Top Related Projects
Open source UI framework written in Python, running on Windows, Linux, macOS, Android and iOS
Desktop/Android/HTML5/iOS Java game development framework
Godot Engine – Multi-platform 2D and 3D game engine
Cocos2d-x is a suite of open-source, cross-platform, game-development tools utilized by millions of developers across the globe. Its core has evolved to serve as the foundation for Cocos Creator 1.x & 2.x.
🐍🎮 pygame (the library) is a Free and Open Source python programming language library for making multimedia applications like games built on top of the excellent SDL library. C, Python, Native, OpenGL.
A simple and easy-to-use library to enjoy videogames programming
Quick Overview
Flame is a modular 2D game engine built on top of Flutter. It provides a complete set of out-of-the-box solutions for game development, including rendering, audio, input handling, and more. Flame aims to simplify game development for Flutter developers while offering flexibility and extensibility.
Pros
- Easy integration with Flutter, allowing developers to leverage their existing knowledge
- Comprehensive set of game development tools and components
- Active community and regular updates
- Cross-platform support, enabling development for multiple platforms with a single codebase
Cons
- Limited 3D capabilities compared to some other game engines
- Learning curve for developers new to game development concepts
- Performance may not match native game engines for highly complex games
- Documentation can be inconsistent or outdated in some areas
Code Examples
- Creating a simple game:
import 'package:flame/game.dart';
class MyGame extends FlameGame {
@override
Future<void> onLoad() async {
// Load game assets and initialize components
}
@override
void update(double dt) {
// Update game logic
super.update(dt);
}
}
- Adding a sprite to the game:
import 'package:flame/components.dart';
class MySprite extends SpriteComponent with HasGameRef<MyGame> {
MySprite() : super(size: Vector2(100, 100));
@override
Future<void> onLoad() async {
sprite = await Sprite.load('my_sprite.png');
}
}
- Handling user input:
import 'package:flame/input.dart';
class MyGame extends FlameGame with TapDetector {
@override
void onTapDown(TapDownInfo info) {
// Handle tap event
print('Tapped at ${info.eventPosition.game}');
}
}
Getting Started
To start using Flame, add it to your pubspec.yaml
:
dependencies:
flame: ^1.8.1
Then, create a simple game:
import 'package:flame/game.dart';
import 'package:flutter/widgets.dart';
class MyGame extends FlameGame {
@override
Future<void> onLoad() async {
// Initialize your game here
}
}
void main() {
runApp(GameWidget(game: MyGame()));
}
This sets up a basic Flame game integrated with Flutter. You can now start adding components, handling input, and building your game logic.
Competitor Comparisons
Open source UI framework written in Python, running on Windows, Linux, macOS, Android and iOS
Pros of Kivy
- Cross-platform support for desktop and mobile
- Rich set of UI widgets and tools for complex interfaces
- Mature ecosystem with extensive documentation
Cons of Kivy
- Steeper learning curve, especially for Python beginners
- Larger app size due to bundled dependencies
- Performance can be slower compared to native solutions
Code Comparison
Kivy:
from kivy.app import App
from kivy.uix.button import Button
class MyApp(App):
def build(self):
return Button(text='Hello World')
MyApp().run()
Flame:
import 'package:flame/game.dart';
class MyGame extends Game {
@override
void render(Canvas canvas) {
// Rendering logic here
}
}
Kivy uses Python and focuses on UI development, while Flame uses Dart and is tailored for game development. Kivy's code is more concise for simple UI elements, but Flame offers a game-specific structure. Kivy provides a wider range of application types, whereas Flame excels in 2D game creation with its game loop and rendering system.
Desktop/Android/HTML5/iOS Java game development framework
Pros of libGDX
- Cross-platform development for desktop, mobile, and web
- Extensive ecosystem with many third-party extensions and tools
- Mature and battle-tested framework with a large community
Cons of libGDX
- Steeper learning curve, especially for beginners
- Java-based, which may not be preferred by some developers
- Less frequent updates compared to more modern frameworks
Code Comparison
Flame (Dart):
class MyGame extends FlameGame {
@override
Future<void> onLoad() async {
add(MySpriteComponent());
}
}
libGDX (Java):
public class MyGame extends ApplicationAdapter {
@Override
public void create() {
// Initialize game objects
}
}
Summary
Flame is a Flutter-based game engine, offering seamless integration with the Flutter ecosystem and easier development for mobile platforms. It's more beginner-friendly and has a growing community. libGDX, on the other hand, is a mature cross-platform framework with a wider range of supported platforms and a larger ecosystem. It offers more low-level control but has a steeper learning curve. The choice between the two depends on the developer's preferred language (Dart vs Java), target platforms, and specific project requirements.
Godot Engine – Multi-platform 2D and 3D game engine
Pros of Godot
- Full-featured game engine with built-in editor, supporting 2D and 3D game development
- Larger community and ecosystem, with more resources and plugins available
- Cross-platform support for multiple desktop, mobile, and web platforms
Cons of Godot
- Steeper learning curve, especially for beginners or those new to game development
- Larger project size and potentially slower compile times compared to Flame
- Custom scripting language (GDScript) may require additional learning
Code Comparison
Flame (Dart):
class MyGame extends FlameGame {
@override
Future<void> onLoad() async {
add(MySpriteComponent());
}
}
Godot (GDScript):
extends Node2D
func _ready():
var sprite = Sprite.new()
add_child(sprite)
Both Flame and Godot offer game development capabilities, but Godot provides a more comprehensive solution with its own integrated development environment. Flame, being a Flutter package, is lighter and more focused on 2D mobile game development within the Flutter ecosystem. Godot offers more flexibility and features but may require more time to master, while Flame leverages Flutter's simplicity and Dart language familiarity for quicker development of simpler games.
Cocos2d-x is a suite of open-source, cross-platform, game-development tools utilized by millions of developers across the globe. Its core has evolved to serve as the foundation for Cocos Creator 1.x & 2.x.
Pros of cocos2d-x
- More mature and feature-rich game development framework
- Supports multiple platforms, including mobile, desktop, and web
- Larger community and ecosystem with extensive documentation
Cons of cocos2d-x
- Steeper learning curve, especially for beginners
- Heavier and more complex codebase
- Less frequent updates and slower development cycle
Code Comparison
Flame (Dart):
class MyGame extends FlameGame {
@override
Future<void> onLoad() async {
add(MySpriteComponent());
}
}
cocos2d-x (C++):
class MyScene : public cocos2d::Scene {
public:
static cocos2d::Scene* createScene();
virtual bool init();
CREATE_FUNC(MyScene);
};
Summary
Flame is a lightweight, Flutter-based game engine that's easy to learn and integrate with existing Flutter projects. It's ideal for 2D mobile games and offers a simpler API.
cocos2d-x is a more comprehensive, cross-platform game development framework with a wider range of features and capabilities. It's better suited for larger, more complex games across multiple platforms but requires more time to master.
Choose Flame for rapid development of simple 2D games in Flutter, or cocos2d-x for more advanced, multi-platform game projects with extensive features and customization options.
🐍🎮 pygame (the library) is a Free and Open Source python programming language library for making multimedia applications like games built on top of the excellent SDL library. C, Python, Native, OpenGL.
Pros of Pygame
- More mature and established library with a larger community and extensive documentation
- Simpler to learn and use for beginners, especially those familiar with Python
- Wider range of tutorials, examples, and third-party resources available
Cons of Pygame
- Limited to 2D game development, lacking built-in 3D capabilities
- Performance may be slower compared to Flame, especially for complex games
- Less suitable for mobile game development
Code Comparison
Pygame:
import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
Flame:
import 'package:flame/game.dart';
class MyGame extends Game {
@override
void update(double dt) {}
@override
void render(Canvas canvas) {}
}
void main() => runApp(GameWidget(game: MyGame()));
Both Pygame and Flame offer game development capabilities, but they cater to different audiences and use cases. Pygame is more suitable for beginners and 2D game development in Python, while Flame is better for mobile game development and offers better performance for complex games using Dart and Flutter.
A simple and easy-to-use library to enjoy videogames programming
Pros of raylib
- Multi-platform support (Windows, Linux, macOS, Android, etc.)
- Low-level access to hardware, suitable for performance-critical applications
- Extensive documentation and examples
Cons of raylib
- Steeper learning curve, especially for beginners
- Less abstraction, requiring more manual management of game objects
- C-based API, which may be less familiar to some developers
Code Comparison
raylib:
#include "raylib.h"
int main(void)
{
InitWindow(800, 450, "raylib [core] example - basic window");
while (!WindowShouldClose())
{
BeginDrawing();
ClearBackground(RAYWHITE);
DrawText("Congrats! You created your first window!", 190, 200, 20, LIGHTGRAY);
EndDrawing();
}
CloseWindow();
return 0;
}
Flame:
import 'package:flame/game.dart';
import 'package:flutter/material.dart';
class MyGame extends FlameGame {
@override
void render(Canvas canvas) {
super.render(canvas);
canvas.drawRect(Rect.fromLTWH(0, 0, 100, 100), Paint()..color = Colors.red);
}
}
void main() {
runApp(GameWidget(game: MyGame()));
}
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
A Flutter-based game engine.
Documentation
The full documentation for Flame can be found on docs.flame-engine.org.
To change the version of the documentation, use the version selector noted with version:
in the
top of the page.
Note: The documentation that resides in the main branch is newer than the released documentation on the docs website.
Other useful links:
- The official Flame site.
- Examples of most features which can be tried out from your
browser.
- To access the code for each example, press the
< >
button in the top right corner.
- To access the code for each example, press the
- Tutorials - Some simple tutorials to get started.
- API Reference - The generated dartdoc API reference.
- awesome-flame - A curated list of Tutorials, Games, Libraries and Articles.
Help
There is a Flame community on Blue Fire's Discord server where you can ask any of your Flame related questions.
If you are more comfortable with StackOverflow, you can also create a question there. Add the Flame tag, to make sure that anyone following the tag can help out.
Features
The goal of the Flame Engine is to provide a complete set of out-of-the-way solutions for common problems that games developed with Flutter might share.
Some of the key features provided are:
- A game loop.
- A component/object system (FCS).
- Effects and particles.
- Collision detection.
- Gesture and input handling.
- Images, animations, sprites, and sprite sheets.
- General utilities to make development easier.
On top of those features, you can augment Flame with bridge packages. Through these libraries, you will be able to access bindings to other packages, including custom Flame components and helpers, in order to make integrations seamless.
Flame officially provides bridge libraries to the following packages:
- flame_audio for AudioPlayers: Play multiple audio files simultaneously.
- flame_bloc for Bloc: A predictable state management library.
- flame_fire_atlas for FireAtlas: Create texture atlases for games.
- flame_forge2d for Forge2D: A Box2D physics engine.
- flame_isolate - Makes it easy to use Flutter Isolates in a Flame game.
- flame_lint - Our set of linting (
analysis_options.yaml
) rules. - flame_lottie - Support for Lottie animation in Flame.
- flame_network_assets - Helpers to load game assets from network.
- flame_oxygen for Oxygen: A lightweight Entity Component System (ECS) framework.
- flame_rive for Rive: Create interactive animations.
- flame_svg for flutter_svg: Draw SVG files in Flutter.
- flame_tiled for Tiled: 2D tile map level editor.
Sponsors
The Flame Engine's top sponsors:
Do you or your company want to sponsor Flame? Check out our OpenCollective page, which is also mentioned in the section below, or contact us on Discord.
Support
The simplest way to show us your support is by giving the project a star! :star:
You can also support us monetarily by donating through OpenCollective:

Through GitHub Sponsors:
Or by becoming a patron on Patreon:
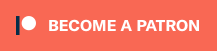
You can also show on your repository that your game is made with Flame by using one of the following badges:
[](https://flame-engine.org)
[](https://flame-engine.org)
[](https://flame-engine.org)
Contributing
Have you found a bug or have a suggestion of how to enhance Flame? Open an issue and we will take a look at it as soon as possible.
Do you want to contribute with a PR? PRs are always welcome, just make sure to create it from the correct branch (main) and follow the checklist which will appear when you open the PR.
Also, before you start, make sure to read our Contributing Guide.
For bigger changes, or if in doubt, make sure to talk about your contribution to the team. Either via an issue, GitHub discussion, or reach out to the team either using the Discord server.
Credits
- The Blue Fire team, who are continuously working on maintaining and improving Flame and its ecosystem.
- All the friendly contributors and people who are helping out in the community.
Top Related Projects
Open source UI framework written in Python, running on Windows, Linux, macOS, Android and iOS
Desktop/Android/HTML5/iOS Java game development framework
Godot Engine – Multi-platform 2D and 3D game engine
Cocos2d-x is a suite of open-source, cross-platform, game-development tools utilized by millions of developers across the globe. Its core has evolved to serve as the foundation for Cocos Creator 1.x & 2.x.
🐍🎮 pygame (the library) is a Free and Open Source python programming language library for making multimedia applications like games built on top of the excellent SDL library. C, Python, Native, OpenGL.
A simple and easy-to-use library to enjoy videogames programming
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot