Top Related Projects
Next generation testing framework powered by Vite.
Delightful JavaScript Testing.
Storybook is the industry standard workshop for building, documenting, and testing UI components in isolation
🐐 Simple and complete React DOM testing utilities that encourage good testing practices.
Fast, easy and reliable testing for anything that runs in a browser.
Quick Overview
Jest Preview is a tool that enhances Jest testing for React applications by allowing developers to visually debug and interact with components during test execution. It provides a browser-like environment for viewing and manipulating components, making it easier to write and debug UI tests.
Pros
- Improves debugging experience for React component tests
- Allows real-time interaction with components during test execution
- Integrates seamlessly with existing Jest and React Testing Library setups
- Supports various styling solutions like CSS Modules, Sass, and Styled Components
Cons
- Adds some complexity to the testing setup
- May slightly increase test execution time
- Limited to React applications (not suitable for other frameworks)
- Requires additional configuration for certain styling solutions
Code Examples
- Basic usage with React Testing Library:
import { render, screen } from '@testing-library/react';
import { preview } from 'jest-preview';
import MyComponent from './MyComponent';
test('renders MyComponent correctly', () => {
render(<MyComponent />);
preview.debug();
expect(screen.getByText('Hello, World!')).toBeInTheDocument();
});
- Using with custom styles:
import { preview } from 'jest-preview';
import './styles.css';
preview.addStylesheet('./styles.css');
test('component has correct styles', () => {
render(<StyledComponent />);
preview.debug();
// Assert styles here
});
- Debugging specific elements:
import { preview } from 'jest-preview';
test('debug specific element', () => {
const { container } = render(<ComplexComponent />);
const element = container.querySelector('.specific-class');
preview.debug(element);
// Assertions and further testing
});
Getting Started
-
Install Jest Preview:
npm install --save-dev jest-preview
-
Add to your Jest setup file (e.g.,
jest.setup.js
):import 'jest-preview/server';
-
Configure Jest in
package.json
orjest.config.js
:{ "jest": { "setupFilesAfterEnv": ["<rootDir>/jest.setup.js"] } }
-
Use in your tests:
import { preview } from 'jest-preview'; test('your test', () => { // Your test code preview.debug(); });
-
Run tests with Jest Preview server:
npx jest-preview
Competitor Comparisons
Next generation testing framework powered by Vite.
Pros of Vitest
- Faster execution due to Vite-based architecture
- Native TypeScript support without additional configuration
- Broader ecosystem compatibility, including Vue and React
Cons of Vitest
- Less mature and potentially less stable than Jest
- May require additional setup for certain Jest-specific features
- Smaller community and fewer resources compared to Jest ecosystem
Code Comparison
Jest Preview:
import { preview } from 'jest-preview';
test('renders correctly', () => {
render(<MyComponent />);
preview();
});
Vitest:
import { test, expect } from 'vitest';
test('renders correctly', () => {
const result = render(<MyComponent />);
expect(result).toMatchSnapshot();
});
Key Differences
- Jest Preview focuses on visual debugging of Jest tests
- Vitest is a complete testing framework alternative to Jest
- Jest Preview enhances existing Jest setups, while Vitest replaces them
- Vitest leverages Vite for improved performance and modern web development
- Jest Preview provides a unique visual debugging experience not natively available in Vitest
Use Cases
-
Choose Jest Preview when:
- You're already using Jest and need visual debugging
- You want to enhance your existing Jest setup without major changes
-
Choose Vitest when:
- Starting a new project with modern web technologies
- Looking for faster test execution in large projects
- Seeking native TypeScript support and Vite integration
Delightful JavaScript Testing.
Pros of Jest
- Comprehensive testing framework with built-in assertion library and mocking capabilities
- Widely adopted in the JavaScript ecosystem with extensive community support
- Supports various testing scenarios, including unit, integration, and snapshot testing
Cons of Jest
- Lacks built-in visual debugging tools for UI components
- Can be complex to set up and configure for specific use cases
- May require additional plugins or tools for advanced UI testing scenarios
Code Comparison
Jest:
test('renders welcome message', () => {
render(<App />);
const welcomeElement = screen.getByText(/Welcome to React/i);
expect(welcomeElement).toBeInTheDocument();
});
Jest Preview:
test('renders welcome message', () => {
render(<App />);
const welcomeElement = screen.getByText(/Welcome to React/i);
expect(welcomeElement).toBeInTheDocument();
preview.debug();
});
Summary
Jest is a powerful and versatile testing framework for JavaScript applications, offering a wide range of features and strong community support. Jest Preview, on the other hand, is a specialized tool that enhances Jest's capabilities for UI component testing by providing visual debugging features.
While Jest excels in general-purpose testing, Jest Preview addresses the specific need for visual inspection and debugging of UI components during testing. Jest Preview builds upon Jest's foundation, adding functionality that can be particularly useful for frontend developers working with complex UI components.
The choice between the two depends on the specific requirements of your project and the level of visual debugging needed for UI component testing.
Storybook is the industry standard workshop for building, documenting, and testing UI components in isolation
Pros of Storybook
- Comprehensive UI development environment with extensive features for component isolation, documentation, and testing
- Large ecosystem with numerous addons and integrations
- Supports multiple frameworks and libraries (React, Vue, Angular, etc.)
Cons of Storybook
- Steeper learning curve and more complex setup compared to Jest Preview
- Can be overkill for smaller projects or teams primarily focused on unit testing
Code Comparison
Jest Preview:
import { preview } from 'jest-preview';
test('renders correctly', () => {
render(<MyComponent />);
preview();
});
Storybook:
import { Story, Meta } from '@storybook/react';
export default {
title: 'MyComponent',
component: MyComponent,
} as Meta;
const Template: Story = (args) => <MyComponent {...args} />;
Key Differences
- Jest Preview focuses on enhancing Jest tests with visual debugging, while Storybook is a comprehensive UI development environment
- Storybook offers more features for component documentation and interaction, whereas Jest Preview is more lightweight and integrated directly into Jest tests
- Jest Preview is specifically designed for Jest, while Storybook supports various testing frameworks and can be used independently of tests
Use Cases
- Choose Jest Preview for quick visual debugging in Jest tests
- Opt for Storybook for comprehensive UI development, documentation, and testing in larger projects or teams
🐐 Simple and complete React DOM testing utilities that encourage good testing practices.
Pros of react-testing-library
- More established and widely adopted in the React community
- Encourages writing tests that closely resemble how users interact with components
- Extensive documentation and community support
Cons of react-testing-library
- Lacks visual debugging capabilities
- May require more setup for complex component testing scenarios
- Limited built-in snapshot testing features
Code Comparison
react-testing-library:
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders component', () => {
render(<MyComponent />);
expect(screen.getByText('Hello')).toBeInTheDocument();
});
jest-preview:
import { render, screen } from '@testing-library/react';
import { preview } from 'jest-preview';
import MyComponent from './MyComponent';
test('renders component', () => {
render(<MyComponent />);
preview.debug();
expect(screen.getByText('Hello')).toBeInTheDocument();
});
Key Differences
- jest-preview focuses on visual debugging, allowing developers to see rendered components in the browser during tests
- react-testing-library emphasizes testing user interactions and accessibility
- jest-preview integrates with react-testing-library, enhancing its capabilities rather than replacing it entirely
Use Cases
- react-testing-library: Ideal for unit and integration testing of React components
- jest-preview: Useful for visually complex components or when debugging styling issues in tests
Fast, easy and reliable testing for anything that runs in a browser.
Pros of Cypress
- Comprehensive end-to-end testing framework with built-in browser automation
- Extensive ecosystem with plugins and integrations for various tools and frameworks
- Real-time test execution with interactive debugging capabilities
Cons of Cypress
- Steeper learning curve due to its comprehensive nature
- Limited cross-browser testing support (primarily focused on Chrome-based browsers)
- Can be slower for large test suites compared to unit testing frameworks
Code Comparison
Jest Preview:
import { preview } from 'jest-preview';
test('renders correctly', () => {
render(<MyComponent />);
preview();
});
Cypress:
describe('MyComponent', () => {
it('renders correctly', () => {
cy.visit('/my-component');
cy.get('.my-component').should('be.visible');
});
});
Key Differences
- Jest Preview focuses on enhancing Jest's snapshot testing with visual previews
- Cypress is a complete end-to-end testing solution with browser automation
- Jest Preview integrates seamlessly with existing Jest test suites
- Cypress requires a separate test runner and configuration
Use Cases
- Jest Preview: Ideal for component-level testing and visual regression testing
- Cypress: Best suited for full end-to-end testing of web applications
Community and Support
- Jest Preview: Smaller community, focused on Jest ecosystem
- Cypress: Large, active community with extensive documentation and third-party resources
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Jest Preview
Debug your Jest tests. Effortlessly. ð ð¼
Try Jest Preview Online. No downloads needed!
Using Vitest? Try Vitest Preview
Why jest-preview
When writing tests using Jest, we usually debug by reading the HTML code. Sometimes, the HTML is too complicated to visualize the UI in our head. jest-preview
previews your Jest tests right in a browser, then you can see your actual UI visually, which helps you write and debug Jest tests faster.
jest-preview
is initially designed to work with Jest and react-testing-library. The package is framework-agnostic, and you can use it with any frontend frameworks and testing libraries. For examples:
Features
- ð Preview your actual app's HTML in a browser in milliseconds.
- ð Auto reload browser when executing
preview.
debug()`. - ð
Support CSS:
- â Direct CSS import
- â
Number of CSS-in-JS libraries, such as:
- â Styled-components
- â Emotion
- â Global CSS
- â CSS Modules
- â Sass
- ð Support viewing images.
How to use jest-preview
in 2 lines of code
+import preview from 'jest-preview';
describe('App', () => {
it('should work as expected', () => {
render(<App />);
+ preview.debug();
});
});
Or:
+import { debug } from 'jest-preview';
describe('App', () => {
it('should work as expected', () => {
render(<App />);
+ debug();
});
});
You also need to start the Jest Preview Server by running the CLI jest-preview
. Please continue to read Usage for the details instructions.
Feedback
Your feedback is very important to us. Please help jest-preview
becomes a better software by submitting feedback here.
Installation
See the Installation Guide on Jest Preview official website.
Usage
See the Usage Guide on Jest Preview official website.
Advanced configurations
Jest Preview comes with Pre-configured transformation. However, in more advanced use cases where you have custom code transformation, check out the Code Transformation Guide.
Upcoming features
- Support more
css-in-js
libraries. - Multiple previews.
- You name it.
Support
Please file an issue, or add a new discussion if you encounter any issues.
You can also mention @JestPreview or @hung_dev on Twitter if you want to have some more discussions or suggestions.
We also have a Discord server:
Contributing
We can't wait to see your contributions. See the Contribution Guide at CONTRIBUTING.md
Contributors â¨
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind are welcome!
Star history
License
MIT
Sponsors
Your financial support helps the project alive and in a development mode. Make an impact by sponsoring us $1 via Open Collective.
- Bronze Sponsor ð¥:
- Your company's logo/ profile picture on README.md and www.jest-preview.com
- Silver Sponsor ð¥:
- All of these above
- Your requests will be prioritized.
- Gold Sponsor ð¥:
- All of these above
- Let's discuss your benefits for this tier, please contact the author
- Diamond Sponsor ð:
- All of these above
- Let's discuss your benefits for this tier, please contact the author
Bronze Sponsors ð¥

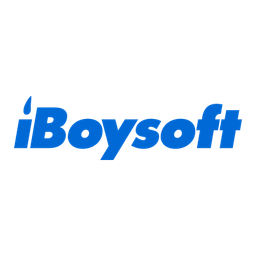
Past Sponsors
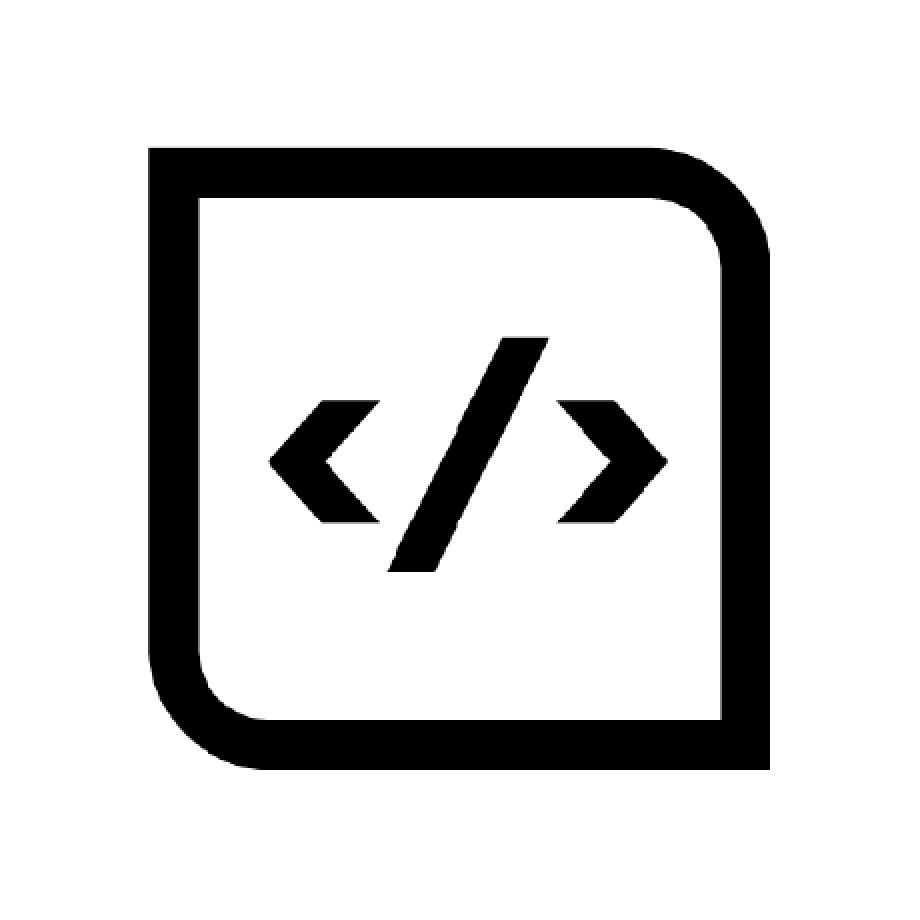

Top Related Projects
Next generation testing framework powered by Vite.
Delightful JavaScript Testing.
Storybook is the industry standard workshop for building, documenting, and testing UI components in isolation
🐐 Simple and complete React DOM testing utilities that encourage good testing practices.
Fast, easy and reliable testing for anything that runs in a browser.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot