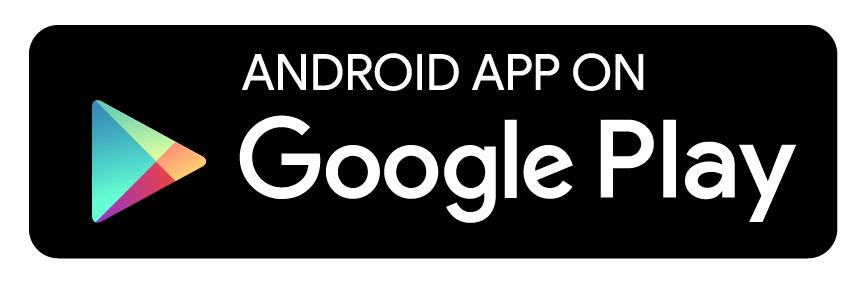
Demo video
Usage
Add dependency to your build.gradle
file:
implementation 'com.alexvasilkov:gesture-views:2.8.3'
License
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
Top Related Projects
Implementation of ImageView for Android that supports zooming, by various touch gestures.
Android library (AAR). Highly configurable, easily extendable deep zoom view for displaying huge images without loss of detail. Perfect for photo galleries, maps, building plans etc.
Adds touch functionality to Android ImageView.
Customizable Android full screen image viewer for Fresco library supporting "pinch to zoom" and "swipe to dismiss" gestures. Made by Stfalcon
Big image viewer supporting pan and zoom, with very little memory usage and full featured image loading choices. Powered by Subsampling Scale Image View, Fresco, Glide, and Picasso. Even with gif and webp support! 🍻
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot