jose
JWA, JWS, JWE, JWT, JWK, JWKS for Node.js, Browser, Cloudflare Workers, Deno, Bun, and other Web-interoperable runtimes
Top Related Projects
JsonWebToken implementation for node.js http://self-issued.info/docs/draft-ietf-oauth-json-web-token.html
Java JWT: JSON Web Token for Java and Android
A simple library to work with JSON Web Token and JSON Web Signature
PHP package for JWT
A modern I/O library for Android, Java, and Kotlin Multiplatform.
Quick Overview
panva/jose is a JavaScript module that implements JSON Object Signing and Encryption (JOSE) standards. It provides a comprehensive set of tools for working with JSON Web Tokens (JWT), JSON Web Signatures (JWS), JSON Web Encryption (JWE), and related technologies. The library is designed to be versatile, secure, and easy to use in both Node.js and browser environments.
Pros
- Comprehensive implementation of JOSE standards
- Supports both Node.js and browser environments
- Actively maintained with regular updates
- Extensive documentation and examples
Cons
- Learning curve for developers new to JOSE concepts
- Dependency on Web Crypto API in browser environments
- Limited support for older Node.js versions
Code Examples
- Creating and verifying a JWT:
import * as jose from 'jose'
const secret = new TextEncoder().encode('your-secret')
const jwt = await new jose.SignJWT({ 'urn:example:claim': true })
.setProtectedHeader({ alg: 'HS256' })
.setIssuedAt()
.setExpirationTime('2h')
.sign(secret)
const { payload, protectedHeader } = await jose.jwtVerify(jwt, secret)
- Encrypting and decrypting data:
import * as jose from 'jose'
const secretKey = await jose.generateSecret('HS256')
const jwe = await new jose.CompactEncrypt(
new TextEncoder().encode('Hello World!')
)
.setProtectedHeader({ alg: 'dir', enc: 'A256GCM' })
.encrypt(secretKey)
const { plaintext, protectedHeader } = await jose.compactDecrypt(jwe, secretKey)
- Generating and verifying JWS:
import * as jose from 'jose'
const privateKey = await jose.generateKeyPair('ES256')
const jws = await new jose.CompactSign(
new TextEncoder().encode('It's a dangerous business, Frodo, going out your door.')
)
.setProtectedHeader({ alg: 'ES256' })
.sign(privateKey.privateKey)
const { payload, protectedHeader } = await jose.compactVerify(jws, privateKey.publicKey)
Getting Started
To use panva/jose in your project, first install it via npm:
npm install jose
Then, import and use the library in your JavaScript code:
import * as jose from 'jose'
// Use jose functions here
const jwt = await new jose.SignJWT({ 'urn:example:claim': true })
.setProtectedHeader({ alg: 'HS256' })
.setIssuedAt()
.setExpirationTime('2h')
.sign(secret)
For more detailed usage instructions and API documentation, refer to the official documentation at https://github.com/panva/jose.
Competitor Comparisons
JsonWebToken implementation for node.js http://self-issued.info/docs/draft-ietf-oauth-json-web-token.html
Pros of node-jsonwebtoken
- Simpler API, easier to get started for basic JWT operations
- Well-established library with a large user base and community support
- Focused specifically on JWT, making it lightweight for simple use cases
Cons of node-jsonwebtoken
- Limited support for other JWS/JWE operations beyond basic JWT
- Less frequent updates and potentially slower adoption of new standards
- Fewer cryptographic algorithm options compared to jose
Code Comparison
node-jsonwebtoken:
const jwt = require('jsonwebtoken');
const token = jwt.sign({ foo: 'bar' }, 'secret', { expiresIn: '1h' });
const decoded = jwt.verify(token, 'secret');
jose:
const jose = require('jose');
const secret = new TextEncoder().encode('secret');
const jwt = await new jose.SignJWT({ foo: 'bar' })
.setProtectedHeader({ alg: 'HS256' })
.setExpirationTime('1h')
.sign(secret);
const { payload } = await jose.jwtVerify(jwt, secret);
Summary
node-jsonwebtoken is a popular choice for simple JWT operations with an easy-to-use API, while jose offers a more comprehensive solution for various JSON Object Signing and Encryption (JOSE) standards, including advanced features and a wider range of algorithms. jose provides better support for newer standards and more cryptographic options, but may have a steeper learning curve for basic use cases.
Java JWT: JSON Web Token for Java and Android
Pros of jjwt
- More comprehensive documentation and examples
- Wider range of supported algorithms and features
- Better integration with Java ecosystem and frameworks
Cons of jjwt
- Limited to Java language, while jose supports multiple languages
- Larger library size and potential performance overhead
- Less frequent updates and maintenance compared to jose
Code Comparison
jjwt:
String jwt = Jwts.builder()
.setSubject("user123")
.signWith(SignatureAlgorithm.HS256, "secret")
.compact();
jose:
const jwt = await new jose.SignJWT({ sub: 'user123' })
.setProtectedHeader({ alg: 'HS256' })
.sign(new TextEncoder().encode('secret'));
Both libraries provide similar functionality for creating and signing JWTs, but jose offers a more modern, Promise-based API. jjwt's API is more Java-centric and follows traditional object-oriented patterns.
jjwt is ideal for Java-based projects that require extensive JWT functionality and integration with Java frameworks. jose is better suited for projects that need cross-language support or prefer a more lightweight, modern API approach.
A simple library to work with JSON Web Token and JSON Web Signature
Pros of JWT
- More comprehensive documentation and examples
- Supports a wider range of PHP versions (5.6+)
- Easier to use for simple JWT operations
Cons of JWT
- Less frequent updates and maintenance
- Fewer supported algorithms and features compared to JOSE
- Limited support for JWE (JSON Web Encryption)
Code Comparison
JWT:
$token = (new Builder())
->withClaim('uid', 1)
->getToken(new Sha256(), new Key('secret'));
echo $token->toString();
JOSE:
$jwt = new SignatureJwt(
['alg' => 'HS256'],
['uid' => 1]
);
$jwt->sign(new HS256(), 'secret');
echo $jwt->toCompactSerialization();
Both libraries provide functionality for creating and verifying JWTs, but JOSE offers a more extensive set of features and adheres more closely to the JOSE specifications. JWT is simpler to use for basic operations, while JOSE provides more flexibility and support for advanced use cases.
JWT is a good choice for projects that require straightforward JWT handling and have simpler requirements. JOSE is better suited for applications that need comprehensive JOSE implementation, including support for JWE and a wider range of algorithms.
PHP package for JWT
Pros of php-jwt
- Lightweight and simple implementation focused specifically on JWT
- Easy to integrate into PHP projects with minimal dependencies
- Well-established and maintained by Firebase, a reputable organization
Cons of php-jwt
- Limited to JWT functionality, lacking support for other JOSE standards
- Less comprehensive feature set compared to jose
- PHP-specific, not suitable for projects using other languages
Code Comparison
php-jwt:
use Firebase\JWT\JWT;
$payload = ['data' => 'example'];
$jwt = JWT::encode($payload, $key, 'HS256');
$decoded = JWT::decode($jwt, $key, ['HS256']);
jose:
const jose = require('jose');
const jwt = await new jose.SignJWT({ data: 'example' })
.setProtectedHeader({ alg: 'HS256' })
.sign(key);
const { payload } = await jose.jwtVerify(jwt, key);
Summary
php-jwt is a straightforward JWT library for PHP, ideal for simple JWT implementations. jose offers a more comprehensive JOSE implementation with support for multiple languages and standards. php-jwt is easier to integrate for PHP-specific projects, while jose provides greater flexibility and features across various environments.
A modern I/O library for Android, Java, and Kotlin Multiplatform.
Pros of Okio
- Broader scope: Okio is a general-purpose I/O library, offering more functionality beyond cryptographic operations
- Performance-focused: Designed for efficient handling of large data streams and files
- Strong backing: Developed and maintained by Square, a well-known tech company
Cons of Okio
- Less specialized: Not specifically tailored for JSON Web Token (JWT) and JSON Web Encryption (JWE) operations
- Steeper learning curve: May require more time to understand and implement for specific cryptographic tasks
- Language limitation: Primarily focused on Java and Kotlin, while Jose supports multiple languages
Code Comparison
Jose (JavaScript):
const jwt = await new jose.SignJWT({ 'urn:example:claim': true })
.setProtectedHeader({ alg: 'ES256' })
.sign(privateKey)
Okio (Kotlin):
val sink = buffer.outputStream().sink().buffer()
sink.writeUtf8("Hello, Okio!")
sink.close()
Note: The code snippets demonstrate different use cases due to the libraries' distinct purposes. Jose focuses on JWT operations, while Okio handles general I/O tasks.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
jose
jose
is JavaScript module for JSON Object Signing and Encryption, providing support for JSON Web Tokens (JWT), JSON Web Signature (JWS), JSON Web Encryption (JWE), JSON Web Key (JWK), JSON Web Key Set (JWKS), and more. The module is designed to work across various Web-interoperable runtimes including Node.js, browsers, Cloudflare Workers, Deno, Bun, and others.
Sponsor
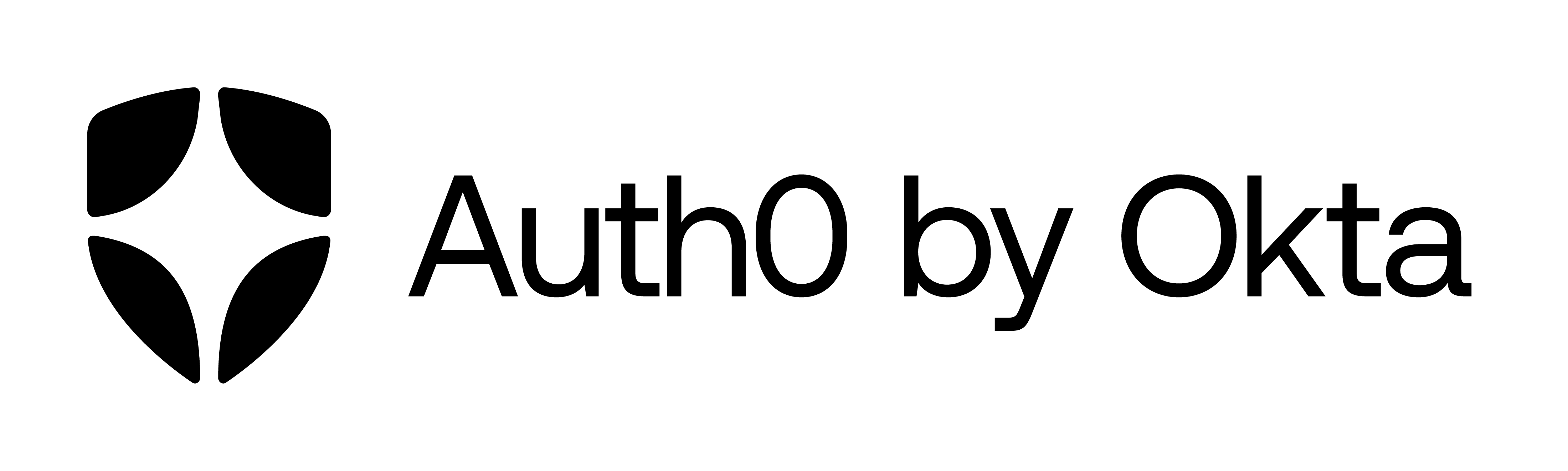
If you want to quickly add JWT authentication to JavaScript apps, feel free to check out Auth0's JavaScript SDK and free plan. Create an Auth0 account; it's free!
ð Help the project
Support from the community to continue maintaining and improving this module is welcome. If you find the module useful, please consider supporting the project by becoming a sponsor.
Dependencies: 0
jose
has no dependencies and it exports tree-shakeable ESM1.
Documentation
jose
is distributed via npmjs.com, jsr.io, jsdelivr.com, and github.com.
example
ESM import1
import * as jose from 'jose'
JSON Web Tokens (JWT)
The jose
module supports JSON Web Tokens (JWT) and provides functionality for signing and verifying tokens, as well as their JWT Claims Set validation.
- JWT Claims Set Validation & Signature Verification using the
jwtVerify
function - Signing using the
SignJWT
class - Utility functions
- Decoding Token's Protected Header
- Decoding JWT Claims Set prior to its validation
Encrypted JSON Web Tokens
The jose
module supports encrypted JSON Web Tokens and provides functionality for encrypting and decrypting tokens, as well as their JWT Claims Set validation.
- Decryption & JWT Claims Set Validation using the
jwtDecrypt
function - Encryption using the
EncryptJWT
class - Utility functions
Key Utilities
The jose
module supports importing, exporting, and generating keys and secrets in various formats, including PEM formats like SPKI, X.509 certificate, and PKCS #8, as well as JSON Web Key (JWK).
- Key Import Functions
- Key and Secret Generation Functions
- Key Export Functions
JSON Web Signature (JWS)
The jose
module supports signing and verification of JWS messages with arbitrary payloads in Compact, Flattened JSON, and General JSON serialization syntaxes.
- Signing - Compact, Flattened JSON, General JSON
- Verification - Compact, Flattened JSON, General JSON
- Utility functions
JSON Web Encryption (JWE)
The jose
module supports encryption and decryption of JWE messages with arbitrary plaintext in Compact, Flattened JSON, and General JSON serialization syntaxes.
- Encryption - Compact, Flattened JSON, General JSON
- Decryption - Compact, Flattened JSON, General JSON
- Utility functions
Other
The following are additional features and utilities provided by the jose
module:
- Calculating JWK Thumbprint
- Calculating JWK Thumbprint URI
- Verification using a JWK Embedded in a JWS Header
- Unsecured JWT
- JOSE Errors
Supported Runtimes
The jose
module is compatible with JavaScript runtimes that support the utilized Web API globals and standard built-in objects or are Node.js.
The following runtimes are supported (this is not an exhaustive list):
Please note that certain algorithms may not be available depending on the runtime used. You can find a list of available algorithms for each runtime in the specific issue links provided above.
Supported Versions
Version | Security Fixes ð | Other Bug Fixes ð | New Features â | Runtime and Module type |
---|---|---|---|---|
v6.x | Security Policy | â | â | Universal2 ESM1 |
v5.x | Security Policy | â | â | Universal2 CJS + ESM |
v4.x | Security Policy | â | â | Universal2 CJS + ESM |
v2.x | Security Policy | â | â | Node.js CJS |
Specifications
Details
- JSON Web Signature (JWS) - RFC7515
- JSON Web Encryption (JWE) - RFC7516
- JSON Web Key (JWK) - RFC7517
- JSON Web Algorithms (JWA) - RFC7518
- JSON Web Token (JWT) - RFC7519
- JSON Web Key Thumbprint - RFC7638
- JSON Web Key Thumbprint URI - RFC9278
- JWS Unencoded Payload Option - RFC7797
- CFRG Elliptic Curve ECDH and Signatures - RFC8037
The algorithm implementations in jose
have been tested using test vectors from their respective specifications as well as RFC7520.
Footnotes
Top Related Projects
JsonWebToken implementation for node.js http://self-issued.info/docs/draft-ietf-oauth-json-web-token.html
Java JWT: JSON Web Token for Java and Android
A simple library to work with JSON Web Token and JSON Web Signature
PHP package for JWT
A modern I/O library for Android, Java, and Kotlin Multiplatform.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot