Top Related Projects
Playwright is a framework for Web Testing and Automation. It allows testing Chromium, Firefox and WebKit with a single API.
Fast, easy and reliable testing for anything that runs in a browser.
A browser automation framework and ecosystem.
Cross-platform automation framework for all kinds of apps, built on top of the W3C WebDriver protocol
Next-gen browser and mobile automation test framework for Node.js
Quick Overview
Puppeteer is a Node.js library developed by Google that provides a high-level API to control Chrome or Chromium over the DevTools Protocol. It allows developers to automate browser actions, generate screenshots and PDFs, crawl websites, and perform end-to-end testing of web applications.
Pros
- Powerful automation capabilities for web scraping, testing, and generating PDFs/screenshots
- Headless mode support for faster execution and server-side usage
- Extensive API with good documentation and active community support
- Built and maintained by Google, ensuring reliability and regular updates
Cons
- Can be resource-intensive, especially when running multiple instances
- Learning curve for developers new to browser automation
- Limited cross-browser support (primarily focused on Chrome/Chromium)
- May require additional setup for certain use cases (e.g., running in Docker containers)
Code Examples
- Navigating to a website and taking a screenshot:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await page.screenshot({ path: 'example.png' });
await browser.close();
})();
- Extracting data from a web page:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const title = await page.title();
const text = await page.$eval('body', el => el.textContent);
console.log(`Title: ${title}`);
console.log(`Text content: ${text}`);
await browser.close();
})();
- Filling out and submitting a form:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/form');
await page.type('#username', 'myusername');
await page.type('#password', 'mypassword');
await page.click('button[type="submit"]');
await page.waitForNavigation();
console.log('Form submitted successfully');
await browser.close();
})();
Getting Started
To get started with Puppeteer, follow these steps:
-
Install Puppeteer in your Node.js project:
npm install puppeteer
-
Create a new JavaScript file (e.g.,
script.js
) and add the following code:const puppeteer = require('puppeteer'); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('https://example.com'); console.log('Page title:', await page.title()); await browser.close(); })();
-
Run the script using Node.js:
node script.js
This basic example demonstrates how to launch a browser, navigate to a website, retrieve the page title, and close the browser.
Competitor Comparisons
Playwright is a framework for Web Testing and Automation. It allows testing Chromium, Firefox and WebKit with a single API.
Pros of Playwright
- Multi-browser support (Chromium, Firefox, WebKit) out of the box
- Better handling of modern web features like shadow DOM and web components
- More powerful and flexible API for complex automation scenarios
Cons of Playwright
- Steeper learning curve due to more extensive API
- Slightly larger package size and longer installation time
- Less mature ecosystem compared to Puppeteer's established community
Code Comparison
Playwright:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await browser.close();
})();
Puppeteer:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await browser.close();
})();
Both Playwright and Puppeteer are powerful tools for browser automation and testing. Playwright offers more comprehensive browser support and advanced features, making it suitable for complex scenarios. Puppeteer, with its established ecosystem and simpler API, remains a solid choice for many projects. The code examples demonstrate the similarity in basic usage, with Playwright requiring an extra import step for specific browser selection.
Fast, easy and reliable testing for anything that runs in a browser.
Pros of Cypress
- Built-in automatic waiting and retry mechanisms, reducing flaky tests
- Rich, interactive test runner with time-travel debugging
- Easier setup and configuration, with less boilerplate code required
Cons of Cypress
- Limited to testing within a single browser tab
- Slower test execution compared to Puppeteer
- Less flexibility for complex scenarios and non-web testing
Code Comparison
Cypress example:
describe('Login', () => {
it('should log in successfully', () => {
cy.visit('/login')
cy.get('#username').type('user@example.com')
cy.get('#password').type('password123')
cy.get('button[type="submit"]').click()
cy.url().should('include', '/dashboard')
})
})
Puppeteer example:
describe('Login', () => {
it('should log in successfully', async () => {
await page.goto('/login')
await page.type('#username', 'user@example.com')
await page.type('#password', 'password123')
await page.click('button[type="submit"]')
await page.waitForNavigation()
expect(page.url()).toContain('/dashboard')
})
})
Both Cypress and Puppeteer are powerful tools for web automation and testing, but they have different strengths and use cases. Cypress excels in end-to-end testing with its user-friendly interface and built-in features, while Puppeteer offers more flexibility for complex scenarios and browser automation beyond testing.
A browser automation framework and ecosystem.
Pros of Selenium
- Supports multiple programming languages (Java, Python, C#, Ruby, etc.)
- Works with various browsers (Chrome, Firefox, Safari, Edge, etc.)
- Extensive community support and documentation
Cons of Selenium
- Slower execution compared to Puppeteer
- More complex setup and configuration
- Less efficient for handling modern web technologies (e.g., SPAs)
Code Comparison
Selenium (Python):
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example.com")
element = driver.find_element_by_id("my-element")
element.click()
driver.quit()
Puppeteer (JavaScript):
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await page.click('#my-element');
await browser.close();
})();
Both Selenium and Puppeteer are powerful tools for web automation and testing. Selenium offers broader language and browser support, making it versatile for diverse development environments. However, Puppeteer excels in performance and modern web handling, particularly for JavaScript-based applications. The choice between them depends on specific project requirements, team expertise, and the target application's technology stack.
Cross-platform automation framework for all kinds of apps, built on top of the W3C WebDriver protocol
Pros of Appium
- Supports multiple platforms (iOS, Android, Windows) for mobile and desktop testing
- Allows testing of native, hybrid, and web apps using a single API
- Supports multiple programming languages (Java, Python, Ruby, etc.)
Cons of Appium
- Slower test execution compared to Puppeteer
- More complex setup and configuration process
- Less suitable for web scraping and automation tasks
Code Comparison
Appium (Java):
AndroidDriver driver = new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), capabilities);
driver.findElement(By.id("com.example.app:id/button")).click();
String text = driver.findElement(By.id("com.example.app:id/result")).getText();
Puppeteer (JavaScript):
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await page.click('#button');
const text = await page.$eval('#result', el => el.textContent);
Puppeteer is primarily focused on web automation and testing for Chrome/Chromium, while Appium is designed for mobile app testing across multiple platforms. Puppeteer offers faster execution and simpler setup for web-based tasks, but Appium provides broader device and platform support for mobile testing scenarios.
Next-gen browser and mobile automation test framework for Node.js
Pros of WebdriverIO
- Supports multiple programming languages and browsers
- Integrates well with various testing frameworks and CI/CD tools
- Provides a more comprehensive set of automation capabilities for web applications
Cons of WebdriverIO
- Steeper learning curve compared to Puppeteer
- Slower execution speed for certain tasks
- Requires more setup and configuration
Code Comparison
WebdriverIO:
const { remote } = require('webdriverio');
(async () => {
const browser = await remote({
capabilities: { browserName: 'chrome' }
});
await browser.url('https://example.com');
const title = await browser.getTitle();
console.log('Title:', title);
await browser.deleteSession();
})();
Puppeteer:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const title = await page.title();
console.log('Title:', title);
await browser.close();
})();
Both WebdriverIO and Puppeteer are powerful tools for web automation and testing. WebdriverIO offers broader compatibility and integration options, making it suitable for complex, cross-browser testing scenarios. Puppeteer, on the other hand, provides a simpler API and faster execution for Chrome-specific tasks. The choice between the two depends on the specific requirements of your project, such as browser support, testing framework integration, and the complexity of your automation needs.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Puppeteer
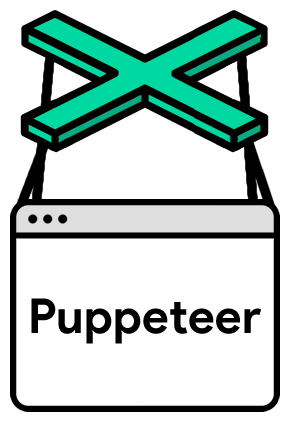
Puppeteer is a JavaScript library which provides a high-level API to control Chrome or Firefox over the DevTools Protocol or WebDriver BiDi. Puppeteer runs in the headless (no visible UI) by default
Get started | API | FAQ | Contributing | Troubleshooting
Installation
npm i puppeteer # Downloads compatible Chrome during installation.
npm i puppeteer-core # Alternatively, install as a library, without downloading Chrome.
Example
import puppeteer from 'puppeteer';
// Or import puppeteer from 'puppeteer-core';
// Launch the browser and open a new blank page
const browser = await puppeteer.launch();
const page = await browser.newPage();
// Navigate the page to a URL.
await page.goto('https://developer.chrome.com/');
// Set screen size.
await page.setViewport({width: 1080, height: 1024});
// Type into search box using accessible input name.
await page.locator('aria/Search').fill('automate beyond recorder');
// Wait and click on first result.
await page.locator('.devsite-result-item-link').click();
// Locate the full title with a unique string.
const textSelector = await page
.locator('text/Customize and automate')
.waitHandle();
const fullTitle = await textSelector?.evaluate(el => el.textContent);
// Print the full title.
console.log('The title of this blog post is "%s".', fullTitle);
await browser.close();
Top Related Projects
Playwright is a framework for Web Testing and Automation. It allows testing Chromium, Firefox and WebKit with a single API.
Fast, easy and reliable testing for anything that runs in a browser.
A browser automation framework and ecosystem.
Cross-platform automation framework for all kinds of apps, built on top of the W3C WebDriver protocol
Next-gen browser and mobile automation test framework for Node.js
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot