leetcode
LeetCode Solutions: A Record of My Problem Solving Journey.( leetcode题解,记录自己的leetcode解题之路。)
Top Related Projects
算法模板,最科学的刷题方式,最快速的刷题路径,你值得拥有~
Demonstrate all the questions on LeetCode in the form of animation.(用动画的形式呈现解LeetCode题目的思路)
✅ Solutions to LeetCode by Go, 100% test coverage, runtime beats 100% / LeetCode 题解
Everything you need to know to get the job.
刷算法全靠套路,认准 labuladong 就够了!English version supported! Crack LeetCode, not only how, but also why.
LeetCode Problems' Solutions
Quick Overview
The azl397985856/leetcode repository is a comprehensive collection of LeetCode problem solutions and explanations in multiple programming languages. It aims to help developers prepare for technical interviews and improve their problem-solving skills by providing detailed walkthroughs and optimized solutions for various LeetCode challenges.
Pros
- Extensive coverage of LeetCode problems with solutions in multiple languages
- Detailed explanations and thought processes for solving each problem
- Regular updates and contributions from the community
- Includes additional resources like interview preparation tips and algorithm explanations
Cons
- Some solutions may not be optimized for all edge cases
- The repository's large size can be overwhelming for beginners
- Not all problems have solutions in every supported language
- Some explanations may be more detailed in Chinese than in English
Code Examples
# Two Sum problem solution
def twoSum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
// Reverse Linked List solution
var reverseList = function(head) {
let prev = null;
let current = head;
while (current !== null) {
let nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
};
// Valid Parentheses solution
public boolean isValid(String s) {
Stack<Character> stack = new Stack<>();
for (char c : s.toCharArray()) {
if (c == '(' || c == '[' || c == '{') {
stack.push(c);
} else {
if (stack.isEmpty()) return false;
if (c == ')' && stack.peek() != '(') return false;
if (c == ']' && stack.peek() != '[') return false;
if (c == '}' && stack.peek() != '{') return false;
stack.pop();
}
}
return stack.isEmpty();
}
Getting Started
To use this repository:
- Clone the repository:
git clone https://github.com/azl397985856/leetcode.git
- Navigate to the problem you want to study.
- Choose the solution in your preferred programming language.
- Read the explanation and study the code.
- Try to implement the solution yourself before checking the provided code.
- Practice regularly and contribute your own solutions if you have optimizations or alternative approaches.
Competitor Comparisons
算法模板,最科学的刷题方式,最快速的刷题路径,你值得拥有~
Pros of algorithm-pattern
- More focused on algorithmic patterns and problem-solving strategies
- Cleaner organization with categorized content
- Includes visual aids and diagrams for better understanding
Cons of algorithm-pattern
- Less comprehensive coverage of LeetCode problems
- Fewer detailed explanations for individual problems
- Limited language support (primarily Go)
Code Comparison
algorithm-pattern (Go):
func twoSum(nums []int, target int) []int {
m := make(map[int]int)
for i, num := range nums {
if j, ok := m[target-num]; ok {
return []int{j, i}
}
m[num] = i
}
return nil
}
leetcode (JavaScript):
var twoSum = function(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
};
Both repositories offer valuable resources for algorithm learning and LeetCode problem-solving. algorithm-pattern focuses on patterns and strategies with a cleaner organization, while leetcode provides a more comprehensive collection of problems with detailed explanations. The choice between the two depends on individual learning preferences and goals.
Demonstrate all the questions on LeetCode in the form of animation.(用动画的形式呈现解LeetCode题目的思路)
Pros of LeetCodeAnimation
- Provides animated visualizations of algorithms, making complex concepts easier to understand
- Offers a unique learning experience through visual representations of problem-solving processes
- Includes a variety of programming languages for solutions, catering to a wider audience
Cons of LeetCodeAnimation
- Has fewer problems covered compared to leetcode
- Lacks detailed explanations and analysis of time/space complexity for some problems
- Updates less frequently, with fewer recent contributions
Code Comparison
LeetCodeAnimation (Python):
def twoSum(nums, target):
hash_table = {}
for i, num in enumerate(nums):
if target - num in hash_table:
return [hash_table[target - num], i]
hash_table[num] = i
return []
leetcode (JavaScript):
var twoSum = function(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) return [map.get(complement), i];
map.set(nums[i], i);
}
};
Both repositories provide solutions to the "Two Sum" problem, but leetcode offers implementations in multiple languages and often includes more detailed explanations of the approach and complexity analysis.
✅ Solutions to LeetCode by Go, 100% test coverage, runtime beats 100% / LeetCode 题解
Pros of LeetCode-Go
- Written in Go, offering solutions for developers focused on this language
- Comprehensive documentation with detailed explanations for each solution
- Includes time and space complexity analysis for most problems
Cons of LeetCode-Go
- Fewer total problems solved compared to leetcode
- Less active community engagement and fewer contributors
- Lacks multilingual support, focusing solely on Go
Code Comparison
LeetCode-Go (Go):
func twoSum(nums []int, target int) []int {
m := make(map[int]int)
for i, num := range nums {
if j, ok := m[target-num]; ok {
return []int{j, i}
}
m[num] = i
}
return nil
}
leetcode (JavaScript):
var twoSum = function(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
if (map.has(target - nums[i])) {
return [map.get(target - nums[i]), i];
}
map.set(nums[i], i);
}
};
Both repositories provide solutions to LeetCode problems, but they cater to different audiences. LeetCode-Go is ideal for Go developers, offering in-depth explanations and complexity analysis. On the other hand, leetcode provides a broader range of solutions in multiple languages, making it more versatile for a diverse audience. The code comparison shows similar approaches to solving the "Two Sum" problem, with each implementation tailored to its respective language.
Everything you need to know to get the job.
Pros of interviews
- More comprehensive coverage of interview topics beyond just LeetCode problems
- Includes additional resources like books, articles, and interview preparation tips
- Offers a wider range of programming languages in code examples
Cons of interviews
- Less frequently updated compared to leetcode
- Fewer detailed problem explanations and solution breakdowns
- Smaller community engagement and fewer contributors
Code Comparison
interviews (Java):
public ListNode reverseList(ListNode head) {
ListNode prev = null;
while (head != null) {
ListNode next = head.next;
head.next = prev;
prev = head;
head = next;
}
return prev;
}
leetcode (JavaScript):
var reverseList = function(head) {
let prev = null;
while (head) {
[head.next, prev, head] = [prev, head, head.next];
}
return prev;
};
Both repositories provide solutions to the "Reverse Linked List" problem, but leetcode uses a more concise approach with JavaScript's destructuring assignment, while interviews uses a more traditional iterative approach in Java.
刷算法全靠套路,认准 labuladong 就够了!English version supported! Crack LeetCode, not only how, but also why.
Pros of fucking-algorithm
- More comprehensive explanations and in-depth analysis of algorithms
- Focuses on problem-solving techniques and thought processes
- Includes detailed diagrams and visualizations to aid understanding
Cons of fucking-algorithm
- Primarily in Chinese, which may limit accessibility for non-Chinese speakers
- Less frequent updates compared to leetcode
- Fewer problems covered overall
Code Comparison
leetcode:
var twoSum = function(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
};
fucking-algorithm:
def twoSum(nums: List[int], target: int) -> List[int]:
hashtable = dict()
for i, num in enumerate(nums):
if target - num in hashtable:
return [hashtable[target - num], i]
hashtable[nums[i]] = i
return []
Both repositories provide solutions to LeetCode problems, but fucking-algorithm offers more detailed explanations and focuses on teaching problem-solving strategies. leetcode covers a wider range of problems and is updated more frequently. The code examples show similar approaches to the "Two Sum" problem, with leetcode using JavaScript and fucking-algorithm using Python.
LeetCode Problems' Solutions
Pros of leetcode (haoel)
- More comprehensive coverage of LeetCode problems (500+ solutions)
- Solutions provided in multiple programming languages (C++, Python, Java, etc.)
- Includes detailed explanations and time/space complexity analysis for many solutions
Cons of leetcode (haoel)
- Less frequent updates compared to the azl397985856 repository
- Lacks a structured organization or categorization of problems
- Some solutions may be outdated or not optimized for current LeetCode requirements
Code Comparison
leetcode (azl397985856):
var twoSum = function(nums, target) {
const map = new Map();
for(let i = 0; i < nums.length; i++) {
if(map.has(target - nums[i])) {
return [map.get(target - nums[i]), i];
}
map.set(nums[i], i);
}
};
leetcode (haoel):
class Solution {
public:
vector<int> twoSum(vector<int>& nums, int target) {
unordered_map<int, int> m;
for (int i = 0; i < nums.size(); i++) {
if (m.find(target - nums[i]) != m.end()) {
return {m[target - nums[i]], i};
}
m[nums[i]] = i;
}
return {};
}
};
Both implementations use a hash map to solve the Two Sum problem efficiently, but they are written in different languages (JavaScript vs. C++) and have slight variations in syntax and structure.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
LeetCode
ç®ä½ä¸æ | English
æä»¬ç slogan æ¯ï¼ åªæçç»ææ¡åºç¡çæ°æ®ç»æä¸ç®æ³ï¼æè½å¯¹å¤æé®é¢è¿åæä½ã
ð¥ð¥ð¥ æçãç®æ³éå ³ä¹è·¯ãåºçäº ð¥ð¥ð¥
æçæ°ä¹¦ãç®æ³éå ³ä¹è·¯ãåºçäºã
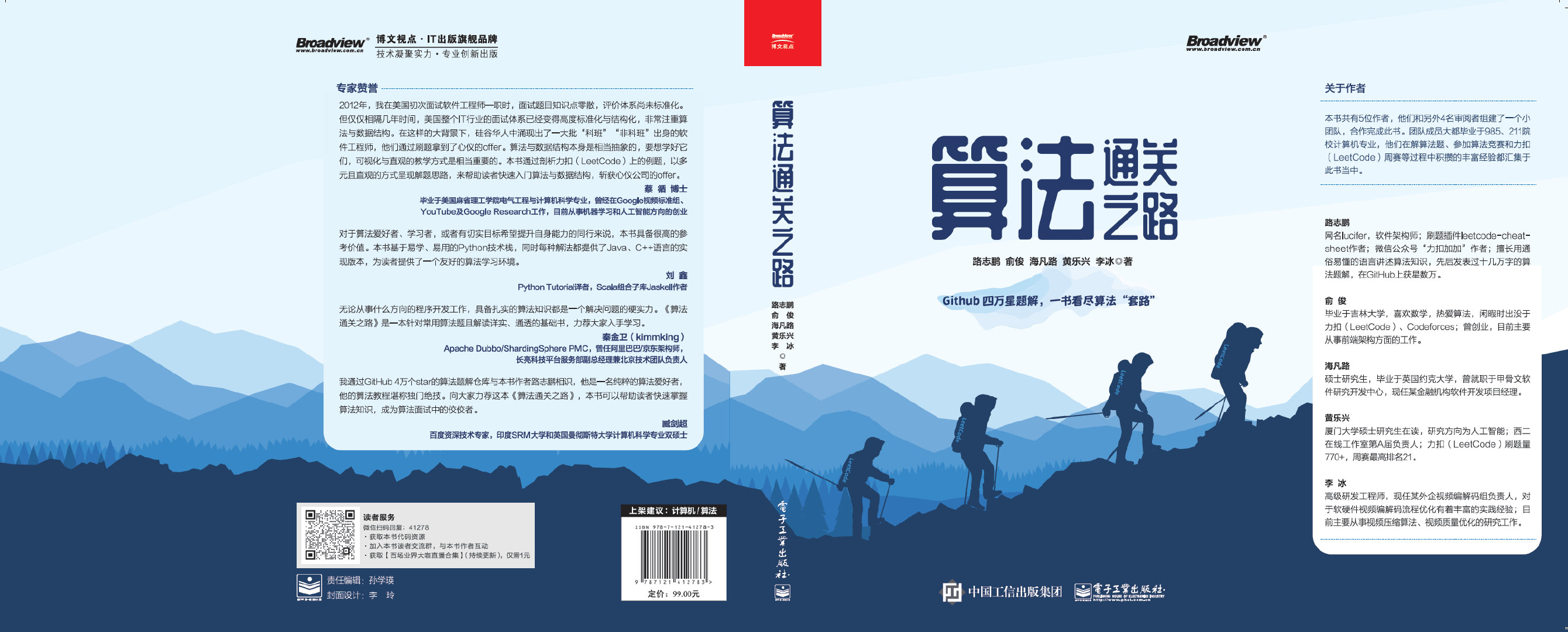
å¾çå è½½ä¸åºæ¥å¦ä½è§£å³ï¼
https://github.com/fe-lucifer/fanqiang
忣ä¸å±ææ£
忣å è´¹é¢ç®å·²ç»æäºå¾å¤ç»å ¸çäºï¼ä¹è¦çäºææçé¢åï¼åªæ¯å¾å¤å ¬å¸ççé¢é½æ¯éå®çã个人è§å¾å¦æä½ å夿¾å·¥ä½çæ¶åï¼å¯ä»¥ä¹°ä¸ä¸ªä¼åãå¦å¤ä¼åå¾å¤leetbook ä¹å¯ä»¥çï¼ç»åå¦ä¹ 计åï¼æçè¿æ¯è®é«çã
ç°å¨åæ£å¨æ¯æ¥ä¸é¢åºç¡ä¸è¿æäºä¸ä¸ª plus ä¼åææï¼æ¯å¤©å·é¢å¯ä»¥è·å¾ç§¯åï¼ç§¯åå¯ä»¥å æ¢åæ£å¨è¾¹ã
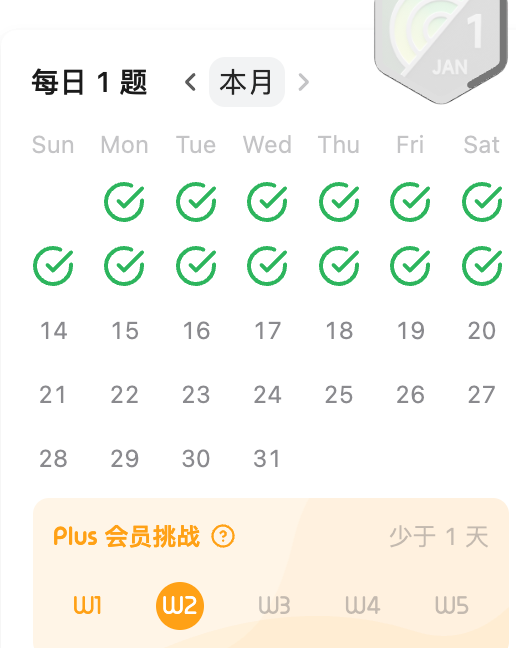
å¦æä½ è¦ä¹°åæ£ä¼åçè¯ï¼è¿éææçä¸å±åæ£ææ£ï¼https://leetcode.cn/premium/?promoChannel=lucifer (年度ä¼åå¤é两个æä¼åï¼å£åº¦ä¼åå¤é两å¨ä¼å)
:calendar:ã91 天å¦ç®æ³ãéæ¶æ´»å¨
å¾å¤æè²æºæå®£ä¼ ç 7 天ï¼ä¸ä¸ªææå®ç®æ³é¢è¯çï¼æå¤§æ¦é½äºè§£äºä¸ï¼ä¸æä¹é è°±ãå¦ä¹ ç®æ³è¿ä¸è¥¿ï¼è¿æ¯è¦é ç§¯ç´¯ï¼æ²¡æé忝ä¸å¯è½æè´¨åçãè¿æçäººéæ©ç书ï¼è¿æ¯ä¸ä¸ªä¸éçéæ©ã使¯å¾å¤äººéäºè¿æ¶çæè è´¨éå·®ç书ï¼åæè ä¸ä¼å»å书ä¸ç»çç»ä¹ é¢ï¼å¯¼è´ææå¾å·®ã
åºäºè¿å 个åå ï¼æç»ç»äºä¸ä¸ª 91 天å·é¢æ´»å¨ï¼éè¿ä¸ä¸ªç¸å¯¹æ¯è¾é¿çæ¶é´ï¼91 天ï¼ç»åºææ°çå¦ä¹ è·¯å¾ï¼å¹¶å¼ºå¶å¤§å®¶æå¡è¿ç§é«å¼ºåº¦ç»ä¹ æ¥è®©å¤§å®¶**å¨ 91 天åéè§æ´å¥½çèªå·±**ãè¯¦ç»æ´»å¨ä»ç»å¯ä»¥ç¹ä¸æ¹é¾æ¥æ¥çãå¦å¤å¾æç讲ä¹ä¹å¨ä¸é¢äºï¼å¤§å®¶å¯ä»¥ççåä¸åä½ çå£å³ã
æåéç»å¤§å®¶ä¸å¥è¯ï¼ **åæä¸å»ï¼ä¼æçªç¶é´æé¿çä¸å¤©**ã
- ð¥ð¥ð¥ð¥ æ´»å¨é¦é¡µ ð¥ð¥ð¥ð¥
- 91 ç¬¬ä¸æè®²ä¹ - äºåä¸é¢ï¼ä¸ï¼
- 91 ç¬¬ä¸æè®²ä¹ - äºåä¸é¢ï¼ä¸ï¼
1V1 è¾ å¯¼
妿大家è§å¾ä¸é¢çé使´»å¨æçæ¯è¾ä½ï¼æç®å乿¥å 1v1 ç®æ³è¾ 导ï¼ä»·æ ¼æ ¹æ®ä½ çç®æ³åºç¡ä»¥åæ³è¦å¦ä¹ çå 容èå®æå ´è¶£çå¯ä»¥å æå¾®ä¿¡ï¼å¤æ³¨âç®æ³è¾ 导âï¼å¾®ä¿¡å· DevelopeEngineerã
:octocat: ä»åºä»ç»
leetcode é¢è§£ï¼è®°å½èªå·±ç leetcode è§£é¢ä¹è·¯ã
æ¬ä»åºç®åå为äºä¸ªé¨åï¼
-
第ä¸ä¸ªé¨åæ¯ leetcode ç»å ¸é¢ç®çè§£æï¼å æ¬æè·¯ï¼å ³é®ç¹åå ·ä½ç代ç å®ç°ã
-
第äºé¨åæ¯å¯¹äºæ°æ®ç»æä¸ç®æ³çæ»ç»
-
第ä¸é¨åæ¯ anki å¡çï¼ å° leetcode é¢ç®æç §ä¸å®çæ¹å¼è®°å½å¨ anki ä¸ï¼æ¹ä¾¿å¤§å®¶è®°å¿ã
-
第åé¨åæ¯æ¯æ¥ä¸é¢ï¼æ¯æ¥ä¸é¢æ¯å¨äº¤æµç¾¤ï¼å æ¬å¾®ä¿¡å qqï¼éè¿è¡çä¸ç§æ´»å¨ï¼å¤§å®¶ä¸èµ· è§£ä¸éé¢ï¼è¿æ ·è®¨è®ºé®é¢æ´å éä¸ï¼ä¼å¾å°æ´å¤çåé¦ãèä¸ è¿äºé¢ç®å¯ä»¥è¢«è®°å½ä¸æ¥ï¼æ¥åä¼è¿è¡çéæ·»å å°ä»åºçé¢è§£æ¨¡åã
-
第äºé¨åæ¯è®¡åï¼ è¿éä¼è®°å½å°æ¥è¦å å ¥å°ä»¥ä¸ä¸ä¸ªé¨åå 容
:blue_book: çµå书
注æï¼è¿éççµå书并䏿¯ãç®æ³éå ³ä¹è·¯ãççµåçï¼èæ¯æ¬ä»åºå 容ççµåçï¼
éæ¶å è´¹ä¸è½½ï¼åæéæ¶å¯è½æ¶è´¹
å¯ä»¥å»æçå ¬ä¼å·ã忣å å ãåå°åå¤çµå书è·åï¼
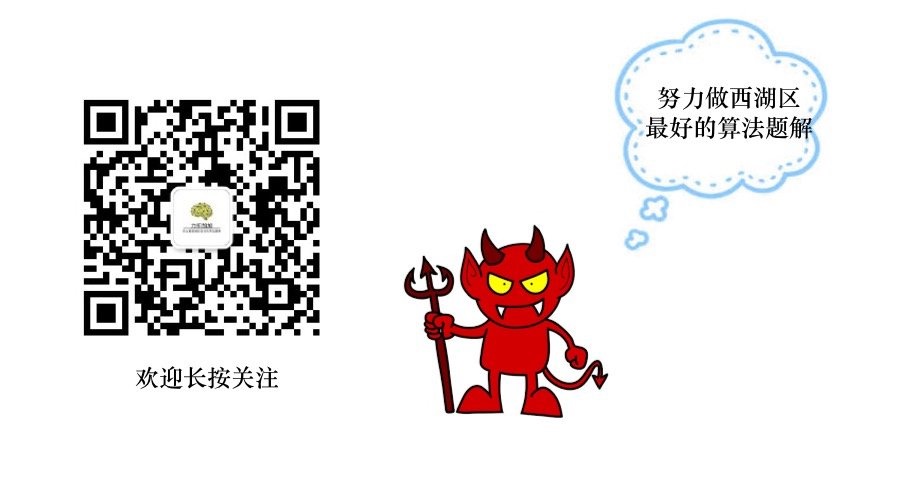
epub è¿æ¯æå¨å¾ç
å¦å¤æäºå 容åªå¨å ¬ä¼å·åå¸ï¼å æ¤å¤§å®¶è§å¾å 容ä¸éçè¯ï¼å¯ä»¥å ³æ³¨ä¸ä¸ã妿åç» â ä¸ªææ å°±æ´æ£å¦ï¼
:meat_on_bone: ä»åºé£ç¨æå
- è¿éæä¸å¼ äºèç½å ¬å¸é¢è¯ä¸ç»å¸¸èå¯çé®é¢ç±»åæ»ç»çæç»´å¯¼å¾ï¼æä»¬å¯ä»¥ç»åå¾çä¸çä¿¡æ¯åæä¸ä¸ã
ï¼å¾çæ¥èª leetcode)
å ¶ä¸ç®æ³ï¼ä¸»è¦æ¯ä»¥ä¸å ç§ï¼
- åºç¡æå·§ï¼åæ²»ãäºåãè´ªå¿
- æåºç®æ³ï¼å¿«éæåºãå½å¹¶æåºãè®¡æ°æåº
- æç´¢ç®æ³ï¼å溯ãéå½ã深度ä¼å éåï¼å¹¿åº¦ä¼å éåï¼äºåæç´¢æ ç
- å¾è®ºï¼æçè·¯å¾ãæå°çææ
- 卿è§åï¼èå é®é¢ãæé¿ååºå
æ°æ®ç»æï¼ä¸»è¦æå¦ä¸å ç§ï¼
- æ°ç»ä¸é¾è¡¨ï¼å / ååé¾è¡¨
- æ ä¸éå
- åå¸è¡¨
- å ï¼æå¤§å ï¼ æå°å
- æ ä¸å¾ï¼æè¿å ¬å ±ç¥å ãå¹¶æ¥é
- å符串ï¼åç¼æ ï¼åå ¸æ ï¼ ï¼ åç¼æ
æå¨ç½ä¸æ¾å°ä¸ä»½ ãInterview Cheat Sheetãï¼è¿ä¸ª PDF å举äºé¢è¯ç**æ¨¡æ¿æ¥éª¤**ãï¼è¯¦ç»æç¤ºäºå¦ä½ä¸æ¥æ¥å®æé¢è¯ã
è¿ä¸ª pdf å¼å¤´å°±æå°äºå¥½ç代ç ä¸ä¸ªæ åï¼
- å¯è¯»æ§
- æ¶é´å¤æåº¦
- 空é´å¤æåº¦
è¿åç太好äºã
ç´§æ¥çï¼åä¸¾äº 15 ç®æ³é¢è¯çæ¥éª¤ãæ¯å¦æ¥éª¤ä¸ï¼å½é¢è¯å®æé®å®åï¼ä½ éè¦å 䏿¥å ³é®ç¹ï¼ä¹ååä¸é¢å注éå代ç ï¼ ç宿çæåå°±æ¯ï¼é¢è¯åªè¦æç §è¿ä¸ªæ¥åï¼æåçè¹è¹æå
æ°æ®ç»æä¸ç®æ³çæ»ç»
- æ°æ®ç»ææ»è§
- é¾è¡¨ä¸é¢
- æ ä¸é¢
- å ä¸é¢ï¼ä¸ï¼
- å ä¸é¢ï¼ä¸ï¼
- äºåä¸é¢ï¼ä¸ï¼
- äºåä¸é¢ï¼ä¸ï¼
- 卿è§åï¼éç½®çï¼
- å¤§è¯æç´¢
- äºåæ çéå
- åæº¯
- å夫æ¼ç¼ç 忏¸ç¨ç¼ç
- å¸éè¿æ»¤å¨ð
- åç¼æ ð
- ãæ¥ç¨å®æãä¸é¢
- ãæé äºåæ ãä¸é¢
- æ»å¨çªå£ï¼æè·¯ + 模æ¿ï¼
- ä½è¿ç®
- å°å²é®é¢ð
- æå¤§å ¬çº¦æ°
- å¹¶æ¥é
- 平衡äºåæ ä¸é¢
- èæ°´æ± æ½æ ·
- åè°æ
:exclamation: æä¹å· LeetCodeï¼
- ææ¯å¦ä½å· LeetCode ç
- ç®æ³å°ç½å¦ä½é«æãå¿«éå· leetcodeï¼
- å·é¢æçä½ï¼æè®¸ä½ 就差è¿ä¹ä¸ä¸ªæä»¶
- 忣å·é¢æä»¶
:computer: æä»¶
æè®¸æ¯ä¸ä¸ªå¯ä»¥æ¹åä½ å·é¢æççæµè§å¨æ©å±æä»¶ã
æä»¶å°åï¼https://chrome.google.com/webstore/detail/leetcode-cheatsheet/fniccleejlofifaakbgppmbbcdfjonle?hl=en-USã
ä¸è½è®¿é®è°·æååºçæåå¯ä»¥å»æçå ¬ä¼å·å夿件è·å离线çãå¼ºçæ¨è大家使ç¨è°·æååºå®è£ ï¼ è¿æ ·å¦æææ´æ°å¯ä»¥èªå¨å®è£ ï¼æ¯ç«å±ä»¬çæä»¶æ´æ°è¿æ¯è®å¿«çã
å¦å¤å¤§å®¶ä¹å¯ä»¥ä½¿ç¨ zerotrac å¼åçç¨äºè®¡ç®åæ£ä¸é¢ç®åæ°çç½ç«ãè¿éçåæ°æçæ¯ç«èµåï¼å¤§å®¶å¯ä»¥æ ¹æ®èªå·±çç«èµåéæ©ç¨å¾®æ¯èªå·±ç«èµåé«ä¸ç¹çé¢ç®è¿è¡ç»ä¹ ï¼æ³¨æè¿ä¸ªåªæ¯æ ¹æ®éè¿äººæ°ç计ç®çä¸ä¸ªé¢ä¼°åæ°ãå°åï¼https://zerotrac.github.io/leetcode_problem_rating/
ç²¾éé¢è§£
- åå ¸åºåå é¤
- 䏿¬¡æå®åç¼å
- åèè·³å¨çç®æ³é¢è¯é¢æ¯ä»ä¹é¾åº¦ï¼
- åèè·³å¨çç®æ³é¢è¯é¢æ¯ä»ä¹é¾åº¦ï¼ï¼ç¬¬äºå¼¹ï¼
- ãææ¯ä½ çå¦å¦åã - ç¬¬ä¸æ
- ä¸æå¸¦ä½ çæäºåæ çåºåå
- ç©¿ä¸è¡£ææå°±ä¸è®¤è¯ä½ äºï¼æ¥èèæé¿ä¸åååºå
- ä½ çè¡£æææäº - ãæé¿å ¬å ±ååºåã
- 䏿çæãæå¤§ååºååé®é¢ã
leetcode ç»å ¸é¢ç®çè§£æï¼200 å¤éï¼
è¿éä» åä¸¾å ·æ**代表æ§é¢ç®**ï¼å¹¶ä¸æ¯å ¨é¨é¢ç®
ç®åæ´æ°äº 200 å¤éé¢è§£ï¼å ä¸ä¸é¢æ¶åçé¢ç®ï¼å·®ä¸å¤æ 300 éã
ç®åé¾åº¦é¢ç®åé
è¿éçé¢ç®é¾åº¦æ¯è¾å°ï¼ 大夿¯æ¨¡æé¢ï¼æè æ¯å¾å®¹æçåºè§£æ³çé¢ç®ï¼å¦å¤ç®åé¢ç®ä¸è¬ä½¿ç¨æ´åæ³é½æ¯å¯ä»¥è§£å³çã è¿ä¸ªæ¶ååªæçä¸ä¸æ°æ®èå´ï¼æèä¸ä½ çç®æ³å¤æåº¦å°±è¡äºã
å½ç¶ä¹ä¸æé¤å¾å¤ hard é¢ç®ä¹å¯ä»¥æ´å模æï¼å¤§å®¶å¹³æ¶å¤æ³¨ææ°æ®èå´å³å¯ã
以䏿¯æå举çç»å ¸é¢ç®ï¼å¸¦ 91 åæ ·ç表示åºèª 91 天å¦ç®æ³æ´»å¨ï¼ï¼
-
0066. å ä¸ 91
-
0155. æå°æ ð
-
0232. ç¨æ å®ç°éå ð 91
-
0342. 4 çå¹ ð
ä¸çé¾åº¦é¢ç®åé
ä¸çé¢ç®æ¯åæ£æ¯ä¾æå¤§çé¨åï¼å æ¤è¿é¨åæçé¢è§£ä¹æ¯æå¤çã 大家ä¸è¦å¤ªè¿è¿½æ±é¾é¢ï¼å æä¸çé¾åº¦é¢ç®åçäºå说ã
è¿é¨åçé¢ç®è¦ä¸éè¦æä»¬ææé¢ç®çå å«ä¿¡æ¯ï¼ å°å ¶æ½è±¡æç®åé¢ç®ã è¦ä¹æ¯ä¸äºåèµ·æ¥æ¯è¾éº»ç¦çé¢ç®ï¼ ä¸äºäººç¼ç è½åä¸è¡å°±æäºãå æ¤å¤§å®¶ä¸å®è¦èªå·±åï¼ å³ä½¿çäºé¢è§£ âä¼äºâï¼ä¹è¦èªå·±ç ä¸éãèªå·±ä¸äº²èªåä¸éï¼éé¢çç»èæ°¸è¿ä¸ç¥éã
以䏿¯æå举çç»å ¸é¢ç®ï¼å¸¦ 91 åæ ·ç表示åºèª 91 天å¦ç®æ³æ´»å¨ï¼ï¼
-
Increasing Digits ð
-
Longest Contiguously Strictly Increasing Sublist After Deletion ð
-
Bus Fare ð
-
0050. Pow(x, n) ð
-
0075. é¢è²åç±» ð
-
0200. å²å±¿æ°é ð
-
0322. é¶é±å æ¢ ð
-
0735. è¡æç¢°æ ð
å°é¾é¾åº¦é¢ç®åé
å°é¾é¾åº¦é¢ç®ä»ç±»åä¸è¯´å¤æ¯ï¼
- å¾
- 设计é¢
- 游æåºæ¯é¢ç®
- ä¸çé¢ç®ç follow up
ä»è§£æ³ä¸æ¥è¯´ï¼å¤æ¯ï¼
- å¾ç®æ³
- 卿è§å
- äºåæ³
- DFS & BFS
- ç¶æå缩
- åªæ
ä»é»è¾ä¸è¯´ï¼ è¦ä¹å°±æ¯é叏龿³å°ï¼è¦ä¹å°±æ¯é常é¾å代ç ã ç±äºææ¶åéè¦ç»åå¤ç§ç®æ³ï¼å æ¤è¿é¨åé¢ç®çé¾åº¦æ¯æå¤§çã
è¿éææ»ç»äºå 个æå·§ï¼
- çé¢ç®çæ°æ®èå´ï¼ çè½å¦æ´å模æ
- æ´åæä¸¾ææå¯è½çç®æ³å¾ä¸å¥ï¼æ¯å¦å¾çé¢ç®ã
- 对äºä»£ç é常é¾åçé¢ç®ï¼å¯ä»¥æ»ç»åè®°å¿è§£é¢æ¨¡æ¿ï¼åå°è§£é¢åå
- 对äºç»åå¤ç§ç®æ³çé¢ç®ï¼å å°è¯ç®åé®é¢ï¼å°é®é¢ååæå 个å°é®é¢ï¼ç¶ååç»åèµ·æ¥ã
以䏿¯æå举çç»å ¸é¢ç®ï¼å¸¦ 91 åæ ·ç表示åºèª 91 天å¦ç®æ³æ´»å¨ï¼ï¼
-
LCP 21. è¿½éæ¸¸æ ð
-
0715. Range 模å ð
-
1639. éè¿ç»å®è¯å ¸æé ç®æ åç¬¦ä¸²çæ¹æ¡æ° new
-
2306. å ¬å¸å½å æä¸¾ä¼å好é¢
-
2312. 忍头å 卿è§åç»å ¸é¢
-
2842. ç»è®¡ä¸ä¸ªå符串ç k ååºåç¾ä¸½å¼æå¤§çæ°ç®
-
3041. ä¿®æ¹æ°ç»åæå¤§åæ°ç»ä¸çè¿ç»å ç´ æ°ç®
:trident: anki å¡ç
Anki 主è¦å为两个é¨åï¼ä¸é¨åæ¯å ³é®ç¹å°é¢ç®çæ å°ï¼å¦ä¸é¨åæ¯é¢ç®å°æè·¯ï¼å ³é®ç¹ï¼ä»£ç çæ å°ã
å ¨é¨å¡çé½å¨ anki-card
ä½¿ç¨æ¹æ³ï¼
anki - æä»¶ - å¯¼å ¥ - ä¸ææ ¼å¼éæ©âæå ç anki éåâï¼ç¶åéä¸ä½ ä¸è½½å¥½çæä»¶ï¼ç¡®å®å³å¯ã
æ´å¤å ³äº anki ä½¿ç¨æ¹æ³ç请æ¥ç anki å®ç½
å ³äºæ
大家ä¹å¯ä»¥å æå¾®ä¿¡å¥½åè¿è¡äº¤æµï¼
:chart_with_upwards_trend: 大äºä»¶
-
2019-07-10 ï¼çºªå¿µé¡¹ç® Star çªç ´ 1W çä¸ä¸ªçæï¼ è®°å½äºé¡¹ç®ç"å ´èµ·"ä¹è·¯ï¼å¤§å®¶æå ´è¶£å¯ä»¥çä¸ä¸ï¼å¦æå¯¹è¿ä¸ªé¡¹ç®æå ´è¶£ï¼è¯·ç¹å»ä¸ä¸ Starï¼ é¡¹ç®ä¼**æç»æ´æ°**ï¼æè°¢å¤§å®¶çæ¯æã
-
2019-10-08: 纪念 LeetCode é¡¹ç® Star çªç ´ 2Wï¼å¹¶ä¸ Github æç´¢âLeetCodeâï¼æå第ä¸ã
-
2020-04-12: 项ç®çªç ´ä¸ä¸ Starã
-
2020-04-14: å®ç½
忣å å
ä¸çº¿å¦ ðððððï¼æä¸é¢è®²è§£ï¼æ¯æ¥ä¸é¢ï¼ä¸è½½åºåè§é¢é¢è§£ï¼åç»ä¼å¢å æ´å¤å 容ï¼è¿ä¸èµ¶ç´§æ¶èèµ·æ¥ï¼å°åï¼http://leetcode-solution.cn/
- 2021-02-23: star ç ´åä¸
:gift_heart: è´¡ç®
- å¦æææ³æ³ååæï¼è¯·æ issue æè è¿ç¾¤æ
- 妿æ³è´¡ç®å¢å é¢è§£æè
ç¿»è¯ï¼ å¯ä»¥åè è´¡ç®æå
å ³äºå¦ä½æäº¤é¢è§£ï¼æåäºä¸ä»½ æå
- 妿éè¦ä¿®æ¹é¡¹ç®ä¸å¾çï¼è¿é åæ¾äºé¡¹ç®ä¸ç»å¶å¾çæºä»£ç ï¼å¤§å®¶å¯ä»¥ç¨ draw.io æå¼è¿è¡ç¼è¾ã
:love_letter: 鸣谢
æè°¢ä¸ºè¿ä¸ªé¡¹ç®ä½åºè´¡ç®çææ å°ä¼ä¼´
License
Top Related Projects
算法模板,最科学的刷题方式,最快速的刷题路径,你值得拥有~
Demonstrate all the questions on LeetCode in the form of animation.(用动画的形式呈现解LeetCode题目的思路)
✅ Solutions to LeetCode by Go, 100% test coverage, runtime beats 100% / LeetCode 题解
Everything you need to know to get the job.
刷算法全靠套路,认准 labuladong 就够了!English version supported! Crack LeetCode, not only how, but also why.
LeetCode Problems' Solutions
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot