react-refresh-webpack-plugin
A Webpack plugin to enable "Fast Refresh" (also previously known as Hot Reloading) for React components.
Top Related Projects
Set up a modern web app by running one command.
The React Framework
Next generation frontend tooling. It's fast!
Serves a webpack app. Updates the browser on changes. Documentation https://webpack.js.org/configuration/dev-server/.
Tweak React components in real time. (Deprecated: use Fast Refresh instead.)
Create and build modern JavaScript projects with zero initial configuration.
Quick Overview
The react-refresh-webpack-plugin
is a Webpack plugin that enables fast, reliable, and consistent live-reloading for React applications. It provides a seamless development experience by automatically updating the browser when changes are made to the codebase, without the need for a full page refresh.
Pros
- Fast and Reliable: The plugin leverages React's built-in Fast Refresh feature, ensuring that updates are applied quickly and without interrupting the user's workflow.
- Consistent Behavior: The plugin maintains a consistent behavior across different browsers and environments, providing a reliable development experience.
- Easy Integration: The plugin is designed to be easily integrated into existing Webpack-based React projects, with minimal configuration required.
- Compatibility: The plugin supports a wide range of React versions, from 16.9 to the latest, ensuring compatibility with your project's requirements.
Cons
- Webpack-specific: The plugin is designed specifically for Webpack, which may limit its usefulness for developers using other bundlers like Rollup or Parcel.
- Potential Compatibility Issues: While the plugin supports a wide range of React versions, there may be edge cases or specific project configurations where compatibility issues could arise.
- Limited Customization: The plugin provides a relatively limited set of configuration options, which may not meet the needs of all developers.
- Dependency on React Fast Refresh: The plugin's performance and reliability are dependent on the stability and performance of the React Fast Refresh feature, which is still an experimental technology.
Code Examples
Here are a few examples of how to use the react-refresh-webpack-plugin
in your Webpack configuration:
- Basic Configuration:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
module.exports = {
// ... other Webpack configuration
plugins: [
new ReactRefreshWebpackPlugin(),
],
devServer: {
hot: true,
},
};
- Customizing the Plugin:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
module.exports = {
// ... other Webpack configuration
plugins: [
new ReactRefreshWebpackPlugin({
overlay: {
sockPort: 8080, // Custom socket port
},
}),
],
devServer: {
hot: true,
port: 8080, // Custom dev server port
},
};
- Integrating with Babel:
module.exports = {
// ... other Webpack configuration
module: {
rules: [
{
test: /\.(js|jsx)$/,
exclude: /node_modules/,
use: [
{
loader: 'babel-loader',
options: {
plugins: [
require.resolve('react-refresh/babel'),
],
},
},
],
},
],
},
plugins: [
new ReactRefreshWebpackPlugin(),
],
devServer: {
hot: true,
},
};
- Disabling the Plugin in Production:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
module.exports = (env) => {
const isProduction = env.production;
return {
// ... other Webpack configuration
plugins: [
!isProduction && new ReactRefreshWebpackPlugin(),
].filter(Boolean),
devServer: {
hot: !isProduction,
},
};
};
Getting Started
To get started with the react-refresh-webpack-plugin
, follow these steps:
- Install the plugin and its dependencies:
npm install --save-dev @pmmmwh/react-refresh-webpack-plugin react-refresh
- Update your Webpack configuration to include the plugin:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
module.exports = {
// ... other Webpack configuration
plugins: [
new ReactRefreshWeb
Competitor Comparisons
Set up a modern web app by running one command.
Pros of Create React App
- Provides a complete, opinionated setup for React projects out of the box
- Includes a development server, build tools, and testing utilities
- Abstracts away complex configuration, making it easier for beginners
Cons of Create React App
- Less flexibility in configuration compared to custom Webpack setups
- Can be overkill for smaller projects or those requiring specific optimizations
- Updating to newer versions can sometimes be challenging due to dependencies
Code Comparison
Create React App:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(<App />, document.getElementById('root'));
React Refresh Webpack Plugin:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
module.exports = {
// ... other webpack config
plugins: [
new ReactRefreshWebpackPlugin(),
],
};
While Create React App provides a complete React development environment, React Refresh Webpack Plugin focuses specifically on enabling fast refresh functionality in custom Webpack setups. Create React App is ideal for quickly starting new React projects, whereas React Refresh Webpack Plugin offers more granular control over the development experience in existing Webpack configurations.
The React Framework
Pros of Next.js
- Full-featured React framework with built-in routing, server-side rendering, and API routes
- Automatic code splitting and optimized performance out of the box
- Extensive ecosystem and community support
Cons of Next.js
- Steeper learning curve for developers new to server-side rendering concepts
- Less flexibility in customizing the build process compared to a standalone Webpack plugin
Code Comparison
Next.js (pages/index.js):
export default function Home() {
return <h1>Welcome to Next.js!</h1>
}
react-refresh-webpack-plugin (webpack.config.js):
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
module.exports = {
// ... other webpack configurations
plugins: [new ReactRefreshWebpackPlugin()],
};
Summary
Next.js is a comprehensive React framework that provides a full-stack solution, while react-refresh-webpack-plugin is a focused tool for enabling Fast Refresh in Webpack-based React applications. Next.js offers more features and abstractions but may be overkill for simpler projects. The Webpack plugin provides more granular control over the development experience but requires manual setup and integration with other tools.
Next generation frontend tooling. It's fast!
Pros of Vite
- Faster development server startup and hot module replacement
- Built-in support for TypeScript, JSX, and CSS modules without additional configuration
- Optimized build process with pre-bundling of dependencies
Cons of Vite
- Less mature ecosystem compared to Webpack-based solutions
- May require adjustments for projects heavily reliant on Webpack-specific features
- Limited plugin ecosystem compared to Webpack
Code Comparison
react-refresh-webpack-plugin configuration:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
module.exports = {
// ... other webpack config
plugins: [new ReactRefreshWebpackPlugin()],
};
Vite configuration:
// vite.config.js
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
export default defineConfig({
plugins: [react()]
})
Summary
Vite offers a more streamlined development experience with faster startup times and built-in support for modern web technologies. However, react-refresh-webpack-plugin may be more suitable for projects deeply integrated with Webpack or requiring specific Webpack features. Vite's configuration is generally simpler, but it may require additional adjustments for complex projects transitioning from Webpack.
Serves a webpack app. Updates the browser on changes. Documentation https://webpack.js.org/configuration/dev-server/.
Pros of webpack-dev-server
- More comprehensive development server solution with built-in hot module replacement (HMR)
- Broader compatibility with various webpack configurations and project types
- Extensive documentation and large community support
Cons of webpack-dev-server
- Heavier setup and configuration required for optimal performance
- May not provide as seamless React component state preservation during hot reloading
Code Comparison
webpack-dev-server configuration:
module.exports = {
devServer: {
contentBase: './dist',
hot: true
}
};
react-refresh-webpack-plugin configuration:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
module.exports = {
plugins: [
new ReactRefreshWebpackPlugin()
]
};
Summary
webpack-dev-server is a more versatile development server solution with broader application, while react-refresh-webpack-plugin focuses specifically on enhancing React development experience with improved hot reloading capabilities. The choice between the two depends on project requirements and the level of React-specific optimization needed.
Tweak React components in real time. (Deprecated: use Fast Refresh instead.)
Pros of react-hot-loader
- More mature and battle-tested, with a longer history of use in production environments
- Supports a wider range of React versions, including older ones
- Offers more configuration options for advanced use cases
Cons of react-hot-loader
- Higher performance overhead, especially for larger applications
- More complex setup and configuration process
- Occasional issues with state preservation during hot reloading
Code Comparison
react-hot-loader:
import { hot } from 'react-hot-loader/root';
import App from './App';
export default hot(App);
react-refresh-webpack-plugin:
// No additional code required in your React components
// Configuration is done in webpack.config.js
react-refresh-webpack-plugin generally requires less boilerplate code in your React components, as most of the configuration is done in the webpack config file. This leads to cleaner component code and easier maintenance.
react-hot-loader, while more verbose, offers greater flexibility in terms of which components are hot-reloadable and how they behave during updates.
Overall, react-refresh-webpack-plugin is becoming the preferred choice for newer projects due to its improved performance, simpler setup, and better integration with modern React features. However, react-hot-loader still has its place, especially in projects with specific requirements or those using older React versions.
Create and build modern JavaScript projects with zero initial configuration.
Pros of Neutrino
- More comprehensive build tool that provides a complete development environment
- Offers a modular approach with presets for various frameworks and tools
- Supports multiple programming paradigms and project types
Cons of Neutrino
- Steeper learning curve due to its broader scope and functionality
- May introduce unnecessary complexity for simpler projects
- Less focused on specific React hot reloading functionality
Code Comparison
Neutrino configuration:
module.exports = {
use: [
'@neutrinojs/react',
'@neutrinojs/jest',
neutrino => {
neutrino.config.module.rule('compile').use('babel').options({
plugins: [require.resolve('react-refresh/babel')]
});
}
]
};
React Refresh Webpack Plugin configuration:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
module.exports = {
plugins: [new ReactRefreshWebpackPlugin()]
};
While Neutrino provides a more comprehensive build setup, React Refresh Webpack Plugin focuses specifically on enabling fast refresh functionality for React applications. Neutrino requires more configuration but offers greater flexibility, whereas React Refresh Webpack Plugin is simpler to set up for its specific use case.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
React Refresh Webpack Plugin
An EXPERIMENTAL Webpack plugin to enable "Fast Refresh" (also known as Hot Reloading) for React components.
This plugin is not 100% stable. We're hoping to land a v1 release soon - please help us by reporting any issues you've encountered!
Getting Started
Prerequisites
Ensure that you are using at least the minimum supported versions of this plugin's peer dependencies - older versions unfortunately do not contain code to orchestrate "Fast Refresh", and thus cannot be made compatible.
We recommend using the following versions:
Dependency | Version |
---|---|
react | 16.13.0 +, 17.x or 18.x |
react-dom | 16.13.0 +, 17.x or 18.x |
react-refresh | 0.10.0 + |
webpack | 4.46.0 + or 5.2.0 + |
Minimum requirements
Dependency | Version |
---|---|
react | 16.9.0 |
react-dom | 16.9.0 |
react-refresh | 0.10.0 |
webpack | 4.43.0 |
Using custom renderers (e.g. react-three-fiber
, react-pdf
, ink
)
To ensure full support of "Fast Refresh" with components rendered by custom renderers,
you should ensure the renderer you're using depends on a recent version of react-reconciler
.
We recommend version 0.25.0
or above, but any versions above 0.22.0
should work.
If the renderer is not compatible, please file them an issue instead.
Installation
With all prerequisites met, you can install this plugin using your package manager of choice:
# if you prefer npm
npm install -D @pmmmwh/react-refresh-webpack-plugin react-refresh
# if you prefer yarn
yarn add -D @pmmmwh/react-refresh-webpack-plugin react-refresh
# if you prefer pnpm
pnpm add -D @pmmmwh/react-refresh-webpack-plugin react-refresh
The react-refresh
package (from the React team) is a required peer dependency of this plugin.
We recommend using version 0.10.0
or above.
Support for TypeScript
TypeScript support is available out-of-the-box for those who use webpack.config.ts
.
Our exported types however depends on type-fest
, so you'll have to add it as a devDependency
:
# if you prefer npm
npm install -D type-fest
# if you prefer yarn
yarn add -D type-fest
# if you prefer pnpm
pnpm add -D type-fest
:memo: Note:
type-fest@4.x
only supports Node.js v16 or above,type-fest@3.x
only supports Node.js v14.16 or above, andtype-fest@2.x
only supports Node.js v12.20 or above. If you're using an older version of Node.js, please installtype-fest@1.x
.
Usage
For most setups, we recommend integrating with babel-loader
.
It covers the most use cases and is officially supported by the React team.
The example below will assume you're using webpack-dev-server
.
If you haven't done so, set up your development Webpack configuration for Hot Module Replacement (HMR).
const isDevelopment = process.env.NODE_ENV !== 'production';
module.exports = {
mode: isDevelopment ? 'development' : 'production',
devServer: {
hot: true,
},
};
Using webpack-hot-middleware
const webpack = require('webpack');
const isDevelopment = process.env.NODE_ENV !== 'production';
module.exports = {
mode: isDevelopment ? 'development' : 'production',
plugins: [isDevelopment && new webpack.HotModuleReplacementPlugin()].filter(Boolean),
};
Using webpack-plugin-serve
const { WebpackPluginServe } = require('webpack-plugin-serve');
const isDevelopment = process.env.NODE_ENV !== 'production';
module.exports = {
mode: isDevelopment ? 'development' : 'production',
plugins: [isDevelopment && new WebpackPluginServe()].filter(Boolean),
};
Then, add the react-refresh/babel
plugin to your Babel configuration and this plugin to your Webpack configuration.
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
const isDevelopment = process.env.NODE_ENV !== 'production';
module.exports = {
mode: isDevelopment ? 'development' : 'production',
module: {
rules: [
{
test: /\.[jt]sx?$/,
exclude: /node_modules/,
use: [
{
loader: require.resolve('babel-loader'),
options: {
plugins: [isDevelopment && require.resolve('react-refresh/babel')].filter(Boolean),
},
},
],
},
],
},
plugins: [isDevelopment && new ReactRefreshWebpackPlugin()].filter(Boolean),
};
:memo: Note:
Ensure both the Babel transform (
react-refresh/babel
) and this plugin are enabled only indevelopment
mode!
Using ts-loader
:warning: Warning: This is an un-official integration maintained by the community.
Install react-refresh-typescript
.
Ensure your TypeScript version is at least 4.0.
# if you prefer npm
npm install -D react-refresh-typescript
# if you prefer yarn
yarn add -D react-refresh-typescript
# if you prefer pnpm
pnpm add -D react-refresh-typescript
Then, instead of wiring up react-refresh/babel
via babel-loader
,
you can wire-up react-refresh-typescript
with ts-loader
:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
const ReactRefreshTypeScript = require('react-refresh-typescript');
const isDevelopment = process.env.NODE_ENV !== 'production';
module.exports = {
mode: isDevelopment ? 'development' : 'production',
module: {
rules: [
{
test: /\.[jt]sx?$/,
exclude: /node_modules/,
use: [
{
loader: require.resolve('ts-loader'),
options: {
getCustomTransformers: () => ({
before: [isDevelopment && ReactRefreshTypeScript()].filter(Boolean),
}),
transpileOnly: isDevelopment,
},
},
],
},
],
},
plugins: [isDevelopment && new ReactRefreshWebpackPlugin()].filter(Boolean),
};
It is recommended to run
ts-loader
withtranspileOnly
is set totrue
. You can useForkTsCheckerWebpackPlugin
as an alternative if you need typechecking during development.
Using swc-loader
:warning: Warning: This is an un-official integration maintained by the community.
Ensure your @swc/core
version is at least 1.2.86
.
It is also recommended to use swc-loader
version 0.1.13
or above.
Then, instead of wiring up react-refresh/babel
via babel-loader
,
you can wire-up swc-loader
and use the refresh
transform:
const ReactRefreshWebpackPlugin = require('@pmmmwh/react-refresh-webpack-plugin');
const isDevelopment = process.env.NODE_ENV !== 'production';
module.exports = {
mode: isDevelopment ? 'development' : 'production',
module: {
rules: [
{
test: /\.[jt]sx?$/,
exclude: /node_modules/,
use: [
{
loader: require.resolve('swc-loader'),
options: {
jsc: {
transform: {
react: {
development: isDevelopment,
refresh: isDevelopment,
},
},
},
},
},
],
},
],
},
plugins: [isDevelopment && new ReactRefreshWebpackPlugin()].filter(Boolean),
};
Starting from version
0.1.13
,swc-loader
will set thedevelopment
option based on Webpack'smode
option.swc
won't enable fast refresh whendevelopment
isfalse
.
For more information on how to set up "Fast Refresh" with different integrations, please check out our examples.
Overlay Integration
This plugin integrates with the most common Webpack HMR solutions to surface errors during development - in the form of an error overlay.
By default, webpack-dev-server
is used,
but you can set the overlay.sockIntegration
option to match what you're using.
The supported versions are as follows:
Dependency | Version |
---|---|
webpack-dev-server | 3.6.0 + or 4.x or 5.x |
webpack-hot-middleware | 2.x |
webpack-plugin-serve | 0.x or 1.x |
API
Please refer to the API docs for all available options.
FAQs and Troubleshooting
Please refer to the Troubleshooting guide for FAQs and resolutions to common issues.
License
This project is licensed under the terms of the MIT License.
Special Thanks
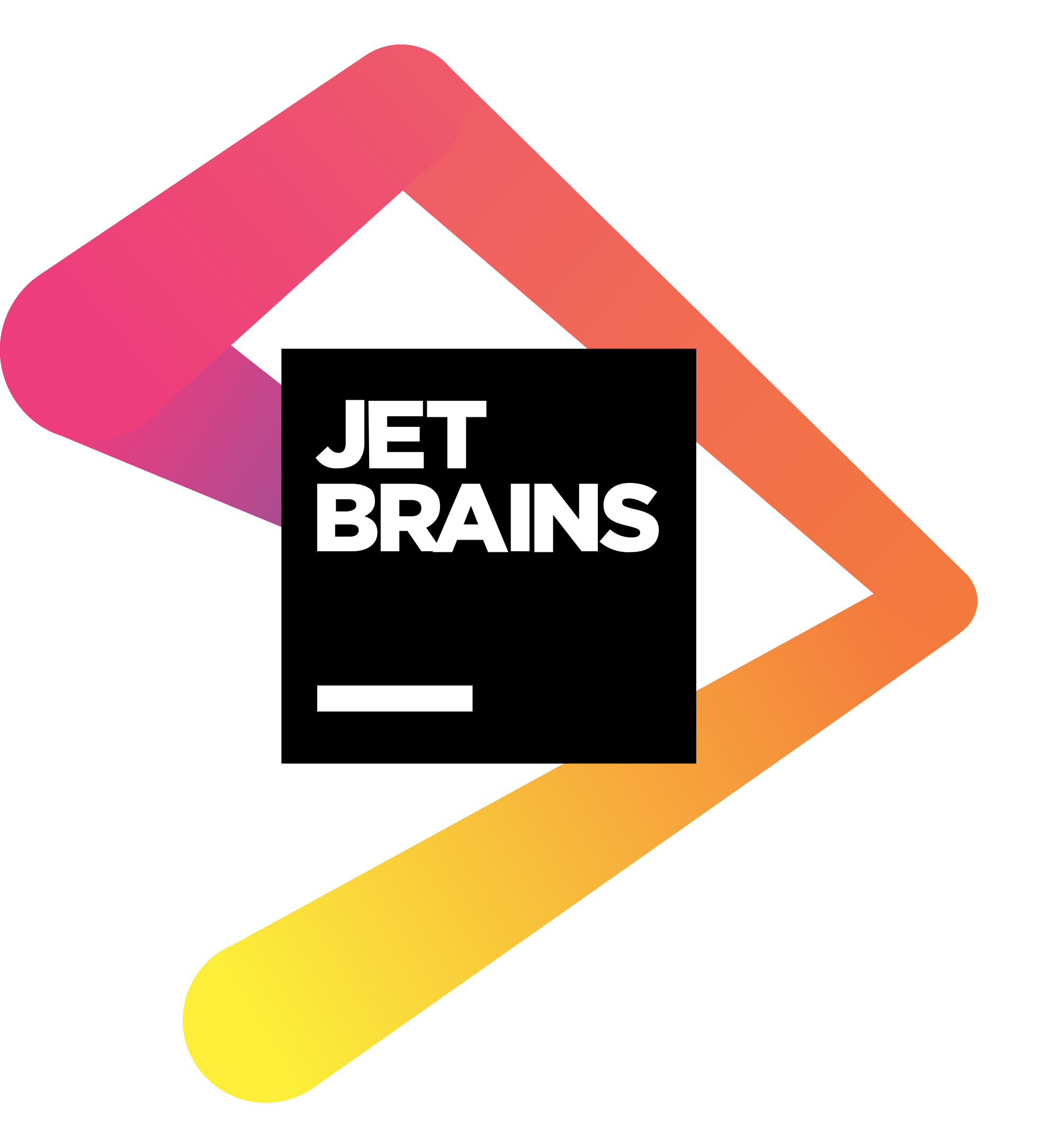
Top Related Projects
Set up a modern web app by running one command.
The React Framework
Next generation frontend tooling. It's fast!
Serves a webpack app. Updates the browser on changes. Documentation https://webpack.js.org/configuration/dev-server/.
Tweak React components in real time. (Deprecated: use Fast Refresh instead.)
Create and build modern JavaScript projects with zero initial configuration.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot