glide-data-grid
🚀 Glide Data Grid is a no compromise, outrageously react fast data grid with rich rendering, first class accessibility, and full TypeScript support.
Top Related Projects
JavaScript Data Grid / Data Table with a Spreadsheet Look & Feel. Works with React, Angular, and Vue. Supported by the Handsontable team ⚡
The best JavaScript Data Table for building Enterprise Applications. Supports React / Angular / Vue / Plain JavaScript.
🤖 Headless UI for building powerful tables & datagrids for TS/JS - React-Table, Vue-Table, Solid-Table, Svelte-Table
React components for efficiently rendering large lists and tabular data
Feature-rich and customizable data grid React component
A lightning fast JavaScript grid/spreadsheet
Quick Overview
Glide Data Grid is a powerful and customizable data grid component for React applications. It offers high-performance rendering, virtual scrolling, and a wide range of features for displaying and interacting with large datasets. The grid is designed to handle millions of rows efficiently while providing a smooth user experience.
Pros
- High performance with virtual scrolling, capable of handling millions of rows
- Extensive customization options for cell rendering and behavior
- Built-in support for various data types and editing capabilities
- Responsive design and touch-friendly for mobile devices
Cons
- Steep learning curve due to its extensive API and configuration options
- Limited built-in theming options, requiring custom CSS for advanced styling
- Dependency on React, not suitable for non-React projects
- Some advanced features may require additional setup or custom implementations
Code Examples
- Basic Grid Setup:
import { DataEditor } from "@glideapps/glide-data-grid";
function MyGrid() {
return (
<DataEditor
columns={columns}
rows={numRows}
getCellContent={getCellContent}
/>
);
}
- Custom Cell Renderer:
function getCellContent([col, row]) {
return {
kind: "custom",
backgroundColor: "#f0f0f0",
content: (props) => <MyCustomCell {...props} />,
};
}
- Handling Cell Edits:
function onCellEdited([col, row], newValue) {
// Update your data source here
console.log(`Cell (${col}, ${row}) edited. New value: ${newValue}`);
}
<DataEditor
// ... other props
onCellEdited={onCellEdited}
/>
Getting Started
-
Install the package:
npm install @glideapps/glide-data-grid
-
Import and use the DataEditor component:
import React from "react";
import { DataEditor } from "@glideapps/glide-data-grid";
import "@glideapps/glide-data-grid/dist/index.css";
const columns = [
{ title: "Name", width: 100 },
{ title: "Age", width: 80 },
];
const getCellContent = ([col, row]) => {
if (col === 0) return { kind: "text", data: `Person ${row}` };
return { kind: "number", data: 20 + row };
};
function MyGrid() {
return (
<DataEditor
columns={columns}
rows={1000}
getCellContent={getCellContent}
/>
);
}
export default MyGrid;
This example sets up a basic grid with two columns and 1000 rows, using simple cell content generation. Customize the columns
and getCellContent
function to match your data structure and requirements.
Competitor Comparisons
JavaScript Data Grid / Data Table with a Spreadsheet Look & Feel. Works with React, Angular, and Vue. Supported by the Handsontable team ⚡
Pros of Handsontable
- More mature and feature-rich, with a longer development history
- Offers both open-source and commercial versions with additional features
- Supports multiple frameworks (React, Angular, Vue) out of the box
Cons of Handsontable
- Larger bundle size, which may impact performance for smaller projects
- Steeper learning curve due to its extensive feature set
- Commercial license required for some advanced features
Code Comparison
Handsontable:
const hot = new Handsontable(container, {
data: data,
rowHeaders: true,
colHeaders: true,
filters: true,
dropdownMenu: true
});
Glide Data Grid:
<DataEditor
getCellContent={getCellContent}
columns={columns}
rows={rows}
onCellEdited={onCellEdited}
/>
Handsontable provides a more configuration-based approach, while Glide Data Grid focuses on a component-based structure with props for customization. Handsontable's setup includes built-in features like filters and dropdown menus, whereas Glide Data Grid requires custom implementation of such features through props and callbacks.
The best JavaScript Data Table for building Enterprise Applications. Supports React / Angular / Vue / Plain JavaScript.
Pros of ag-grid
- More comprehensive feature set, including advanced filtering, sorting, and grouping
- Extensive documentation and community support
- Multiple framework integrations (React, Angular, Vue)
Cons of ag-grid
- Steeper learning curve due to its complexity
- Larger bundle size, which may impact performance
- Commercial license required for some advanced features
Code Comparison
ag-grid:
import { AgGridReact } from 'ag-grid-react';
<AgGridReact
columnDefs={columnDefs}
rowData={rowData}
onGridReady={onGridReady}
pagination={true}
/>
glide-data-grid:
import { DataEditor } from "@glideapps/glide-data-grid";
<DataEditor
getCellContent={getCellContent}
columns={columns}
rows={rows}
onCellEdited={onCellEdited}
/>
Both libraries offer powerful data grid solutions, but ag-grid provides a more feature-rich experience at the cost of complexity and potential licensing fees. glide-data-grid, while less comprehensive, offers a simpler API and focuses on performance. The choice between the two depends on specific project requirements, budget constraints, and desired level of customization.
🤖 Headless UI for building powerful tables & datagrids for TS/JS - React-Table, Vue-Table, Solid-Table, Svelte-Table
Pros of Table
- Framework-agnostic with adapters for React, Vue, Solid, and Svelte
- Highly customizable with a modular plugin system
- Extensive documentation and community support
Cons of Table
- Steeper learning curve due to its flexibility and feature-rich nature
- May require more setup and configuration for basic use cases
- Potentially larger bundle size when using multiple features
Code Comparison
Table:
import { useReactTable, getCoreRowModel } from '@tanstack/react-table'
const table = useReactTable({
data,
columns,
getCoreRowModel: getCoreRowModel(),
})
Glide Data Grid:
import { DataEditor } from "@glideapps/glide-data-grid";
<DataEditor
getCellContent={getCellContent}
columns={columns}
rows={numRows}
/>
Key Differences
- Table focuses on flexibility and extensibility across frameworks, while Glide Data Grid is specifically designed for React
- Glide Data Grid emphasizes performance and handling large datasets efficiently
- Table provides more built-in features for sorting, filtering, and pagination, whereas Glide Data Grid requires custom implementations for these functionalities
Use Cases
- Choose Table for complex, feature-rich tables across different frameworks
- Opt for Glide Data Grid when dealing with large datasets and prioritizing performance in React applications
React components for efficiently rendering large lists and tabular data
Pros of react-virtualized
- More mature and widely adopted, with a larger community and ecosystem
- Offers a broader range of components beyond just data grids (e.g., lists, tables, masonry)
- Highly customizable and flexible for various use cases
Cons of react-virtualized
- Less focused on data grid functionality compared to glide-data-grid
- May require more setup and configuration for complex data grid scenarios
- Performance can be less optimized for large datasets in grid-specific use cases
Code Comparison
react-virtualized:
import { Grid } from 'react-virtualized';
<Grid
cellRenderer={({ columnIndex, key, rowIndex, style }) => (
<div key={key} style={style}>
{data[rowIndex][columnIndex]}
</div>
)}
columnCount={columnCount}
columnWidth={100}
height={300}
rowCount={rowCount}
rowHeight={30}
width={300}
/>
glide-data-grid:
import { DataEditor } from "@glideapps/glide-data-grid";
<DataEditor
getCellContent={([col, row]) => data[row][col]}
columns={columns}
rows={rowCount}
height={300}
width={300}
/>
Both libraries offer efficient rendering of large datasets, but glide-data-grid provides a more streamlined API specifically for data grid scenarios, while react-virtualized offers greater flexibility for various virtualized rendering use cases.
Feature-rich and customizable data grid React component
Pros of react-data-grid
- More mature and established project with a larger community
- Extensive documentation and examples available
- Built-in support for various features like sorting, filtering, and cell editing
Cons of react-data-grid
- Less performant with large datasets compared to glide-data-grid
- Limited customization options for cell rendering and styling
- Steeper learning curve for advanced features
Code Comparison
react-data-grid:
import ReactDataGrid from 'react-data-grid';
const columns = [
{ key: 'id', name: 'ID' },
{ key: 'name', name: 'Name' }
];
const rows = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Jane' }
];
<ReactDataGrid columns={columns} rows={rows} />
glide-data-grid:
import { DataEditor } from "@glideapps/glide-data-grid";
const columns = [
{ title: "ID", width: 100 },
{ title: "Name", width: 200 }
];
const getCellContent = ([col, row]) => ({
kind: "text",
data: row === 0 ? "John" : "Jane"
});
<DataEditor getCellContent={getCellContent} columns={columns} rows={2} />
Both libraries offer React-based data grid components, but glide-data-grid focuses on performance and customization, while react-data-grid provides more out-of-the-box features. The code examples show the basic setup for each library, highlighting the differences in API and implementation.
A lightning fast JavaScript grid/spreadsheet
Pros of SlickGrid
- Mature and battle-tested: SlickGrid has been around for a long time and has been used in many production environments
- Extensive documentation and community support
- Highly customizable with a wide range of plugins and extensions
Cons of SlickGrid
- Older technology stack: primarily built for jQuery, which may not align with modern web development practices
- Steeper learning curve due to its extensive feature set and configuration options
- Less frequent updates and maintenance compared to more recent projects
Code Comparison
SlickGrid:
var grid = new Slick.Grid("#myGrid", data, columns, options);
grid.onCellChange.subscribe(function (e, args) {
console.log("Cell changed: ", args);
});
Glide Data Grid:
const gridRef = useRef(null);
return <DataEditor {...props} ref={gridRef} />;
// Event handling is done through props
SlickGrid uses a more traditional JavaScript approach with event subscriptions, while Glide Data Grid leverages React's component-based architecture and prop-based event handling. Glide Data Grid offers a more modern, React-friendly API, making it easier to integrate into React applications. However, SlickGrid's maturity and extensive feature set may be advantageous for complex grid requirements in various environments.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
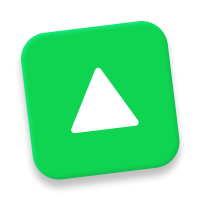
Glide Data Grid
A canvas-based data grid, supporting millions of rows, rapid updating, and native scrolling.
Built as the basis for the Glide Data Editor. We're hiring.
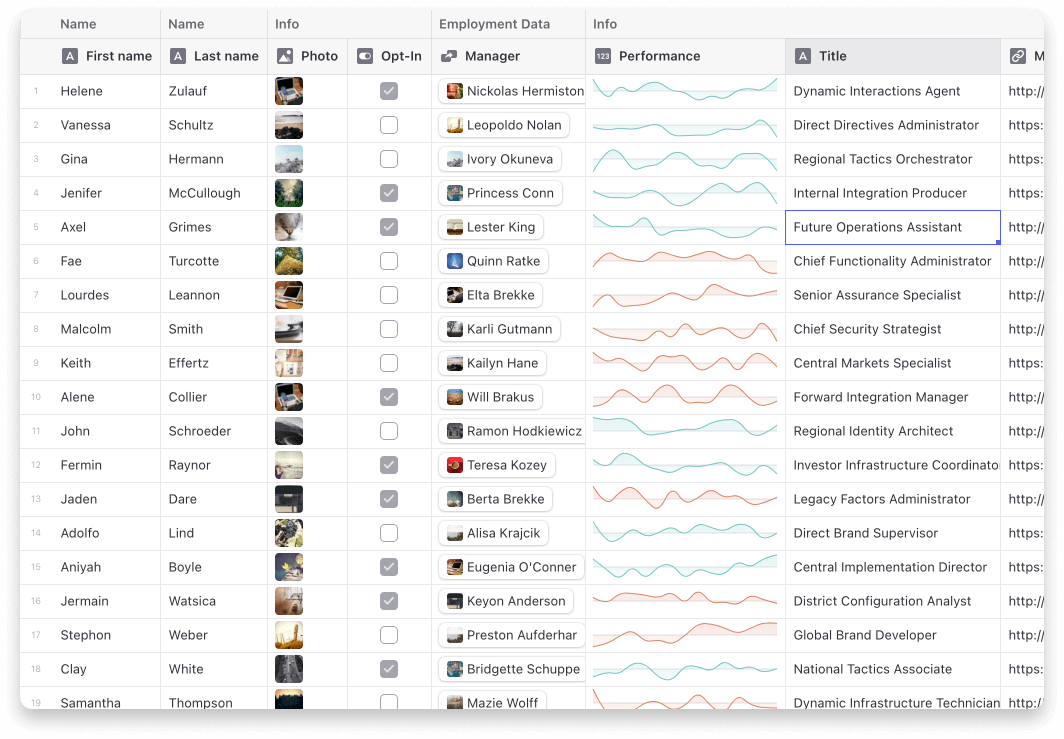
ð©âð» Demo and features
Lots of fun examples are in our Storybook.
You can also visit our main site.
Features
- It scales to millions of rows. Cells are rendered lazily on demand for memory efficiency.
- Scrolling is extremely fast. Native scrolling keeps everything buttery smooth.
- Supports multiple types of cells. Numbers, text, markdown, bubble, image, drilldown, uri
- Fully Free & Open Source. MIT licensed, so you can use Grid in commercial projects.
- Editing is built in.
- Resizable and movable columns.
- Variable sized rows.
- Merged cells.
- Single and multi-select rows, cells, and columns.
- Cell rendering can be fully customized.
â¡ Quick Start
First make sure you are using React 16 or greater. Then install the data grid:
npm i @glideapps/glide-data-grid
You may also need to install the peer dependencies if you don't have them already:
npm i lodash marked react-responsive-carousel
Create a new DataEditor
wherever you need to display lots and lots of data
<DataEditor getCellContent={getData} columns={columns} rows={numRows} />
Don't forget to import mandatory CSS
import "@glideapps/glide-data-grid/dist/index.css";
Making your columns is easy
// Grid columns may also provide icon, overlayIcon, menu, style, and theme overrides
const columns: GridColumn[] = [
{ title: "First Name", width: 100 },
{ title: "Last Name", width: 100 },
];
Last provide data to the grid
// If fetching data is slow you can use the DataEditor ref to send updates for cells
// once data is loaded.
function getData([col, row]: Item): GridCell {
const person = data[row];
if (col === 0) {
return {
kind: GridCellKind.Text,
data: person.firstName,
allowOverlay: false,
displayData: person.firstName,
};
} else if (col === 1) {
return {
kind: GridCellKind.Text,
data: person.lastName,
allowOverlay: false,
displayData: person.lastName,
};
} else {
throw new Error();
}
}
You can edit this example live on CodeSandbox
Full API documentation
The full API documentation is on the main site
ð FAQ
Nothing shows up!
Please read the Prerequisites section in the docs.
It crashes when I try to edit a cell!
Please read the Prerequisites section in the docs.
Does it work with screen readers and other a11y tools?
Yes. Unfortunately none of the primary developers are accessibility users so there are likely flaws in the implementation we are not aware of. Bug reports welcome!
Does it support my data source?
Yes.
Data Grid is agnostic about the way you load/store/generate/mutate your data. What it requires is that you tell it which columns you have, how many rows, and to give it a function it can call to get the data for a cell in a specific row and column.
Does it do sorting, searching, and filtering?
Search is included. You provide the trigger, we do the search. Example in our storybook.
Filtering and sorting are something you would have to implement with your data source. There are hooks for adding column header menus if you want that.
The reason we don't add filtering/sorting in by default is that these are usually very application-specific, and can often also be implemented more efficiently in the data source, via a database query, for example.
Can it do frozen columns?
Yes!
Can I render my own cells?
Yes, but the renderer has to use HTML Canvas. Simple example in our Storybook.
Why does Data Grid use HTML Canvas?
Originally we had implemented our Grid using virtualized rendering. We virtualized both in the horizontal and vertical direction using react-virtualized. The problem is simply scrolling performance. Once you need to load/unload hundreds of DOM elements per frame nothing can save you.
There are some hacks you can do like setting timers and entering into a "low fidelity" rendering mode where you only render a single element per cell. This works okay until you want to show hundreds of cells and you are right back to choppy scrolling. It also doesn't really look or feel great.
I want to use this with Next.js / Vercel, but I'm getting weird errors
The easiest way to use the grid with Next is to create a component which wraps up your grid and then import it as a dynamic.
home.tsx
import type { NextPage } from "next";
import dynamic from "next/dynamic";
import styles from "../styles/Home.module.css";
const Grid = dynamic(
() => {
return import("../components/Grid");
},
{ ssr: false }
);
export const Home: NextPage = () => {
return (
<div className={styles.container}>
<main className={styles.main}>
<h1 className={styles.title}>Hi</h1>
<Grid />
</main>
</div>
);
};
grid.tsx
import React from "react";
import DataEditor from "@glideapps/glide-data-grid";
export default function Grid() {
return <DataEditor {...args} />;
}
Top Related Projects
JavaScript Data Grid / Data Table with a Spreadsheet Look & Feel. Works with React, Angular, and Vue. Supported by the Handsontable team ⚡
The best JavaScript Data Table for building Enterprise Applications. Supports React / Angular / Vue / Plain JavaScript.
🤖 Headless UI for building powerful tables & datagrids for TS/JS - React-Table, Vue-Table, Solid-Table, Svelte-Table
React components for efficiently rendering large lists and tabular data
Feature-rich and customizable data grid React component
A lightning fast JavaScript grid/spreadsheet
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot