Top Related Projects
The React Framework
Build Better Websites. Create modern, resilient user experiences with web fundamentals.
RedwoodGraphQL
web development, streamlined
The Intuitive Vue Framework.
The best React-based framework with performance, scalability and security built in.
Quick Overview
Blitz.js is a full-stack React framework built on Next.js, designed to accelerate web application development. It provides a seamless, zero-API data layer and includes everything you need to build scalable, production-ready applications with React.
Pros
- Zero-API data layer, eliminating the need for manual API creation and management
- Built-in authentication and authorization system
- Automatic code splitting and optimized performance
- Strong conventions and best practices for scalable application architecture
Cons
- Steeper learning curve compared to plain React or Next.js
- Less flexibility in some areas due to opinionated structure
- Smaller community and ecosystem compared to more established frameworks
- May be overkill for simple projects or static websites
Code Examples
- Defining a model and query:
// app/products/queries/getProduct.ts
import { resolver } from "blitz"
import db from "db"
export default resolver.pipe(
resolver.zod(z.object({ id: z.number() })),
async ({ id }) => {
const product = await db.product.findFirst({ where: { id } })
return product
}
)
- Using the query in a React component:
// app/products/pages/[id].tsx
import { useQuery, useParam } from "blitz"
import getProduct from "app/products/queries/getProduct"
export default function Product() {
const id = useParam("id", "number")
const [product] = useQuery(getProduct, { id })
return (
<div>
<h1>{product.name}</h1>
<p>{product.description}</p>
</div>
)
}
- Defining and using a mutation:
// app/products/mutations/createProduct.ts
import { resolver } from "blitz"
import db from "db"
export default resolver.pipe(
resolver.zod(z.object({ name: z.string(), price: z.number() })),
async (input) => {
const product = await db.product.create({ data: input })
return product
}
)
// Using the mutation in a component
import { useMutation } from "blitz"
import createProduct from "app/products/mutations/createProduct"
export default function CreateProductForm() {
const [createProductMutation] = useMutation(createProduct)
return (
<form
onSubmit={async (event) => {
event.preventDefault()
const product = await createProductMutation({ name: "New Product", price: 9.99 })
console.log(product)
}}
>
{/* Form fields */}
</form>
)
}
Getting Started
To create a new Blitz.js project, run the following commands:
npm install -g blitz
blitz new my-blitz-app
cd my-blitz-app
blitz dev
This will create a new Blitz.js project and start the development server. You can then open your browser and navigate to http://localhost:3000
to see your new Blitz.js application running.
Competitor Comparisons
The React Framework
Pros of Next.js
- Larger community and ecosystem, with more resources and third-party integrations
- Better performance optimization out of the box, including automatic code splitting
- More flexible, allowing for both static site generation and server-side rendering
Cons of Next.js
- Requires more configuration and boilerplate code for full-stack applications
- Less opinionated, which can lead to inconsistencies in project structure
- Lacks built-in authentication and database integration
Code Comparison
Next.js:
import { useRouter } from 'next/router'
function Page() {
const router = useRouter()
return <p>Post: {router.query.slug}</p>
}
export default Page
Blitz:
import { useParam } from "blitz"
function Page() {
const slug = useParam("slug", "string")
return <p>Post: {slug}</p>
}
export default Page
Both frameworks offer similar routing capabilities, but Blitz provides a more streamlined approach with its useParam
hook, while Next.js requires the use of the useRouter
hook and accessing the query
object.
Build Better Websites. Create modern, resilient user experiences with web fundamentals.
Pros of Remix
- Built-in server-side rendering (SSR) and code splitting for improved performance
- Seamless integration with existing web standards and APIs
- More flexible routing system with nested routes and layouts
Cons of Remix
- Steeper learning curve, especially for developers new to SSR concepts
- Less opinionated structure, which may require more setup and configuration
- Smaller ecosystem and community compared to Blitz
Code Comparison
Remix route example:
export default function Index() {
return <h1>Welcome to Remix</h1>;
}
export function loader() {
return json({ message: "Hello from loader" });
}
Blitz page example:
import { BlitzPage } from "@blitzjs/next";
const Home: BlitzPage = () => {
return <h1>Welcome to Blitz</h1>;
};
export default Home;
Both Remix and Blitz are modern web frameworks built on top of React, but they have different approaches to building applications. Remix focuses on web standards and server-side rendering, while Blitz provides a more opinionated, full-stack solution with built-in authentication and database integration. The choice between the two depends on project requirements, team expertise, and desired development experience.
RedwoodGraphQL
Pros of RedwoodJS GraphQL
- More flexible GraphQL schema definition and customization options
- Built-in support for GraphQL subscriptions and real-time updates
- Seamless integration with Prisma for database operations
Cons of RedwoodJS GraphQL
- Steeper learning curve for developers new to GraphQL
- Potentially more complex setup and configuration compared to Blitz.js
- May require additional tooling for optimal performance in large-scale applications
Code Comparison
RedwoodJS GraphQL:
export const schema = gql`
type User {
id: Int!
name: String!
email: String!
}
type Query {
users: [User!]!
}
`
Blitz.js:
import { resolver } from "blitz"
import db from "db"
export default resolver.pipe(
resolver.authorize(),
async () => {
return await db.user.findMany()
}
)
The RedwoodJS example shows a GraphQL schema definition, while the Blitz.js example demonstrates a resolver function for fetching users. RedwoodJS requires explicit schema definition, whereas Blitz.js uses a more straightforward approach with built-in resolver functions.
Both frameworks aim to simplify full-stack development, but they take different approaches. RedwoodJS leverages GraphQL for flexible data fetching, while Blitz.js focuses on a more traditional API structure with built-in conventions.
web development, streamlined
Pros of SvelteKit
- Lighter weight and faster performance due to Svelte's compilation approach
- Simpler learning curve with less boilerplate code
- More flexible routing system with dynamic route parameters
Cons of SvelteKit
- Smaller ecosystem and community compared to Blitz.js
- Less opinionated, which may require more decision-making for developers
- Lacks built-in authentication and authorization features
Code Comparison
SvelteKit route file:
<script>
export let data;
</script>
<h1>{data.title}</h1>
<p>{data.content}</p>
Blitz.js page file:
import { useQuery } from 'blitz'
import getPost from 'app/posts/queries/getPost'
export default function Post({ id }) {
const [post] = useQuery(getPost, { id })
return (
<>
<h1>{post.title}</h1>
<p>{post.content}</p>
</>
)
}
SvelteKit focuses on simplicity and performance, while Blitz.js provides a more comprehensive full-stack solution with built-in features. SvelteKit's approach may be more suitable for smaller projects or those requiring high performance, while Blitz.js might be better for larger, more complex applications that benefit from its integrated toolset and conventions.
The Intuitive Vue Framework.
Pros of Nuxt
- More mature and established ecosystem with a larger community
- Better documentation and learning resources
- Seamless integration with Vue.js and its ecosystem
Cons of Nuxt
- Less opinionated, requiring more configuration for complex setups
- Lacks built-in authentication and database solutions
- May have a steeper learning curve for beginners
Code Comparison
Nuxt route definition:
// pages/users/_id.vue
export default {
async asyncData({ params }) {
const user = await fetchUser(params.id)
return { user }
}
}
Blitz route definition:
// app/users/[id].js
import { useQuery } from 'blitz'
import getUser from 'app/users/queries/getUser'
export default function User({ id }) {
const [user] = useQuery(getUser, { id })
return <div>{user.name}</div>
}
Nuxt focuses on file-based routing and Vue.js components, while Blitz uses a more React-centric approach with built-in data fetching hooks. Blitz provides a more integrated full-stack experience, whereas Nuxt primarily focuses on the frontend with optional server-side rendering capabilities.
The best React-based framework with performance, scalability and security built in.
Pros of Gatsby
- Extensive plugin ecosystem for easy integration of various features and data sources
- Strong focus on performance optimization, including automatic code splitting and image optimization
- Large and active community, providing extensive resources and support
Cons of Gatsby
- Steeper learning curve, especially for developers new to GraphQL
- Build times can be slow for large sites with many pages or complex data structures
- Less suitable for dynamic content and frequently updated data
Code Comparison
Gatsby (Static Site Generation):
export const query = graphql`
query {
allMarkdownRemark {
edges {
node {
frontmatter {
title
}
}
}
}
}
`
Blitz (Full-stack React Framework):
import { useQuery } from "blitz"
import getPosts from "app/posts/queries/getPosts"
const Posts = () => {
const [posts] = useQuery(getPosts)
return <PostList posts={posts} />
}
Gatsby uses GraphQL for data fetching, while Blitz leverages React Query for server-state management. Gatsby is primarily focused on static site generation, whereas Blitz provides a full-stack solution with built-in authentication, database integration, and API routes. Blitz offers a more Rails-like developer experience with conventions and code generation, while Gatsby excels in creating static websites with optimized performance.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
The Missing Fullstack Toolkit for Next.js
Quick Start
Install Blitz
Run npm install -g blitz
or yarn global add blitz
You can alternatively use npx
Create a New App
blitz new myAppName
cd myAppName
blitz dev
- View your brand new app at http://localhost:3000
Welcome to the Blitz Community ð
The Blitz community is warm, safe, diverse, inclusive, and fun! LGBTQ+, women, and minorities are especially welcome. Please read our Code of Conduct.
Join our Discord Community where we help each other build Blitz apps. It's also where we collaborate on building Blitz itself.
For questions and longer form discussions, post in our forum.
There's still a lot of work to do, so you are especially invited to join us in building Blitz! A good place to start is The Contributing Guide.
Financial Contributors
Your financial contributions help ensure Blitz continues to be developed and maintained! We have monthly sponsorship options starting at $5/month.
ð View options and contribute at GitHub Sponsors, PayPal, or Open Collective
ð± Seedling Sponsors
![]() |
|
![]() |
![]() |
|
![]() |
ð¥ Bronze Sponsors
![]() |
|
![]() |
ð¥ Silver Sponsors
![]() |
ð Gold Sponsors
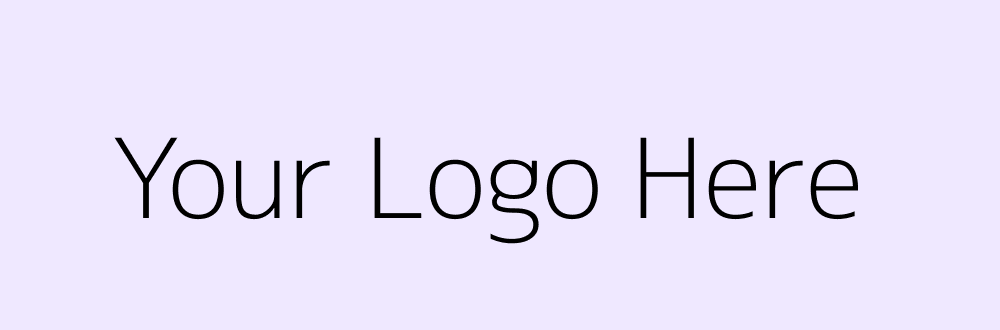
ð Diamond Sponsors
![]() |
Core Team â¨
Brandon Bayer Creator |
Dillon Raphael |
Siddharth Suresh |
Maintainers (Level 2) â¨
Code ownership, pull request approvals and merging, etc (see Maintainers L2)
Simon Knott SuperJSON |
JH.Lee SuperJSON |
Maintainers (Level 1) â¨
Issue triage, pull request triage, community encouragement and moderation, etc (see Maintainers L1)
Jeremy Liberman |
Contributors â¨
Thanks to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!
Top Related Projects
The React Framework
Build Better Websites. Create modern, resilient user experiences with web fundamentals.
RedwoodGraphQL
web development, streamlined
The Intuitive Vue Framework.
The best React-based framework with performance, scalability and security built in.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot