swagger-core
Examples and server integrations for generating the Swagger API Specification, which enables easy access to your REST API
Top Related Projects
The OpenAPI Specification Repository
OpenAPI Generator allows generation of API client libraries (SDK generation), server stubs, documentation and configuration automatically given an OpenAPI Spec (v2, v3)
📘 OpenAPI/Swagger-generated API Reference Documentation
Turn any OpenAPI2/3 and Postman Collection file into an API server with mocking, transformations and validations.
API Blueprint
Swagger 2.0 implementation for go
Quick Overview
Swagger Core is a Java-based library that helps developers design, build, document, and consume RESTful APIs. It provides tools for generating Swagger/OpenAPI specifications from Java code and annotations, making it easier to create and maintain API documentation.
Pros
- Simplifies API documentation process by generating Swagger/OpenAPI specs from code
- Integrates well with popular Java frameworks like Spring and JAX-RS
- Supports both Swagger 2.0 and OpenAPI 3.0 specifications
- Offers extensive customization options through annotations and configuration
Cons
- Learning curve for developers new to Swagger/OpenAPI concepts
- Can add complexity to projects, especially for smaller APIs
- May require additional setup and configuration in some environments
- Documentation generation might not capture all API nuances without manual intervention
Code Examples
- Adding Swagger annotations to a REST endpoint:
@Path("/users")
@Api(value = "User Management")
public class UserResource {
@GET
@Path("/{id}")
@ApiOperation(value = "Get user by ID", response = User.class)
@ApiResponses(value = {
@ApiResponse(code = 200, message = "Successfully retrieved user"),
@ApiResponse(code = 404, message = "User not found")
})
public Response getUserById(@PathParam("id") Long id) {
// Implementation
}
}
- Configuring Swagger in a Spring Boot application:
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.api"))
.paths(PathSelectors.any())
.build()
.apiInfo(apiInfo());
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("My API")
.description("API documentation using Swagger")
.version("1.0.0")
.build();
}
}
- Using Swagger annotations to describe a model:
@ApiModel(description = "User information")
public class User {
@ApiModelProperty(value = "Unique identifier of the user", example = "1")
private Long id;
@ApiModelProperty(value = "Name of the user", example = "John Doe")
private String name;
@ApiModelProperty(value = "Email address of the user", example = "john@example.com")
private String email;
// Getters and setters
}
Getting Started
To start using Swagger Core in your Java project:
- Add the following dependencies to your
pom.xml
(for Maven):
<dependency>
<groupId>io.swagger.core.v3</groupId>
<artifactId>swagger-jaxrs2</artifactId>
<version>2.2.8</version>
</dependency>
<dependency>
<groupId>io.swagger.core.v3</groupId>
<artifactId>swagger-jaxrs2-servlet-initializer</artifactId>
<version>2.2.8</version>
</dependency>
- Add Swagger annotations to your API endpoints and models.
- Configure Swagger in your application (e.g., using
SwaggerConfig
for Spring Boot). - Run your application and access the Swagger UI (typically at
/swagger-ui.html
) to view your API documentation.
Competitor Comparisons
The OpenAPI Specification Repository
Pros of OpenAPI-Specification
- More comprehensive and widely adopted industry standard
- Supports a broader range of API description features
- Actively maintained by a consortium of industry leaders
Cons of OpenAPI-Specification
- Steeper learning curve for beginners
- Less direct integration with Java ecosystem
Code Comparison
OpenAPI-Specification (YAML):
openapi: 3.0.0
info:
title: Sample API
version: 1.0.0
paths:
/users:
get:
summary: List users
responses:
'200':
description: Successful response
Swagger-Core (Java):
@Path("/users")
public class UserResource {
@GET
@ApiOperation(value = "List users")
@ApiResponses(value = {
@ApiResponse(code = 200, message = "Successful response")
})
public Response getUsers() {
// Implementation
}
}
Key Differences
- OpenAPI-Specification focuses on the API description format
- Swagger-Core provides tools for Java integration and code-first API development
- OpenAPI-Specification is language-agnostic, while Swagger-Core is Java-centric
Use Cases
- Choose OpenAPI-Specification for standardized API documentation across multiple languages
- Opt for Swagger-Core when working primarily with Java and seeking tight integration with the language ecosystem
Community and Ecosystem
- OpenAPI-Specification has a larger, more diverse community
- Swagger-Core benefits from Swagger's established tooling and integrations
OpenAPI Generator allows generation of API client libraries (SDK generation), server stubs, documentation and configuration automatically given an OpenAPI Spec (v2, v3)
Pros of openapi-generator
- Supports a wider range of programming languages and frameworks
- More frequent updates and active community contributions
- Offers more customization options for code generation
Cons of openapi-generator
- Steeper learning curve due to more complex configuration options
- May generate more boilerplate code in some cases
- Requires more setup time for initial configuration
Code Comparison
swagger-core (Java):
@Path("/users")
public class UserResource {
@GET
@Produces(MediaType.APPLICATION_JSON)
public Response getUsers() {
// Implementation
}
}
openapi-generator (Java):
@RestController
@RequestMapping("/users")
public class UserApiController implements UserApi {
@Override
public ResponseEntity<List<User>> getUsers() {
// Implementation
}
}
Both swagger-core and openapi-generator are popular tools for working with OpenAPI (formerly known as Swagger) specifications. swagger-core focuses on Java annotations for generating OpenAPI specifications, while openapi-generator is a more versatile tool for generating client SDKs, server stubs, and documentation across multiple programming languages.
swagger-core is generally easier to use for Java developers who want to generate OpenAPI specifications from their existing code. It integrates well with Java frameworks and requires less configuration.
openapi-generator, on the other hand, offers more flexibility and supports a broader range of use cases. It's particularly useful for generating client libraries and server stubs in various languages based on an existing OpenAPI specification.
📘 OpenAPI/Swagger-generated API Reference Documentation
Pros of Redoc
- Offers a more modern and visually appealing documentation interface
- Provides better support for customization and theming
- Generates a single-page application, making navigation smoother
Cons of Redoc
- Limited to OpenAPI/Swagger specification rendering
- May require additional setup for complex documentation needs
- Less extensive ecosystem compared to Swagger Core
Code Comparison
Redoc usage example:
<!DOCTYPE html>
<html>
<head>
<title>API Documentation</title>
<meta charset="utf-8"/>
<script src="https://cdn.jsdelivr.net/npm/redoc@next/bundles/redoc.standalone.js"></script>
</head>
<body>
<redoc spec-url="https://petstore.swagger.io/v2/swagger.json"></redoc>
</body>
</html>
Swagger Core usage example (Java):
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
}
Redoc focuses on rendering OpenAPI specifications, while Swagger Core provides a more comprehensive toolkit for generating, serving, and consuming OpenAPI-enabled APIs. Redoc excels in creating attractive, user-friendly documentation, whereas Swagger Core offers broader functionality for API development and integration.
Turn any OpenAPI2/3 and Postman Collection file into an API server with mocking, transformations and validations.
Pros of Prism
- Lightweight and fast API mock server and validator
- Supports multiple API description formats (OpenAPI, Postman Collections)
- Easy to use CLI and programmatic interface
Cons of Prism
- Limited to mocking and validation, lacks full API development lifecycle support
- Smaller community and ecosystem compared to Swagger Core
- Less extensive documentation and examples
Code Comparison
Prism (JavaScript):
const { createServer } = require('@stoplight/prism-http-server');
const server = createServer({
spec: './openapi.yaml',
port: 4010
});
server.listen();
Swagger Core (Java):
OpenAPI oas = new OpenAPIV3Parser().read("openapi.yaml");
SwaggerModule.setOpenAPI(oas);
Swagger swagger = new Swagger(oas);
String swaggerJson = Json.pretty(swagger);
Key Differences
- Prism focuses on API mocking and validation, while Swagger Core provides a more comprehensive API development toolkit
- Prism is primarily JavaScript-based, whereas Swagger Core is Java-centric
- Swagger Core has deeper integration with the broader Swagger ecosystem and tools
- Prism offers a simpler setup for quick API prototyping and testing
Use Cases
- Choose Prism for rapid API prototyping, mocking, and validation
- Opt for Swagger Core when building a full-fledged API development workflow, especially in Java environments
API Blueprint
Pros of API Blueprint
- Uses Markdown syntax, making it more human-readable and easier to write
- Supports more detailed documentation, including examples and descriptions
- Better suited for design-first API development
Cons of API Blueprint
- Less widely adopted compared to Swagger/OpenAPI
- Limited tooling support for code generation and validation
- Steeper learning curve for developers familiar with JSON/YAML-based formats
Code Comparison
API Blueprint:
# GET /users/{id}
+ Parameters
+ id: 1 (number, required) - User ID
+ Response 200 (application/json)
+ Body
{
"id": 1,
"name": "John Doe"
}
Swagger Core:
/users/{id}:
get:
parameters:
- name: id
in: path
required: true
schema:
type: integer
responses:
'200':
content:
application/json:
schema:
type: object
properties:
id:
type: integer
name:
type: string
Summary
API Blueprint offers a more human-readable format and is better suited for design-first approaches, while Swagger Core benefits from wider adoption and extensive tooling support. The choice between the two depends on project requirements, team preferences, and existing ecosystem integration.
Swagger 2.0 implementation for go
Pros of go-swagger
- Written in Go, offering better performance and concurrency support
- Generates server stubs and client SDK in Go, ideal for Go-based projects
- Supports OpenAPI 2.0 (Swagger) specification out of the box
Cons of go-swagger
- Limited to Go ecosystem, less versatile than swagger-core
- Smaller community and fewer resources compared to swagger-core
- May require more manual configuration for complex scenarios
Code Comparison
swagger-core (Java):
@Path("/users")
@Api(value = "/users", description = "User operations")
public class UserResource {
@GET
@ApiOperation(value = "Get all users", response = User.class, responseContainer = "List")
public Response getUsers() {
// Implementation
}
}
go-swagger (Go):
// swagger:route GET /users users listUsers
// Responses:
// 200: usersResponse
func (h *Handler) GetUsers(w http.ResponseWriter, r *http.Request) {
// Implementation
}
Summary
swagger-core is a more established and versatile tool supporting multiple languages, while go-swagger is tailored specifically for Go projects. go-swagger offers better performance and native Go integration, but has a smaller community and less flexibility across different programming languages. The choice between the two depends on the project's requirements and the development team's preferred language ecosystem.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Swagger Core 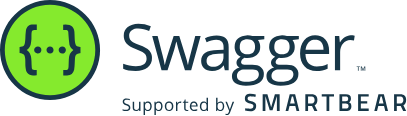
NOTE: If you're looking for Swagger Core 1.5.X and OpenAPI 2.0, please refer to 1.5 branch.
NOTE: Since version 2.1.7, Swagger Core also supports the Jakarta namespace. There are a parallel set of artifacts with the -jakarta
suffix, providing the same functionality as the unsuffixed (i.e.: javax
) artifacts.
Please see the Wiki for more details.
NOTE: Since version 2.2.0 Swagger Core supports OpenAPI 3.1; see this page for details
Swagger Core is a Java implementation of the OpenAPI Specification. Current version supports JAX-RS2 (javax
and jakarta
namespaces).
Get started with Swagger Core!
See the guide on getting started with Swagger Core to get started with adding Swagger to your API.
See the Wiki!
The github wiki contains documentation, samples, contributions, etc. Start there.
Compatibility
The OpenAPI Specification has undergone several revisions since initial creation in 2010. The Swagger Core project has the following compatibilities with the OpenAPI Specification:
Swagger core Version | Release Date | OpenAPI Spec compatibility | Notes | Status |
---|---|---|---|---|
2.2.34 (current stable) | 2025-06-20 | 3.x | tag v2.2.34 | Supported |
2.2.33 | 2025-06-12 | 3.x | tag v2.2.33 | Supported |
2.2.32 | 2025-05-14 | 3.x | tag v2.2.32 | Supported |
2.2.31 | 2025-05-13 | 3.x | tag v2.2.31 | Supported |
2.2.30 | 2025-04-07 | 3.x | tag v2.2.30 | Supported |
2.2.29 | 2025-03-10 | 3.x | tag v2.2.29 | Supported |
2.2.28 | 2025-01-16 | 3.x | tag v2.2.28 | Supported |
2.2.27 | 2024-12-11 | 3.x | tag v2.2.27 | Supported |
2.2.26 | 2024-11-18 | 3.x | tag v2.2.26 | Supported |
2.2.25 | 2024-10-02 | 3.x | tag v2.2.25 | Supported |
2.2.24 | 2024-09-23 | 3.x | tag v2.2.24 | Supported |
2.2.23 | 2024-08-28 | 3.x | tag v2.2.23 | Supported |
2.2.22 | 2024-05-15 | 3.x | tag v2.2.22 | Supported |
2.2.21 | 2024-03-20 | 3.x | tag v2.2.21 | Supported |
2.2.20 | 2023-12-19 | 3.x | tag v2.2.20 | Supported |
2.2.19 | 2023-11-10 | 3.x | tag v2.2.19 | Supported |
2.2.18 | 2023-10-25 | 3.x | tag v2.2.18 | Supported |
2.2.17 | 2023-10-12 | 3.x | tag v2.2.17 | Supported |
2.2.16 | 2023-09-18 | 3.x | tag v2.2.16 | Supported |
2.2.15 | 2023-07-08 | 3.x | tag v2.2.15 | Supported |
2.2.14 | 2023-06-26 | 3.x | tag v2.2.14 | Supported |
2.2.13 | 2023-06-24 | 3.x | tag v2.2.13 | Supported |
2.2.12 | 2023-06-13 | 3.x | tag v2.2.12 | Supported |
2.2.11 | 2023-06-01 | 3.x | tag v2.2.11 | Supported |
2.2.10 | 2023-05-15 | 3.x | tag v2.2.10 | Supported |
2.2.9 | 2023-03-20 | 3.x | tag v2.2.9 | Supported |
2.2.8 | 2023-01-06 | 3.x | tag v2.2.8 | Supported |
2.2.7 | 2022-11-15 | 3.0 | tag v2.2.7 | Supported |
2.2.6 | 2022-11-02 | 3.0 | tag v2.2.6 | Supported |
2.2.5 | 2022-11-02 | 3.0 | tag v2.2.5 | Supported |
2.2.4 | 2022-10-16 | 3.0 | tag v2.2.4 | Supported |
2.2.3 | 2022-09-27 | 3.0 | tag v2.2.3 | Supported |
2.2.2 | 2022-07-20 | 3.0 | tag v2.2.2 | Supported |
2.2.1 | 2022-06-15 | 3.0 | tag v2.2.1 | Supported |
2.2.0 | 2022-04-04 | 3.0 | tag v2.2.0 | Supported |
2.1.13 | 2022-02-07 | 3.0 | tag v2.1.13 | Supported |
2.1.12 | 2021-12-23 | 3.0 | tag v2.1.12 | Supported |
2.1.11 | 2021-09-29 | 3.0 | tag v2.1.11 | Supported |
2.1.10 | 2021-06-28 | 3.0 | tag v2.1.10 | Supported |
2.1.9 | 2021-04-20 | 3.0 | tag v2.1.9 | Supported |
2.1.8 | 2021-04-18 | 3.0 | tag v2.1.8 | Supported |
2.1.7 | 2021-02-18 | 3.0 | tag v2.1.7 | Supported |
2.1.6 | 2020-12-04 | 3.0 | tag v2.1.6 | Supported |
2.1.5 | 2020-10-01 | 3.0 | tag v2.1.5 | Supported |
2.1.4 | 2020-07-24 | 3.0 | tag v2.1.4 | Supported |
2.1.3 | 2020-06-27 | 3.0 | tag v2.1.3 | Supported |
2.1.2 | 2020-04-01 | 3.0 | tag v2.1.2 | Supported |
2.1.1 | 2020-01-02 | 3.0 | tag v2.1.1 | Supported |
2.1.0 | 2019-11-16 | 3.0 | tag v2.1.0 | Supported |
2.0.10 | 2019-10-11 | 3.0 | tag v2.0.10 | Supported |
2.0.9 | 2019-08-22 | 3.0 | tag v2.0.9 | Supported |
2.0.8 | 2019-04-24 | 3.0 | tag v2.0.8 | Supported |
2.0.7 | 2019-02-18 | 3.0 | tag v2.0.7 | Supported |
2.0.6 | 2018-11-27 | 3.0 | tag v2.0.6 | Supported |
2.0.5 | 2018-09-19 | 3.0 | tag v2.0.5 | Supported |
2.0.4 | 2018-09-05 | 3.0 | tag v2.0.4 | Supported |
2.0.3 | 2018-08-09 | 3.0 | tag v2.0.3 | Supported |
1.6.14 (current stable) | 2024-03-19 | 2.0 | tag v1.6.14 | Supported |
1.6.13 | 2024-01-26 | 2.0 | tag v1.6.13 | Supported |
1.6.12 | 2023-10-14 | 2.0 | tag v1.6.12 | Supported |
1.6.11 | 2023-05-15 | 2.0 | tag v1.6.11 | Supported |
1.6.10 | 2023-03-21 | 2.0 | tag v1.6.10 | Supported |
1.6.9 | 2022-11-15 | 2.0 | tag v1.6.9 | Supported |
1.6.8 | 2022-10-16 | 2.0 | tag v1.6.8 | Supported |
1.6.7 | 2022-09-27 | 2.0 | tag v1.6.7 | Supported |
1.6.6 | 2022-04-04 | 2.0 | tag v1.6.6 | Supported |
1.6.5 | 2022-02-07 | 2.0 | tag v1.6.5 | Supported |
1.6.4 | 2021-12-23 | 2.0 | tag v1.6.4 | Supported |
1.6.3 | 2021-09-29 | 2.0 | tag v1.6.3 | Supported |
1.6.2 | 2020-07-01 | 2.0 | tag v1.6.2 | Supported |
1.6.1 | 2020-04-01 | 2.0 | tag v1.6.1 | Supported |
1.6.0 | 2019-11-16 | 2.0 | tag v1.6.0 | Supported |
1.5.24 | 2019-10-11 | 2.0 | tag v1.5.24 | Supported |
1.5.23 | 2019-08-22 | 2.0 | tag v1.5.23 | Supported |
1.5.22 | 2019-02-18 | 2.0 | tag v1.5.22 | Supported |
1.5.21 | 2018-08-09 | 2.0 | tag v1.5.21 | Supported |
1.5.20 | 2018-05-23 | 2.0 | tag v1.5.20 | Supported |
2.0.2 | 2018-05-23 | 3.0 | tag v2.0.2 | Supported |
2.0.1 | 2018-04-16 | 3.0 | tag v2.0.1 | Supported |
1.5.19 | 2018-04-16 | 2.0 | tag v1.5.19 | Supported |
2.0.0 | 2018-03-20 | 3.0 | tag v2.0.0 | Supported |
2.0.0-rc4 | 2018-01-22 | 3.0 | tag v2.0.0-rc4 | Supported |
2.0.0-rc3 | 2017-11-21 | 3.0 | tag v2.0.0-rc3 | Supported |
2.0.0-rc2 | 2017-09-29 | 3.0 | tag v2.0.0-rc2 | Supported |
2.0.0-rc1 | 2017-08-17 | 3.0 | tag v2.0.0-rc1 | Supported |
1.5.18 | 2018-01-22 | 2.0 | tag v1.5.18 | Supported |
1.5.17 | 2017-11-21 | 2.0 | tag v1.5.17 | Supported |
1.5.16 | 2017-07-15 | 2.0 | tag v1.5.16 | Supported |
1.3.12 | 2014-12-23 | 1.2 | tag v1.3.12 | Supported |
1.2.4 | 2013-06-19 | 1.1 | tag swagger-project_2.10.0-1.2.4 | Deprecated |
1.0.0 | 2011-10-16 | 1.0 | tag v1.0 | Deprecated |
Change History
If you're interested in the change history of swagger and the Swagger Core framework, see here.
Prerequisites
You need the following installed and available in your $PATH:
- Java 11
- Apache maven 3.0.4 or greater
- Jackson 2.4.5 or greater
To build from source (currently 2.2.35-SNAPSHOT)
# first time building locally
mvn -N
Subsequent builds:
mvn install
This will build the modules.
Of course if you don't want to build locally you can grab artifacts from maven central:
https://repo1.maven.org/maven2/io/swagger/core/
Sample Apps
The samples have moved to a new repository and contain various integrations and configurations.
Security contact
Please disclose any security-related issues or vulnerabilities by emailing security@swagger.io, instead of using the public issue tracker.
Top Related Projects
The OpenAPI Specification Repository
OpenAPI Generator allows generation of API client libraries (SDK generation), server stubs, documentation and configuration automatically given an OpenAPI Spec (v2, v3)
📘 OpenAPI/Swagger-generated API Reference Documentation
Turn any OpenAPI2/3 and Postman Collection file into an API server with mocking, transformations and validations.
API Blueprint
Swagger 2.0 implementation for go
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot