dva
🌱 React and redux based, lightweight and elm-style framework. (Inspired by elm and choo)
Top Related Projects
Quick Overview
DVA is a lightweight front-end framework based on Redux, Redux-saga, and React-router. It provides a simple and intuitive way to handle complex application states and side effects in React applications. DVA aims to reduce boilerplate code and simplify the development process for React developers.
Pros
- Simplifies Redux and Redux-saga integration
- Provides a clear and organized structure for managing application state
- Reduces boilerplate code compared to vanilla Redux
- Offers built-in support for asynchronous actions and side effects
Cons
- Learning curve for developers new to Redux and Redux-saga concepts
- May be overkill for small, simple applications
- Limited flexibility compared to using Redux directly
- Less active development and community support compared to some alternatives
Code Examples
- Defining a model:
export default {
namespace: 'counter',
state: {
count: 0,
},
reducers: {
increment(state) {
return { ...state, count: state.count + 1 };
},
},
effects: {
*incrementAsync(action, { call, put }) {
yield call(delay, 1000);
yield put({ type: 'increment' });
},
},
};
- Connecting a component to the store:
import { connect } from 'dva';
const Counter = ({ count, dispatch }) => (
<div>
<h2>{count}</h2>
<button onClick={() => dispatch({ type: 'counter/increment' })}>+</button>
<button onClick={() => dispatch({ type: 'counter/incrementAsync' })}>+ Async</button>
</div>
);
export default connect(({ counter }) => ({
count: counter.count,
}))(Counter);
- Setting up the DVA app:
import dva from 'dva';
import counterModel from './models/counter';
const app = dva();
app.model(counterModel);
app.router(require('./router').default);
app.start('#root');
Getting Started
- Install DVA:
npm install dva
- Create a new DVA app:
import dva from 'dva';
import './index.css';
const app = dva();
app.model(require('./models/example').default);
app.router(require('./router').default);
app.start('#root');
- Define a model:
export default {
namespace: 'example',
state: {},
reducers: {},
effects: {},
};
- Create a route component:
import React from 'react';
import { connect } from 'dva';
const IndexPage = () => {
return (
<div>
<h1>Welcome to DVA</h1>
</div>
);
};
export default connect()(IndexPage);
Competitor Comparisons
The library for web and native user interfaces.
Pros of React
- Larger ecosystem and community support
- More flexible and can be used with various state management solutions
- Better performance for large-scale applications
Cons of React
- Steeper learning curve for beginners
- Requires additional libraries for routing and state management
- More boilerplate code for setting up a complete application
Code Comparison
React:
import React from 'react';
import { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return <button onClick={() => setCount(count + 1)}>{count}</button>;
}
DVA:
import dva from 'dva';
const app = dva();
app.model({
namespace: 'count',
state: 0,
reducers: {
add(state) { return state + 1; },
},
});
DVA is built on top of React and Redux, providing a more opinionated and integrated approach to building React applications. It simplifies the process of combining React, Redux, and Redux-saga, making it easier for developers to get started with a complete solution. However, React offers more flexibility and is better suited for larger, more complex applications that may require custom state management solutions.
A JS library for predictable global state management
Pros of Redux
- More widely adopted and mature ecosystem
- Flexible and can be used with any UI library
- Extensive middleware support for advanced features
Cons of Redux
- Steeper learning curve and more boilerplate code
- Requires additional libraries for async operations
- Can be overkill for smaller applications
Code Comparison
Redux:
const reducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
default:
return state;
}
};
Dva:
app.model({
namespace: 'count',
state: { count: 0 },
reducers: {
increment(state) {
return { ...state, count: state.count + 1 };
},
},
});
Redux requires more setup and explicit action creators, while Dva simplifies the process with a more declarative approach. Dva integrates effects and subscriptions directly into the model, whereas Redux typically relies on middleware for such functionality.
Redux is better suited for large-scale applications with complex state management needs, while Dva offers a more streamlined experience for React applications, especially those built with the Ant Design ecosystem.
A framework in react community ✨
Pros of umi
- More comprehensive framework with built-in routing, state management, and plugins
- Supports server-side rendering and TypeScript out of the box
- Offers a plugin system for extending functionality
Cons of umi
- Steeper learning curve due to its more complex architecture
- Less flexibility for custom configurations compared to dva
- Potentially overkill for smaller projects
Code comparison
umi:
import { defineConfig } from 'umi';
export default defineConfig({
routes: [{ path: '/', component: '@/pages/index' }],
});
dva:
import dva from 'dva';
const app = dva();
app.model(require('./models/example').default);
app.router(require('./router').default);
app.start('#root');
Key differences
- umi provides a more opinionated structure with built-in features
- dva focuses on state management and is more lightweight
- umi uses a configuration file for setup, while dva uses a more programmatic approach
Use cases
- Choose umi for larger, more complex applications that benefit from its integrated features
- Opt for dva when you need a simple, flexible state management solution for React applications
Community and ecosystem
- umi has a larger community and more frequent updates
- dva is more focused and has a smaller, but dedicated user base
The Redux Framework
Pros of Rematch
- Simpler API and less boilerplate code
- Better TypeScript support out of the box
- More flexible plugin system for extending functionality
Cons of Rematch
- Smaller community and ecosystem compared to Dva
- Less opinionated, which may lead to inconsistent patterns across projects
- Lacks built-in routing integration
Code Comparison
Dva:
const app = dva();
app.model({
namespace: 'count',
state: 0,
reducers: {
add(state) { return state + 1 },
},
});
app.router(() => <App />);
app.start('#root');
Rematch:
const count = {
state: 0,
reducers: {
add(state) { return state + 1 },
},
};
const store = init({ models: { count } });
Both Dva and Rematch are Redux-based state management libraries for React applications. Dva provides a more comprehensive solution with built-in effects handling and routing integration, while Rematch focuses on simplifying Redux usage with a more straightforward API.
Dva has a larger community and more extensive documentation, making it easier for beginners to get started. However, Rematch offers better TypeScript support and a more flexible plugin system, which can be advantageous for more complex projects.
The choice between Dva and Rematch ultimately depends on the specific needs of your project and your team's preferences for state management patterns.
Simple, scalable state management.
Pros of MobX
- More flexible and can be used with any UI framework, not just React
- Simpler learning curve with less boilerplate code
- Better performance for large-scale applications due to fine-grained reactivity
Cons of MobX
- Less opinionated structure, which can lead to inconsistent code organization
- Potential for overuse of observables, causing unnecessary re-renders
- Lacks built-in middleware support for handling side effects
Code Comparison
MobX:
import { makeObservable, observable, action } from "mobx";
class TodoStore {
todos = [];
constructor() {
makeObservable(this, {
todos: observable,
addTodo: action
});
}
addTodo(text) {
this.todos.push({ text, completed: false });
}
}
Dva:
import dva from 'dva';
const app = dva();
app.model({
namespace: 'todos',
state: [],
reducers: {
add(state, { payload: todo }) {
return [...state, todo];
},
},
});
Summary
MobX offers more flexibility and simplicity, making it suitable for various project sizes and frameworks. It excels in performance for complex applications but may require more discipline in code organization. Dva, built on Redux, provides a more structured approach with built-in side effect handling, but has a steeper learning curve and is primarily designed for React applications.
🗃️ Centralized State Management for Vue.js.
Pros of Vuex
- Tightly integrated with Vue.js ecosystem
- Extensive documentation and large community support
- Built-in devtools for easier debugging and state inspection
Cons of Vuex
- More boilerplate code required for complex applications
- Steeper learning curve for beginners
- Limited to Vue.js applications
Code Comparison
Vuex:
const store = new Vuex.Store({
state: { count: 0 },
mutations: {
increment(state) { state.count++ }
}
})
Dva:
const app = dva();
app.model({
namespace: 'count',
state: 0,
reducers: {
increment(state) { return state + 1; }
}
});
Key Differences
- Dva combines Redux, Redux-saga, and React-router, while Vuex is specifically designed for Vue.js
- Dva uses a more declarative approach with models, while Vuex relies on mutations and actions
- Dva supports middleware out of the box, whereas Vuex requires plugins for similar functionality
Use Cases
- Choose Vuex for Vue.js applications, especially those with complex state management needs
- Opt for Dva when building React applications that require integrated routing and side-effect management
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
English | ç®ä½ä¸æ
dva
Lightweight front-end framework based on redux, redux-saga and react-router. (Inspired by elm and choo)
Features
- Easy to learn, easy to use: only 6 apis, very friendly to redux users, and API reduce to 0 when use with umi
- Elm concepts: organize models with
reducers
,effects
andsubscriptions
- Support HMR: support HMR for components, routes and models with babel-plugin-dva-hmr
- Plugin system: e.g. we have dva-loading plugin to handle loading state automatically
Demos
- Count: Simple count example
- User Dashboard: User management dashboard
- AntDesign Proï¼(Demo)ï¼out-of-box UI solution for enterprise applications
- HackerNews: (Demo)ï¼HackerNews Clone
- antd-admin: (Demo)ï¼A admin dashboard application demo built upon Ant Design and Dva.js
- github-stars: (Demo)ï¼Github star management application
- Account System: A small inventory management system
- react-native-dva-starter: react-native example integrated dva and react-navigation
Quick Start
More documentation, checkout https://dvajs.com/
FAQ
Why is it called dva?
D.Vaâs mech is nimble and powerful â its twin Fusion Cannons blast away with autofire at short range, and she can use its Boosters to barrel over enemies and obstacles, or deflect attacks with her projectile-dismantling Defense Matrix.
ââ From OverWatch
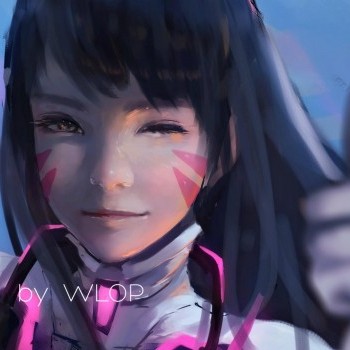
Is it production ready?
Sure! We have 1000+ projects using dva in Alibaba.
Does it support IE8?
No.
Next
Some basic articles.
- The 8 Concepts, and know how they are connected together
- dva APIs
- Checkout dva knowledgemap, including all the basic knowledge with ES6, React, dva
- Checkout more FAQ
- If your project is created by dva-cli, checkout how to Configure it
Want more?
- çç dva çå身 React + Redux æä½³å®è·µï¼ç¥é dva æ¯æä¹æ¥ç
- å¨ gitc å享 dva ç PPT ï¼React åºç¨æ¡æ¶å¨èèéæçå®è·µ
- 妿è¿å¨ç¨ dva@1.xï¼è¯·å°½å¿« åçº§å° 2.x
Community
Slack Group | Github Issue | éé群 | 微信群 |
---|---|---|---|
sorrycc.slack.com | umijs/umi/issues | ![]() | ![]() |
License
Top Related Projects
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot