Top Related Projects
A modern animation library for React and JavaScript
GSAP (GreenSock Animation Platform), a JavaScript animation library for the modern web
Javascript library to create physics-based animations
JavaScript/TypeScript animation engine
Quick Overview
Motion One is a lightweight animation library for the web, designed to provide a simple API for creating high-performance animations. It offers a declarative approach to animation, making it easy to create complex animations with minimal code.
Pros
- Lightweight and performant, with a small bundle size
- Easy-to-use API with a declarative syntax
- Supports both CSS and DOM animations
- Provides smooth animations with automatic hardware acceleration
Cons
- Limited documentation compared to more established animation libraries
- Fewer advanced features compared to larger animation frameworks
- May require additional setup for complex animation scenarios
- Relatively new library, which may lead to fewer community resources and examples
Code Examples
Creating a simple fade-in animation:
import { animate } from "motion"
animate(".box", { opacity: 1 }, { duration: 1 })
Animating multiple properties with staggered delays:
import { animate, stagger } from "motion"
animate(".item",
{ opacity: 1, y: 0 },
{ delay: stagger(0.1), duration: 0.5 }
)
Creating a spring animation:
import { animate, spring } from "motion"
animate(".ball",
{ x: 100 },
{ easing: spring() }
)
Getting Started
To get started with Motion One, first install it via npm:
npm install motion
Then, import and use it in your JavaScript file:
import { animate } from "motion"
// Animate an element with the class "box"
animate(".box", {
scale: 1.2,
rotate: 180
}, {
duration: 1,
easing: "ease-in-out"
})
This will create a simple animation that scales and rotates the element with the class "box" over a duration of 1 second, using an ease-in-out easing function.
Competitor Comparisons
A modern animation library for React and JavaScript
Pros of Motion
- Smaller bundle size, resulting in faster load times
- More focused API, potentially easier to learn and use
- Better performance for simple animations
Cons of Motion
- Less feature-rich compared to MotionOne
- May require more custom code for complex animations
- Limited support for advanced animation techniques
Code Comparison
Motion:
animate(element, { opacity: 0, y: 100 }, { duration: 1 })
MotionOne:
animate(
element,
{ opacity: [1, 0], y: [0, 100] },
{ duration: 1, easing: [0.22, 1, 0.36, 1] }
)
Motion focuses on simplicity and performance, making it ideal for basic animations and projects where bundle size is crucial. MotionOne offers more advanced features and flexibility, suitable for complex animations and projects requiring fine-grained control. The code comparison shows that Motion has a more concise syntax, while MotionOne provides more options for customization.
GSAP (GreenSock Animation Platform), a JavaScript animation library for the modern web
Pros of GSAP
- More mature and feature-rich animation library with a larger ecosystem
- Better performance for complex animations and large-scale projects
- Extensive plugin system for advanced features like ScrollTrigger and MorphSVG
Cons of GSAP
- Steeper learning curve due to its extensive API and features
- Larger file size, which may impact initial load times for smaller projects
- Commercial license required for some advanced features and use cases
Code Comparison
GSAP:
gsap.to(".box", {
duration: 1,
x: 100,
y: 50,
rotation: 45,
ease: "power2.inOut"
});
Motion One:
animate(".box", {
x: 100,
y: 50,
rotate: 45
}, {
duration: 1,
easing: [.17, .67, .83, .67]
});
Both libraries offer similar syntax for basic animations, but GSAP provides more built-in easing functions and a wider range of animation properties. Motion One uses a more modern, Promise-based API and focuses on simplicity and performance for web animations. GSAP is generally more suitable for complex projects with advanced animation requirements, while Motion One is ideal for simpler animations and projects prioritizing small bundle sizes.
Javascript library to create physics-based animations
Pros of dynamics.js
- Lightweight and focused on physics-based animations
- Provides a simple API for creating complex animations
- Supports both JavaScript and CSS animations
Cons of dynamics.js
- Less actively maintained (last update in 2018)
- Limited documentation and examples
- Smaller community and ecosystem compared to Motion One
Code Comparison
dynamics.js:
dynamics.animate(element, {
translateX: 100,
scale: 2
}, {
type: dynamics.spring,
duration: 1000
});
Motion One:
import { animate } from "motion";
animate(element, {
x: 100,
scale: 2
}, {
type: "spring",
duration: 1
});
Summary
dynamics.js is a lightweight library focused on physics-based animations with a simple API. However, it's less actively maintained and has limited documentation. Motion One, on the other hand, offers a more comprehensive animation toolkit with better documentation and active development. The code comparison shows similarities in syntax, but Motion One uses more modern JavaScript conventions and offers a wider range of features beyond basic animations.
JavaScript/TypeScript animation engine
Pros of tween.js
- Lightweight and focused solely on tweening, making it easier to integrate into existing projects
- Extensive documentation and a large community, providing better support and resources
- More flexible easing functions, allowing for custom easing and a wider range of animation styles
Cons of tween.js
- Limited to tweening numerical values, lacking support for more complex animations like SVG or color transitions
- Requires more manual setup and management of animations compared to Motion One's declarative API
- Less performant for complex animations or large numbers of concurrent tweens
Code Comparison
tween.js:
const tween = new TWEEN.Tween({ x: 0 })
.to({ x: 100 }, 1000)
.easing(TWEEN.Easing.Quadratic.Out)
.onUpdate((object) => {
element.style.transform = `translateX(${object.x}px)`;
})
.start();
Motion One:
import { animate } from "motion";
animate(element, { x: 100 }, { duration: 1, easing: "ease-out" });
The code comparison demonstrates Motion One's more concise and declarative approach to animations, while tween.js offers more granular control over the animation process.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
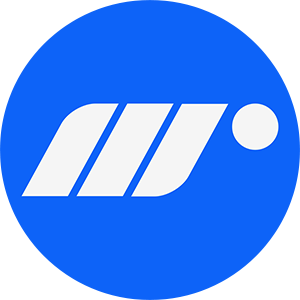
Motion One
This is the Motion One monorepo. It contains the source code for all Motion One libraries.
ðµï¸ââï¸ Source code
motion
: The main entry point for Motion One.@motionone/animation
: A minimal, focused polyfill for WAAPI.@motionone/dom
: DOM-specific APIs likeanimate
andscroll
.@motionone/easing
: JavaScript implementations of web easing functions.@motionone/generators
: Keyframe generators likespring
andglide
.@motionone/types
: Shared types for Motion One packages.@motionone/utils
: Shared utility functions across Motion One packages.
ð DevTools
Create Motion One and CSS animations faster than ever with Motion DevTools.
ð Documentation
Full docs are available at motion.dev.
Top Related Projects
A modern animation library for React and JavaScript
GSAP (GreenSock Animation Platform), a JavaScript animation library for the modern web
Javascript library to create physics-based animations
JavaScript/TypeScript animation engine
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot