d2l-en
Interactive deep learning book with multi-framework code, math, and discussions. Adopted at 500 universities from 70 countries including Stanford, MIT, Harvard, and Cambridge.
Top Related Projects
12 weeks, 26 lessons, 52 quizzes, classic Machine Learning for all
VIP cheatsheets for Stanford's CS 229 Machine Learning
A series of Jupyter notebooks that walk you through the fundamentals of Machine Learning and Deep Learning in Python using Scikit-Learn, Keras and TensorFlow 2.
The fastai book, published as Jupyter Notebooks
An Open Source Machine Learning Framework for Everyone
Tensors and Dynamic neural networks in Python with strong GPU acceleration
Quick Overview
The d2l-ai/d2l-en repository is a collection of interactive Jupyter notebooks that provide a comprehensive introduction to deep learning. It covers a wide range of topics, from basic machine learning concepts to advanced deep learning techniques, and is designed to be a self-contained learning resource for both beginners and experienced practitioners.
Pros
- Comprehensive Coverage: The repository covers a broad range of deep learning topics, from fundamental concepts to state-of-the-art techniques, making it a valuable resource for both beginners and experienced practitioners.
- Interactive Learning: The Jupyter notebooks provide an interactive learning experience, allowing users to experiment with code and visualize the results.
- Actively Maintained: The repository is actively maintained by the Dive into Deep Learning (D2L) team, ensuring that the content is up-to-date and relevant.
- Multilingual Support: The repository is available in multiple languages, including English, Chinese, and French, making it accessible to a global audience.
Cons
- Steep Learning Curve: The content can be challenging for complete beginners, as it assumes a certain level of familiarity with machine learning and programming.
- Dependency on Jupyter Notebooks: The interactive nature of the content relies on Jupyter Notebooks, which may not be the preferred environment for all users.
- Limited Hands-on Exercises: While the notebooks provide a lot of theoretical content, there may be a need for more practical, hands-on exercises to reinforce the concepts.
- Potential Outdated Content: As the field of deep learning is rapidly evolving, some of the content in the repository may become outdated over time, requiring regular updates.
Code Examples
Here are a few code examples from the d2l-ai/d2l-en repository:
- Implementing a Simple Neural Network:
import torch
from torch import nn
# Define the model
model = nn.Sequential(
nn.Flatten(),
nn.Linear(784, 256),
nn.ReLU(),
nn.Linear(256, 10)
)
# Define the loss function and optimizer
loss_fn = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.1)
# Train the model
for epoch in range(10):
# Forward pass
pred = model(X_train)
loss = loss_fn(pred, y_train)
# Backward pass and optimization
optimizer.zero_grad()
loss.backward()
optimizer.step()
This code example demonstrates how to implement a simple neural network using PyTorch, including defining the model, loss function, and optimizer, and training the model.
- Convolutional Neural Network for Image Classification:
import torch.nn as nn
# Define the CNN model
class CNN(nn.Module):
def __init__(self):
super(CNN, self).__init__()
self.conv1 = nn.Conv2d(1, 6, 5)
self.pool = nn.MaxPool2d(2, 2)
self.conv2 = nn.Conv2d(6, 16, 5)
self.fc1 = nn.Linear(16 * 5 * 5, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
def forward(self, x):
x = self.pool(F.relu(self.conv1(x)))
x = self.pool(F.relu(self.conv2(x)))
x = x.view(-1, 16 * 5 * 5)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.fc3(x)
return x
This code example demonstrates how to implement a Convolutional Neural Network (CNN) for image classification using PyTorch, including defining the model architecture and the forward pass.
- Recurrent Neural Network for Sequence Modeling:
import torch.nn as nn
# Define the RNN model
class RNN(nn.Module):
def __init__(
Competitor Comparisons
12 weeks, 26 lessons, 52 quizzes, classic Machine Learning for all
Pros of ML-For-Beginners
- More beginner-friendly with a structured curriculum and clear learning path
- Covers a wider range of ML topics, including ethics and real-world applications
- Includes hands-on projects and quizzes for interactive learning
Cons of ML-For-Beginners
- Less in-depth coverage of advanced topics compared to d2l-en
- Fewer code examples and mathematical explanations
- Limited focus on deep learning compared to d2l-en's comprehensive approach
Code Comparison
ML-For-Beginners (Python):
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
d2l-en (PyTorch):
def load_array(data_arrays, batch_size, is_train=True):
dataset = data.TensorDataset(*data_arrays)
return data.DataLoader(dataset, batch_size, shuffle=is_train)
Both repositories offer valuable resources for learning machine learning, but they cater to different audiences and learning styles. ML-For-Beginners is more suitable for newcomers to the field, while d2l-en provides a deeper, more comprehensive approach to machine learning and deep learning concepts.
VIP cheatsheets for Stanford's CS 229 Machine Learning
Pros of stanford-cs-229-machine-learning
- Concise and focused content, ideal for quick reference
- Visually appealing cheat sheets for easy comprehension
- Covers a wide range of ML topics in a compact format
Cons of stanford-cs-229-machine-learning
- Less in-depth explanations compared to d2l-en
- Limited code examples and practical implementations
- May not be suitable for beginners without prior ML knowledge
Code Comparison
d2l-en provides more comprehensive code examples:
def train_epoch(net, train_iter, loss, updater):
metric = Accumulator(3)
for X, y in train_iter:
y_hat = net(X)
l = loss(y_hat, y)
l.backward()
updater.step()
metric.add(float(l) * len(y), accuracy(y_hat, y), len(y))
return metric[0] / metric[2], metric[1] / metric[2]
stanford-cs-229-machine-learning focuses more on theoretical concepts and formulas:
# Logistic Regression
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def cost_function(h, y):
return (-y * np.log(h) - (1 - y) * np.log(1 - h)).mean()
Both repositories offer valuable resources for learning machine learning, with d2l-en providing a more comprehensive and hands-on approach, while stanford-cs-229-machine-learning offers concise summaries and quick reference materials.
A series of Jupyter notebooks that walk you through the fundamentals of Machine Learning and Deep Learning in Python using Scikit-Learn, Keras and TensorFlow 2.
Pros of handson-ml2
- More focused on practical implementation and hands-on coding exercises
- Covers a wider range of machine learning topics, including traditional ML algorithms
- Includes more detailed explanations of code snippets and implementation details
Cons of handson-ml2
- Less emphasis on theoretical foundations and mathematical concepts
- Fewer in-depth explanations of advanced deep learning architectures
- Limited coverage of cutting-edge research topics in AI and deep learning
Code Comparison
handson-ml2:
from sklearn.ensemble import RandomForestClassifier
rf_clf = RandomForestClassifier(n_estimators=100, random_state=42)
rf_clf.fit(X_train, y_train)
y_pred = rf_clf.predict(X_test)
d2l-en:
import torch
from torch import nn
net = nn.Sequential(nn.Linear(num_inputs, 1))
loss = nn.MSELoss()
trainer = torch.optim.SGD(net.parameters(), lr=0.03)
The handson-ml2 example demonstrates the use of scikit-learn for traditional machine learning, while d2l-en focuses on deep learning with PyTorch. This reflects the different emphases of the two repositories, with handson-ml2 covering a broader range of ML techniques and d2l-en specializing in deep learning concepts and implementations.
The fastai book, published as Jupyter Notebooks
Pros of fastbook
- More focused on practical implementation and real-world applications
- Emphasizes a top-down learning approach, starting with high-level concepts
- Integrates seamlessly with the fastai library, providing a cohesive learning experience
Cons of fastbook
- Less comprehensive coverage of theoretical foundations compared to d2l-en
- Primarily focuses on PyTorch, limiting exposure to other deep learning frameworks
- May be less suitable for readers seeking a rigorous mathematical treatment
Code Comparison
fastbook example:
from fastai.vision.all import *
path = untar_data(URLs.PETS)/'images'
def is_cat(x): return x[0].isupper()
dls = ImageDataLoaders.from_name_func(
path, get_image_files(path), valid_pct=0.2, seed=42,
label_func=is_cat, item_tfms=Resize(224))
d2l-en example:
import torch
from torch import nn
from d2l import torch as d2l
net = nn.Sequential(nn.Linear(4, 8), nn.ReLU(), nn.Linear(8, 1))
X = torch.rand(size=(2, 4))
y = net(X).sum()
An Open Source Machine Learning Framework for Everyone
Pros of TensorFlow
- Comprehensive machine learning framework with extensive tools and libraries
- Strong industry adoption and support from Google
- Excellent performance optimization and scalability for large-scale deployments
Cons of TensorFlow
- Steeper learning curve compared to D2L-en's educational focus
- Less emphasis on teaching concepts; more geared towards practical implementation
- Larger codebase and more complex architecture
Code Comparison
D2L-en (educational focus):
def softmax(X):
X_exp = torch.exp(X)
partition = X_exp.sum(1, keepdim=True)
return X_exp / partition
TensorFlow (production-ready):
def softmax(logits, axis=-1):
return tf.nn.softmax(logits, axis=axis)
Summary
D2L-en is designed for learning deep learning concepts with clear, educational code examples. TensorFlow, on the other hand, is a production-grade framework focused on performance and scalability. While D2L-en prioritizes understanding, TensorFlow offers a more comprehensive toolkit for real-world machine learning applications.
Tensors and Dynamic neural networks in Python with strong GPU acceleration
Pros of PyTorch
- Larger community and more extensive ecosystem
- More flexible and dynamic computational graph
- Better support for production deployment
Cons of PyTorch
- Steeper learning curve for beginners
- Less focus on educational content and tutorials
- More complex API for some basic operations
Code Comparison
d2l-en:
import torch
from torch import nn
net = nn.Sequential(nn.Linear(20, 256), nn.ReLU(), nn.Linear(256, 10))
X = torch.rand(2, 20)
Y = net(X)
PyTorch:
import torch
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(20, 256)
self.fc2 = nn.Linear(256, 10)
def forward(self, x):
x = torch.relu(self.fc1(x))
return self.fc2(x)
net = Net()
X = torch.rand(2, 20)
Y = net(X)
The d2l-en example uses a more concise Sequential model, while the PyTorch example demonstrates a custom Module class, offering more flexibility but requiring more code.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
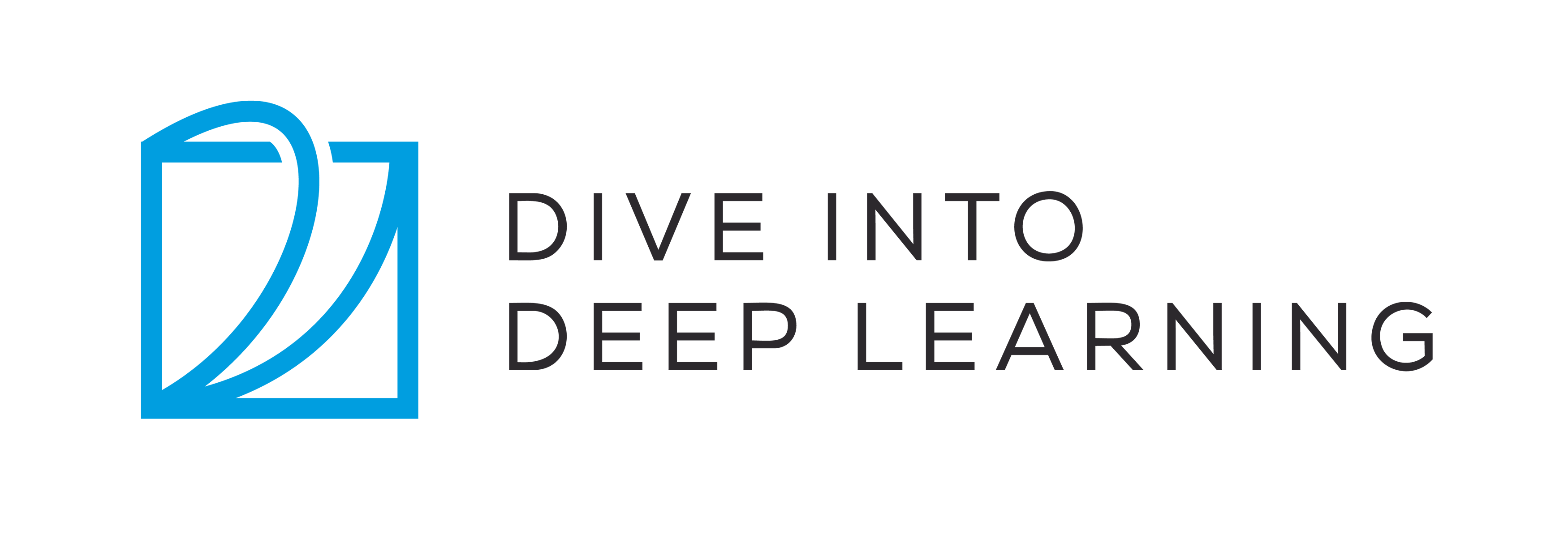
D2L.ai: Interactive Deep Learning Book with Multi-Framework Code, Math, and Discussions
Book website | STAT 157 Course at UC Berkeley
The best way to understand deep learning is learning by doing.
This open-source book represents our attempt to make deep learning approachable, teaching you the concepts, the context, and the code. The entire book is drafted in Jupyter notebooks, seamlessly integrating exposition figures, math, and interactive examples with self-contained code.
Our goal is to offer a resource that could
- be freely available for everyone;
- offer sufficient technical depth to provide a starting point on the path to actually becoming an applied machine learning scientist;
- include runnable code, showing readers how to solve problems in practice;
- allow for rapid updates, both by us and also by the community at large;
- be complemented by a forum for interactive discussion of technical details and to answer questions.
Universities Using D2L
If you find this book useful, please star (â ) this repository or cite this book using the following bibtex entry:
@book{zhang2023dive,
title={Dive into Deep Learning},
author={Zhang, Aston and Lipton, Zachary C. and Li, Mu and Smola, Alexander J.},
publisher={Cambridge University Press},
note={\url{https://D2L.ai}},
year={2023}
}
Endorsements
"In less than a decade, the AI revolution has swept from research labs to broad industries to every corner of our daily life. Dive into Deep Learning is an excellent text on deep learning and deserves attention from anyone who wants to learn why deep learning has ignited the AI revolution: the most powerful technology force of our time."
— Jensen Huang, Founder and CEO, NVIDIA
"This is a timely, fascinating book, providing with not only a comprehensive overview of deep learning principles but also detailed algorithms with hands-on programming code, and moreover, a state-of-the-art introduction to deep learning in computer vision and natural language processing. Dive into this book if you want to dive into deep learning!"
— Jiawei Han, Michael Aiken Chair Professor, University of Illinois at Urbana-Champaign
"This is a highly welcome addition to the machine learning literature, with a focus on hands-on experience implemented via the integration of Jupyter notebooks. Students of deep learning should find this invaluable to become proficient in this field."
— Bernhard Schölkopf, Director, Max Planck Institute for Intelligent Systems
"Dive into Deep Learning strikes an excellent balance between hands-on learning and in-depth explanation. I've used it in my deep learning course and recommend it to anyone who wants to develop a thorough and practical understanding of deep learning."
— Colin Raffel, Assistant Professor, University of North Carolina, Chapel Hill
Contributing (Learn How)
This open source book has benefited from pedagogical suggestions, typo corrections, and other improvements from community contributors. Your help is valuable for making the book better for everyone.
Dear D2L contributors, please email your GitHub ID and name to d2lbook.en AT gmail DOT com so your name will appear on the acknowledgments. Thanks.
License Summary
This open source book is made available under the Creative Commons Attribution-ShareAlike 4.0 International License. See LICENSE file.
The sample and reference code within this open source book is made available under a modified MIT license. See the LICENSE-SAMPLECODE file.
Chinese version | Discuss and report issues | Code of conduct
Top Related Projects
12 weeks, 26 lessons, 52 quizzes, classic Machine Learning for all
VIP cheatsheets for Stanford's CS 229 Machine Learning
A series of Jupyter notebooks that walk you through the fundamentals of Machine Learning and Deep Learning in Python using Scikit-Learn, Keras and TensorFlow 2.
The fastai book, published as Jupyter Notebooks
An Open Source Machine Learning Framework for Everyone
Tensors and Dynamic neural networks in Python with strong GPU acceleration
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot