Top Related Projects
A browser based code editor
IntelliJ IDEA Community Edition & IntelliJ Platform
Eclipse Theia is a cloud & desktop IDE framework implemented in TypeScript.
A React-based UI toolkit for the web
The library for web and native user interfaces.
Visual Studio Code
Quick Overview
Enso is a hybrid visual and textual programming language with a focus on data processing and analysis. It combines visual programming with traditional coding, allowing users to create and manipulate data flows through a graphical interface while also providing the ability to write custom code when needed.
Pros
- Intuitive visual programming interface for data processing tasks
- Seamless integration of visual and textual programming paradigms
- Strong focus on data analysis and visualization
- Supports multiple programming languages within the same environment
Cons
- Relatively new project, still in active development
- Limited ecosystem compared to more established data processing tools
- Steeper learning curve for users unfamiliar with visual programming concepts
- Documentation and community support are still growing
Code Examples
import Standard.Visualization.Plot
data = [1, 2, 3, 4, 5]
labels = ["A", "B", "C", "D", "E"]
plot = Plot.bar_chart data labels
plot.show
This example creates a simple bar chart using Enso's visualization capabilities.
import Standard.Table
data = Table.from_csv "data.csv"
filtered = data.filter (row -> row.age > 30)
sorted = filtered.sort_by "salary" descending=True
result = sorted.take 10
result.to_csv "top_10_salaries.csv"
This code snippet demonstrates data manipulation using Enso, including reading from a CSV, filtering, sorting, and writing results back to a file.
import Standard.Math
numbers = [1, 2, 3, 4, 5]
squared = numbers.map (x -> x * x)
sum = squared.fold 0 (+)
print sum
This example shows functional programming concepts in Enso, including mapping and folding operations on a list of numbers.
Getting Started
To get started with Enso:
- Download and install Enso from the official website: https://enso.org/
- Launch the Enso IDE
- Create a new project by clicking "New Project" in the welcome screen
- Add a new node to your project by right-clicking in the canvas and selecting "Add Node"
- Start building your data flow by connecting nodes and adding custom code as needed
- Run your project by clicking the "Run" button in the top toolbar
For more detailed instructions and tutorials, refer to the official Enso documentation: https://docs.enso.org/
Competitor Comparisons
A browser based code editor
Pros of Monaco Editor
- Widely adopted and battle-tested in popular applications like VS Code
- Extensive documentation and community support
- Rich set of features for code editing, including syntax highlighting and IntelliSense
Cons of Monaco Editor
- Focused solely on code editing, lacking visual programming capabilities
- Heavier and more complex for simple text editing use cases
- May require additional setup for language-specific features
Code Comparison
Monaco Editor:
monaco.editor.create(document.getElementById('container'), {
value: 'function hello() {\n\tconsole.log("Hello world!");\n}',
language: 'javascript'
});
Enso:
main =
print "Hello, World!"
Summary
Monaco Editor is a powerful code editor component, while Enso is a visual programming language and environment. Monaco excels in traditional text-based coding scenarios, offering advanced features for developers. Enso, on the other hand, focuses on visual programming and data flow, making it more suitable for non-programmers and data-centric applications. The choice between them depends on the specific use case and target audience of your project.
IntelliJ IDEA Community Edition & IntelliJ Platform
Pros of IntelliJ IDEA Community Edition
- Extensive language support and robust IDE features
- Large, active community and frequent updates
- Powerful code analysis and refactoring tools
Cons of IntelliJ IDEA Community Edition
- Larger resource footprint and slower startup times
- Steeper learning curve for new users
- Limited visual programming capabilities
Code Comparison
Enso (Visual Programming):
main =
x = 5
y = 10
result = x + y
IO.println result
IntelliJ IDEA (Java):
public class Main {
public static void main(String[] args) {
int x = 5;
int y = 10;
int result = x + y;
System.out.println(result);
}
}
Enso focuses on visual programming with a concise syntax, while IntelliJ IDEA supports traditional text-based programming languages like Java. Enso's approach may be more intuitive for certain tasks, but IntelliJ IDEA offers more comprehensive features for large-scale software development across multiple languages.
Eclipse Theia is a cloud & desktop IDE framework implemented in TypeScript.
Pros of Theia
- More mature and widely adopted project with a larger community
- Extensive plugin ecosystem and compatibility with VS Code extensions
- Flexible architecture allowing for both desktop and cloud-based deployments
Cons of Theia
- Heavier resource footprint due to its comprehensive feature set
- Steeper learning curve for developers new to the Eclipse ecosystem
- Less focus on visual programming compared to Enso's core concept
Code Comparison
Theia (TypeScript):
import { injectable } from 'inversify';
import { MenuModelRegistry } from '@theia/core';
@injectable()
export class MyMenuContribution implements MenuContribution {
registerMenus(menus: MenuModelRegistry): void {
// Menu registration logic
}
}
Enso (Enso language):
type Menu_Contribution
register_menus : Menu_Model_Registry -> Nothing
my_menu_contribution : Menu_Contribution
my_menu_contribution.register_menus = menus ->
# Menu registration logic
While both projects aim to provide extensible development environments, Theia focuses on creating a versatile, web-based IDE platform, whereas Enso emphasizes visual programming and data processing workflows. Theia offers a more traditional coding experience with its text-based approach, while Enso provides a unique visual programming paradigm.
A React-based UI toolkit for the web
Pros of Blueprint
- Extensive UI component library for web applications
- Well-documented with interactive examples
- Large community and active development
Cons of Blueprint
- Focused solely on React, limiting its use in other frameworks
- Steeper learning curve due to its comprehensive nature
- Larger bundle size compared to more lightweight alternatives
Code Comparison
Blueprint (TypeScript):
import { Button, Intent } from "@blueprintjs/core";
<Button intent={Intent.PRIMARY} text="Click me" />
Enso (Enso language):
Button.new "Click me" . set_style Primary
Summary
Blueprint is a robust UI component library for React applications, offering a wide range of pre-built components and extensive documentation. It's well-suited for large-scale enterprise applications but may be overkill for smaller projects.
Enso, on the other hand, is a general-purpose programming language with visual components. It focuses on data processing and visualization, making it more versatile for various programming tasks beyond UI development.
While Blueprint excels in creating complex web interfaces, Enso provides a unique visual programming experience that can be applied to a broader range of problems, from data analysis to machine learning.
The library for web and native user interfaces.
Pros of React
- Larger community and ecosystem, with more resources and third-party libraries
- More mature and battle-tested in production environments
- Wider adoption in industry, potentially leading to more job opportunities
Cons of React
- Steeper learning curve, especially for beginners
- More complex state management for large applications
- Frequent updates and changes in best practices can be challenging to keep up with
Code Comparison
React component:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
Enso component (approximate equivalent):
type Welcome name =
Text.concat "Hello, " name
Summary
React is a widely-adopted JavaScript library for building user interfaces, while Enso is a general-purpose programming language with visual programming capabilities. React excels in web development and has a vast ecosystem, but can be more complex for beginners. Enso aims to simplify programming with its visual approach and hybrid paradigm, but has a smaller community and fewer resources available. The choice between them depends on the specific project requirements and the developer's preferences.
Visual Studio Code
Pros of VS Code
- Massive ecosystem with extensive extensions and themes
- Robust debugging capabilities for multiple languages
- Large and active community, frequent updates
Cons of VS Code
- Heavier resource usage, especially with many extensions
- Can be complex for beginners due to numerous features
- Less focused on visual programming compared to Enso
Code Comparison
VS Code (JavaScript):
const greeting = (name) => {
console.log(`Hello, ${name}!`);
};
greeting('World');
Enso (Visual Programming):
main =
name = "World"
greeting = "Hello, " + name + "!"
IO.println greeting
VS Code offers a traditional text-based coding experience, while Enso provides a visual programming environment. VS Code's code is more familiar to traditional programmers, whereas Enso's approach may be more intuitive for those new to programming or preferring visual representations.
VS Code is a versatile, feature-rich editor suitable for various programming tasks, while Enso focuses on visual programming and data analysis. VS Code has a larger user base and more extensive language support, but Enso offers a unique visual approach to coding that may appeal to certain users and use cases.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Enso.org. Get insights you can rely on. In real time.
Enso is an award-winning interactive programming language with dual visual and textual representations. It is a tool that spans the entire stack, going from high-level visualization and communication to the nitty-gritty of backend services, all in a single language. Watch the following introduction video to learn what Enso is, and how it helps companies build data workflows in minutes instead of weeks.
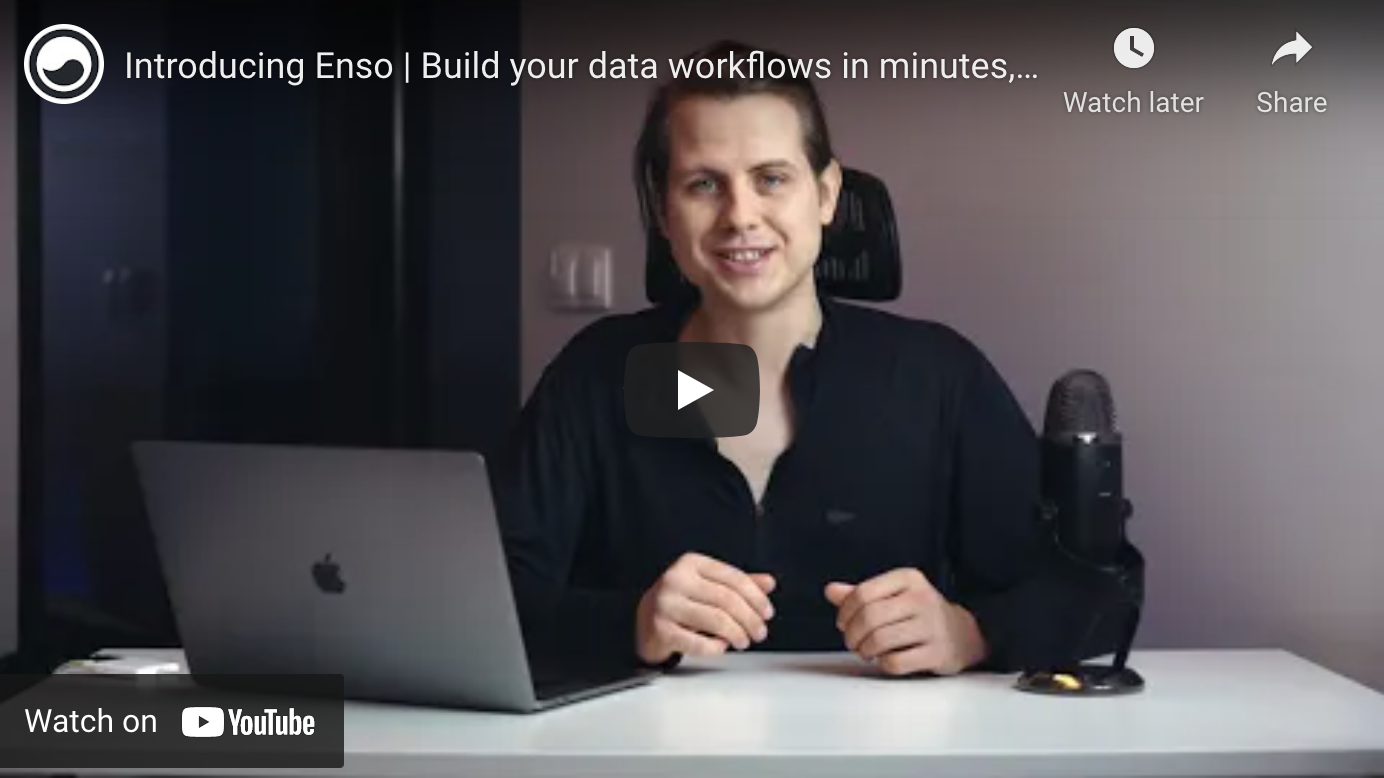
Enso's Features
Turning your data into knowledge is slow and error-prone. You canât trust tools that donât embrace best practices and provide quality assurance. Enso redefines the way you can work with your data: it is interactive, provides intelligent assistance, and was designed on a strong mathematical foundation, so you can always trust the results you get.
-
Intelligent suggestions of possible next steps. Build workflows in minutes instead of weeks.
Enso analyses the data, suggests possible next steps, and displays related help and examples. It lets you build dashboards, RPA workflows, and apps, with no coding required. Enso ships with a robust set of libraries, allowing you to work with local files, databases, HTTP services, and other applications in a seamless fashion.
Learn more â
-
A powerful, purely functional language. Both visual and textual.
Enso incorporates many recent innovations in data processing and programming language design to allow you to work interactively and trust the results that you get. It is a purely functional programming language with higher-order functions, user-defined algebraic datatypes, pattern-matching, and two equivalent representations that you can switch between on-demand.
Learn more â
-
Mix languages with close-to-zero interop overhead.
Import any library from Enso, Java, JavaScript, R, or Python, and use functions, callbacks, and data types without any wrappers. Enso uses GraalVM to compile them to the same instruction set with a unified memory model.
Learn more â
-
A cutting-edge visualization engine.
Enso is equipped with a highly-tailored WebGL visualization engine capable of displaying many millions of data points at 60 frames per second in a web browser. Currently, Enso includes a set of core data visualizations out of the box, and you can easily extend it with libraries such as D3.js, Three.js, Babylon.js, deck.gl, VTK.js, Potree, and many more.
Learn more â
Getting Started
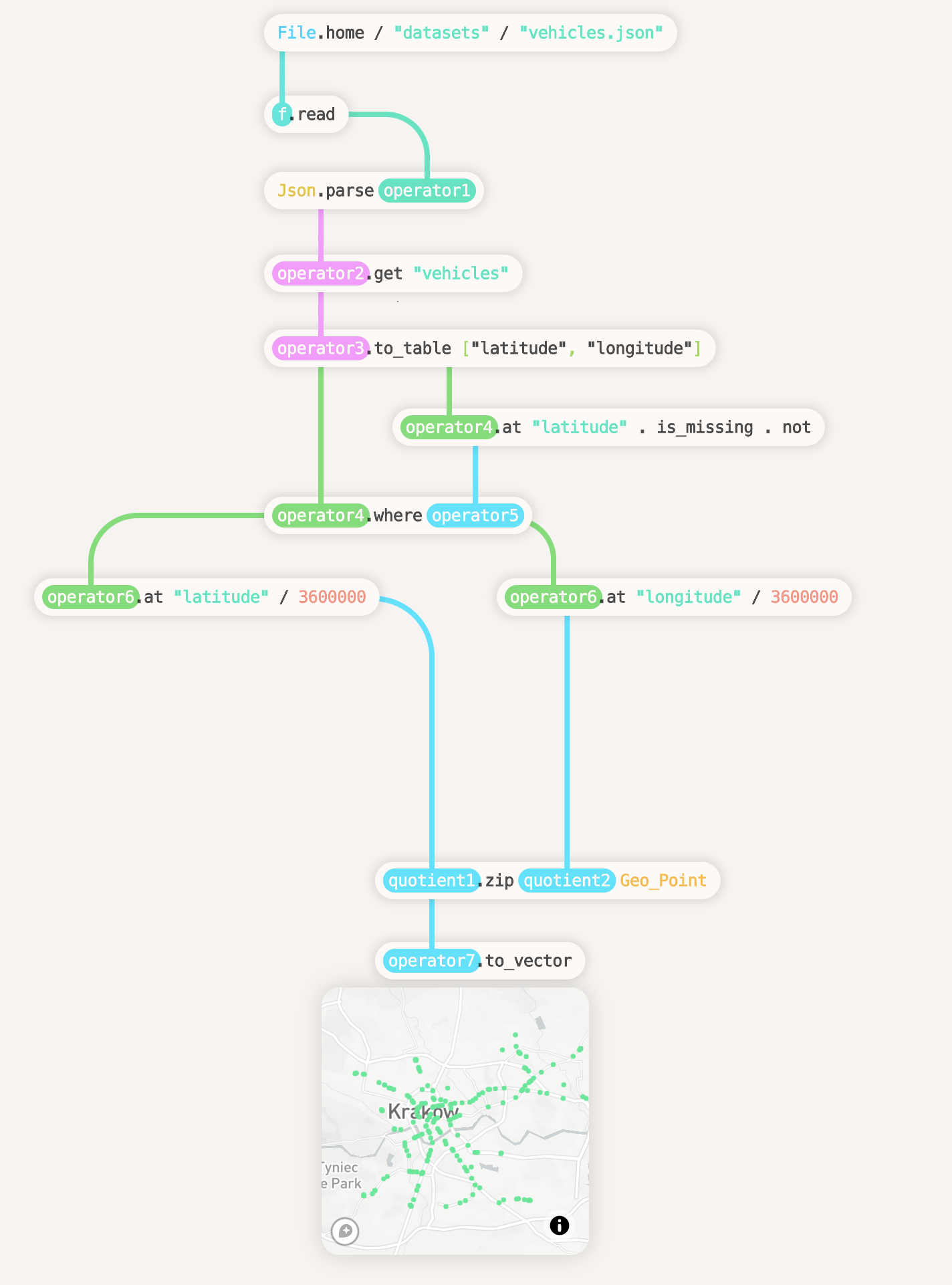
-
Keep Up With the Latest Updates
Enso Source Code
If you want to start using Enso, please see the download links in the getting started section above. Alternatively, you can get the IDE here. This section is intended for people interested in contributing to the development of Enso.
Enso is a community-driven open source project which is, and will always be, open and free to use. Join us, help us to build it, and spread the word!
Project Components
Enso consists of several sub projects:
-
Enso Engine: The Enso Engine is the set of tools that implement the Enso language and its associated services. These include the Enso interpreter, a just-in-time compiler and runtime (both powered by GraalVM), and a language server that lets you inspect Enso code as it runs. These components can be used on their own as command line tools.
-
Enso IDE: The Enso IDE is a desktop application that allows working with the visual form of Enso. It consists of an Electron application, a high performance WebGL UI framework, and the searcher which provides contextual search, hints, and documentation for all of Enso's functionality.
License
The Enso Engine is licensed under the Apache 2.0, as specified in the LICENSE file. The Enso IDE is licensed under the AGPL 3.0, as specified in the LICENSE file.
This license set was chosen to provide you with complete freedom to use Enso, create libraries, and release them under any license of your choice, while also allowing us to release commercial products on top of the platform, including Enso Cloud and Enso Enterprise server managers.
Contributing to Enso
Enso is a community-driven open source project which is and will always be open and free to use. We are committed to a fully transparent development process and highly appreciate every contribution. If you love the vision behind Enso and you want to redefine the data processing world, join us and help us track down bugs, implement new features, improve the documentation or spread the word!
If you'd like to help us make this vision a reality, please feel free to join our chat, and take a look at our development and contribution guidelines. The latter describes all the ways in which you can help out with the project, as well as provides detailed instructions for building and hacking on Enso.
If you believe that you have found a security vulnerability in Enso, or that you have a bug report that poses a security risk to Enso's users, please take a look at our security guidelines for a course of action.
Enso's Design
If you would like to gain a better understanding of the principles on which Enso
is based, or just delve into the why's and what's of Enso's design, please take
a look in the docs/
folder. It is split up into subfolders for each
component of Enso. You can view this same documentation in a rendered form at
the developer docs website.
This folder also contains a document on Enso's design philosophy, that details the thought process that we use when contemplating changes or additions to the language.
This documentation will evolve as Enso does, both to help newcomers to the project understand the reasoning behind the code, and also to act as a record of the decisions that have been made through Enso's evolution.
Top Related Projects
A browser based code editor
IntelliJ IDEA Community Edition & IntelliJ Platform
Eclipse Theia is a cloud & desktop IDE framework implemented in TypeScript.
A React-based UI toolkit for the web
The library for web and native user interfaces.
Visual Studio Code
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot