expo
An open-source framework for making universal native apps with React. Expo runs on Android, iOS, and the web.
Top Related Projects
A framework for building native applications using React
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Flutter makes it easy and fast to build beautiful apps for mobile and beyond
⚡ Empowering JavaScript with native platform APIs. ✨ Best of all worlds (TypeScript, Swift, Objective C, Kotlin, Java, Dart). Use what you love ❤️ Angular, React, Solid, Svelte, Vue with: iOS (UIKit, SwiftUI), Android (View, Jetpack Compose), Dart (Flutter) and you name it compatible.
Remote Administration Tool for Windows
Quick Overview
Expo is an open-source platform for making universal native apps for Android, iOS, and the web with JavaScript and React. It provides a set of tools and services built around React Native and native platforms that help you develop, build, deploy, and quickly iterate on iOS, Android, and web apps from the same JavaScript/TypeScript codebase.
Pros
- Rapid development with hot reloading and instant updates
- Cross-platform compatibility (iOS, Android, and web)
- Large ecosystem of pre-built components and APIs
- Simplified build process and over-the-air updates
Cons
- Limited access to native modules compared to pure React Native
- Larger app size due to included Expo runtime
- Potential performance overhead for complex applications
- Some advanced features require ejecting from the managed workflow
Code Examples
- Creating a new Expo project:
import { StatusBar } from 'expo-status-bar';
import { StyleSheet, Text, View } from 'react-native';
export default function App() {
return (
<View style={styles.container}>
<Text>Open up App.js to start working on your app!</Text>
<StatusBar style="auto" />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
});
- Using Expo's camera component:
import React, { useState, useEffect } from 'react';
import { Text, View, TouchableOpacity } from 'react-native';
import { Camera } from 'expo-camera';
export default function App() {
const [hasPermission, setHasPermission] = useState(null);
const [type, setType] = useState(Camera.Constants.Type.back);
useEffect(() => {
(async () => {
const { status } = await Camera.requestPermissionsAsync();
setHasPermission(status === 'granted');
})();
}, []);
if (hasPermission === null) {
return <View />;
}
if (hasPermission === false) {
return <Text>No access to camera</Text>;
}
return (
<View style={{ flex: 1 }}>
<Camera style={{ flex: 1 }} type={type}>
<View
style={{
flex: 1,
backgroundColor: 'transparent',
flexDirection: 'row',
}}>
<TouchableOpacity
style={{
flex: 0.1,
alignSelf: 'flex-end',
alignItems: 'center',
}}
onPress={() => {
setType(
type === Camera.Constants.Type.back
? Camera.Constants.Type.front
: Camera.Constants.Type.back
);
}}>
<Text style={{ fontSize: 18, marginBottom: 10, color: 'white' }}> Flip </Text>
</TouchableOpacity>
</View>
</Camera>
</View>
);
}
- Using Expo's location service:
import React, { useState, useEffect } from 'react';
import { Platform, Text, View, StyleSheet } from 'react-native';
import * as Location from 'expo-location';
export default function App() {
const [location, setLocation] = useState(null);
const [errorMsg, setErrorMsg] = useState(null);
useEffect(() => {
(async () => {
let { status } = await Location.requestForegroundPermissionsAsync();
if (status !== 'granted') {
setErrorMsg('Permission to access location was denied');
return;
}
let location = await Location.getCurrentPositionAsync({});
setLocation(location);
})();
}, []);
let text = 'Waiting..';
if (errorMsg) {
text = errorMsg;
} else if (location) {
Competitor Comparisons
A framework for building native applications using React
Pros of React Native
- More flexible and customizable, allowing deeper access to native modules
- Better performance for complex applications due to direct native code integration
- Larger ecosystem and community support for third-party libraries and plugins
Cons of React Native
- Steeper learning curve, especially for developers new to mobile development
- Requires more setup and configuration compared to Expo's streamlined approach
- More frequent need for native code knowledge and maintenance
Code Comparison
React Native (basic component):
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const MyComponent = () => (
<View style={styles.container}>
<Text>Hello, React Native!</Text>
</View>
);
Expo (similar component):
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const MyComponent = () => (
<View style={styles.container}>
<Text>Hello, Expo!</Text>
</View>
);
The code structure is very similar, as Expo is built on top of React Native. The main differences lie in the project setup, available APIs, and development workflow rather than the component code itself.
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Pros of Ionic Framework
- Offers a wider range of UI components and native plugins
- Supports multiple frameworks (Angular, React, Vue)
- More mature ecosystem with extensive documentation and community support
Cons of Ionic Framework
- Steeper learning curve, especially for beginners
- Larger bundle sizes compared to Expo
- May require more configuration for optimal performance
Code Comparison
Expo:
import React from 'react';
import { View, Text } from 'react-native';
export default function App() {
return (
<View>
<Text>Hello, Expo!</Text>
</View>
);
}
Ionic Framework:
import { IonContent, IonHeader, IonPage, IonTitle, IonToolbar } from '@ionic/react';
const Home: React.FC = () => {
return (
<IonPage>
<IonHeader>
<IonToolbar>
<IonTitle>Hello, Ionic!</IonTitle>
</IonToolbar>
</IonHeader>
<IonContent className="ion-padding">
<p>Welcome to Ionic Framework</p>
</IonContent>
</IonPage>
);
};
The code comparison shows that Ionic Framework requires more boilerplate and specific components, while Expo uses standard React Native components. Ionic's approach provides more structure but may be more complex for simple applications.
Flutter makes it easy and fast to build beautiful apps for mobile and beyond
Pros of Flutter
- Native performance: Flutter compiles to native code, offering better performance for complex apps
- Rich set of pre-built widgets: Extensive library of customizable UI components
- Hot reload: Faster development cycle with instant updates to the running app
Cons of Flutter
- Larger app size: Flutter apps tend to be larger due to bundled runtime and widgets
- Less mature ecosystem: Fewer third-party libraries and plugins compared to React Native
- Steeper learning curve: Dart language and widget-based approach may require more time to master
Code Comparison
Flutter:
import 'package:flutter/material.dart';
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Flutter App')),
body: Center(child: Text('Hello, World!')),
),
);
}
}
Expo:
import React from 'react';
import { View, Text } from 'react-native';
export default function App() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Hello, World!</Text>
</View>
);
}
⚡ Empowering JavaScript with native platform APIs. ✨ Best of all worlds (TypeScript, Swift, Objective C, Kotlin, Java, Dart). Use what you love ❤️ Angular, React, Solid, Svelte, Vue with: iOS (UIKit, SwiftUI), Android (View, Jetpack Compose), Dart (Flutter) and you name it compatible.
Pros of NativeScript
- Direct access to native APIs without plugins
- Supports multiple JavaScript frameworks (Angular, Vue.js, Svelte)
- Allows use of existing native libraries and CocoaPods
Cons of NativeScript
- Steeper learning curve for developers
- Smaller community and ecosystem compared to Expo
- Requires more setup and configuration
Code Comparison
NativeScript (XML and JavaScript):
<Page>
<StackLayout>
<Label text="Hello, NativeScript!" />
<Button text="Tap me" tap="{{ onTap }}" />
</StackLayout>
</Page>
exports.onTap = function() {
console.log("Button tapped!");
};
Expo (React Native):
import React from 'react';
import { View, Text, Button } from 'react-native';
export default function App() {
return (
<View>
<Text>Hello, Expo!</Text>
<Button title="Tap me" onPress={() => console.log("Button tapped!")} />
</View>
);
}
Both NativeScript and Expo offer cross-platform mobile app development solutions, but they differ in their approach and target audience. NativeScript provides more flexibility and direct access to native APIs, while Expo offers a simpler development experience with a larger ecosystem. The choice between the two depends on the project requirements and developer preferences.
Remote Administration Tool for Windows
Pros of Quasar
- Offers a wider range of UI components and layouts out-of-the-box
- Supports multiple build targets including mobile, desktop, and web
- Provides a more flexible and customizable development experience
Cons of Quasar
- Steeper learning curve, especially for developers new to Vue.js
- Smaller community and ecosystem compared to Expo
- Less comprehensive documentation and tutorials
Code Comparison
Quasar (Vue.js):
<template>
<q-page>
<q-btn color="primary" label="Click me" @click="handleClick" />
</q-page>
</template>
Expo (React Native):
import React from 'react';
import { View, Button } from 'react-native';
export default function App() {
return (
<View>
<Button title="Click me" onPress={handleClick} />
</View>
);
}
Both frameworks aim to simplify cross-platform development, but they differ in their approach and ecosystem. Quasar leverages Vue.js and offers more built-in components, while Expo focuses on React Native with a simpler setup and stronger mobile focus. The choice between them often depends on the developer's familiarity with Vue.js or React, as well as the specific project requirements.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Expo
Try Expo in the Browser ⢠Read the Documentation ⢠Learn more on our blog ⢠Request a feature
Follow us on
Introduction
Expo is an open-source platform for making universal native apps that run on Android, iOS, and the web. It includes a universal runtime and libraries that let you build native apps by writing React and JavaScript.
This repository includes the Expo SDK, Modules API, Go app, CLI, Router, documentation, and various other supporting tools. Expo Application Services (EAS) is a platform of hosted services that are deeply integrated with Expo open source tools. EAS helps you build, ship, and iterate on your app as an individual or a team.
Read the Expo Community Guidelines before interacting in the repository. Thank you for helping keep the Expo community open and welcoming!
Table of contents
ð Documentation
Learn about building and deploying universal apps in our official docs!
ðº Project Layout
packages
All the source code for Expo modules, if you want to edit a library or just see how it works this is where you'll find it.apps
This is where you can find Expo projects which are linked to the development modules. You'll do most of your testing in here.apps/expo-go
This is where you can find the source code for Expo Go.apps/expo-go/ios/Exponent.xcworkspace
is the Xcode workspace. When developing iOS, always open this instead ofExponent.xcodeproj
because the workspace also loads the CocoaPods dependencies.docs
The source code for https://docs.expo.devtemplates
The template projects you get when you runnpx create-expo-app
react-native-lab
This is our fork ofreact-native
used to build Expo Go.guides
In-depth tutorials for advanced topics like contributing to the client.tools
contain build and configuration tools.template-files
contains templates for files that require private keys. They are populated using the keys intemplate-files/keys.json
.template-files/ios/dependencies.json
specifies the CocoaPods dependencies of the app.
ð Badges
Let everyone know your app can be run instantly in the Expo Go app!
[](https://expo.dev/client)
[](https://expo.dev/client)
ð Contributing
If you like Expo and want to help make it better then check out our contributing guide! Check out the CLI package to work on the Expo CLI.
â FAQ
If you have questions about Expo and want answers, then check out our Frequently Asked Questions!
If you still have questions you can ask them on our Discord and Forums or X @expo.
ð The Team
Curious about who makes Expo? Here are our team members!
License
The Expo source code is made available under the MIT license. Some of the dependencies are licensed differently, with the BSD license, for example.
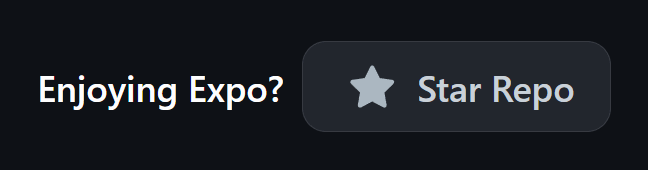
Top Related Projects
A framework for building native applications using React
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Flutter makes it easy and fast to build beautiful apps for mobile and beyond
⚡ Empowering JavaScript with native platform APIs. ✨ Best of all worlds (TypeScript, Swift, Objective C, Kotlin, Java, Dart). Use what you love ❤️ Angular, React, Solid, Svelte, Vue with: iOS (UIKit, SwiftUI), Android (View, Jetpack Compose), Dart (Flutter) and you name it compatible.
Remote Administration Tool for Windows
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot