Top Related Projects
A visual graph editor based on G6 and React
Graph theory (network) library for visualisation and analysis
Directed graph layout for JavaScript
:dizzy: Display dynamic, automatically organised, customizable network views.
⚠️ This project is not maintained anymore! Please go to https://github.com/visjs
Force-directed graph layout using velocity Verlet integration.
Quick Overview
G6 is a graph visualization engine for JavaScript, providing developers with powerful tools to create interactive and customizable graph visualizations. It offers a wide range of graph types, layouts, and interactions, making it suitable for various applications such as data analysis, network visualization, and knowledge graphs.
Pros
- Extensive graph visualization capabilities with support for various graph types and layouts
- High performance and efficient rendering, even for large-scale graphs
- Rich set of built-in interactions and customization options
- Active development and community support
Cons
- Steep learning curve for advanced features and customizations
- Documentation can be inconsistent or outdated in some areas
- Limited built-in support for certain specialized graph types
Code Examples
- Creating a basic graph:
import G6 from '@antv/g6';
const data = {
nodes: [
{ id: 'node1', label: 'Node 1' },
{ id: 'node2', label: 'Node 2' },
],
edges: [
{ source: 'node1', target: 'node2' },
],
};
const graph = new G6.Graph({
container: 'mountNode',
width: 800,
height: 600,
});
graph.data(data);
graph.render();
- Applying a custom layout:
import G6 from '@antv/g6';
const graph = new G6.Graph({
container: 'mountNode',
width: 800,
height: 600,
layout: {
type: 'force',
preventOverlap: true,
nodeStrength: -30,
edgeStrength: 0.1,
},
});
- Adding custom node shapes:
import G6 from '@antv/g6';
G6.registerNode('custom-node', {
draw(cfg, group) {
const shape = group.addShape('rect', {
attrs: {
x: -30,
y: -15,
width: 60,
height: 30,
radius: 5,
fill: '#1890FF',
stroke: '#0050B3',
},
});
return shape;
},
});
const graph = new G6.Graph({
// ... other configurations
defaultNode: {
type: 'custom-node',
},
});
Getting Started
To get started with G6, follow these steps:
-
Install G6 using npm:
npm install @antv/g6
-
Import G6 in your project:
import G6 from '@antv/g6';
-
Create a container element in your HTML:
<div id="mountNode"></div>
-
Initialize a graph instance and render your data:
const graph = new G6.Graph({ container: 'mountNode', width: 800, height: 600, }); const data = { nodes: [ { id: 'node1', label: 'Node 1' }, { id: 'node2', label: 'Node 2' }, ], edges: [ { source: 'node1', target: 'node2' }, ], }; graph.data(data); graph.render();
This will create a basic graph visualization. From here, you can explore more advanced features and customizations provided by G6.
Competitor Comparisons
A visual graph editor based on G6 and React
Pros of GGEditor
- Provides a higher-level abstraction for graph editing, with built-in support for flow charts and mind maps
- Offers a more comprehensive set of editing tools and interactions out of the box
- Integrates well with React, making it easier to use in React-based applications
Cons of GGEditor
- Less flexible and customizable compared to G6's lower-level API
- Smaller community and fewer updates, potentially leading to less support and fewer features
- More opinionated design, which may not suit all use cases
Code Comparison
G6 (lower-level graph rendering):
const graph = new G6.Graph({
container: 'mountNode',
width: 800,
height: 600,
});
graph.data(data);
graph.render();
GGEditor (higher-level graph editing):
<GGEditor>
<Flow data={data} />
<EditableLabel />
<ContextMenu />
<Toolbar />
</GGEditor>
G6 provides a more flexible, lower-level API for graph rendering and manipulation, while GGEditor offers a higher-level, more opinionated approach with built-in editing components. G6 is better suited for custom graph visualizations, while GGEditor is more appropriate for quickly implementing editable diagrams with predefined functionality.
Graph theory (network) library for visualisation and analysis
Pros of Cytoscape.js
- More extensive documentation and examples
- Larger community and ecosystem of plugins
- Better support for complex graph analysis algorithms
Cons of Cytoscape.js
- Steeper learning curve for beginners
- Less focus on performance optimization for large-scale graphs
- More complex API for basic graph operations
Code Comparison
G6 example:
const graph = new G6.Graph({
container: 'mountNode',
width: 800,
height: 600,
});
graph.data(data);
graph.render();
Cytoscape.js example:
const cy = cytoscape({
container: document.getElementById('cy'),
elements: data,
style: [ /* ... */ ],
layout: { name: 'grid' }
});
Both libraries offer similar basic functionality for creating and rendering graphs. G6 provides a more straightforward API for simple use cases, while Cytoscape.js offers more flexibility and options out of the box. G6 focuses on performance and customization for large-scale graphs, whereas Cytoscape.js excels in providing a comprehensive set of graph analysis tools and algorithms.
Directed graph layout for JavaScript
Pros of dagre
- Specialized in directed graph layout algorithms
- Lightweight and focused on graph layout computation
- Easier integration with other visualization libraries
Cons of dagre
- Limited built-in rendering capabilities
- Less comprehensive documentation and examples
- Smaller community and fewer updates
Code Comparison
dagre:
var g = new dagre.graphlib.Graph();
g.setNode("a", { label: "A" });
g.setNode("b", { label: "B" });
g.setEdge("a", "b");
dagre.layout(g);
G6:
const graph = new G6.Graph({
container: 'mountNode',
width: 800,
height: 600,
});
graph.data({
nodes: [{ id: 'a', label: 'A' }, { id: 'b', label: 'B' }],
edges: [{ source: 'a', target: 'b' }],
});
graph.render();
Summary
dagre is a specialized library for directed graph layout algorithms, offering lightweight and focused functionality. It's easier to integrate with other visualization libraries but lacks built-in rendering capabilities. G6, on the other hand, provides a more comprehensive graph visualization solution with built-in rendering and a wider range of features. G6 has better documentation and a larger community, making it more suitable for complex graph visualization projects. dagre might be preferred for simpler layout computations or when used in conjunction with other rendering libraries.
:dizzy: Display dynamic, automatically organised, customizable network views.
Pros of vis-network
- More mature and established project with a larger community
- Extensive documentation and examples
- Supports a wider range of graph visualization types
Cons of vis-network
- Heavier library size compared to G6
- Less focus on performance optimization for large datasets
- Limited customization options for advanced visualizations
Code Comparison
vis-network:
var nodes = new vis.DataSet([
{ id: 1, label: 'Node 1' },
{ id: 2, label: 'Node 2' }
]);
var edges = new vis.DataSet([
{ from: 1, to: 2 }
]);
var network = new vis.Network(container, { nodes, edges }, options);
G6:
const data = {
nodes: [
{ id: 'node1', label: 'Node 1' },
{ id: 'node2', label: 'Node 2' }
],
edges: [{ source: 'node1', target: 'node2' }]
};
const graph = new G6.Graph({
container: 'mountNode',
width: 800,
height: 600
});
graph.data(data);
graph.render();
Both libraries offer similar functionality for creating basic network graphs, but G6 provides a more concise API and focuses on performance optimization. vis-network offers more built-in options and graph types out of the box, while G6 emphasizes customization and extensibility for complex visualizations.
⚠️ This project is not maintained anymore! Please go to https://github.com/visjs
Pros of vis
- More comprehensive library with support for various visualization types (networks, timelines, graphs)
- Longer development history and larger community, potentially leading to better stability and support
- Extensive documentation and examples available
Cons of vis
- Larger file size and potentially heavier performance impact
- Less focused on graph visualization specifically compared to G6
- May have a steeper learning curve due to its broader scope
Code Comparison
vis:
var nodes = new vis.DataSet([
{id: 1, label: 'Node 1'},
{id: 2, label: 'Node 2'}
]);
var edges = new vis.DataSet([
{from: 1, to: 2}
]);
var network = new vis.Network(container, {nodes: nodes, edges: edges}, options);
G6:
const data = {
nodes: [
{ id: 'node1', label: 'Node 1' },
{ id: 'node2', label: 'Node 2' }
],
edges: [{ source: 'node1', target: 'node2' }]
};
const graph = new G6.Graph({
container: 'mountNode',
width: 800,
height: 600
});
graph.data(data);
graph.render();
Both libraries offer straightforward ways to create and render graphs, but G6's API appears more concise for basic graph creation.
Force-directed graph layout using velocity Verlet integration.
Pros of d3-force
- More flexible and customizable for force-directed layouts
- Part of the larger D3 ecosystem, integrating well with other D3 modules
- Lightweight and focused specifically on force simulations
Cons of d3-force
- Steeper learning curve, especially for those new to D3
- Requires more manual setup and configuration for complex visualizations
- Less out-of-the-box functionality for graph visualization compared to G6
Code Comparison
d3-force:
const simulation = d3.forceSimulation(nodes)
.force("link", d3.forceLink(links).id(d => d.id))
.force("charge", d3.forceManyBody())
.force("center", d3.forceCenter(width / 2, height / 2));
G6:
const graph = new G6.Graph({
container: 'mountNode',
width: 800,
height: 600,
layout: { type: 'force' }
});
graph.data(data);
graph.render();
Summary
d3-force offers more flexibility and integration with the D3 ecosystem but requires more setup. G6 provides a more comprehensive graph visualization solution with easier initial setup but may be less customizable for specific force-directed layouts.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
English | ç®ä½ä¸æ
G6ï¼å¾å¯è§åæå¼æ
ä»ç» ⢠æ¡ä¾ ⢠æç¨ ⢠API
G6 æ¯ä¸ä¸ªå¾å¯è§å弿ã宿ä¾äºå¾çç»å¶ãå¸å±ãåæã交äºãå¨ç»ã主é¢ãæä»¶çå¾å¯è§åååæçåºç¡è½åãåºäº G6ï¼ç¨æ·å¯ä»¥å¿«éæå»ºèªå·±çå¾å¯è§ååæåºç¨ï¼è®©å ³ç³»æ°æ®åå¾ç®åï¼éæï¼ææä¹ã
â¨ ç¹æ§
G6 ä½ä¸ºä¸æ¬¾ä¸ä¸çå¾å¯è§å弿ï¼å ·æä»¥ä¸ç¹æ§ï¼
- **丰å¯çå ç´ **ï¼å 置丰å¯çèç¹ãè¾¹ãCombo UI å ç´ ï¼æ ·å¼é 置丰å¯ï¼æ¯ææ°æ®åè°ï¼ä¸å ·å¤æçµæ´»æ©å±èªå®ä¹å ç´ çæºå¶ã
- 坿§ç交äºï¼å ç½® 10+ 交äºè¡ä¸ºï¼ä¸æä¾ä¸°å¯çåç±»äºä»¶ï¼ä¾¿äºæ©å±èªå®ä¹ç交äºè¡ä¸ºã
- 髿§è½å¸å±ï¼å ç½® 10+ 常ç¨çå¾å¸å±ï¼é¨ååºäº GPUãRust å¹¶è¡è®¡ç®æåæ§è½ï¼æ¯æèªå®ä¹å¸å±ã
- **便æ·çç»ä»¶**ï¼ä¼åå ç½®ç»ä»¶åè½åæ§è½ï¼ä¸æçµæ´»çæ©å±æ§ï¼ä¾¿äºä¸å¡å®ç°å®å¶è½åã
- **å¤ä¸»é¢è²æ¿**ï¼æä¾äºäº®è²ãæè²ä¸¤å¥å 置主é¢ï¼å¨ AntV æ°è²æ¿åæä¸ï¼èå ¥ 20+ 常ç¨ç¤¾åºè²æ¿ã
- å¤ç¯å¢æ¸²æï¼åæ¥ G è½åï¼ æ¯æ CanvasãSVG 以å WebGLï¼å Node.js æå¡ç«¯æ¸²æï¼åºäº WebGL æä¾å¼ºå¤§ 3D 渲æå空é´äº¤äºçæä»¶å ã
- **React ä½ç³»**ï¼å©ç¨ React å端çæï¼æ¯æ React èç¹ï¼å¤§å¤§ä¸°å¯ G6 çèç¹åç°æ ·å¼ã
ð¨ å¼å§ä½¿ç¨
å¯ä»¥éè¿ NPM æ Yarn çå 管ç卿¥å®è£ ã
$ npm install @antv/g6
æåå®è£
ä¹åï¼å¯ä»¥éè¿ import 导å
¥ Graph
对象ã
<div id="container"></div>
import { Graph } from '@antv/g6';
// å夿°æ®
const data = {
nodes: [
/* your nodes data */
],
edges: [
/* your edges data */
],
};
// åå§åå¾è¡¨å®ä¾
const graph = new Graph({
container: 'container',
data,
node: {
palette: {
type: 'group',
field: 'cluster',
},
},
layout: {
type: 'force',
},
behaviors: ['drag-canvas', 'drag-node'],
});
// 渲æå¯è§å
graph.render();
ä¸å顺å©ï¼ä½ å¯ä»¥å¾å°ä¸é¢çå导å¾!
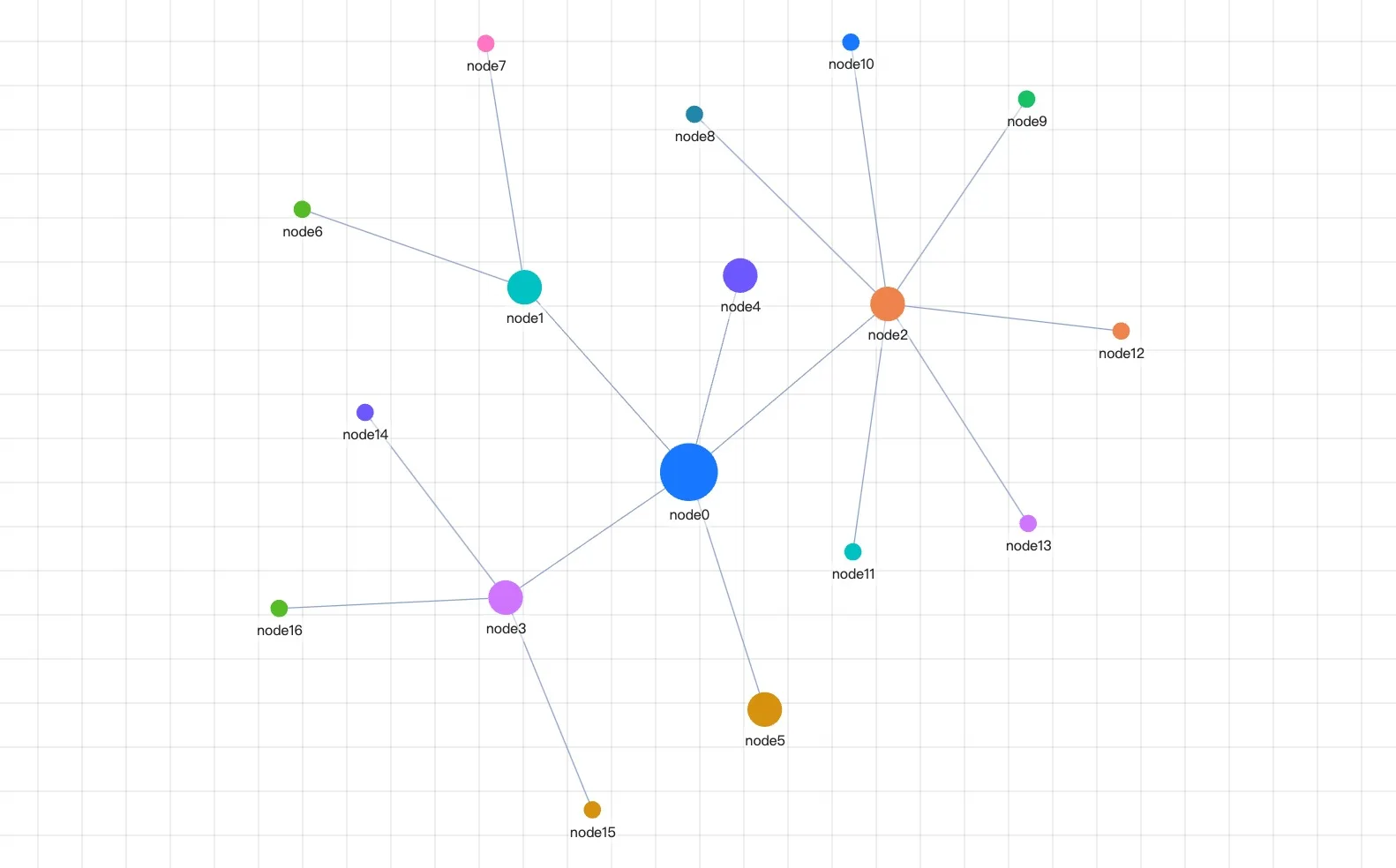
ð çæ
- Ant Design Chartsï¼ React å¾è¡¨åºï¼åºäº G2ãG6ãX6ãL7ã
- Graphinï¼åºäº G6 ç React ç®åå°è£ ï¼ä»¥åå¾å¯è§ååºç¨ç åç SDKã
æ´å¤çæå¼æºé¡¹ç®ï¼æ¬¢è¿ PR æ¶å½è¿æ¥ã
ð® è´¡ç®
- é®é¢åé¦ï¼ä½¿ç¨è¿ç¨éå°ç G6 çé®é¢ï¼æ¬¢è¿æäº¤ Issueï¼å¹¶éä¸å¯ä»¥å¤ç°é®é¢çæå°æ¡ä¾ä»£ç ã
- è´¡ç®æåï¼å¦ä½åä¸å° G6 çå¼ååè´¡ç®ã
- æ³æ³è®¨è®ºï¼å¨ GitHub Discussion 䏿è éé群éé¢è®¨è®ºã
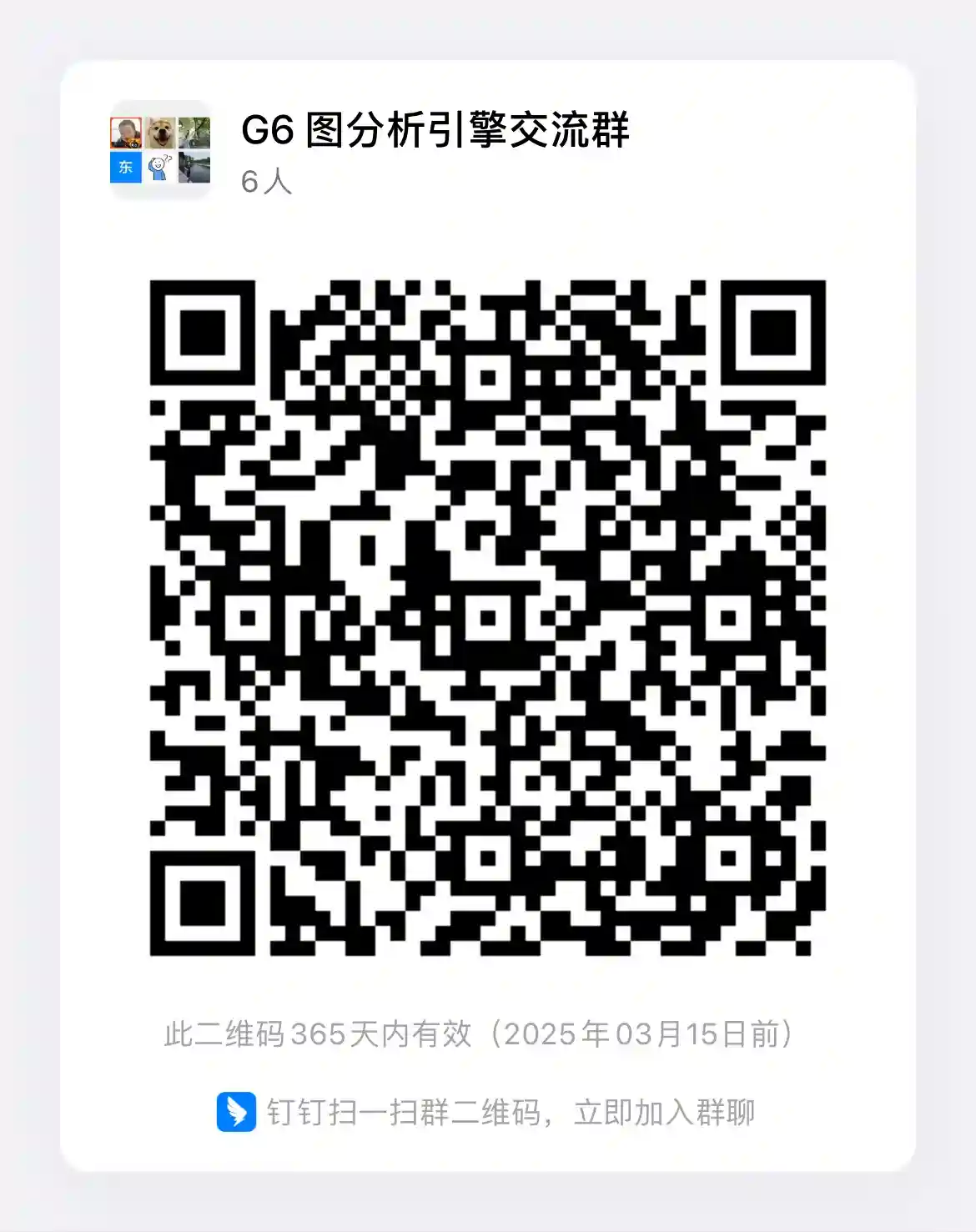
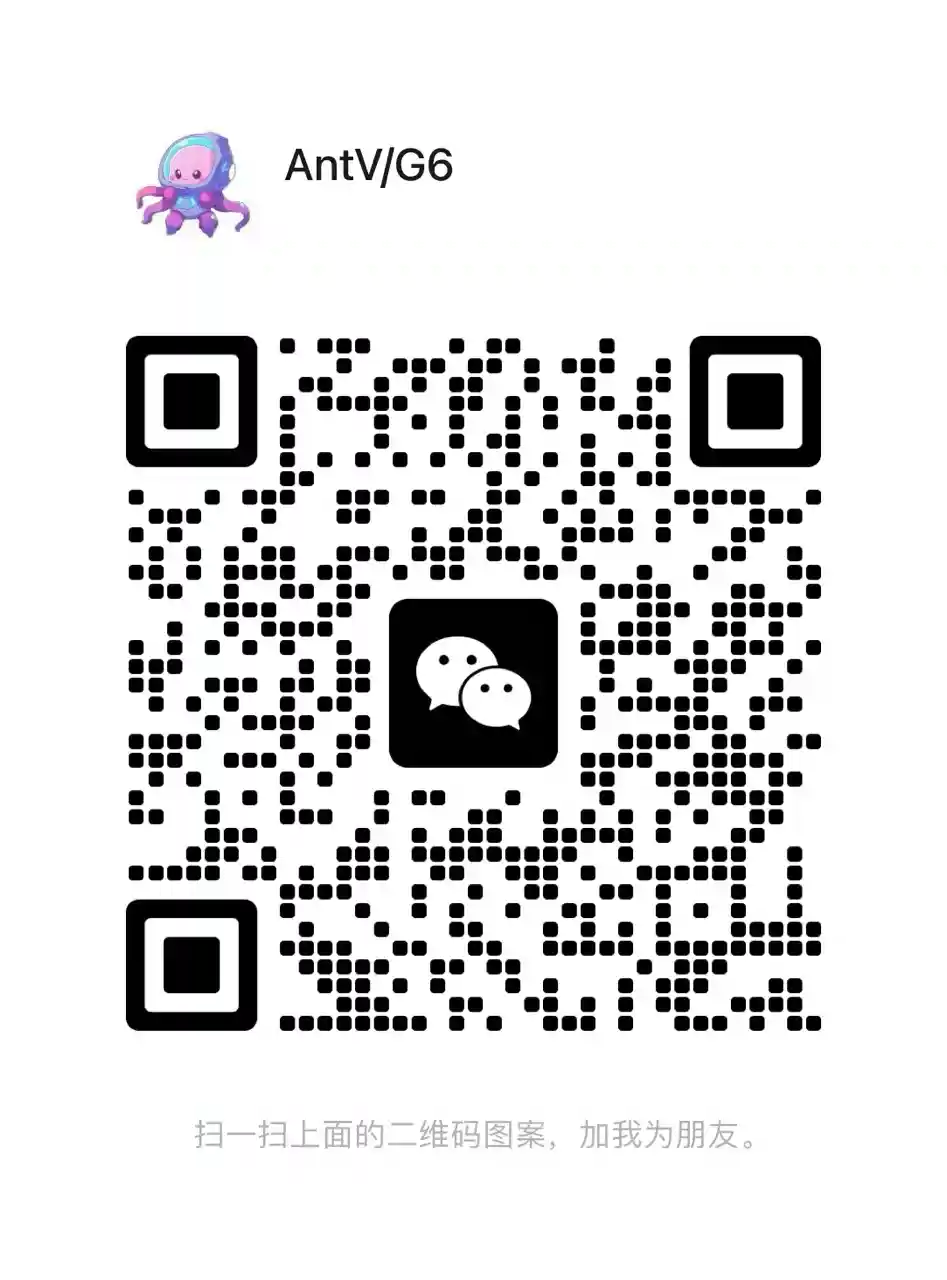
ð License
MIT.
Top Related Projects
A visual graph editor based on G6 and React
Graph theory (network) library for visualisation and analysis
Directed graph layout for JavaScript
:dizzy: Display dynamic, automatically organised, customizable network views.
⚠️ This project is not maintained anymore! Please go to https://github.com/visjs
Force-directed graph layout using velocity Verlet integration.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot