Top Related Projects
Laravel is a web application framework with expressive, elegant syntax. We’ve already laid the foundation for your next big idea — freeing you to create without sweating the small things.
Fast, unopinionated, minimalist web framework for node.
Spring Boot helps you to create Spring-powered, production-grade applications and services with absolute minimum fuss.
The Web framework for perfectionists with deadlines.
Ruby on Rails
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript 🚀
Quick Overview
Vapor is a web framework for Swift, designed to build scalable, high-performance web applications and APIs. It leverages Swift's powerful features to provide a clean, expressive, and efficient development experience for server-side Swift programming.
Pros
- Fast performance due to Swift's compiled nature and Vapor's optimized architecture
- Strong type safety and compile-time checks, reducing runtime errors
- Extensive ecosystem with many packages and integrations available
- Seamless integration with Apple's ecosystem and tools
Cons
- Smaller community compared to more established web frameworks
- Limited hosting options, as it requires Swift-compatible environments
- Steeper learning curve for developers not familiar with Swift
- Less mature than some other web frameworks, potentially leading to fewer resources and examples
Code Examples
- Creating a basic route:
import Vapor
let app = try Application(.detect())
defer { app.shutdown() }
app.get("hello") { req -> String in
return "Hello, world!"
}
try app.run()
- Handling JSON requests and responses:
import Vapor
struct User: Content {
let name: String
let age: Int
}
app.post("users") { req -> User in
let user = try req.content.decode(User.self)
// Save user to database
return user
}
- Using middleware:
import Vapor
struct LogMiddleware: Middleware {
func respond(to request: Request, chainingTo next: Responder) -> EventLoopFuture<Response> {
print("Received request: \(request.url)")
return next.respond(to: request)
}
}
app.middleware.use(LogMiddleware())
Getting Started
To get started with Vapor, follow these steps:
- Install Swift on your system (if not already installed)
- Install Vapor Toolbox:
brew install vapor
- Create a new Vapor project:
vapor new MyProject cd MyProject
- Build and run the project:
swift run
Your Vapor application will now be running at http://localhost:8080
.
Competitor Comparisons
Laravel is a web application framework with expressive, elegant syntax. We’ve already laid the foundation for your next big idea — freeing you to create without sweating the small things.
Pros of Laravel
- Extensive ecosystem with a wide range of packages and tools
- Robust documentation and large community support
- Built-in features like Eloquent ORM and Artisan CLI
Cons of Laravel
- Heavier framework with more overhead compared to Vapor
- Steeper learning curve for beginners
- Slower performance in some scenarios due to its full-stack nature
Code Comparison
Laravel (PHP):
Route::get('/hello', function () {
return view('hello');
});
Vapor (Swift):
app.get("hello") { req in
return "Hello, world!"
}
Laravel uses a more traditional PHP syntax with a routing closure, while Vapor employs Swift's concise syntax for route handling. Laravel typically returns views, whereas Vapor can directly return strings or other types.
Laravel is a full-featured PHP framework with a comprehensive set of tools and libraries, making it suitable for large-scale applications. Vapor, on the other hand, is a lightweight Swift web framework focused on performance and simplicity, ideal for building fast and efficient web services.
While Laravel offers a complete solution out of the box, Vapor provides more flexibility and control over the application structure. Laravel's extensive documentation and community support make it easier for beginners to get started, but Vapor's simplicity can be advantageous for developers familiar with Swift and seeking high-performance solutions.
Fast, unopinionated, minimalist web framework for node.
Pros of Express
- Larger ecosystem and community support
- More mature and battle-tested in production environments
- Extensive middleware library for various functionalities
Cons of Express
- JavaScript-based, which may be less performant than Swift
- Callback-heavy architecture can lead to "callback hell"
- Less opinionated, requiring more setup for larger applications
Code Comparison
Express:
const express = require('express')
const app = express()
app.get('/', (req, res) => {
res.send('Hello World!')
})
Vapor:
import Vapor
let app = try Application(.detect())
defer { app.shutdown() }
app.get { req in
return "Hello World!"
}
Both frameworks offer simple routing and request handling, but Vapor's syntax is more concise and leverages Swift's type safety. Express uses JavaScript's callback style, while Vapor utilizes Swift's async/await pattern for cleaner asynchronous code.
Express is widely adopted and has a vast ecosystem, making it easier to find solutions and third-party packages. However, Vapor's use of Swift provides better performance and type safety, which can lead to fewer runtime errors and easier maintenance for larger projects.
Express is more flexible and less opinionated, allowing developers to structure their applications as they see fit. Vapor, on the other hand, provides a more structured approach, which can be beneficial for maintaining consistency in larger teams or projects.
Spring Boot helps you to create Spring-powered, production-grade applications and services with absolute minimum fuss.
Pros of Spring Boot
- Extensive ecosystem with a wide range of libraries and integrations
- Robust documentation and large community support
- Production-ready features like health checks and metrics out of the box
Cons of Spring Boot
- Steeper learning curve due to its comprehensive nature
- Heavier resource consumption, especially for smaller applications
- Can be overly complex for simple projects
Code Comparison
Spring Boot:
@RestController
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
Vapor:
struct HelloController: RouteCollection {
func boot(routes: RoutesBuilder) throws {
routes.get("hello") { req in
return "Hello, World!"
}
}
}
Both frameworks offer simple ways to create routes and handle HTTP requests, but Spring Boot uses Java annotations while Vapor uses Swift's more functional approach. Spring Boot's syntax is more declarative, while Vapor's is more concise and aligns closely with Swift's language features.
Spring Boot is generally more suitable for large-scale enterprise applications, offering a comprehensive set of tools and integrations. Vapor, on the other hand, excels in building lightweight, high-performance web applications and APIs, particularly for Swift developers looking for a server-side solution in their preferred language.
The Web framework for perfectionists with deadlines.
Pros of Django
- Extensive ecosystem with a wide range of built-in features and third-party packages
- Robust ORM with powerful database abstraction and migration tools
- Comprehensive documentation and large, active community support
Cons of Django
- Steeper learning curve due to its monolithic nature and "batteries-included" approach
- Can be slower in performance compared to more lightweight frameworks
- Less flexibility in choosing components, as many are tightly integrated
Code Comparison
Django (Python):
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.CharField(max_length=50)
published_date = models.DateField()
Vapor (Swift):
import Fluent
import Vapor
final class Book: Model, Content {
static let schema = "books"
@ID(key: .id) var id: UUID?
@Field(key: "title") var title: String
@Field(key: "author") var author: String
@Field(key: "published_date") var publishedDate: Date
}
Both frameworks provide elegant ways to define models, but Django's ORM is more feature-rich out of the box, while Vapor's approach is more lightweight and Swift-native.
Ruby on Rails
Pros of Rails
- Mature ecosystem with extensive libraries and gems
- Strong convention over configuration principles
- Large and active community for support and resources
Cons of Rails
- Slower performance compared to Vapor
- Steeper learning curve for beginners
- Higher memory consumption
Code Comparison
Rails (Ruby):
class UsersController < ApplicationController
def index
@users = User.all
render json: @users
end
end
Vapor (Swift):
struct UsersController: RouteCollection {
func boot(routes: RoutesBuilder) throws {
routes.get("users") { req in
try await User.query(on: req.db).all()
}
}
}
Key Differences
- Rails uses Ruby, while Vapor uses Swift
- Vapor's syntax is more concise and type-safe
- Rails follows a more traditional MVC structure
- Vapor leverages Swift's async/await for handling asynchronous operations
Use Cases
- Rails: Large-scale web applications, rapid prototyping
- Vapor: High-performance APIs, microservices, iOS/macOS backend services
Both frameworks offer robust solutions for web development, with Rails excelling in rapid development and Vapor focusing on performance and Swift integration.
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript 🚀
Pros of Nest
- Larger community and ecosystem, with more third-party packages and resources
- Built-in support for TypeScript, offering better type safety and tooling
- Modular architecture with dependency injection, promoting scalable and maintainable code
Cons of Nest
- Steeper learning curve due to its opinionated structure and decorators
- Potentially slower performance compared to Vapor's lightweight design
- Heavier memory footprint, which may impact resource usage in some scenarios
Code Comparison
Nest (TypeScript):
@Controller('cats')
export class CatsController {
@Get()
findAll(): string {
return 'This action returns all cats';
}
}
Vapor (Swift):
struct CatsController: RouteCollection {
func boot(routes: RoutesBuilder) throws {
routes.get("cats") { req in
return "This action returns all cats"
}
}
}
Both frameworks offer clean and expressive syntax for defining routes and controllers. Nest uses decorators and classes, while Vapor employs structs and protocol conformance. Nest's approach may be more familiar to developers coming from other object-oriented languages, while Vapor's style aligns closely with Swift's idiomatic patterns.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Vapor is an HTTP web framework for Swift. It provides a beautifully expressive and easy-to-use foundation for your next website, API, or cloud project.
Take a look at some of the awesome stuff created with Vapor.
ð§ Community
Join the welcoming community of fellow Vapor developers on Discord.
ð Contributing
To contribute a feature or idea to Vapor, create an issue explaining your idea or bring it up on Discord.
If you find a bug, please create an issue.
If you find a security vulnerability, please contact security@vapor.codes as soon as possible.
ð Sponsors
Support Vapor's development by becoming a sponsor.
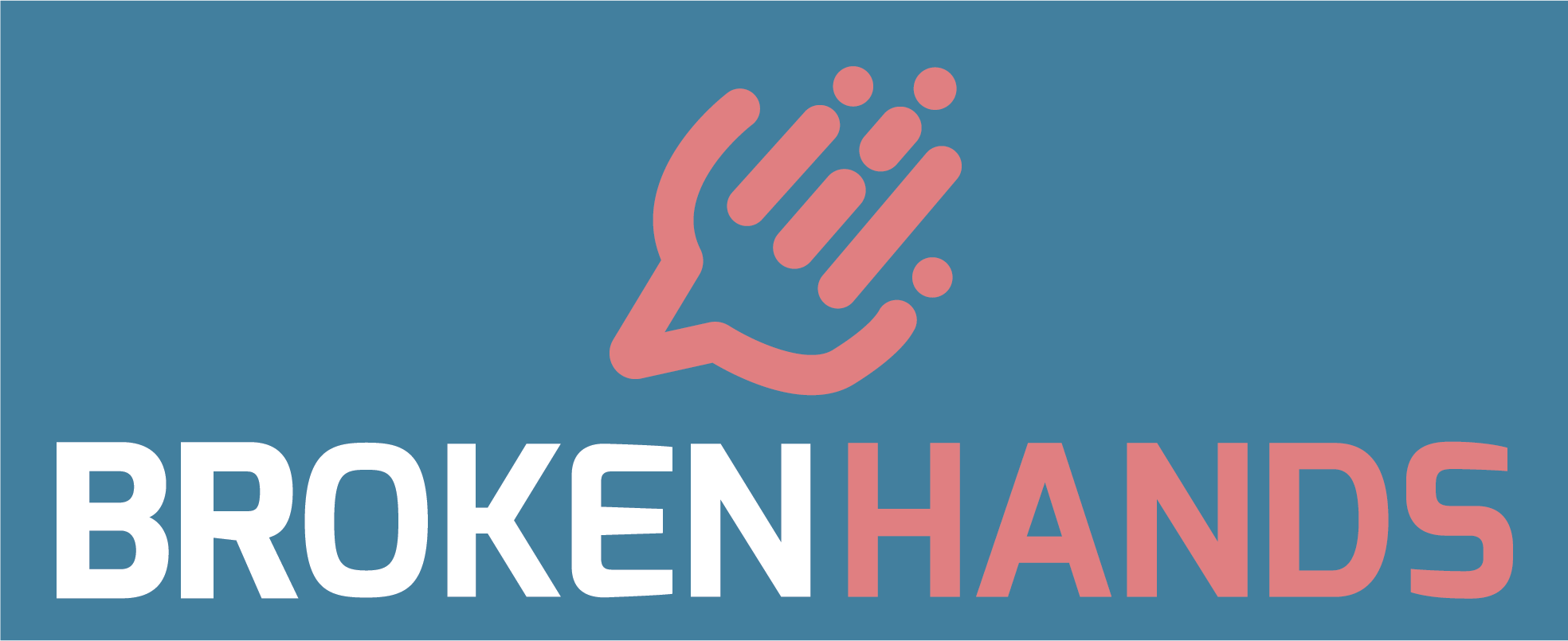
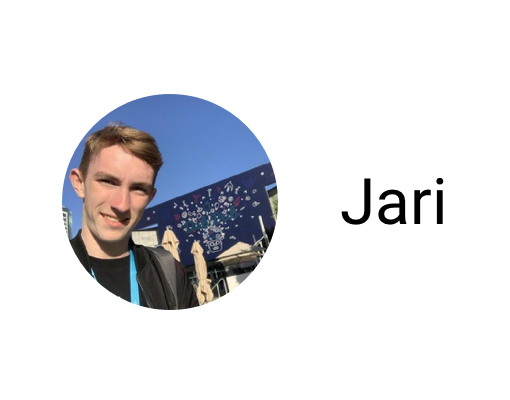
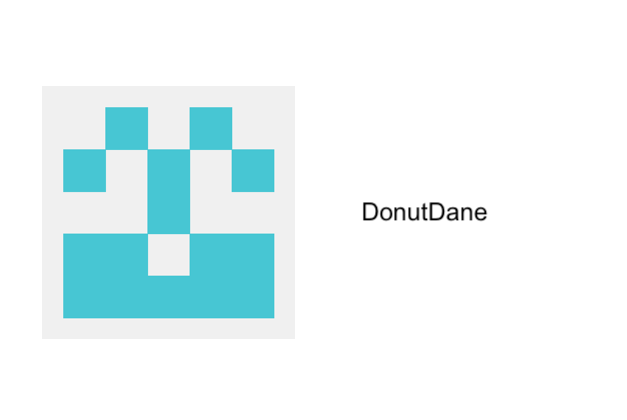

ð Backers
Support Vapor's development by becoming a backer.



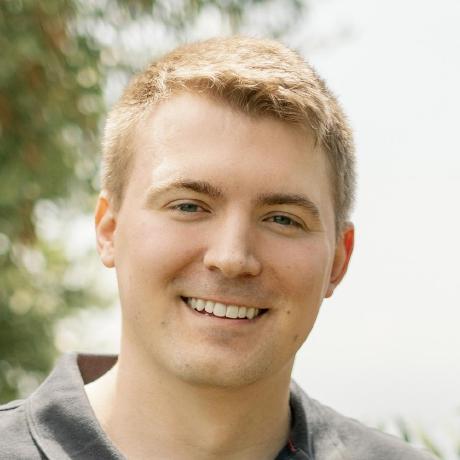
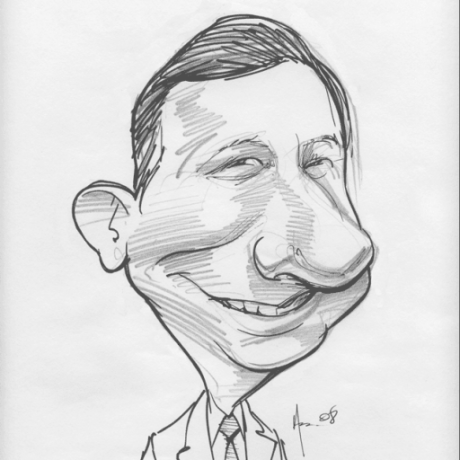


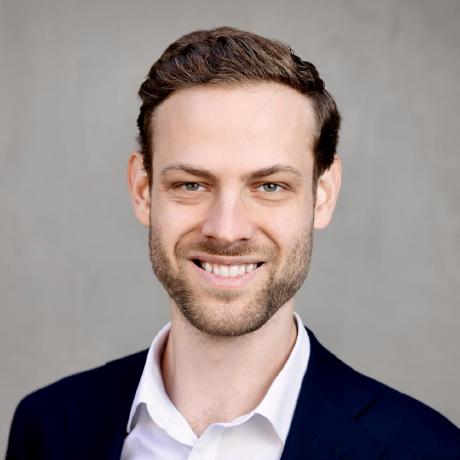

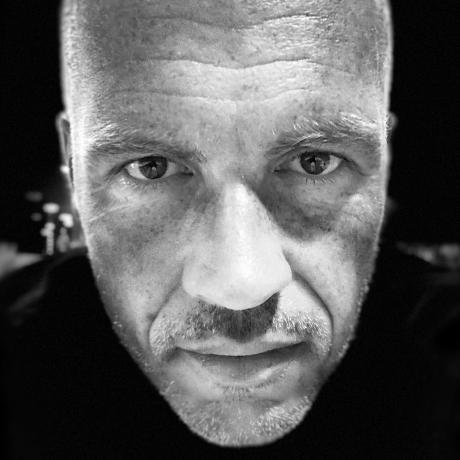


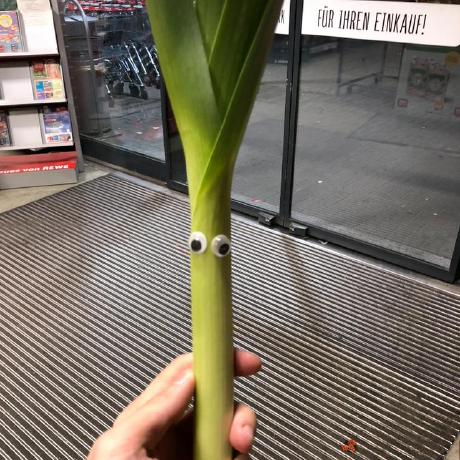

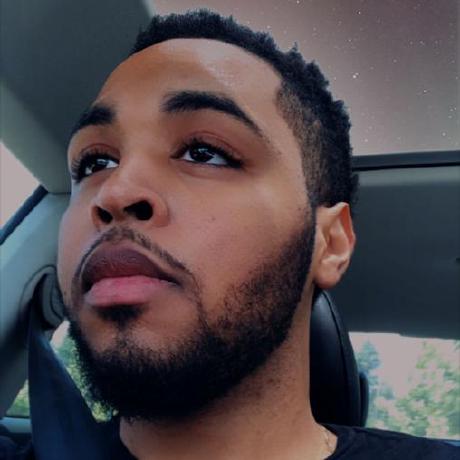

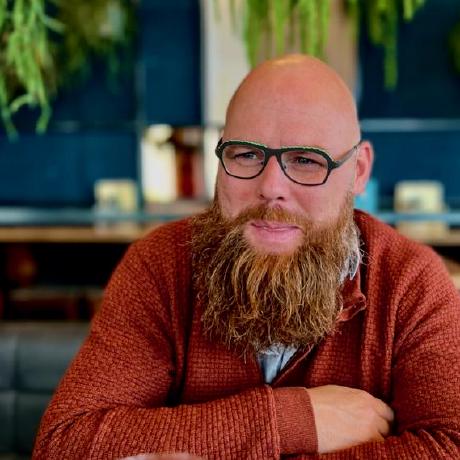
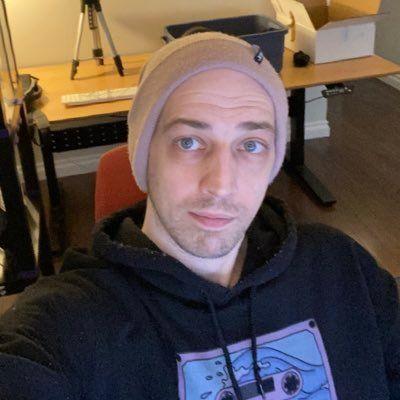

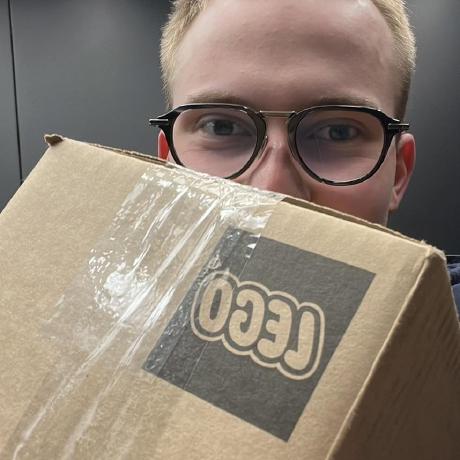
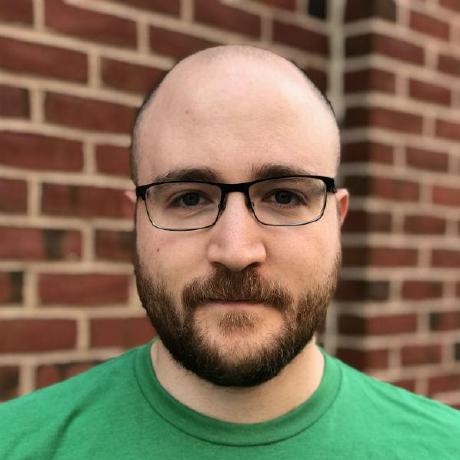


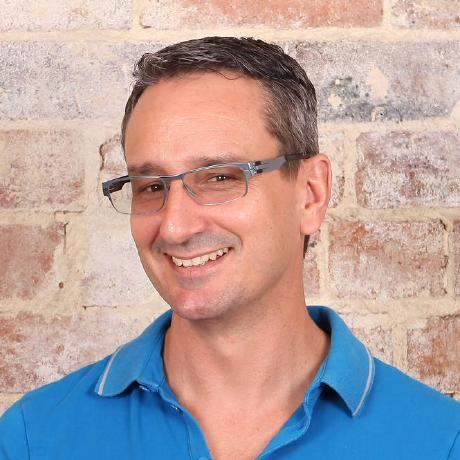
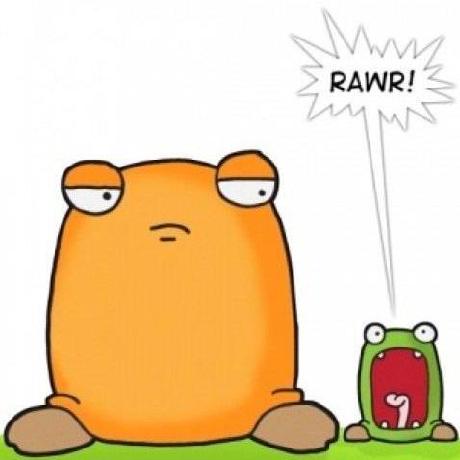
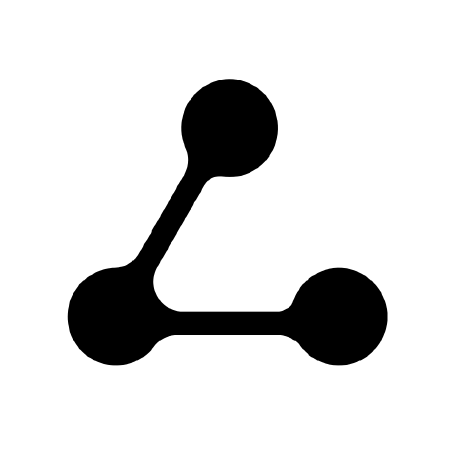
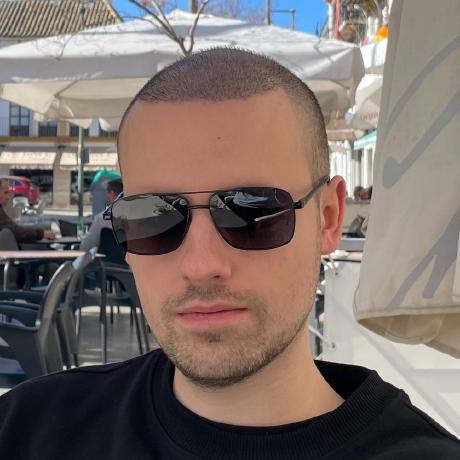


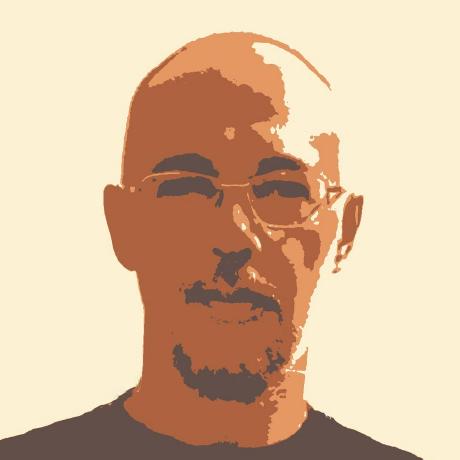
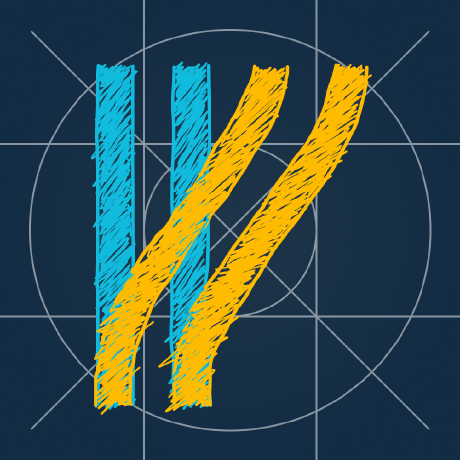
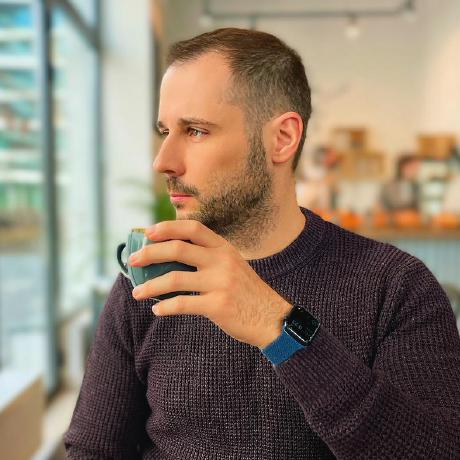
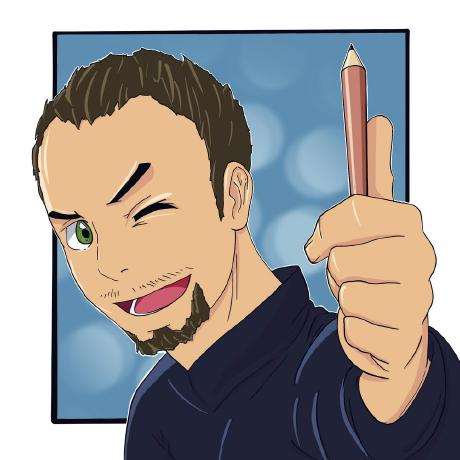

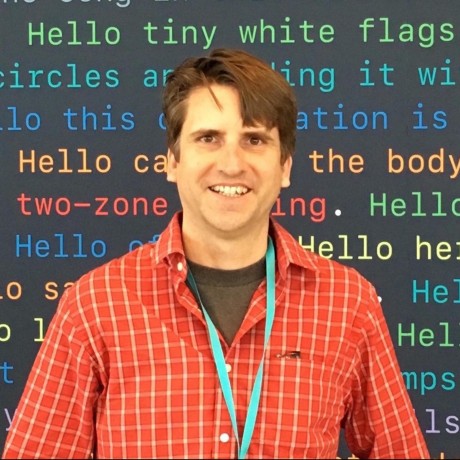

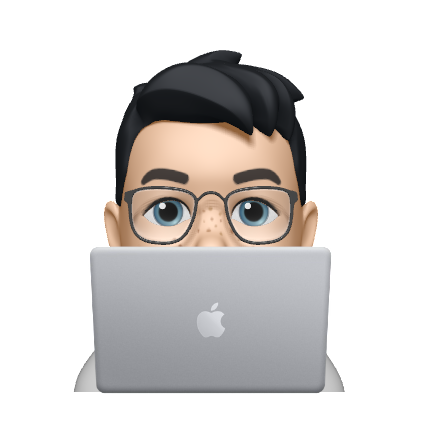
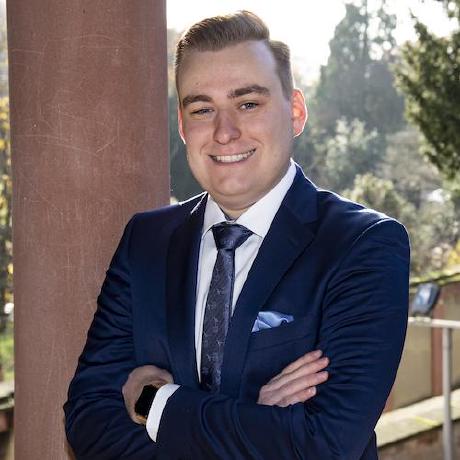

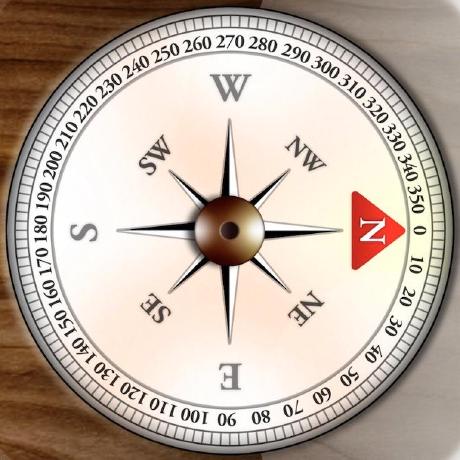

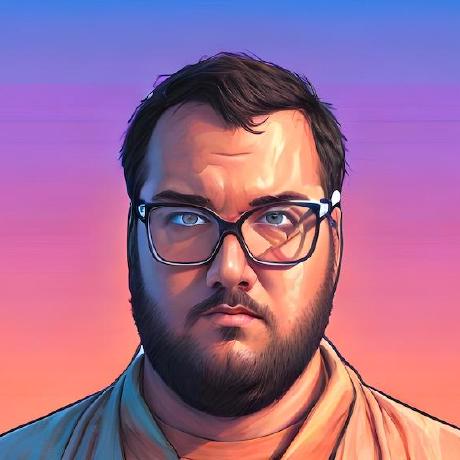
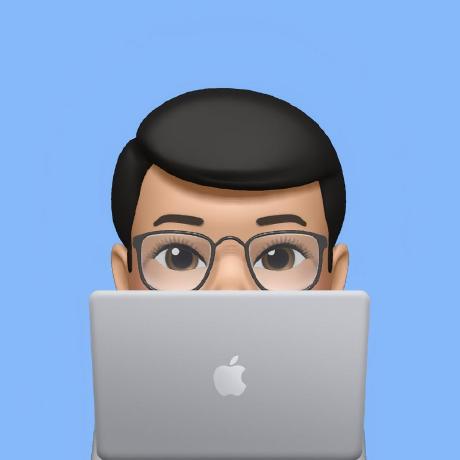
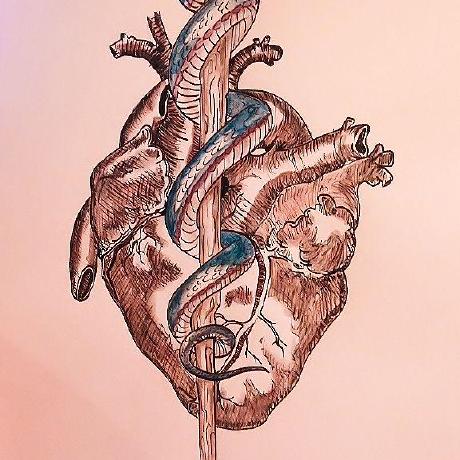
Top Related Projects
Laravel is a web application framework with expressive, elegant syntax. We’ve already laid the foundation for your next big idea — freeing you to create without sweating the small things.
Fast, unopinionated, minimalist web framework for node.
Spring Boot helps you to create Spring-powered, production-grade applications and services with absolute minimum fuss.
The Web framework for perfectionists with deadlines.
Ruby on Rails
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications with TypeScript/JavaScript 🚀
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot