game-server
Distributed Java game server, including cluster management server, gateway server, hall server, game logic server, background monitoring server and a running web version of fishing. State machine, behavior tree, A* pathfinding, navigation mesh and other AI tools
Top Related Projects
A fast,scalable,distributed game server framework for Node.js.
Unified Realtime/API framework for .NET platform and Unity.
Distributed server for social and realtime games and apps.
Quick Overview
The jzyong/game-server repository is a comprehensive game server framework written in Java. It provides a foundation for building scalable and efficient multiplayer game servers, offering various modules and tools to handle common game server functionalities.
Pros
- Modular architecture allowing for easy customization and extension
- Includes support for both TCP and UDP networking protocols
- Provides built-in tools for performance monitoring and testing
- Offers integration with popular databases like MongoDB and Redis
Cons
- Limited documentation, which may make it challenging for newcomers to get started
- Primarily focused on Java, which might not be suitable for developers preferring other languages
- Some modules may require additional configuration or setup
Code Examples
- Creating a simple game server:
public class SimpleGameServer extends GameServer {
public static void main(String[] args) {
SimpleGameServer server = new SimpleGameServer();
server.start();
}
@Override
protected void initNetty() {
setTcpPort(8888);
super.initNetty();
}
}
- Handling a client connection:
public class ClientConnectionHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) {
System.out.println("New client connected: " + ctx.channel().remoteAddress());
}
}
- Sending a message to all connected clients:
public void broadcastMessage(String message) {
for (Channel channel : connectedClients) {
channel.writeAndFlush(message);
}
}
Getting Started
To get started with the jzyong/game-server framework:
-
Clone the repository:
git clone https://github.com/jzyong/game-server.git
-
Import the project into your preferred Java IDE.
-
Add the necessary dependencies to your project's build file (e.g., Maven or Gradle).
-
Create a new class extending
GameServer
and implement your game logic:public class MyGameServer extends GameServer { @Override protected void initNetty() { setTcpPort(8888); super.initNetty(); } // Implement other necessary methods }
-
Run your game server class to start the server.
Competitor Comparisons
A fast,scalable,distributed game server framework for Node.js.
Pros of Pomelo
- More mature and widely adopted project with a larger community
- Extensive documentation and examples available
- Built-in support for multiple protocols (WebSocket, Socket.io, etc.)
Cons of Pomelo
- Steeper learning curve due to its comprehensive feature set
- Heavier resource usage, which may impact performance for smaller projects
- Less active development in recent years
Code Comparison
Pomelo (JavaScript):
var pomelo = require('pomelo');
var app = pomelo.createApp();
app.set('name', 'MyGame');
app.start();
game-server (Java):
public class GameServer {
public static void main(String[] args) {
Server server = new Server();
server.start();
}
}
Key Differences
- Language: Pomelo is built with JavaScript/Node.js, while game-server uses Java
- Architecture: Pomelo offers a more complex, distributed architecture, whereas game-server provides a simpler, monolithic approach
- Scalability: Pomelo is designed for large-scale games, while game-server is more suitable for smaller projects or prototypes
- Community: Pomelo has a larger user base and more third-party resources available
- Performance: game-server may offer better performance for certain use cases due to its Java backend
Both projects aim to simplify game server development, but they cater to different needs and preferences. Pomelo is better suited for large-scale, distributed games, while game-server offers a more straightforward approach for smaller projects or developers more comfortable with Java.
Unified Realtime/API framework for .NET platform and Unity.
Pros of MagicOnion
- Built on top of gRPC, offering high-performance RPC communication
- Supports real-time streaming and bidirectional communication
- Seamless integration with Unity for game development
Cons of MagicOnion
- Steeper learning curve due to its reliance on gRPC concepts
- Limited to C# and Unity environments, less versatile than game-server
Code Comparison
MagicOnion:
[Service]
public interface IMyFirstService : IService<IMyFirstService>
{
UnaryResult<string> SayHello(string name);
}
game-server:
@Service
public class HelloWorldService {
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
Key Differences
- MagicOnion uses a more declarative approach with interfaces and attributes
- game-server follows a traditional OOP style with concrete classes
- MagicOnion's RPC system is more tightly integrated with its framework
- game-server offers a simpler, more familiar structure for Java developers
Use Cases
MagicOnion is ideal for:
- Unity-based games requiring high-performance networking
- Projects needing real-time bidirectional communication
game-server is better suited for:
- Java-based game servers with traditional architectures
- Developers seeking a more straightforward, less opinionated framework
Both projects aim to simplify game server development, but cater to different ecosystems and programming paradigms.
Distributed server for social and realtime games and apps.
Pros of Nakama
- More comprehensive feature set, including social networking, matchmaking, and leaderboards
- Better documentation and community support
- Cross-platform compatibility (supports multiple programming languages)
Cons of Nakama
- Steeper learning curve due to its extensive features
- Potentially higher resource requirements for deployment and maintenance
- Less flexibility for custom game logic compared to game-server's modular approach
Code Comparison
Nakama (Go):
nk.MatchCreate(ctx, "match_id", map[string]interface{}{
"custom_id": "my_match",
})
game-server (Java):
GameRoom room = new GameRoom(roomId);
roomManager.addRoom(room);
Summary
Nakama offers a more feature-rich and well-supported solution, ideal for developers seeking a comprehensive game server framework. game-server provides a simpler, more modular approach that may be easier to customize for specific game requirements. The choice between the two depends on the project's complexity, required features, and development team's expertise.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
ç®ä»
ä¸ä¸ªåºäºæ£çãMMORPG游æçåå¸å¼java游ææå¡å¨ï¼ç论ä¸å¯ä»¥æ éæ°´å¹³æ©å±ç½å ³æï¼å¤§å æã游ææè¾¾å°äººæ°æ¿è½½ãå®ç°äºé群注åä¸å¿ï¼ç½å ³ãç»éãåå°æå¡å¨çæ§çéç¨æå¡å¨;å°è£ äºredisé群ãmongodbçæ°æ®åºå¤çï¼å°è£ äºæ¶æ¯éåãçº¿ç¨æ¨¡åãå导表ç常ç¨å·¥å ·ç±»ãç½å ³æå¡å¨ä½¿ç¨minaå°è£ äºTCPãUDPãWebSocketãHTTPéä¿¡ï¼ä½¿è¯¥æ¡æ¶è½åæ¶æ¯æå¤ç§åè®®ç客æ·ç«¯è¿è¡æ¸¸æãæ¯ä¸ªä»¥scriptsååç»å°¾çç®å½é½ä¸ºç¸åºé¡¹ç®çèæ¬æä»¶ã
ææ¡£
详ç»è¯·æ¥çwiki
项ç®å·²æ æ°åè½å¼åï¼åªç»´æ¤ï¼
- æ°çæ¬æå¡å¨ å¯åè GameServer4j
- game-ai ç§»å¨å°GameAi4j
TODO
- æ´æ°JDKå°17
交æµ
- QQ群:144709243(已满) 143469012
æè°¢
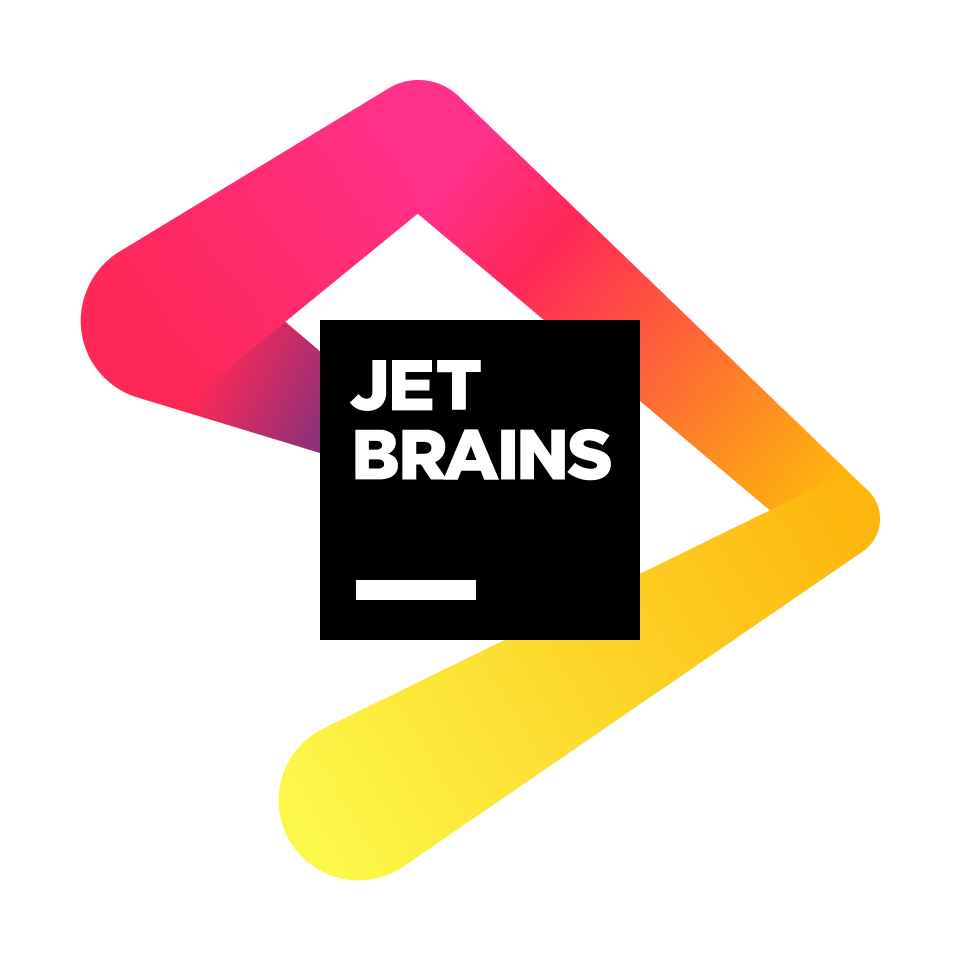
Top Related Projects
A fast,scalable,distributed game server framework for Node.js.
Unified Realtime/API framework for .NET platform and Unity.
Distributed server for social and realtime games and apps.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot