Top Related Projects
The next open source file uploader for web browsers :dog:
File Upload widget with multiple file selection, drag&drop support, progress bar, validation and preview images, audio and video for jQuery. Supports cross-domain, chunked and resumable file uploads. Works with any server-side platform (Google App Engine, PHP, Python, Ruby on Rails, Java, etc.) that supports standard HTML form file uploads.
A JavaScript library for providing multiple simultaneous, stable, fault-tolerant and resumable/restartable uploads via the HTML5 File API.
A JavaScript library providing multiple simultaneous, stable, fault-tolerant and resumable/restartable file uploads via the HTML5 File API.
Multiple file upload plugin with image previews, drag and drop, progress bars. S3 and Azure support, image scaling, form support, chunking, resume, pause, and tons of other features.
Dropzone is an easy to use drag'n'drop library. It supports image previews and shows nice progress bars.
Quick Overview
tus-js-client is a JavaScript client for the tus resumable upload protocol. It allows for reliable and resumable file uploads in web browsers and Node.js environments, supporting large file uploads that can be paused and resumed even after browser crashes or network interruptions.
Pros
- Supports resumable uploads, allowing for efficient handling of large files
- Works in both browser and Node.js environments
- Implements the tus protocol, ensuring compatibility with tus servers
- Provides progress tracking and upload control (pause, resume, abort)
Cons
- Requires a tus-compatible server for full functionality
- May have a steeper learning curve compared to simple file upload solutions
- Limited to HTTP(S) protocol, not suitable for other transfer protocols
- Dependency on the tus protocol may limit flexibility in some use cases
Code Examples
Creating a new upload:
const file = new File(["Hello World!"], "hello.txt", { type: "text/plain" });
const upload = new tus.Upload(file, {
endpoint: "https://tusd.tusdemo.net/files/",
retryDelays: [0, 3000, 5000, 10000, 20000],
metadata: {
filename: file.name,
filetype: file.type
},
onError: function(error) {
console.log("Failed because: " + error)
},
onProgress: function(bytesUploaded, bytesTotal) {
const percentage = ((bytesUploaded / bytesTotal) * 100).toFixed(2)
console.log(bytesUploaded, bytesTotal, percentage + "%")
},
onSuccess: function() {
console.log("Download %s from %s", upload.file.name, upload.url)
}
})
// Start the upload
upload.start()
Resuming an upload:
const previousUploads = await tus.Upload.findPreviousUploads()
if (previousUploads.length) {
upload.resumeFromPreviousUpload(previousUploads[0])
}
Pausing and resuming an upload:
// Pause the upload
upload.abort()
// Resume the upload
upload.start()
Getting Started
-
Install the library:
npm install tus-js-client
-
Import the library:
import * as tus from "tus-js-client"
-
Create an upload instance and start the upload:
const upload = new tus.Upload(file, { endpoint: "https://your-tus-endpoint.com/files/", onError: function(error) { console.log("An error occurred: " + error.message) }, onProgress: function(bytesUploaded, bytesTotal) { const percentage = ((bytesUploaded / bytesTotal) * 100).toFixed(2) console.log(percentage + "%") }, onSuccess: function() { console.log("Upload completed successfully!") } }) upload.start()
Competitor Comparisons
The next open source file uploader for web browsers :dog:
Pros of Uppy
- More comprehensive file upload solution with a rich UI and plugin ecosystem
- Supports various upload sources (local, remote URLs, webcam, etc.)
- Integrates with multiple backend services and storage providers
Cons of Uppy
- Larger bundle size due to its extensive feature set
- Steeper learning curve for basic implementations
- May be overkill for simple upload requirements
Code Comparison
Uppy (basic setup):
const Uppy = require('@uppy/core')
const XHRUpload = require('@uppy/xhr-upload')
const uppy = new Uppy()
.use(XHRUpload, { endpoint: 'https://example.com/upload' })
uppy.on('complete', (result) => {
console.log('Upload complete! We've uploaded these files:', result.successful)
})
tus-js-client (basic setup):
var upload = new tus.Upload(file, {
endpoint: "https://example.com/files/",
retryDelays: [0, 3000, 5000, 10000, 20000],
metadata: {
filename: file.name,
filetype: file.type
},
onError: function(error) {
console.log("Failed because: " + error)
},
onProgress: function(bytesUploaded, bytesTotal) {
var percentage = (bytesUploaded / bytesTotal * 100).toFixed(2)
console.log(bytesUploaded, bytesTotal, percentage + "%")
},
onSuccess: function() {
console.log("Download %s from %s", upload.file.name, upload.url)
}
})
upload.start()
Both libraries offer robust file upload capabilities, but Uppy provides a more feature-rich solution with a polished UI, while tus-js-client focuses specifically on resumable uploads using the tus protocol.
File Upload widget with multiple file selection, drag&drop support, progress bar, validation and preview images, audio and video for jQuery. Supports cross-domain, chunked and resumable file uploads. Works with any server-side platform (Google App Engine, PHP, Python, Ruby on Rails, Java, etc.) that supports standard HTML form file uploads.
Pros of jQuery-File-Upload
- Extensive browser support, including older versions
- Rich UI components and customizable templates
- Integrated image resizing and processing capabilities
Cons of jQuery-File-Upload
- Dependency on jQuery library
- Larger codebase and potentially heavier resource usage
- Less focus on resumable uploads compared to tus-js-client
Code Comparison
tus-js-client:
var upload = new tus.Upload(file, {
endpoint: "https://tusd.tusdemo.net/files/",
retryDelays: [0, 3000, 5000, 10000, 20000],
metadata: {
filename: file.name,
filetype: file.type
},
onError: function(error) {
console.log("Failed because: " + error)
},
onProgress: function(bytesUploaded, bytesTotal) {
var percentage = (bytesUploaded / bytesTotal * 100).toFixed(2)
console.log(bytesUploaded, bytesTotal, percentage + "%")
},
onSuccess: function() {
console.log("Download %s from %s", upload.file.name, upload.url)
}
})
jQuery-File-Upload:
$('#fileupload').fileupload({
url: 'server/php/',
dataType: 'json',
done: function (e, data) {
$.each(data.result.files, function (index, file) {
$('<p/>').text(file.name).appendTo('#files');
});
},
progressall: function (e, data) {
var progress = parseInt(data.loaded / data.total * 100, 10);
$('#progress .progress-bar').css(
'width',
progress + '%'
);
}
});
A JavaScript library for providing multiple simultaneous, stable, fault-tolerant and resumable/restartable uploads via the HTML5 File API.
Pros of resumable.js
- More flexible and customizable, allowing for greater control over the upload process
- Supports a wider range of server implementations and protocols
- Has been around longer and may have a more mature ecosystem
Cons of resumable.js
- Less standardized approach compared to the TUS protocol
- May require more server-side configuration and implementation
- Not as actively maintained as tus-js-client
Code Comparison
tus-js-client:
const upload = new tus.Upload(file, {
endpoint: "https://tusd.tusdemo.net/files/",
retryDelays: [0, 3000, 5000, 10000, 20000],
metadata: {
filename: file.name,
filetype: file.type
},
onError: function(error) {
console.log("Failed because: " + error)
},
onProgress: function(bytesUploaded, bytesTotal) {
var percentage = (bytesUploaded / bytesTotal * 100).toFixed(2)
console.log(bytesUploaded, bytesTotal, percentage + "%")
},
onSuccess: function() {
console.log("Download %s from %s", upload.file.name, upload.url)
}
})
upload.start()
resumable.js:
var r = new Resumable({
target: '/upload',
chunkSize: 1*1024*1024,
simultaneousUploads: 4,
testChunks: true,
throttleProgressCallbacks: 1
});
r.assignBrowse(document.getElementById('browseButton'));
r.on('fileAdded', function(file) {
r.upload();
});
r.on('fileSuccess', function(file, message) {
console.log(file.fileName + ' successfully uploaded');
});
r.on('fileError', function(file, message) {
console.log(file.fileName + ' failed to upload: ' + message);
});
A JavaScript library providing multiple simultaneous, stable, fault-tolerant and resumable/restartable file uploads via the HTML5 File API.
Pros of flow.js
- Supports a wider range of browsers, including older versions
- Offers more customization options for chunk size and concurrent uploads
- Provides built-in pause and resume functionality
Cons of flow.js
- Less focused on the tus protocol, which may limit compatibility with some servers
- Requires more manual configuration for optimal performance
- Has fewer built-in security features compared to tus-js-client
Code Comparison
tus-js-client:
var upload = new tus.Upload(file, {
endpoint: "https://tusd.tusdemo.net/files/",
retryDelays: [0, 3000, 5000, 10000, 20000],
metadata: {
filename: file.name,
filetype: file.type
},
onError: function(error) {
console.log("Failed because: " + error)
},
onProgress: function(bytesUploaded, bytesTotal) {
var percentage = (bytesUploaded / bytesTotal * 100).toFixed(2)
console.log(bytesUploaded, bytesTotal, percentage + "%")
},
onSuccess: function() {
console.log("Download %s from %s", upload.file.name, upload.url)
}
})
flow.js:
var flow = new Flow({
target: '/api/photo/redeem-upload-token',
query: { upload_token: 'my_token' }
});
flow.assignBrowse(document.getElementById('browseButton'));
flow.on('fileAdded', function(file, event){
console.log(file, event);
});
flow.on('fileSuccess', function(file, message){
console.log(file, message);
});
flow.on('fileError', function(file, message){
console.log(file, message);
});
Multiple file upload plugin with image previews, drag and drop, progress bars. S3 and Azure support, image scaling, form support, chunking, resume, pause, and tons of other features.
Pros of Fine-Uploader
- More comprehensive feature set, including drag-and-drop, image previews, and progress bars
- Supports multiple upload protocols (traditional multipart, chunked, and resumable)
- Extensive documentation and examples for various use cases
Cons of Fine-Uploader
- Larger file size and potentially more complex setup compared to tus-js-client
- Less focused on a single, standardized protocol (tus)
- May have a steeper learning curve for simple use cases
Code Comparison
Fine-Uploader:
var uploader = new qq.FineUploader({
element: document.getElementById('uploader'),
request: {
endpoint: '/uploads'
}
});
tus-js-client:
var upload = new tus.Upload(file, {
endpoint: '/uploads',
onProgress: function(bytesUploaded, bytesTotal) {
var percentage = (bytesUploaded / bytesTotal * 100).toFixed(2);
console.log(percentage + '%');
}
});
Summary
Fine-Uploader offers a more feature-rich solution with support for multiple upload protocols, making it suitable for complex use cases. However, this comes at the cost of a larger file size and potentially more complex setup. tus-js-client, on the other hand, focuses specifically on the tus resumable upload protocol, providing a simpler and more lightweight solution for projects that prioritize resumable uploads.
Dropzone is an easy to use drag'n'drop library. It supports image previews and shows nice progress bars.
Pros of Dropzone
- More comprehensive file upload solution with a user-friendly drag-and-drop interface
- Extensive customization options for appearance and behavior
- Broader browser support, including older versions
Cons of Dropzone
- Larger file size and potentially heavier resource usage
- Less specialized for handling large file uploads or resumable uploads
- May require more setup for advanced features
Code Comparison
tus-js-client:
var upload = new tus.Upload(file, {
endpoint: "https://tusd.tusdemo.net/files/",
retryDelays: [0, 3000, 5000, 10000, 20000],
metadata: {
filename: file.name,
filetype: file.type
},
onError: function(error) {
console.log("Failed because: " + error)
},
onProgress: function(bytesUploaded, bytesTotal) {
var percentage = (bytesUploaded / bytesTotal * 100).toFixed(2)
console.log(bytesUploaded, bytesTotal, percentage + "%")
},
onSuccess: function() {
console.log("Download %s from %s", upload.file.name, upload.url)
}
})
Dropzone:
Dropzone.options.myDropzone = {
url: "/file/post",
paramName: "file",
maxFilesize: 2,
acceptedFiles: "image/*",
addRemoveLinks: true,
dictDefaultMessage: "Drop files here to upload",
init: function() {
this.on("success", function(file, response) {
console.log("File uploaded successfully");
});
}
};
Both libraries offer file upload functionality, but tus-js-client focuses on resumable uploads with a simpler API, while Dropzone provides a more feature-rich and customizable drag-and-drop interface for general file uploads.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
tus-js-client
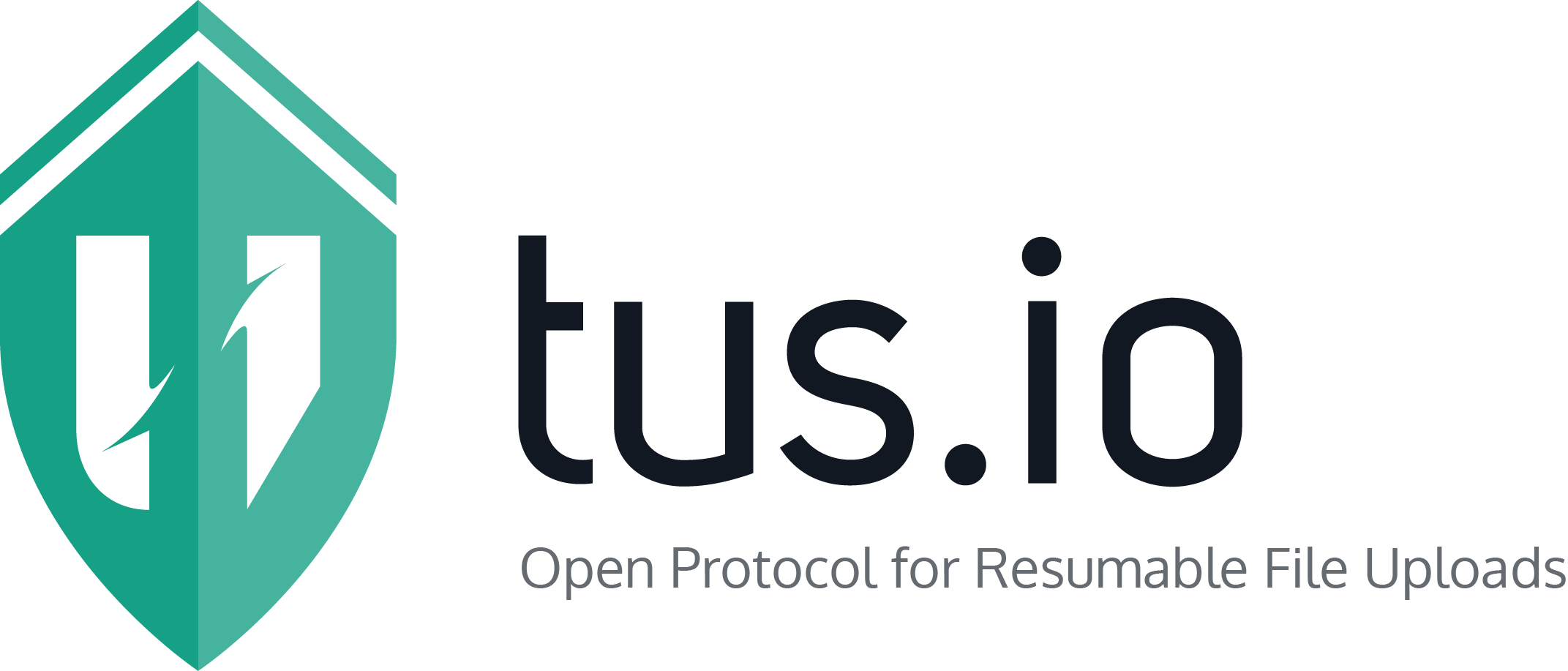
tus is a protocol based on HTTP for resumable file uploads. Resumable means that an upload can be interrupted at any moment and can be resumed without re-uploading the previous data again. An interruption may happen willingly, if the user wants to pause, or by accident in case of an network issue or server outage.
tus-js-client is a pure JavaScript client for the tus resumable upload protocol and can be used inside browsers, Node.js, React Native and Apache Cordova applications.
Protocol version: 1.0.0
This branch contains tus-js-client v4. If you are looking for the previous major release, after which breaking changes have been introduced, please look at the v3.1.3 tag.
Example
input.addEventListener('change', function (e) {
// Get the selected file from the input element
var file = e.target.files[0]
// Create a new tus upload
var upload = new tus.Upload(file, {
endpoint: 'http://localhost:1080/files/',
retryDelays: [0, 3000, 5000, 10000, 20000],
metadata: {
filename: file.name,
filetype: file.type,
},
onError: function (error) {
console.log('Failed because: ' + error)
},
onProgress: function (bytesUploaded, bytesTotal) {
var percentage = ((bytesUploaded / bytesTotal) * 100).toFixed(2)
console.log(bytesUploaded, bytesTotal, percentage + '%')
},
onSuccess: function () {
console.log('Download %s from %s', upload.file.name, upload.url)
},
})
// Check if there are any previous uploads to continue.
upload.findPreviousUploads().then(function (previousUploads) {
// Found previous uploads so we select the first one.
if (previousUploads.length) {
upload.resumeFromPreviousUpload(previousUploads[0])
}
// Start the upload
upload.start()
})
})
Documentation
- Installation & Requirements
- Usage & Examples
- API Reference
- Contribution Guidelines
- FAQ & Common issues
Build status
License
This project is licensed under the MIT license, see LICENSE
.
Top Related Projects
The next open source file uploader for web browsers :dog:
File Upload widget with multiple file selection, drag&drop support, progress bar, validation and preview images, audio and video for jQuery. Supports cross-domain, chunked and resumable file uploads. Works with any server-side platform (Google App Engine, PHP, Python, Ruby on Rails, Java, etc.) that supports standard HTML form file uploads.
A JavaScript library for providing multiple simultaneous, stable, fault-tolerant and resumable/restartable uploads via the HTML5 File API.
A JavaScript library providing multiple simultaneous, stable, fault-tolerant and resumable/restartable file uploads via the HTML5 File API.
Multiple file upload plugin with image previews, drag and drop, progress bars. S3 and Azure support, image scaling, form support, chunking, resume, pause, and tons of other features.
Dropzone is an easy to use drag'n'drop library. It supports image previews and shows nice progress bars.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot