Top Related Projects
A powerful little TUI framework 🏗
Terminal UI library with rich, interactive widgets — written in Golang
Tcell is an alternate terminal package, similar in some ways to termbox, but better in others.
Minimalist Go package aimed at creating Console User Interfaces.
Golang terminal dashboard
Pure Go termbox implementation
Quick Overview
Bubbles is a TUI (Text-based User Interface) component library for Go, created by Charm. It provides a collection of components and utilities for building interactive terminal applications with ease. Bubbles is designed to work seamlessly with the Bubble Tea framework, offering a rich set of customizable UI elements.
Pros
- Extensive collection of ready-to-use TUI components
- Highly customizable and extensible
- Seamless integration with the Bubble Tea framework
- Active development and community support
Cons
- Learning curve for those new to TUI development
- Limited to terminal-based applications
- May require additional setup for complex layouts
Code Examples
- Creating a simple progress bar:
import "github.com/charmbracelet/bubbles/progress"
p := progress.New(progress.WithDefaultGradient())
p.Width = 50
str := p.ViewAs(0.4)
fmt.Println(str)
- Implementing a text input field:
import "github.com/charmbracelet/bubbles/textinput"
ti := textinput.New()
ti.Placeholder = "Enter your name"
ti.Focus()
fmt.Println(ti.View())
- Using a paginator component:
import "github.com/charmbracelet/bubbles/paginator"
p := paginator.New()
p.SetTotalPages(10)
p.Page = 3
fmt.Println(p.View())
Getting Started
To start using Bubbles in your Go project:
-
Install the library:
go get github.com/charmbracelet/bubbles
-
Import the desired components in your Go file:
import ( "github.com/charmbracelet/bubbles/progress" "github.com/charmbracelet/bubbles/textinput" // other components as needed )
-
Create and use the components in your Bubble Tea program:
func (m model) View() string { return fmt.Sprintf( "Progress: %s\nInput: %s", m.progress.View(), m.textInput.View(), ) }
For more detailed usage and examples, refer to the official documentation and examples in the GitHub repository.
Competitor Comparisons
A powerful little TUI framework 🏗
Pros of Bubbletea
- More comprehensive framework for building terminal user interfaces
- Provides a robust event-driven architecture
- Offers greater flexibility for complex applications
Cons of Bubbletea
- Steeper learning curve for beginners
- Requires more boilerplate code for simple applications
- May be overkill for small, straightforward projects
Code Comparison
Bubbles (creating a simple progress bar):
progress := bubbles.NewProgress()
progress.ShowPercentage = true
progress.Width = 50
progress.SetPercent(0.5)
Bubbletea (creating a simple progress bar):
type model struct {
progress float64
}
func (m model) View() string {
return fmt.Sprintf("Progress: %.0f%%", m.progress*100)
}
Bubbles provides ready-to-use components like progress bars, while Bubbletea requires more custom implementation but offers greater control over the application's structure and behavior.
Terminal UI library with rich, interactive widgets — written in Golang
Pros of tview
- More comprehensive set of UI components and layouts
- Better suited for complex, full-screen terminal applications
- Extensive documentation and examples
Cons of tview
- Steeper learning curve due to more complex API
- Less focus on individual, reusable components
- Heavier dependency footprint
Code Comparison
tview example:
app := tview.NewApplication()
box := tview.NewBox().SetBorder(true).SetTitle("Hello, world!")
app.SetRoot(box, true).Run()
Bubbles example:
m := model{list: list.New([]list.Item{}, list.NewDefaultDelegate(), 0, 0)}
p := tea.NewProgram(m)
if _, err := p.Run(); err != nil {
fmt.Println("Error running program:", err)
}
Key Differences
- tview focuses on building complete applications with a rich set of widgets
- Bubbles provides modular components that can be easily combined
- tview uses a more traditional object-oriented approach
- Bubbles follows a more functional and composable design pattern
Use Cases
- Choose tview for complex, full-screen terminal applications with multiple layouts
- Opt for Bubbles when building smaller CLI tools or when you need highly customizable components
Both libraries offer powerful tools for creating terminal user interfaces, but they cater to different development styles and project requirements.
Tcell is an alternate terminal package, similar in some ways to termbox, but better in others.
Pros of tcell
- Lower-level library providing more fine-grained control over terminal operations
- Broader platform support, including Windows
- More mature and established project with a larger user base
Cons of tcell
- Steeper learning curve due to its lower-level nature
- Requires more boilerplate code to create interactive terminal applications
- Less opinionated, which may lead to more inconsistent user interfaces
Code Comparison
tcell:
screen, _ := tcell.NewScreen()
screen.Init()
defer screen.Fini()
screen.SetContent(0, 0, 'H', nil, tcell.StyleDefault)
screen.Show()
bubbles:
m := model{}
p := tea.NewProgram(m)
if _, err := p.Run(); err != nil {
fmt.Printf("Alas, there's been an error: %v", err)
}
Summary
tcell is a lower-level terminal library offering more control and broader platform support, while bubbles provides a higher-level, more opinionated approach for creating interactive terminal user interfaces. tcell may be preferred for projects requiring fine-grained control or cross-platform compatibility, while bubbles excels in rapid development of consistent, interactive TUIs with less boilerplate code.
Minimalist Go package aimed at creating Console User Interfaces.
Pros of gocui
- More mature and established project with a longer history
- Provides lower-level control over the terminal UI
- Better suited for complex, full-screen terminal applications
Cons of gocui
- Steeper learning curve and more complex API
- Less active development and fewer recent updates
- Lacks some modern features and conveniences found in Bubbles
Code Comparison
gocui example:
g, err := gocui.NewGui(gocui.OutputNormal)
if err != nil {
log.Panicln(err)
}
defer g.Close()
g.SetManagerFunc(layout)
Bubbles example:
m := model{list: list.New(items, list.NewDefaultDelegate(), 0, 0)}
p := tea.NewProgram(m)
if _, err := p.Run(); err != nil {
fmt.Println("Error running program:", err)
os.Exit(1)
}
Both libraries offer ways to create terminal user interfaces in Go, but they differ in their approach and level of abstraction. gocui provides more fine-grained control over the UI elements, while Bubbles offers a higher-level, more user-friendly API with pre-built components. The choice between them depends on the specific requirements of your project and your preferred level of control over the UI implementation.
Golang terminal dashboard
Pros of termui
- More comprehensive set of UI components out-of-the-box
- Established project with a longer history and larger community
- Supports both mouse and keyboard input
Cons of termui
- Less active development and maintenance
- Steeper learning curve for beginners
- Limited flexibility for custom component creation
Code Comparison
termui:
ui.Init()
p := widgets.NewParagraph()
p.Text = "Hello World!"
ui.Render(p)
bubbles:
p := paragraph.New()
p.SetContent("Hello World!")
m := model{paragraph: p}
tea.NewProgram(m).Run()
Summary
termui offers a more comprehensive set of UI components and has a longer history in the Go terminal UI ecosystem. It provides built-in support for both mouse and keyboard input, which can be advantageous for certain applications.
On the other hand, bubbles, part of the Charm framework, focuses on simplicity and composability. It has a more active development cycle and is designed to be more flexible for creating custom components. bubbles also integrates seamlessly with other Charm tools, making it a good choice for developers already using the Charm ecosystem.
The code comparison shows that termui has a slightly more straightforward initialization process, while bubbles requires setting up a model and program structure. This reflects bubbles' focus on composability and state management, which can lead to more maintainable code in complex applications.
Pure Go termbox implementation
Pros of termbox-go
- Lower-level API, offering more fine-grained control over terminal output
- Lightweight and minimal dependencies
- Cross-platform support for various terminal types
Cons of termbox-go
- Requires more boilerplate code for common UI elements
- Less intuitive for creating complex, interactive terminal interfaces
- Limited built-in widgets and components
Code Comparison
termbox-go:
err := termbox.Init()
if err != nil {
panic(err)
}
defer termbox.Close()
termbox.SetOutputMode(termbox.Output256)
bubbles:
p := tea.NewProgram(initialModel())
if err := p.Start(); err != nil {
fmt.Printf("Alas, there's been an error: %v", err)
os.Exit(1)
}
Summary
termbox-go provides a lower-level API for terminal manipulation, offering more control but requiring more code for common UI elements. It's lightweight and cross-platform but lacks built-in widgets.
bubbles, part of the Charm framework, offers a higher-level API with pre-built components and a more intuitive approach to creating interactive terminal UIs. It simplifies development but may have a steeper learning curve for those familiar with lower-level APIs.
Choose termbox-go for fine-grained control and minimal dependencies, or bubbles for rapid development of feature-rich terminal applications with less boilerplate code.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Bubbles
Some components for Bubble Tea applications. These components are used in production in Glow, Charm and many other applications.
Spinner
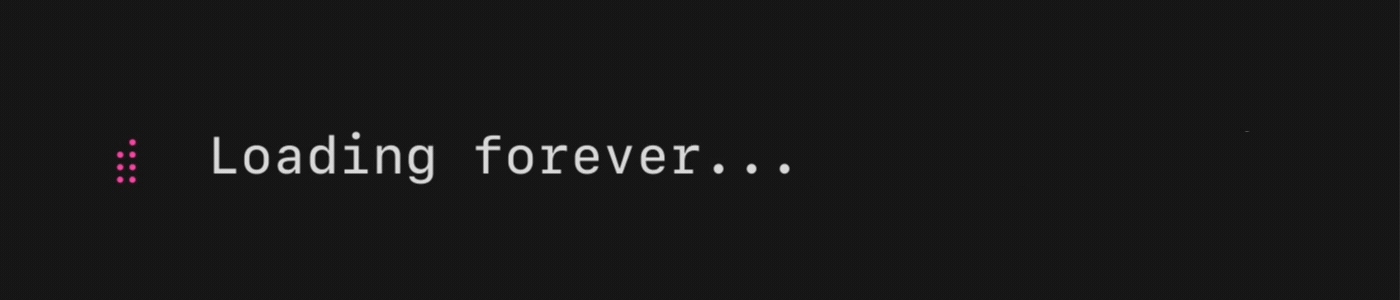
A spinner, useful for indicating that some kind an operation is happening. There are a couple default ones, but you can also pass your own âframes.â
Text Input
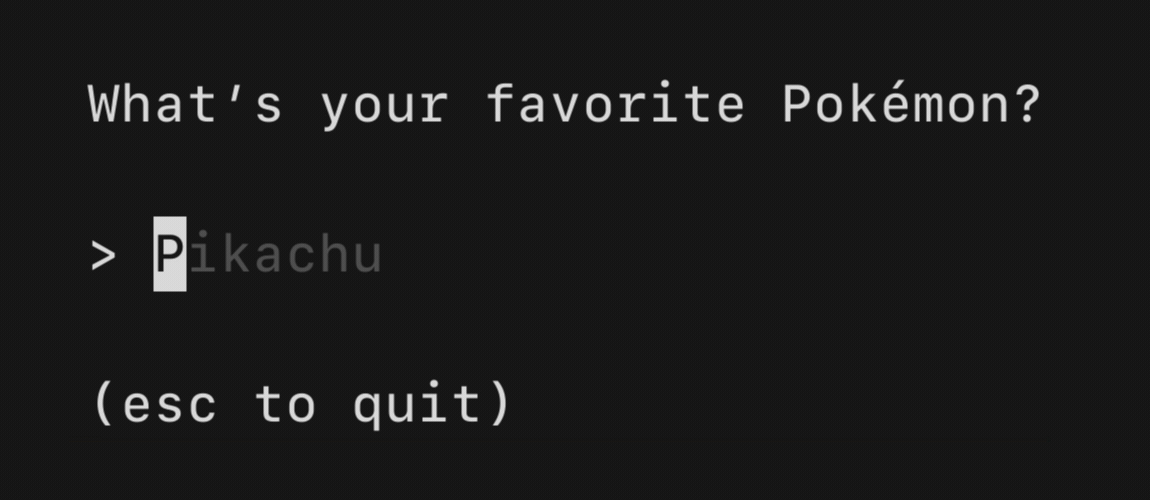
A text input field, akin to an <input type="text">
in HTML. Supports unicode,
pasting, in-place scrolling when the value exceeds the width of the element and
the common, and many customization options.
Text Area
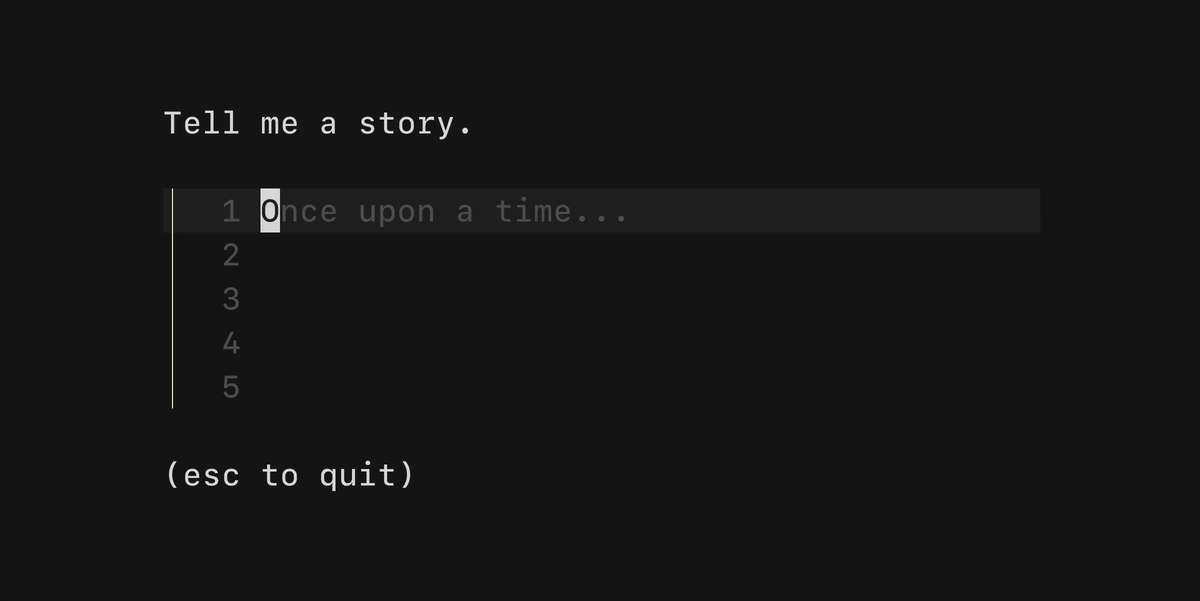
A text area field, akin to an <textarea />
in HTML. Allows for input that
spans multiple lines. Supports unicode, pasting, vertical scrolling when the
value exceeds the width and height of the element, and many customization
options.
Table
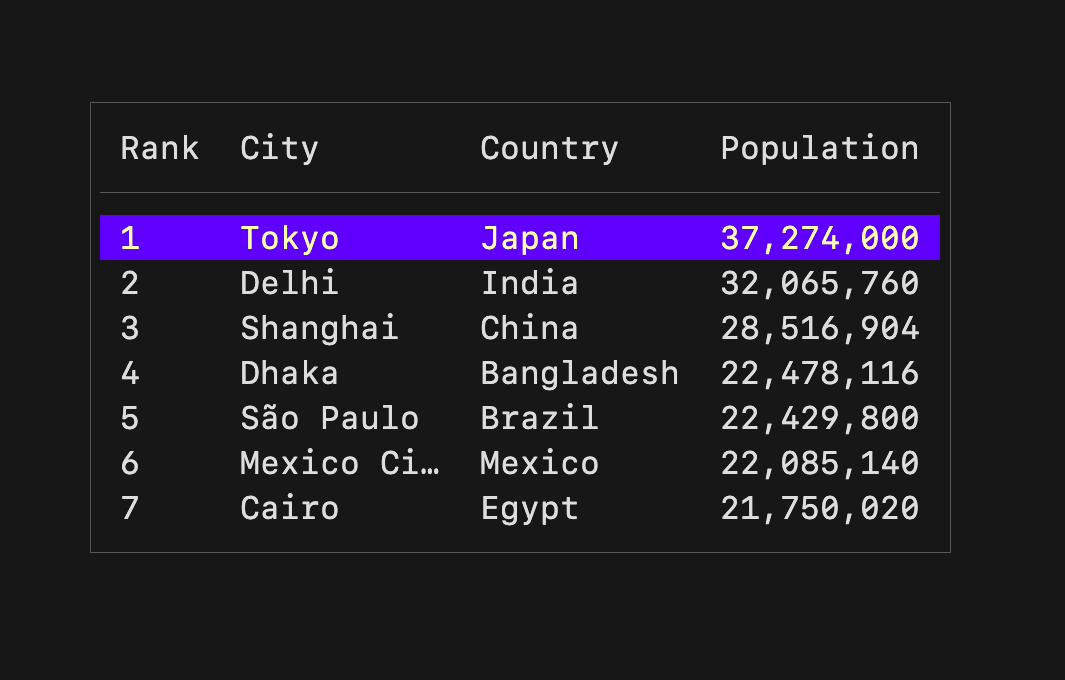
A component for displaying and navigating tabular data (columns and rows). Supports vertical scrolling and many customization options.
Progress

A simple, customizable progress meter, with optional animation via Harmonica. Supports solid and gradient fills. The empty and filled runes can be set to whatever you'd like. The percentage readout is customizable and can also be omitted entirely.
Paginator
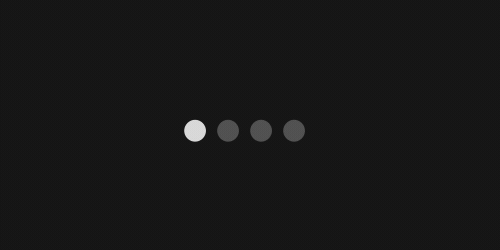
A component for handling pagination logic and optionally drawing pagination UI. Supports "dot-style" pagination (similar to what you might see on iOS) and numeric page numbering, but you could also just use this component for the logic and visualize pagination however you like.
Viewport
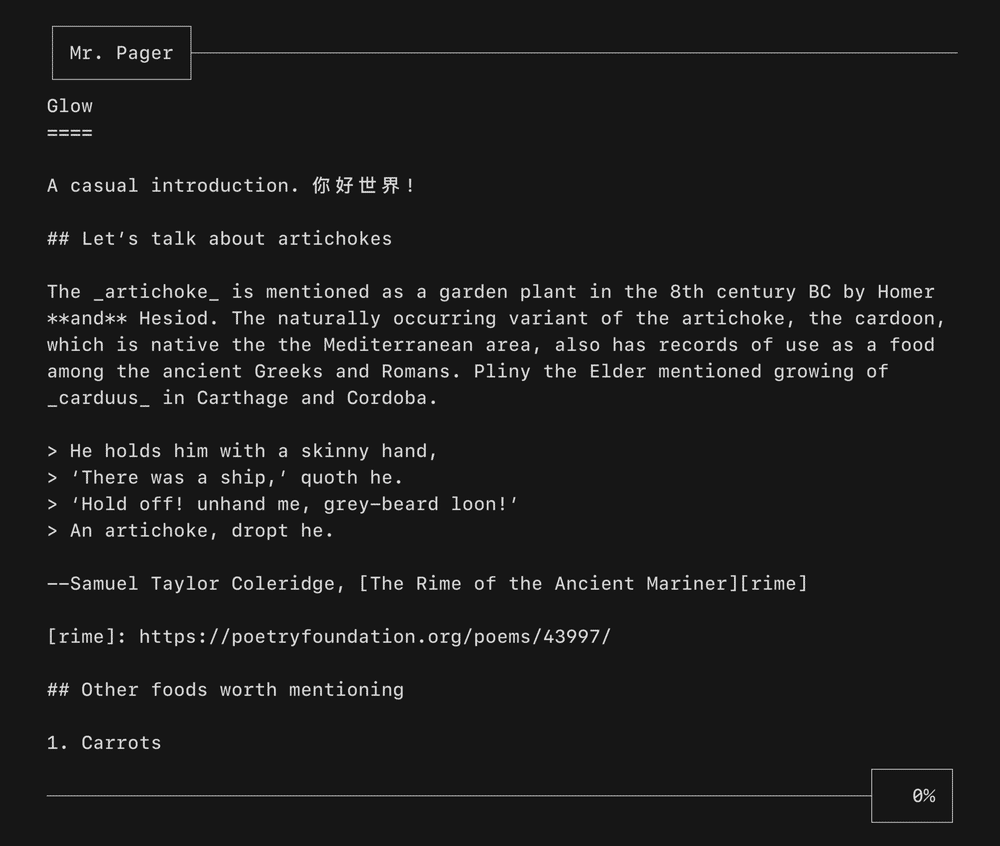
A viewport for vertically scrolling content. Optionally includes standard pager keybindings and mouse wheel support. A high performance mode is available for applications which make use of the alternate screen buffer.
This component is well complemented with Reflow for ANSI-aware indenting and text wrapping.
List
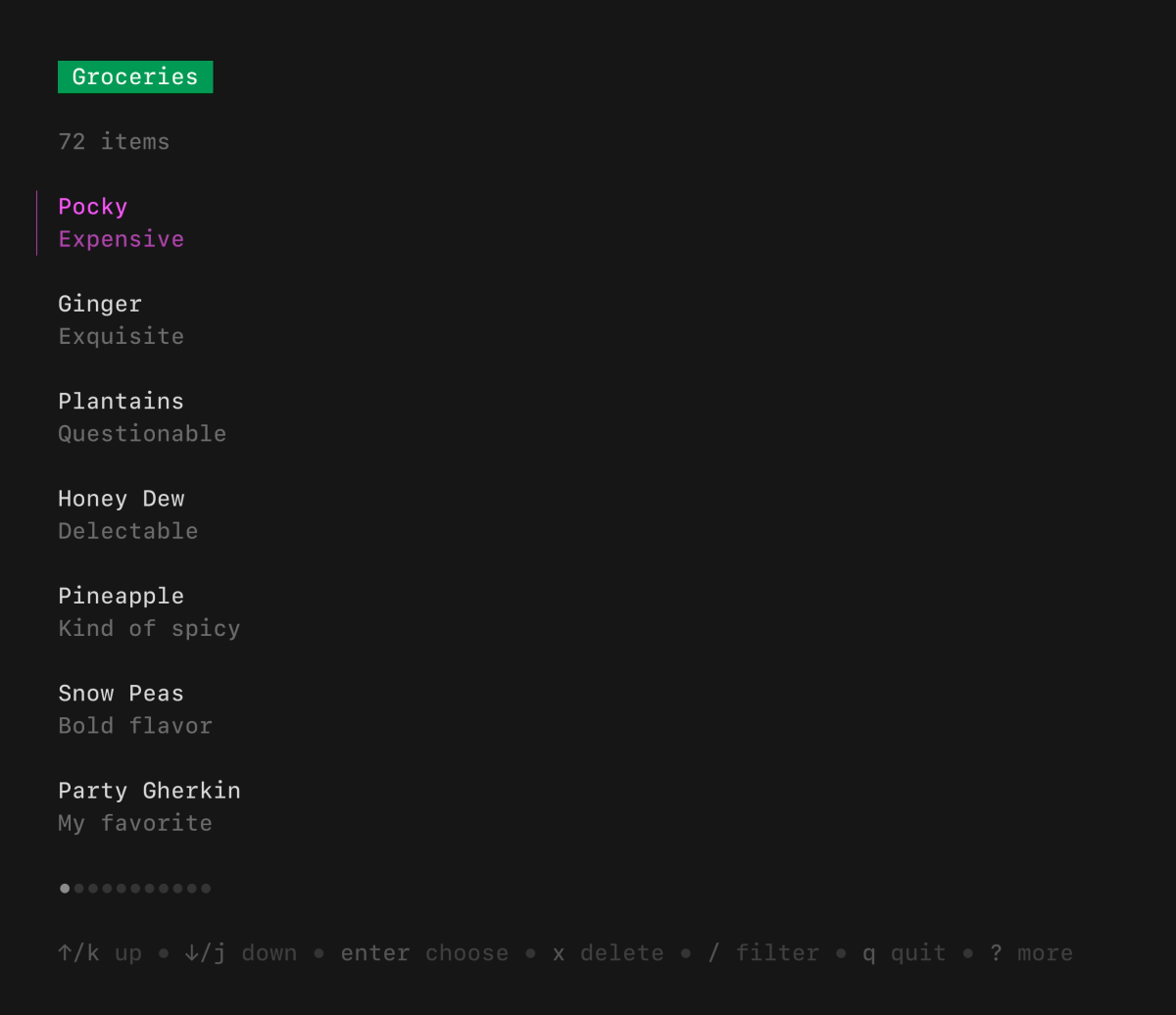
A customizable, batteries-included component for browsing a set of items. Features pagination, fuzzy filtering, auto-generated help, an activity spinner, and status messages, all of which can be enabled and disabled as needed. Extrapolated from Glow.
File Picker
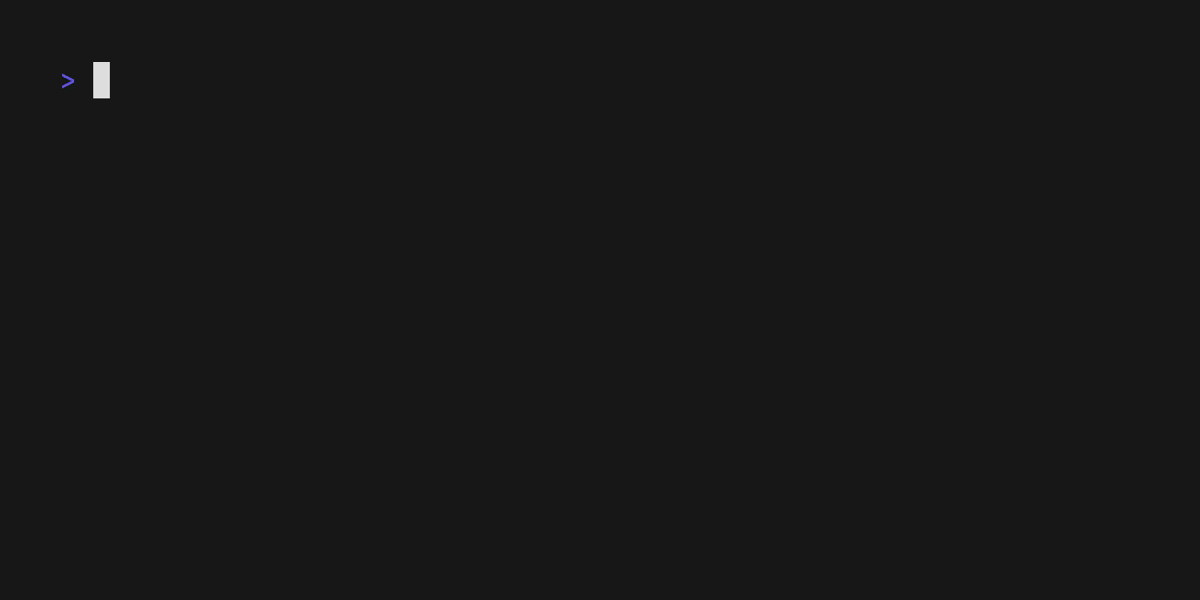
A customizable component for picking a file from the file system. Navigate through directories and select files, optionally limit to certain file extensions.
Timer
A simple, flexible component for counting down. The update frequency and output can be customized as you like.
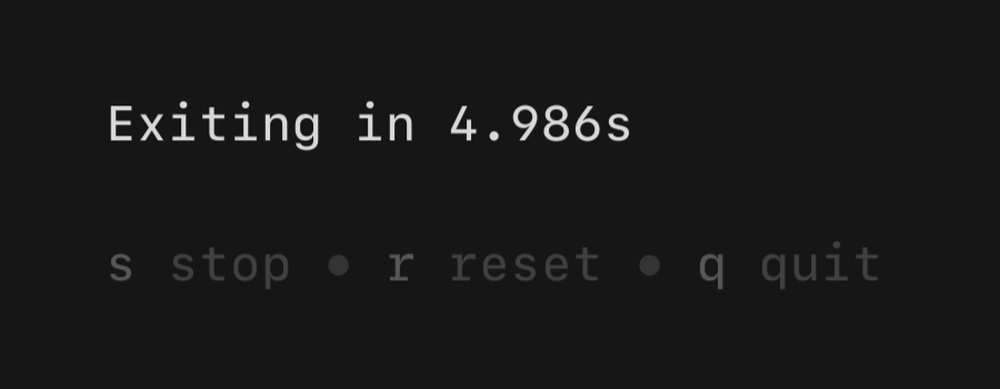
Stopwatch
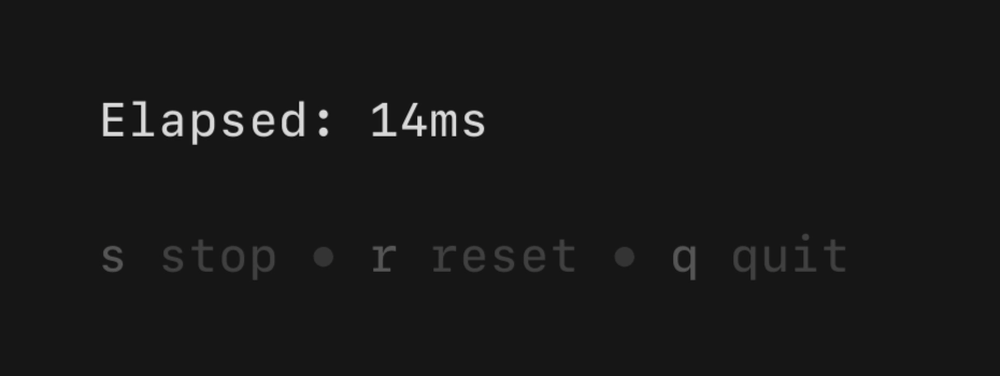
A simple, flexible component for counting up. The update frequency and output can be customized as you see fit.
Help
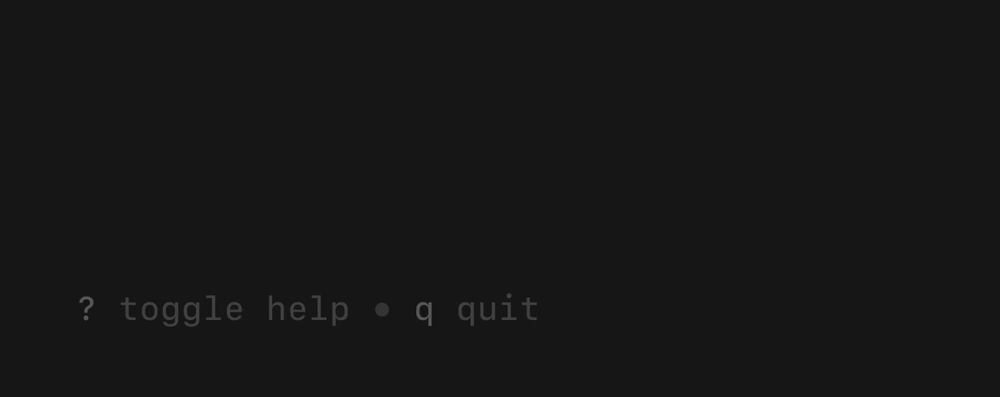
A customizable horizontal mini help view that automatically generates itself from your keybindings. It features single and multi-line modes, which the user can optionally toggle between. It will truncate gracefully if the terminal is too wide for the content.
Key
A non-visual component for managing keybindings. Itâs useful for allowing users to remap keybindings as well as generating help views corresponding to your keybindings.
type KeyMap struct {
Up key.Binding
Down key.Binding
}
var DefaultKeyMap = KeyMap{
Up: key.NewBinding(
key.WithKeys("k", "up"), // actual keybindings
key.WithHelp("â/k", "move up"), // corresponding help text
),
Down: key.NewBinding(
key.WithKeys("j", "down"),
key.WithHelp("â/j", "move down"),
),
}
func (m Model) Update(msg tea.Msg) (tea.Model, tea.Cmd) {
switch msg := msg.(type) {
case tea.KeyMsg:
switch {
case key.Matches(msg, DefaultKeyMap.Up):
// The user pressed up
case key.Matches(msg, DefaultKeyMap.Down):
// The user pressed down
}
}
return m, nil
}
Additional Bubbles
- 76creates/stickers: Responsive flexbox and table components.
- calyptia/go-bubble-table: An interactive, customizable, scrollable table component.
- erikgeiser/promptkit: A collection of common prompts for cases like selection, text input, and confirmation. Each prompt comes with sensible defaults, remappable keybindings, any many customization options.
- evertras/bubble-table: Interactive, customizable, paginated tables.
- knipferrc/teacup: Various handy bubbles and utilities for building Bubble Tea applications.
- mritd/bubbles: Some general-purpose bubbles. Inputs with validation, menu selection, a modified progressbar, and so on.
- kevm/bubbleo: A set of bubbles with a focus on navigation: navigation stacks, breakcrumbs, menus and so on.
- treilik/bubbleboxer: Layout multiple bubbles side-by-side in a layout-tree.
- treilik/bubblelister: An alternate list that is scrollable without pagination and has the ability to contain other bubbles as list items.
If youâve built a Bubble you think should be listed here, please create a Pull Request. Please note that for a project to be included, it must meet the following requirements:
- The README has a demo GIF.
- The README clearly states the purpose of the bubble along with an example on how to use it.
- The bubble must always be in a working state on its
main
branch.
Thank you!
Feedback
Weâd love to hear your thoughts on this project. Feel free to drop us a note!
License
Part of Charm.
Charmçç±å¼æº ⢠Charm loves open source
Top Related Projects
A powerful little TUI framework 🏗
Terminal UI library with rich, interactive widgets — written in Golang
Tcell is an alternate terminal package, similar in some ways to termbox, but better in others.
Minimalist Go package aimed at creating Console User Interfaces.
Golang terminal dashboard
Pure Go termbox implementation
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot