Decompose
Kotlin Multiplatform lifecycle-aware business logic components (aka BLoCs) with routing (navigation) and pluggable UI (Jetpack Compose, SwiftUI, JS React, etc.)
Top Related Projects
Compose Multiplatform, a modern UI framework for Kotlin that makes building performant and beautiful user interfaces easy and enjoyable.
A collection of extension libraries for Jetpack Compose
Mavericks: Android on Autopilot
MVI framework with events, time-travel, and more
Library support for Kotlin coroutines
RxJava bindings for Kotlin
Quick Overview
Decompose is a Kotlin Multiplatform lifecycle-aware business logic components library. It provides a way to organize your application's logic into independent, reusable components that can be easily composed and tested. The library is designed to work seamlessly across different platforms, including Android, iOS, and web applications.
Pros
- Platform-independent architecture, allowing for code sharing across multiple platforms
- Promotes separation of concerns and modular design
- Supports state preservation and restoration out of the box
- Integrates well with popular UI frameworks like Jetpack Compose and SwiftUI
Cons
- Steep learning curve for developers new to component-based architecture
- Limited community resources and examples compared to more established libraries
- May introduce additional complexity for smaller projects
- Requires careful design to avoid over-engineering
Code Examples
- Creating a simple component:
class CounterComponent(
componentContext: ComponentContext
) : ComponentContext by componentContext {
private val _state = MutableValue(0)
val state: Value<Int> = _state
fun increment() {
_state.value++
}
}
- Using the component in a Jetpack Compose UI:
@Composable
fun CounterScreen(component: CounterComponent) {
val count by component.state.collectAsState()
Column {
Text("Count: $count")
Button(onClick = component::increment) {
Text("Increment")
}
}
}
- Creating a child component:
class ParentComponent(
componentContext: ComponentContext
) : ComponentContext by componentContext {
private val childComponent = ChildComponent(childContext())
val childState: Value<ChildState> = childComponent.state
}
Getting Started
To start using Decompose in your Kotlin Multiplatform project:
- Add the Decompose dependency to your
build.gradle.kts
file:
dependencies {
implementation("com.arkivanov.decompose:decompose:1.0.0")
}
- Create your first component:
class RootComponent(
componentContext: ComponentContext
) : ComponentContext by componentContext {
// Component logic here
}
- Use the component in your UI layer (e.g., Jetpack Compose):
@Composable
fun App(root: RootComponent) {
// UI implementation using the root component
}
Competitor Comparisons
Compose Multiplatform, a modern UI framework for Kotlin that makes building performant and beautiful user interfaces easy and enjoyable.
Pros of compose-multiplatform
- Official Jetbrains project with strong community support and regular updates
- Seamless integration with other Jetbrains tools and IDEs
- Comprehensive documentation and extensive examples
Cons of compose-multiplatform
- Steeper learning curve for developers new to Compose
- Limited support for certain platform-specific features
- Larger app size due to inclusion of Compose runtime
Code Comparison
Decompose:
class RootComponent(
componentContext: ComponentContext
) : ComponentContext by componentContext {
private val _model = MutableStateFlow(Model())
val model: StateFlow<Model> = _model.asStateFlow()
}
compose-multiplatform:
@Composable
fun App() {
var text by remember { mutableStateOf("Hello, World!") }
Button(onClick = { text = "Hello, Compose!" }) {
Text(text)
}
}
Summary
While compose-multiplatform offers a more comprehensive solution for cross-platform development with strong Jetbrains ecosystem integration, Decompose provides a lightweight alternative focused on navigation and state management. The choice between the two depends on project requirements, team expertise, and desired level of platform-specific customization.
A collection of extension libraries for Jetpack Compose
Pros of Accompanist
- Developed and maintained by Google, ensuring high-quality and up-to-date components
- Wider range of UI components and utilities for Jetpack Compose
- Extensive documentation and integration with other Google libraries
Cons of Accompanist
- Focused primarily on UI components, lacking navigation and state management features
- May have a steeper learning curve for developers new to Jetpack Compose
- Potentially larger dependency size due to the comprehensive set of features
Code Comparison
Accompanist (Insets):
@Composable
fun MyScreen() {
val insets = rememberInsetsPaddingValues()
Box(Modifier.padding(insets)) {
// Content
}
}
Decompose (Component):
class MyComponent(componentContext: ComponentContext) : ComponentContext by componentContext {
private val _state = MutableValue(initialState)
val state: Value<State> = _state
// Component logic
}
Summary
Accompanist provides a rich set of UI components and utilities for Jetpack Compose, backed by Google's development resources. Decompose, on the other hand, focuses on navigation and state management, offering a more modular approach to app architecture. While Accompanist excels in UI-related features, Decompose provides a robust foundation for building complex, scalable applications with a clear separation of concerns.
Mavericks: Android on Autopilot
Pros of Mavericks
- Well-established and widely adopted in the iOS development community
- Extensive documentation and community support
- Integrates seamlessly with other Airbnb libraries and tools
Cons of Mavericks
- Limited to iOS development, not cross-platform
- Steeper learning curve for developers new to reactive programming
- Requires more boilerplate code compared to Decompose
Code Comparison
Mavericks:
class CounterViewModel(
state: CounterState = CounterState()
) : MavericksViewModel<CounterState>(state) {
fun incrementCounter() = setState { copy(count = count + 1) }
}
Decompose:
class CounterComponent(
componentContext: ComponentContext
) : ComponentContext by componentContext {
private val _state = MutableValue(0)
val state: Value<Int> = _state
fun incrementCounter() { _state.value++ }
}
Summary
Mavericks is a robust solution for iOS development with strong community support, while Decompose offers a more flexible, cross-platform approach with less boilerplate. Mavericks may be preferred for iOS-specific projects, whereas Decompose is better suited for multi-platform development and simpler state management.
MVI framework with events, time-travel, and more
Pros of MVICore
- More focused on MVI architecture, providing a robust implementation
- Includes built-in support for side effects and logging
- Offers a more opinionated approach, which can lead to consistent codebase structure
Cons of MVICore
- Less flexible for different architectural patterns
- May have a steeper learning curve for developers unfamiliar with MVI
- Limited to Kotlin, while Decompose supports multiplatform development
Code Comparison
MVICore:
class FeatureImpl : Feature<Wish, State, News> {
override val initialState: State = State()
override val reducer: Reducer<State, Effect> = { state, effect ->
when (effect) {
is Effect.DataLoaded -> state.copy(data = effect.data)
}
}
}
Decompose:
class ComponentImpl(componentContext: ComponentContext) : Component {
private val _state = MutableValue(State())
override val state: Value<State> = _state
fun onSomeEvent() {
_state.value = _state.value.copy(data = "New Data")
}
}
Both libraries offer different approaches to state management and UI architecture. MVICore is more focused on MVI pattern implementation, while Decompose provides a more flexible foundation for various architectural patterns and multiplatform support.
Library support for Kotlin coroutines
Pros of kotlinx.coroutines
- Widely adopted and well-established in the Kotlin ecosystem
- Comprehensive support for asynchronous programming patterns
- Extensive documentation and community resources
Cons of kotlinx.coroutines
- Steeper learning curve for developers new to coroutines
- Can lead to complex code in certain scenarios
- Not specifically designed for UI component lifecycle management
Code Comparison
kotlinx.coroutines:
launch {
val result = async { fetchData() }
updateUI(result.await())
}
Decompose:
val component = ComponentFactory.create()
component.onViewCreated { view ->
view.render(component.state)
}
Key Differences
- Decompose focuses on UI component lifecycle management and state preservation
- kotlinx.coroutines provides general-purpose asynchronous programming tools
- Decompose offers a more structured approach to building modular UI components
- kotlinx.coroutines is more flexible but requires careful handling of lifecycle-related issues
Use Cases
- kotlinx.coroutines: General asynchronous programming, network requests, background tasks
- Decompose: Building modular and reusable UI components, managing complex navigation flows
Integration
Both libraries can be used together in a project, with Decompose leveraging kotlinx.coroutines for asynchronous operations within its component-based architecture.
RxJava bindings for Kotlin
Pros of RxKotlin
- Mature and widely adopted reactive programming library
- Extensive set of operators for complex data transformations
- Supports multiple programming paradigms (functional, imperative)
Cons of RxKotlin
- Steeper learning curve, especially for developers new to reactive programming
- Can lead to complex and hard-to-debug code if not used carefully
- Potential for memory leaks if not properly managed
Code Comparison
RxKotlin:
Observable.just(1, 2, 3)
.map { it * 2 }
.subscribe { println(it) }
Decompose:
val component = ComponentFactory.create()
component.state.observe { state ->
println(state.value)
}
Key Differences
- RxKotlin focuses on reactive programming and data streams
- Decompose is designed for UI component architecture and navigation
- RxKotlin offers more flexibility for general-purpose reactive programming
- Decompose provides a simpler API for managing UI state and lifecycle
Use Cases
- RxKotlin: Complex data processing, event handling, and asynchronous operations
- Decompose: Building modular and testable UI components, managing navigation flow
Community and Ecosystem
- RxKotlin: Large community, extensive documentation, and third-party libraries
- Decompose: Growing community, focused on Kotlin Multiplatform development
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
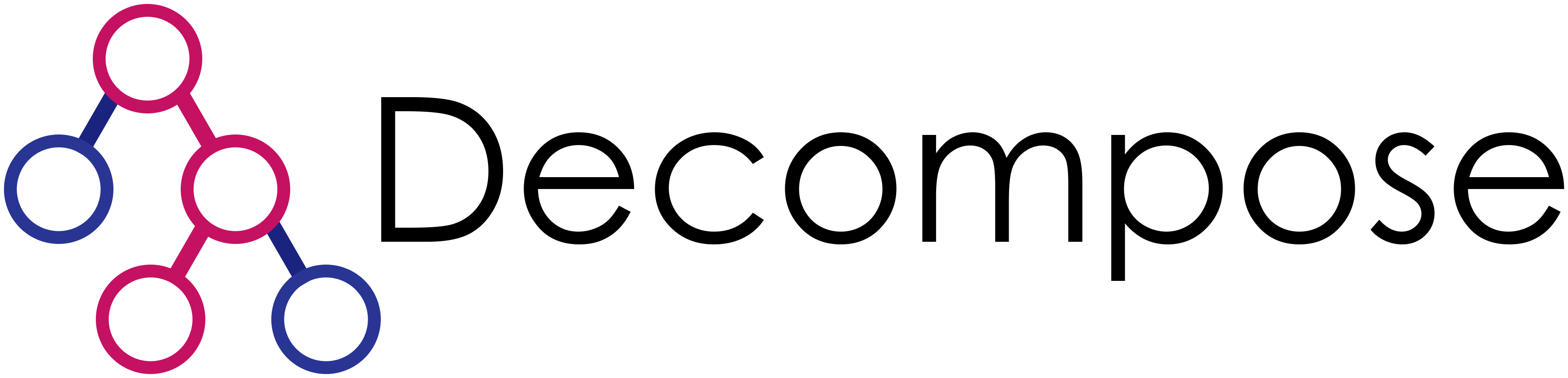
Decompose is a Kotlin Multiplatform library for breaking down your code into tree-structured lifecycle-aware business logic components (aka BLoC), with routing functionality and pluggable UI (Jetpack/Multiplatform Compose, Android Views, SwiftUI, Kotlin/React, etc.).
Please see the project website for documentation and APIs.
Should you have any questions or ideas - there is Discussions section. Also welcome to the Kotlin Slack channel - #decompose! You can also try using DeepWiki!
Why Decompose?
- Decompose breaks the code down into small and independent components and organizes them into trees. Each parent component is only aware of its immediate children.
- Decompose draws clear boundaries between UI and non-UI code, which gives the following benefits:
- Better separation of concerns
- Pluggable platform-specific UI (Compose, SwiftUI, Kotlin/React, etc.)
- Business logic code is testable with pure multiplatform unit tests
- Navigation state is fully exposed - plug any UI you want, animate as you like using your favourite UI framework's API or use predefined API.
- Navigation is a pure function from the old state to a new one - navigate without limits.
- Proper dependency injection (DI) and inversion of control (IoC) via constructor, including but not limited to type-safe arguments.
- Shared navigation logic
- Lifecycle-aware components
- Components in the back stack are not destroyed, they continue working in background without UI
- State preservation (automatically on Android, manually on all other targets via
kotlinx-serialization
) - Instances retaining (aka ViewModels) over configuration changes (mostly useful in Android)
Meme time!

Setup
Please check the Installation section of the documentation.
Supported platforms
In general, Decompose supports the following targets: android
, jvm
, ios
, watchos
, tvos
, macos
, wasmJs
, js
. However, some modules do not support all targets or the support depends on the Decompose version. Please see the installation docs for details.
Overview
Here are some key concepts of the library, more details can be found in the documentation.
- Component - every component represents a piece of logic with its own lifecycle, the UI is optional and is plugged externally
- ComponentContext - every component has its own [ComponentContext], which makes components lifecycle-aware and allows state preservation, instances retaining (aka AndroidX
ViewModel
) and back button handling - Child Stack - enables navigation between child components, nested navigation is also supported
- Child Slot - allows only one child component at a time, or none
- Child Pages - a list of child components with one selected component (e.g. pager-like navigation)
- Child Panels - a multi-pane navigation
- Generic Navigation - provides a way to create your own custom navigation model, when none of the predefined models fit your needs
- Lifecycle - provides a way to listen for lifecycle events in components
- StateKeeper - makes it possible to preserve state or data in a component when it gets destroyed
- InstanceKeeper - retains instances in your components (similar to AndroidX
ViewModel
) - BackPressedHandler - provides a way to handle and intercept back button presses
Decompose is a library
Decompose is a library that can be used as a framework. In its core, Decompose just manipulates instances of ComponentContext
with strict parent-child relationship, which is also called "navigation". The possibility of creating custom implementations of the ComponentContext
interface allows adding custom properties and functions, as well as storing additional data in each instance of ComponentContext
. This makes it a very powerful tool with various use cases.
The "component" term is just one of the possible usages, the recommended one. The Decompose-Router library is a great example of leveraging Decompose to create a custom navigation solution with a completely different API.
Component hierarchy

Pluggable UI hierarchy

Typical component structure

Quick start
Please refer to the Quick start section of the docs.
Samples
Check out the Samples section of the docs for a full description of each sample.
Template
Check out the template repository which may be used to kick-start a project for you.
"Used by" list
Checkout a voluntary list of projects/companies using Decompose: https://github.com/arkivanov/Decompose/discussions/366. Feel free to add your project!
Articles
-
Decompose â experiments with Kotlin Multiplatform lifecycle-aware components and navigation
-
A comprehensive thirty-line navigation for Jetpack/Multiplatform Compose - if you find Decompose verbose and would prefer something built on top of Compose.
-
"Component-based Approach" series by Artur Artikov
Author
Twitter: @arkann1985
Top Related Projects
Compose Multiplatform, a modern UI framework for Kotlin that makes building performant and beautiful user interfaces easy and enjoyable.
A collection of extension libraries for Jetpack Compose
Mavericks: Android on Autopilot
MVI framework with events, time-travel, and more
Library support for Kotlin coroutines
RxJava bindings for Kotlin
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot