mimetype
A fast Golang library for media type and file extension detection, based on magic numbers
Top Related Projects
Fast, dependency-free Go package to infer binary file types based on the magic numbers header signature
Detect the file type of a file, stream, or data
A lightning fast image processing and resizing library for Go
DEPRECATED. Please use mholt/archives instead.
Tink is a multi-language, cross-platform, open source library that provides cryptographic APIs that are secure, easy to use correctly, and hard(er) to misuse.
Quick Overview
Gabriel-vasile/mimetype is a Go library for detecting MIME types and file extensions based on magic numbers. It provides fast and accurate file type identification by examining the content of files rather than relying solely on file extensions.
Pros
- Fast and efficient MIME type detection
- Supports a wide range of file formats
- Can be used with both files and in-memory data
- Actively maintained and regularly updated
Cons
- May not cover all possible file types
- Potential for false positives in some edge cases
- Requires reading file content, which can be slower for large files
- Limited to Go programming language
Code Examples
Detecting MIME type from a file:
file, _ := os.Open("file.pdf")
defer file.Close()
mtype, err := mimetype.DetectReader(file)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(mtype.String()) // Output: application/pdf
Detecting MIME type from a byte slice:
data := []byte{0x89, 0x50, 0x4E, 0x47, 0x0D, 0x0A, 0x1A, 0x0A}
mtype := mimetype.Detect(data)
fmt.Println(mtype.String()) // Output: image/png
Checking if a file matches a specific MIME type:
file, _ := os.Open("image.jpg")
defer file.Close()
mtype, _ := mimetype.DetectFile("image.jpg")
if mtype.Is("image/jpeg") {
fmt.Println("File is a JPEG image")
}
Getting Started
To use gabriel-vasile/mimetype in your Go project, follow these steps:
-
Install the library:
go get github.com/gabriel-vasile/mimetype
-
Import the library in your Go code:
import "github.com/gabriel-vasile/mimetype"
-
Use the library functions to detect MIME types:
mtype, err := mimetype.DetectFile("path/to/file") if err != nil { log.Fatal(err) } fmt.Printf("MIME type: %s, extension: %s\n", mtype.String(), mtype.Extension())
Competitor Comparisons
Fast, dependency-free Go package to infer binary file types based on the magic numbers header signature
Pros of filetype
- Supports a wider range of file types, including audio and video formats
- Provides more detailed file type information, including MIME type and extension
- Has a more extensive and active community, with regular updates and contributions
Cons of filetype
- Slightly larger package size, which may impact performance in some scenarios
- More complex API, potentially requiring a steeper learning curve for new users
- Slower detection speed for certain file types compared to mimetype
Code Comparison
mimetype:
mimetype.Detect(data)
mimetype.DetectFile("file.txt")
mimetype.DetectReader(reader)
filetype:
filetype.Match(data)
filetype.MatchFile("file.txt")
filetype.MatchReader(reader)
filetype.AddMatcher(matcher)
Both libraries offer similar core functionality for detecting file types, but filetype provides additional methods for custom matchers and more detailed type information. mimetype focuses on simplicity and performance, while filetype offers more extensive features and flexibility.
Overall, the choice between these libraries depends on the specific requirements of your project, such as the range of file types you need to detect, performance considerations, and the level of detail required in the file type information.
Detect the file type of a file, stream, or data
Pros of file-type
- More extensive file type support, including less common formats
- Faster detection for certain file types
- Actively maintained with frequent updates
Cons of file-type
- JavaScript/Node.js only, limiting its use in other environments
- Larger package size due to comprehensive file type support
Code Comparison
file-type:
import {fileTypeFromBuffer} from 'file-type';
const buffer = readChunk.sync('path/to/file', 0, 4100);
const type = await fileTypeFromBuffer(buffer);
console.log(type);
mimetype:
import "github.com/gabriel-vasile/mimetype"
mtype, err := mimetype.DetectFile("path/to/file")
if err != nil {
fmt.Println(err)
}
fmt.Println(mtype.String())
Key Differences
- Language: file-type is JavaScript-based, while mimetype is written in Go
- API: file-type uses async/await for detection, mimetype uses synchronous functions
- Flexibility: mimetype can be easily extended with custom MIME types
- Performance: mimetype may have an edge in Go-based applications
Use Cases
- file-type: Ideal for Node.js applications requiring extensive file type support
- mimetype: Better suited for Go projects or when cross-language compatibility is needed
Both libraries offer reliable MIME type detection, with the choice depending on the specific project requirements and programming language preferences.
A lightning fast image processing and resizing library for Go
Pros of govips
- Specialized for image processing and manipulation
- Offers a wide range of image operations (resize, crop, rotate, etc.)
- Utilizes libvips, providing high-performance image processing
Cons of govips
- Limited to image file formats, not a general-purpose MIME type detector
- Larger dependency footprint due to libvips requirement
- Steeper learning curve for basic MIME type detection tasks
Code Comparison
mimetype:
mime, err := mimetype.DetectFile("file.jpg")
if err != nil {
log.Fatal(err)
}
fmt.Println(mime.String()) // Output: image/jpeg
govips:
image, err := vips.NewImageFromFile("file.jpg")
if err != nil {
log.Fatal(err)
}
fmt.Println(image.Format()) // Output: JPEG
Summary
mimetype is a lightweight, general-purpose MIME type detection library, while govips is a comprehensive image processing library. mimetype excels in simplicity and broad file type support, whereas govips offers powerful image manipulation capabilities but is limited to image formats. Choose mimetype for basic file type detection across various formats, and govips for advanced image processing tasks.
DEPRECATED. Please use mholt/archives instead.
Pros of archiver
- Comprehensive archive handling: Supports creating, extracting, and manipulating various archive formats (zip, tar, rar, etc.)
- Command-line interface: Offers a CLI tool for easy use in scripts and terminal operations
- Cross-platform compatibility: Works on multiple operating systems
Cons of archiver
- Larger scope and complexity: Focuses on archive operations rather than just MIME type detection
- Potentially higher resource usage: May require more system resources due to its broader functionality
- Less specialized for MIME type detection: Not optimized specifically for quick and efficient MIME type identification
Code comparison
mimetype:
mimetype, err := mimetype.DetectFile("file.jpg")
if err != nil {
log.Fatal(err)
}
fmt.Println(mimetype.String()) // Output: image/jpeg
archiver:
err := archiver.Archive([]string{"file1.txt", "file2.jpg"}, "archive.zip")
if err != nil {
log.Fatal(err)
}
// No direct MIME type detection functionality
Summary
While mimetype specializes in efficient MIME type detection, archiver offers a broader range of archive-related operations. mimetype is more focused and lightweight for MIME type identification, whereas archiver provides comprehensive archive handling capabilities but may be overkill for simple MIME type detection tasks.
Tink is a multi-language, cross-platform, open source library that provides cryptographic APIs that are secure, easy to use correctly, and hard(er) to misuse.
Pros of Tink
- Comprehensive cryptographic library with support for various algorithms and protocols
- Designed with security best practices in mind, offering protection against common cryptographic pitfalls
- Actively maintained by Google, ensuring regular updates and security patches
Cons of Tink
- Steeper learning curve due to its extensive feature set
- Larger codebase and dependencies, potentially increasing project size
- May be overkill for simple file type detection tasks
Code Comparison
Tink (encryption example):
keysetHandle, err := keyset.NewHandle(aead.AES256GCMKeyTemplate())
a, err := aead.New(keysetHandle)
ciphertext, err := a.Encrypt(plaintext, associatedData)
Mimetype (file type detection):
mime, err := mimetype.DetectFile("file.pdf")
if err != nil {
fmt.Println(err)
}
fmt.Println(mime.String())
Summary
Tink is a robust cryptographic library offering comprehensive security features, while Mimetype focuses specifically on file type detection. Tink provides a wide range of cryptographic operations but may be more complex to implement. Mimetype offers a simpler, more focused solution for determining file types. Choose based on your project's specific needs and complexity requirements.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
mimetype
A package for detecting MIME types and extensions based on magic numbers
Goroutine safe, extensible, no C bindings
Features
- fast and precise MIME type and file extension detection
- long list of supported MIME types
- possibility to extend with other file formats
- common file formats are prioritized
- text vs. binary files differentiation
- safe for concurrent usage
Install
go get github.com/gabriel-vasile/mimetype
Usage
mtype := mimetype.Detect([]byte)
// OR
mtype, err := mimetype.DetectReader(io.Reader)
// OR
mtype, err := mimetype.DetectFile("/path/to/file")
fmt.Println(mtype.String(), mtype.Extension())
See the runnable Go Playground examples.
Usage'
Only use libraries like mimetype as a last resort. Content type detection
using magic numbers is slow, inaccurate, and non-standard. Most of the times
protocols have methods for specifying such metadata; e.g., Content-Type
header
in HTTP and SMTP.
FAQ
Q: My file is in the list of supported MIME types but it is not correctly detected. What should I do?
A: Some file formats (often Microsoft Office documents) keep their signatures towards the end of the file. Try increasing the number of bytes used for detection with:
mimetype.SetLimit(1024*1024) // Set limit to 1MB.
// or
mimetype.SetLimit(0) // No limit, whole file content used.
mimetype.DetectFile("file.doc")
If increasing the limit does not help, please open an issue.
Structure
mimetype uses a hierarchical structure to keep the MIME type detection logic. This reduces the number of calls needed for detecting the file type. The reason behind this choice is that there are file formats used as containers for other file formats. For example, Microsoft Office files are just zip archives, containing specific metadata files. Once a file has been identified as a zip, there is no need to check if it is a text file, but it is worth checking if it is an Microsoft Office file.
To prevent loading entire files into memory, when detecting from a reader or from a file mimetype limits itself to reading only the header of the input.
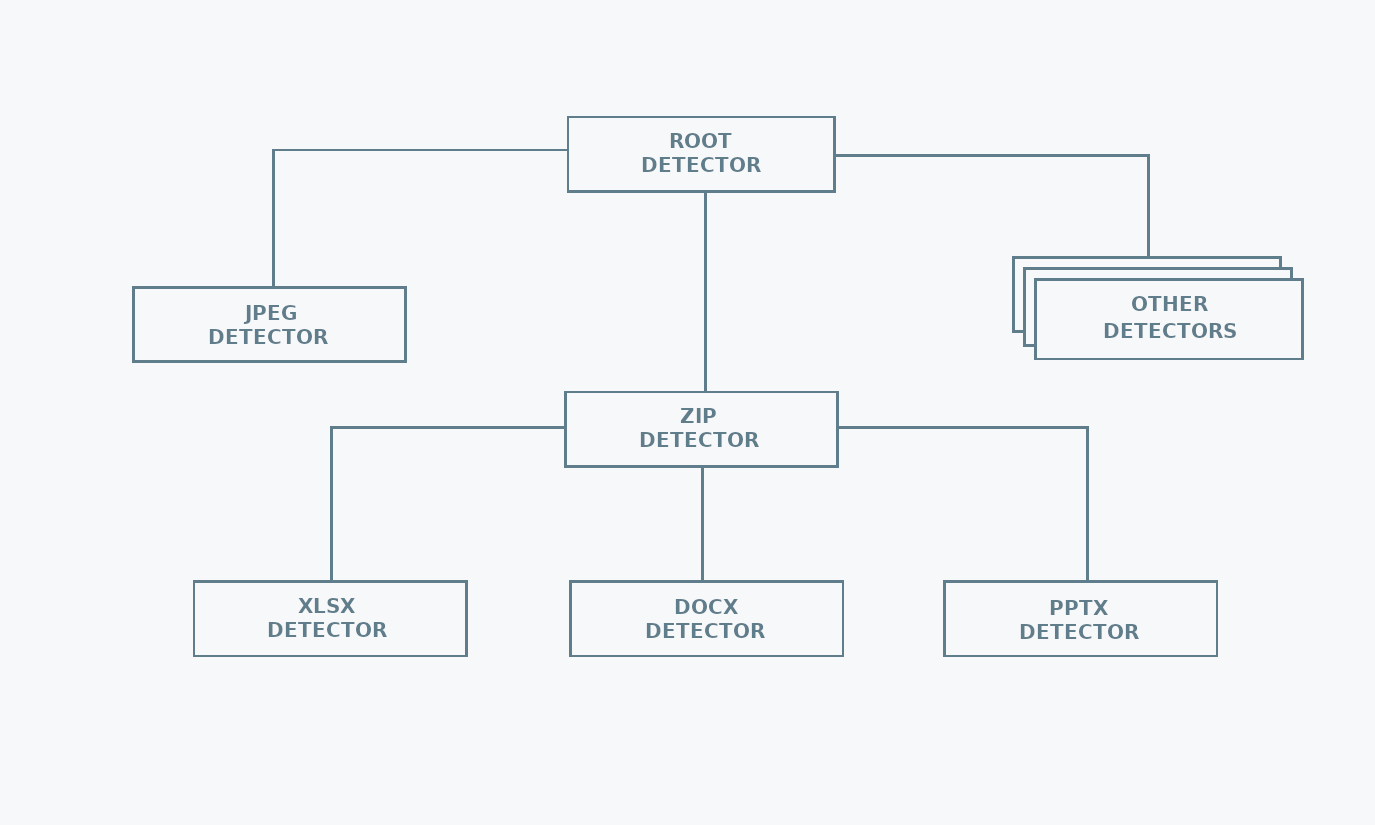
Performance
Thanks to the hierarchical structure, searching for common formats first,
and limiting itself to file headers, mimetype matches the performance of
stdlib http.DetectContentType
while outperforming the alternative package.
mimetype http.DetectContentType filetype
BenchmarkMatchTar-24 250 ns/op 400 ns/op 3778 ns/op
BenchmarkMatchZip-24 524 ns/op 351 ns/op 4884 ns/op
BenchmarkMatchJpeg-24 103 ns/op 228 ns/op 839 ns/op
BenchmarkMatchGif-24 139 ns/op 202 ns/op 751 ns/op
BenchmarkMatchPng-24 165 ns/op 221 ns/op 1176 ns/op
Contributing
See CONTRIBUTING.md.
Top Related Projects
Fast, dependency-free Go package to infer binary file types based on the magic numbers header signature
Detect the file type of a file, stream, or data
A lightning fast image processing and resizing library for Go
DEPRECATED. Please use mholt/archives instead.
Tink is a multi-language, cross-platform, open source library that provides cryptographic APIs that are secure, easy to use correctly, and hard(er) to misuse.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot