Top Related Projects
Pure Go implementation of the WebRTC API
WebRTC Media Server
SRS is a simple, high-efficiency, real-time media server supporting RTMP, WebRTC, HLS, HTTP-FLV, HTTP-TS, SRT, MPEG-DASH, and GB28181.
[ARCHIVED] Contents migrated to monorepo: https://github.com/Kurento/kurento
Quick Overview
The anyRTC-RTMP-OpenSource project is an open-source RTMP (Real-Time Messaging Protocol) server implementation that allows developers to build real-time video and audio streaming applications. It is part of the anyRTC-Community project, which provides a suite of tools and libraries for building real-time communication (RTC) applications.
Pros
- Cross-Platform Compatibility: The project supports multiple platforms, including Windows, macOS, and Linux, making it accessible to a wide range of developers.
- Scalable and Efficient: The server is designed to be scalable and efficient, handling a large number of concurrent connections without compromising performance.
- Customizable: The project provides a modular architecture, allowing developers to customize and extend the server's functionality to fit their specific needs.
- Active Community: The anyRTC-Community project has an active community of contributors and users, providing support, bug fixes, and feature enhancements.
Cons
- Limited Documentation: The project's documentation could be more comprehensive, making it challenging for new users to get started quickly.
- Dependency on Third-Party Libraries: The project relies on several third-party libraries, which may introduce additional complexity and potential compatibility issues.
- Lack of Detailed Benchmarking: The project does not provide detailed benchmarking information, making it difficult to assess its performance characteristics compared to other RTMP server implementations.
- Potential Licensing Concerns: The project's licensing terms may need to be carefully reviewed, as they could impact the use of the server in certain commercial or enterprise-level applications.
Code Examples
Here are a few code examples demonstrating the usage of the anyRTC-RTMP-OpenSource project:
- Initializing the RTMP Server:
#include "RTMPServer.h"
int main() {
RTMPServer server;
server.Init("0.0.0.0", 1935);
server.Start();
// Server is now running and accepting RTMP connections
return 0;
}
- Handling RTMP Client Connections:
class MyRTMPHandler : public RTMPHandler {
public:
virtual void OnConnect(RTMPSession* session) override {
// Handle client connection
}
virtual void OnDisconnect(RTMPSession* session) override {
// Handle client disconnection
}
virtual void OnPublish(RTMPSession* session, const std::string& streamName) override {
// Handle client publishing a stream
}
virtual void OnPlay(RTMPSession* session, const std::string& streamName) override {
// Handle client playing a stream
}
};
int main() {
RTMPServer server;
server.SetRTMPHandler(std::make_unique<MyRTMPHandler>());
server.Init("0.0.0.0", 1935);
server.Start();
return 0;
}
- Integrating with a Media Server:
#include "RTMPServer.h"
#include "MediaServer.h"
int main() {
RTMPServer rtmpServer;
MediaServer mediaServer;
rtmpServer.SetRTMPHandler(std::make_unique<MyRTMPHandler>());
rtmpServer.Init("0.0.0.0", 1935);
mediaServer.Init("0.0.0.0", 8080);
mediaServer.SetRTMPServer(&rtmpServer);
rtmpServer.Start();
mediaServer.Start();
// Server is now running and accepting both RTMP and HTTP connections
return 0;
}
Getting Started
To get started with the anyRTC-RTMP-OpenSource project, follow these steps:
- Clone the repository:
git clone https://github.com/anyrtcIO-Community/anyRTC-RTMP-OpenSource.git
- Build the project:
- On Windows, open the solution file in Visual Studio and build the project.
- On macOS or Linux, navigate to the project directory and run the following commands:
Competitor Comparisons
Pure Go implementation of the WebRTC API
Pros of pion/webrtc
- Extensive documentation and community support
- Actively maintained with frequent updates
- Supports a wide range of WebRTC features and functionality
Cons of pion/webrtc
- Potentially more complex to set up and configure compared to anyRTC-RTMP-OpenSource
- May have a steeper learning curve for developers new to WebRTC
Code Comparison
anyRTC-RTMP-OpenSource:
func (s *Server) Start() error {
s.listener, err = net.Listen("tcp", s.Addr)
if err != nil {
return err
}
defer s.listener.Close()
for {
conn, err := s.listener.Accept()
if err != nil {
return err
}
go s.handleConnection(conn)
}
}
pion/webrtc:
func main() {
// Create a new RTCPeerConnection
peerConnection, err := webrtc.NewPeerConnection(webrtc.Configuration{})
if err != nil {
panic(err)
}
// Add a track
track, err := peerConnection.NewTrack(webrtc.DefaultPayloadTypeVP8, rand.Uint32(), "video", "pion")
if err != nil {
panic(err)
}
_, err = peerConnection.AddTrack(track)
if err != nil {
panic(err)
}
}
WebRTC Media Server
Pros of medooze/media-server
- Supports a wide range of media protocols, including SIP, WebRTC, and RTMP
- Highly scalable and can handle a large number of concurrent connections
- Provides a comprehensive set of features for media processing and management
Cons of medooze/media-server
- Steeper learning curve compared to anyRTC-RTMP-OpenSource
- Requires more system resources to run, which may not be suitable for smaller deployments
- Limited documentation and community support compared to some other media server projects
Code Comparison
anyRTC-RTMP-OpenSource
int main(int argc, char* argv[]) {
// Initialize the RTMP server
CRtmpServer rtmpServer;
rtmpServer.Initialize();
// Start the server
rtmpServer.Start();
// Wait for the server to stop
rtmpServer.WaitForStop();
return 0;
}
media-server
int main(int argc, char* argv[]) {
// Create a new media server instance
MediaServer server;
// Configure the server
server.setLogLevel(LOG_DEBUG);
server.setRTPPortRange(10000, 10100);
// Start the server
server.start();
// Wait for the server to stop
server.join();
return 0;
}
SRS is a simple, high-efficiency, real-time media server supporting RTMP, WebRTC, HLS, HTTP-FLV, HTTP-TS, SRT, MPEG-DASH, and GB28181.
Pros of SRS
- SRS is a more mature and widely-used project, with a larger community and more contributors.
- SRS has a more comprehensive set of features, including support for various streaming protocols and codecs.
- SRS has better documentation and more active development.
Cons of SRS
- SRS may have a steeper learning curve, especially for beginners.
- SRS may be more complex and resource-intensive, depending on the specific use case.
- SRS may have a larger codebase, which could make it more difficult to customize or extend.
Code Comparison
Here's a brief comparison of the code structure between the two projects:
anyRTC-RTMP-OpenSource
int main(int argc, char* argv[]) {
// Initialize the server
ARTCServer server;
server.Init();
// Start the server
server.Start();
// Wait for the server to stop
server.Wait();
return 0;
}
SRS
int main(int argc, char* argv[]) {
// Parse the command line arguments
SrsConfDirective* conf = _srs_config->parse_argv(argc, argv);
// Initialize the server
SrsServer server;
server.initialize(conf);
// Run the server
server.run();
return 0;
}
Both projects have a similar structure, with a main()
function that initializes and runs the server. However, SRS has a more complex configuration system, with the ability to parse command-line arguments and load a configuration file.
[ARCHIVED] Contents migrated to monorepo: https://github.com/Kurento/kurento
Pros of Kurento/kurento-media-server
- Supports a wide range of media processing capabilities, including video and audio processing, recording, and streaming.
- Provides a modular and extensible architecture, allowing developers to easily integrate custom media processing modules.
- Offers a WebRTC-based communication protocol, enabling real-time, low-latency media exchange.
Cons of Kurento/kurento-media-server
- Relatively complex setup and configuration compared to anyRTC-RTMP-OpenSource, which may require more technical expertise.
- Larger codebase and dependencies, which can result in a higher resource footprint and potential performance impact.
- Limited support for RTMP-based streaming, which is a key feature of anyRTC-RTMP-OpenSource.
Code Comparison
Kurento/kurento-media-server (WebRTC-based media processing):
MediaPipeline pipeline = kurento.createMediaPipeline();
WebRtcEndpoint webRtcEp = new WebRtcEndpoint.Builder(pipeline).build();
webRtcEp.connect(webRtcEp);
anyRTC-RTMP-OpenSource (RTMP-based streaming):
RTMPPublisher* publisher = new RTMPPublisher();
publisher->Init("rtmp://localhost/live/stream");
publisher->PushVideoFrame(frame);
publisher->PushAudioFrame(audio);
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
anyLive
anyLive æ¯ anyRTC 弿ºçæ¨ææµé¡¹ç®ãéç¨è·¨å¹³å°æ¶æè®¾è®¡ï¼éç¨WebRTC(93)çæ¬ä¸ºåºç¡æ¡æ¶ï¼ï¼ä¸å¥ä»£ç æ¯æAndroidãiOSãWindowsãMacãUbuntuçå¹³å°ã
åè½ç¹æ§
ç±»å | åè½è¯´æ |
---|---|
飿 ¼ | ç»ä¸C++æ ¸å¿åºä»£ç 飿 ¼éç¨ï¼Google code style |
æ¡æ¶ | WebRTC-93 |
åè®® | rtmpãhttp/httpsãrtspãhlsãm3u8ãmkvãmp3ãmp4ç |
å¸å±èªå®ä¹ | SDK å UI å离ï¼å¯ä»¥èªå®ä¹æ·»å è§é¢UIå± |
滤é | æ¯æåºäºGPUImageç¾é¢æ»¤éï¼å¯èªå®ä¹æ»¤é |
å¸§å¾ | è§é¢ç¬¬ä¸å¸§ãè§é¢å¸§æªå¾åè½ |
ææ¾ | å便æ¾ãå¤ä¸ªåæ¶ææ¾ãè§é¢å表æ»å¨èªå¨ææ¾ãåè¡¨åæ¢è¯¦æ 页颿 ç¼ææ¾ |
èªéé/èªæ¸²æ | å¯èªå®ä¹é³è§é¢ééå±å渲æå±ï¼æ¹ä¾¿æ¥å ¥ç¬¬ä¸æ¹ç¾é¢ãç¾å£°ç |
æ¨æµ | ä¸éå¶ç¨æ·çæ¨æµãææµå°å |
å¾çæ¨æµ | æ¯æç¹æ®åºæ¯ä¸å ³éæå头ï¼å¾çè¿è¡æ¨æµ |
å±å¹å ±äº« | æ¯æå±å¹å ±äº« |
SEI | æ¯æèªå®ä¹ä¿¡æ¯çåé䏿¥æ¶ |
é³éæ£æµ | æ¯æé³é大尿£æµæç¤º |
éå | æ¯ææ¬å°é¢è§éå以åç¼ç éå |
ç¼è§£ç å¨ | H264/H265/Opus/AAC/G.711 |
å¹³å°å ¼å®¹
ç³»ç» | ç¼è¯ç¯å¢ | CPUæ¶æ |
---|---|---|
Android 4.4åä»¥ä¸ | Android StudioãNDK | armeabi-v7aãarm64-v8a |
iOS 9.0åä»¥ä¸ | Xcode14 | arm64 |
Windows 7åä»¥ä¸ | VS2015,VS2017 | x86ãx86-64 |
ç¬¬ä¸æ¹åº
- libfaac 1.28
- libfaad2 2.7
- ffmpeg 4.3
- libsrtp
- libvpx
- pffft
- rapidjson
- usrsctplib
- libyuv newest
- openh264 1.6.0
é å¥è§å
- æ¯æ P2P-CDN ææ¾ï¼ä¸ºç¨æ·èç弿¯
- å å ¥è¿éº¦åè½
- ç¾é¢ç¾å贴纸åº
- ä½å»¶è¿ç´ææ¨ææµ
ææ¯æ¯æ
anyRTC宿¹ç½åï¼https://www.anyrtc.io QQææ¯äº¤æµç¾¤ï¼554714720(已满) 2群ï¼698167259 èç³»çµè¯:021-65650071-816 Email:hi@dync.cc
ææ¯é®é¢ï¼å¼åè 论å
å å¾®ä¿¡å ¥ææ¯ç¾¤äº¤æµï¼
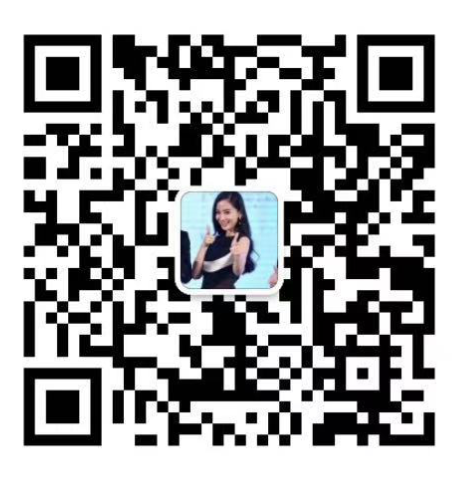
çæå£°æ
è¥æ¬å¼æºé¡¹ç®æ¶åå°å ¶ä»è½¯ä»¶ççæï¼è¯·åæ¶èç³»ä½è è¿è¡ä¿®æ£ã
æèµ
æ¬é¡¹ç®ä¸æ¥åä»»ä½å½¢å¼çæèµ ï¼æ¨çæ¯æå°±æ¯æå¤§çå¨åã
License
anyLive is available under the GNU license. See the LICENSE file for more info.
mailto:hi@dync.cc)
Top Related Projects
Pure Go implementation of the WebRTC API
WebRTC Media Server
SRS is a simple, high-efficiency, real-time media server supporting RTMP, WebRTC, HLS, HTTP-FLV, HTTP-TS, SRT, MPEG-DASH, and GB28181.
[ARCHIVED] Contents migrated to monorepo: https://github.com/Kurento/kurento
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot