auto-animate
A zero-config, drop-in animation utility that adds smooth transitions to your web app. You can use it with React, Vue, or any other JavaScript application.
Top Related Projects
A modern animation library for React and JavaScript
Animate on scroll library
🍿 A cross-browser library of CSS animations. As easy to use as an easy thing.
JavaScript animation engine
GSAP (GreenSock Animation Platform), a JavaScript animation library for the modern web
Quick Overview
Auto Animate is a zero-config, drop-in animation utility that adds smooth transitions to your web app. It automatically animates elements when they're added, removed, or moved within the DOM, providing a polished user experience with minimal effort from developers.
Pros
- Easy to implement with zero configuration required
- Works with any JavaScript framework or vanilla JS
- Lightweight (less than 1.5kb gzipped)
- Customizable with options for animation duration and easing
Cons
- May not be suitable for complex, custom animations
- Limited control over individual element animations
- Potential performance impact on large DOM trees or frequent updates
- Might conflict with other animation libraries if not properly managed
Code Examples
- Basic usage with vanilla JavaScript:
import autoAnimate from '@formkit/auto-animate'
const parent = document.getElementById('parent')
autoAnimate(parent)
// Now any changes to the parent's children will be animated
- Using with React:
import { useAutoAnimate } from '@formkit/auto-animate/react'
function MyComponent() {
const [parent] = useAutoAnimate()
return (
<div ref={parent}>
{/* Child elements here will be animated */}
</div>
)
}
- Customizing animation options:
import autoAnimate from '@formkit/auto-animate'
const parent = document.getElementById('parent')
autoAnimate(parent, {
duration: 250,
easing: 'ease-in-out',
disrespectUserMotionPreference: false
})
Getting Started
To use Auto Animate in your project, follow these steps:
-
Install the package:
npm install @formkit/auto-animate
-
Import and use in your code:
import autoAnimate from '@formkit/auto-animate' // For vanilla JS const parent = document.getElementById('parent') autoAnimate(parent) // For React import { useAutoAnimate } from '@formkit/auto-animate/react' function Component() { const [parent] = useAutoAnimate() return <div ref={parent}>{/* children */}</div> }
-
That's it! Your elements will now animate automatically when added, removed, or moved within the parent container.
Competitor Comparisons
A modern animation library for React and JavaScript
Pros of Motion
- More comprehensive animation library with a wider range of features
- Better support for complex animations and gestures
- Stronger TypeScript integration and type safety
Cons of Motion
- Steeper learning curve due to more complex API
- Larger bundle size, which may impact performance for smaller projects
- Requires more setup and configuration compared to Auto Animate
Code Comparison
Motion:
import { motion } from "framer-motion"
const MyComponent = () => (
<motion.div
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
transition={{ duration: 0.5 }}
>
Hello World
</motion.div>
)
Auto Animate:
import { useAutoAnimate } from '@formkit/auto-animate/react'
const MyComponent = () => {
const [parent] = useAutoAnimate()
return <div ref={parent}>Hello World</div>
}
Motion offers more control over animations but requires more code, while Auto Animate provides simpler implementation with less customization. Motion is better suited for complex animations, while Auto Animate excels in quick, easy-to-implement animations with minimal configuration.
Animate on scroll library
Pros of AOS
- Offers a wide variety of pre-defined animations
- Supports custom easing functions for more control over animation timing
- Provides options for animating elements on scroll
Cons of AOS
- Requires manual setup for each element to be animated
- Limited to scroll-based animations, less versatile for other use cases
- May have a steeper learning curve for complex animations
Code Comparison
AOS:
<div data-aos="fade-up" data-aos-duration="1000">
<p>This element will fade up on scroll</p>
</div>
Auto Animate:
import { autoAnimate } from '@formkit/auto-animate'
const parent = document.getElementById('parent')
autoAnimate(parent)
Key Differences
- AOS focuses on scroll-based animations, while Auto Animate is more general-purpose
- Auto Animate requires less markup and configuration for basic animations
- AOS offers more built-in animation types, while Auto Animate is simpler but more flexible
Use Cases
- Choose AOS for scroll-based animations and when you need a variety of pre-defined effects
- Opt for Auto Animate for simpler, automatic animations in dynamic content or list transitions
Both libraries have their strengths, and the choice depends on your specific project requirements and animation needs.
🍿 A cross-browser library of CSS animations. As easy to use as an easy thing.
Pros of animate.css
- Extensive library of pre-defined animations
- Easy to implement with simple CSS classes
- Wide browser compatibility
Cons of animate.css
- Requires manual triggering of animations
- Limited control over animation timing and sequencing
- Larger file size due to comprehensive animation set
Code Comparison
animate.css:
<h1 class="animate__animated animate__bounce">An animated element</h1>
auto-animate:
import { autoAnimate } from '@formkit/auto-animate'
useEffect(() => {
autoAnimate(parent)
}, [parent])
Key Differences
- animate.css is a CSS-only solution, while auto-animate is a JavaScript library
- auto-animate focuses on automatic animations for DOM changes, while animate.css provides pre-defined animation styles
- animate.css requires manual class application, whereas auto-animate detects and animates changes automatically
Use Cases
animate.css is ideal for:
- Adding simple, pre-defined animations to static elements
- Projects requiring a wide variety of animation styles
auto-animate is better suited for:
- Dynamic content with frequent DOM updates
- Seamless transitions in single-page applications
- Developers seeking a low-effort animation solution
Both libraries have their strengths, and the choice depends on the specific project requirements and developer preferences.
JavaScript animation engine
Pros of Anime
- More powerful and flexible animation engine with a wide range of animation types
- Supports SVG animations and morphing
- Extensive documentation and examples
Cons of Anime
- Steeper learning curve due to its extensive feature set
- Larger file size, which may impact page load times
- Requires more manual setup for animations
Code Comparison
Auto-animate:
import { autoAnimate } from '@formkit/auto-animate'
onMounted(() => {
autoAnimate(elementRef.value)
})
Anime:
import anime from 'animejs/lib/anime.es.js'
anime({
targets: '.element',
translateX: 250,
rotate: '1turn',
duration: 800
})
Summary
Auto-animate is designed for simplicity and ease of use, automatically adding animations to DOM changes. It's lightweight and requires minimal setup, making it ideal for quick implementations.
Anime, on the other hand, offers a more comprehensive animation toolkit with greater control and flexibility. It's better suited for complex, custom animations but requires more manual configuration.
Choose Auto-animate for rapid development and simple animations, or Anime for intricate, highly customized animation sequences.
GSAP (GreenSock Animation Platform), a JavaScript animation library for the modern web
Pros of GSAP
- More powerful and feature-rich animation library
- Extensive ecosystem with plugins for advanced effects
- Better performance for complex animations
Cons of GSAP
- Steeper learning curve
- Larger file size
- Requires more manual setup for basic animations
Code Comparison
GSAP:
import { gsap } from "gsap";
gsap.to(".box", { duration: 1, x: 100, y: 50, rotation: 360 });
Auto Animate:
import { autoAnimate } from '@formkit/auto-animate'
autoAnimate(parentElement)
Key Differences
- GSAP offers more control and customization options
- Auto Animate provides simpler, zero-config animations
- GSAP is better suited for complex, timeline-based animations
- Auto Animate excels at quick, easy-to-implement transitions
Use Cases
- Choose GSAP for advanced, highly customized animations
- Opt for Auto Animate for simple, declarative transitions in UI elements
Community and Support
- GSAP has a larger, more established community
- Auto Animate is newer but gaining popularity for its simplicity
Performance
- GSAP is optimized for complex animations
- Auto Animate is efficient for basic transitions but may struggle with more complex scenarios
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Add motion to your apps with a single line of code.
AutoAnimate is a zero-config, drop-in animation utility that adds smooth transitions to your web app. You can use it with Vue, React, Solid or any other JavaScript application.
With one line of code, you can improve your interfaces, for example:
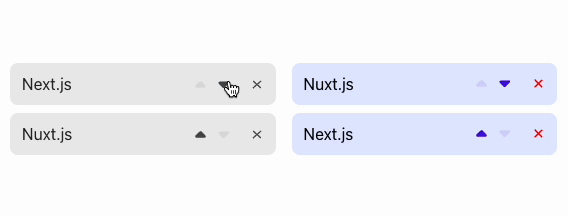
Installation
Install using your package manager of choice.
# yarn
yarn add @formkit/auto-animate
# npm
npm install @formkit/auto-animate
# pnpm
pnpm add @formkit/auto-animate
Boom! Done. That was fast! ð
Usage
ð View the documentation site for usage instructions.
Examples
ð View the documentation site for examples.
Plugins
ð View the documentation site for plugin instructions.
Support us
Is AutoAnimate saving you time?
Please consider supporting us with a recurring or one-time donation! ð
Contributing
Thank you for your willingness to contribute to this free and open source project! When contributing, consider first discussing your desired change with the core team via GitHub issues, Discord, or other method.
Top Related Projects
A modern animation library for React and JavaScript
Animate on scroll library
🍿 A cross-browser library of CSS animations. As easy to use as an easy thing.
JavaScript animation engine
GSAP (GreenSock Animation Platform), a JavaScript animation library for the modern web
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot