recyclerview-animators
An Android Animation library which easily add itemanimator to RecyclerView items.
Top Related Projects
BRVAH:Powerful and flexible RecyclerAdapter
An Android library which provides simple Item animations to RecyclerView items
一款支持上下拉刷新、越界回弹、二级刷新、横向刷新、拉伸回弹、平滑滚动、嵌套滚动的多功能刷新控件
:octocat: ≡ GarlandView seamlessly transitions between multiple lists of content. Made by @Ramotion
Android Carousel LayoutManager for RecyclerView
Quick Overview
RecyclerView Animators is an Android library that provides easy-to-use item animations for RecyclerView. It offers a wide range of pre-built animations and allows developers to create custom animations for adding, removing, and moving items within a RecyclerView.
Pros
- Extensive collection of ready-to-use animations
- Easy integration with existing RecyclerView implementations
- Supports custom animation creation
- Lightweight and performant
Cons
- May impact performance on large lists or complex item layouts
- Some animations might not be suitable for all types of content
- Limited documentation for advanced customization
- Requires additional setup compared to default RecyclerView behavior
Code Examples
- Adding a fade-in animation to a RecyclerView:
val animator = FadeInAnimator()
recyclerView.itemAnimator = animator
- Customizing animation duration:
val animator = SlideInLeftAnimator()
animator.setAddDuration(300)
animator.setRemoveDuration(300)
recyclerView.itemAnimator = animator
- Creating a custom animation:
class CustomAnimator : BaseItemAnimator() {
override fun animateRemoveImpl(holder: RecyclerView.ViewHolder) {
holder.itemView.animate().apply {
translationX(holder.itemView.rootView.width.toFloat())
alpha(0f)
duration = removeDuration
interpolator = interpolator
setListener(DefaultRemoveAnimatorListener(holder))
startDelay = getRemoveDelay(holder)
}.start()
}
// Implement other animation methods as needed
}
Getting Started
To use RecyclerView Animators in your Android project:
- Add the dependency to your app's
build.gradle
file:
dependencies {
implementation 'jp.wasabeef:recyclerview-animators:4.0.2'
}
- Apply an animator to your RecyclerView:
import jp.wasabeef.recyclerview.animators.SlideInUpAnimator
// In your Activity or Fragment
recyclerView.itemAnimator = SlideInUpAnimator()
- Customize the animator if needed:
val animator = SlideInUpAnimator()
animator.setAddDuration(500)
animator.setRemoveDuration(500)
recyclerView.itemAnimator = animator
With these steps, your RecyclerView will now use the specified animations for item changes.
Competitor Comparisons
BRVAH:Powerful and flexible RecyclerAdapter
Pros of BaseRecyclerViewAdapterHelper
- More comprehensive feature set, including item dragging, swiping, and load more functionality
- Simplified adapter creation with less boilerplate code
- Active development and frequent updates
Cons of BaseRecyclerViewAdapterHelper
- Steeper learning curve due to more complex API
- Larger library size, which may impact app size
- Some features may be overkill for simpler RecyclerView implementations
Code Comparison
BaseRecyclerViewAdapterHelper:
class MyAdapter : BaseQuickAdapter<String, BaseViewHolder>(R.layout.item_layout) {
override fun convert(holder: BaseViewHolder, item: String) {
holder.setText(R.id.textView, item)
}
}
recyclerview-animators:
val adapter = ArrayAdapter(this, android.R.layout.simple_list_item_1, items)
recyclerView.adapter = adapter
recyclerView.itemAnimator = SlideInLeftAnimator()
BaseRecyclerViewAdapterHelper offers a more concise way to create adapters with built-in functionality, while recyclerview-animators focuses specifically on animations and requires less modification to existing adapters.
Both libraries serve different primary purposes: BaseRecyclerViewAdapterHelper aims to simplify overall RecyclerView implementation, while recyclerview-animators specializes in enhancing RecyclerView animations. The choice between them depends on the specific needs of your project and the level of customization required.
An Android library which provides simple Item animations to RecyclerView items
Pros of RecyclerViewItemAnimators
- Simpler API with fewer classes, making it easier to implement basic animations
- Focuses on core item animations without additional features, potentially reducing overhead
- Maintained more recently, with the last update in 2022
Cons of RecyclerViewItemAnimators
- Limited animation options compared to recyclerview-animators
- Less customization possibilities for complex animation scenarios
- Smaller community and fewer stars on GitHub, which may indicate less widespread adoption
Code Comparison
RecyclerViewItemAnimators:
RecyclerView.ItemAnimator itemAnimator = new SlideInOutLeftItemAnimator(recyclerView);
recyclerView.setItemAnimator(itemAnimator);
recyclerview-animators:
RecyclerView.ItemAnimator animator = new SlideInLeftAnimator();
animator.setAddDuration(1000);
animator.setRemoveDuration(1000);
recyclerView.setItemAnimator(animator);
Both libraries offer simple implementation, but recyclerview-animators provides more granular control over animation durations and types. RecyclerViewItemAnimators has a more straightforward approach, which may be preferable for simpler use cases.
一款支持上下拉刷新、越界回弹、二级刷新、横向刷新、拉伸回弹、平滑滚动、嵌套滚动的多功能刷新控件
Pros of SmoothRefreshLayout
- More comprehensive refresh and load-more functionality
- Supports multiple views, not limited to RecyclerView
- Highly customizable with various built-in header and footer styles
Cons of SmoothRefreshLayout
- Steeper learning curve due to more complex API
- Potentially higher overhead for simple use cases
- Less focus on item animations compared to recyclerview-animators
Code Comparison
SmoothRefreshLayout:
SmoothRefreshLayout refreshLayout = findViewById(R.id.refresh_layout);
refreshLayout.setOnRefreshListener(new RefreshingListenerAdapter() {
@Override
public void onRefreshing() {
// Handle refresh
}
});
recyclerview-animators:
RecyclerView recyclerView = findViewById(R.id.recycler_view);
recyclerView.setItemAnimator(new SlideInUpAnimator());
SmoothRefreshLayout offers more comprehensive refresh functionality but requires more setup, while recyclerview-animators focuses on simple, easy-to-implement item animations for RecyclerView.
SmoothRefreshLayout is better suited for complex layouts with custom refresh behaviors, while recyclerview-animators excels at adding polished animations to RecyclerView items with minimal code.
Both libraries serve different primary purposes: SmoothRefreshLayout for advanced refresh/load-more functionality, and recyclerview-animators for enhancing RecyclerView item animations. The choice between them depends on the specific requirements of your project.
:octocat: ≡ GarlandView seamlessly transitions between multiple lists of content. Made by @Ramotion
Pros of garland-view-android
- Unique and visually appealing UI component for displaying scrollable lists
- Customizable animation effects for a more engaging user experience
- Supports both vertical and horizontal orientations
Cons of garland-view-android
- More complex implementation compared to standard RecyclerView animations
- Limited to specific use cases where the garland-style view is appropriate
- May require more system resources due to advanced animations
Code Comparison
garland-view-android:
val garlandView = findViewById<GarlandView>(R.id.garland_view)
garlandView.setAdapter(GarlandAdapter(itemsList))
garlandView.setItemTransformer(GarlandAnimationTransformer())
recyclerview-animators:
val recyclerView = findViewById<RecyclerView>(R.id.recycler_view)
recyclerView.itemAnimator = SlideInLeftAnimator()
recyclerView.adapter = MyAdapter(itemsList)
Both libraries enhance the visual appeal of list views in Android applications. garland-view-android offers a unique and eye-catching scrollable list component with customizable animations, suitable for specific design requirements. recyclerview-animators, on the other hand, provides a wider range of animation options for standard RecyclerViews, offering more flexibility and easier implementation for general use cases. The choice between the two depends on the specific project requirements and desired visual effects.
Android Carousel LayoutManager for RecyclerView
Pros of CarouselLayoutManager
- Specialized for carousel-like layouts, offering a unique visual presentation
- Provides built-in scaling and alpha effects for items based on their position
- Supports both horizontal and vertical orientations
Cons of CarouselLayoutManager
- Limited to carousel-style layouts, less versatile for general RecyclerView animations
- May require more setup and customization for basic list animations
- Less actively maintained compared to recyclerview-animators
Code Comparison
CarouselLayoutManager:
val layoutManager = CarouselLayoutManager(CarouselLayoutManager.HORIZONTAL)
recyclerView.layoutManager = layoutManager
recyclerview-animators:
val animator = SlideInLeftAnimator()
recyclerView.itemAnimator = animator
CarouselLayoutManager focuses on layout management, while recyclerview-animators provides item animations that can be applied to any RecyclerView layout. The former requires setting a custom LayoutManager, while the latter modifies the ItemAnimator of the RecyclerView.
recyclerview-animators offers a wider range of animation options and can be easily applied to existing RecyclerViews without changing their layout structure. CarouselLayoutManager, on the other hand, provides a specific layout style with built-in visual effects, making it more suitable for projects requiring a carousel-like presentation of items.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
RecyclerView Animators
RecyclerView Animators is an Android library that allows developers to easily create RecyclerView with animations.
Please feel free to use this.
Features
- Animate addition and removal of
ItemAnimator
- Appearance animations for items in
RecyclerView.Adapter
Demo
ItemAnimator
Adapters
How do I use it?
Setup
Gradle
On your module's build.gradle
file add this implementation statement to the dependencies
section:
dependencies {
// Kotlin
implementation 'jp.wasabeef:recyclerview-animators:4.0.2'
}
Also make sure that the repositories
section includes not only "mavenCentral()"
but also a maven
section with the "google()"
endpoint.
repositories {
google()
mavenCentral()
jcenter()
}
ItemAnimator
Step 1
Set RecyclerView ItemAnimator.
val recyclerView = findViewById<RecyclerView>(R.id.list)
recyclerView.itemAnimator = SlideInLeftAnimator()
val recyclerView = findViewById<RecyclerView>(R.id.list)
recyclerView.itemAnimator = SlideInUpAnimator(OvershootInterpolator(1f))
Step 2
Please use the following
notifyItemChanged(int)
notifyItemInserted(int)
notifyItemRemoved(int)
notifyItemRangeChanged(int, int)
notifyItemRangeInserted(int, int)
notifyItemRangeRemoved(int, int)
If you want your animations to work, do not rely on calling
notifyDataSetChanged()
; as it is the RecyclerView's default behavior, animations are not triggered to start inside this method.
fun remove(position: Int) {
dataSet.removeAt(position)
notifyItemRemoved(position)
}
fun add(text: String, position: Int) {
dataSet.add(position, text)
notifyItemInserted(position)
}
Advanced Step 3
You can change the durations.
recyclerView.itemAnimator?.apply {
addDuration = 1000
removeDuration = 100
moveDuration = 1000
changeDuration = 100
}
Advanced Step 4
Change the interpolator.
recyclerView.itemAnimator = SlideInLeftAnimator().apply {
setInterpolator(OvershootInterpolator())
}
Advanced Step 5
By implementing AnimateViewHolder, you can override preset animation. So, custom animation can be set depending on view holder.
class MyViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView), AnimateViewHolder {
override fun preAnimateRemoveImpl(holder: RecyclerView.ViewHolder) {
// do something
}
override fun animateRemoveImpl(holder: RecyclerView.ViewHolder, listener: ViewPropertyAnimatorListener) {
itemView.animate().apply {
translationY(-itemView.height * 0.3f)
alpha(0f)
duration = 300
setListener(listener)
}.start()
}
override fun preAnimateAddImpl(holder: RecyclerView.ViewHolder) {
itemView.setTranslationY(-itemView.height * 0.3f)
itemView.setAlpha(0f)
}
override fun animateAddImpl(holder: RecyclerView.ViewHolder, listener: ViewPropertyAnimatorListener) {
itemView.animate().apply {
translationY(0f)
alpha(1f)
duration = 300
setListener(listener)
}.start()
}
}
Animators
Cool
LandingAnimator
Scale
ScaleInAnimator
, ScaleInTopAnimator
, ScaleInBottomAnimator
ScaleInLeftAnimator
, ScaleInRightAnimator
Fade
FadeInAnimator
, FadeInDownAnimator
, FadeInUpAnimator
FadeInLeftAnimator
, FadeInRightAnimator
Flip
FlipInTopXAnimator
, FlipInBottomXAnimator
FlipInLeftYAnimator
, FlipInRightYAnimator
Slide
SlideInLeftAnimator
, SlideInRightAnimator
, OvershootInLeftAnimator
, OvershootInRightAnimator
SlideInUpAnimator
, SlideInDownAnimator
RecyclerView.Adapter
Step 1
Set RecyclerView ItemAnimator.
val recyclerView = findViewById<RecyclerView>(R.id.list)
recyclerView.adapter = AlphaInAnimationAdapter(MyAdapter())
Java
RecyclerView recyclerView = findViewById(R.id.list);
recyclerView.setAdapter(new AlphaInAnimationAdapter(MyAdapter());
Advanced Step 2
recyclerView.adapter = AlphaInAnimationAdapter(MyAdapter()).apply {
// Change the durations.
setDuration(1000)
// Change the interpolator.
setInterpolator(vershootInterpolator())
// Disable the first scroll mode.
setFirstOnly(false)
}
Java
AlphaInAnimationAdapter alphaInAnimationAdapter = new AlphaInAnimationAdapter(new MyAdapter());
alphaInAnimationAdapter.setDuration(1000);
alphaInAnimationAdapter.setInterpolator(new OvershootInterpolator());
alphaInAnimationAdapter.setFirstOnly(false);
Advanced Step 3
Multiple Animations
val alphaAdapter = AlphaInAnimationAdapter(MyAdapter())
recyclerView.adapter = ScaleInAnimationAdapter(alphaAdapter)
Java
recyclerView.setAdapter(new ScaleInAnimationAdapter(alphaInAnimationAdapter));
Adapters
Alpha
AlphaInAnimationAdapter
Scale
ScaleInAnimationAdapter
Slide
SlideInBottomAnimationAdapter
SlideInRightAnimationAdapter
, SlideInLeftAnimationAdapter
Applications using RecyclerView Animators
Please ping me or send a pull request if you would like to be added here.
Icon | Application |
---|---|
Ameba Ownd | |
QuitNow! | |
AbemaTV | |
CL |
Developed By
Daichi Furiya (Wasabeef) - dadadada.chop@gmail.com
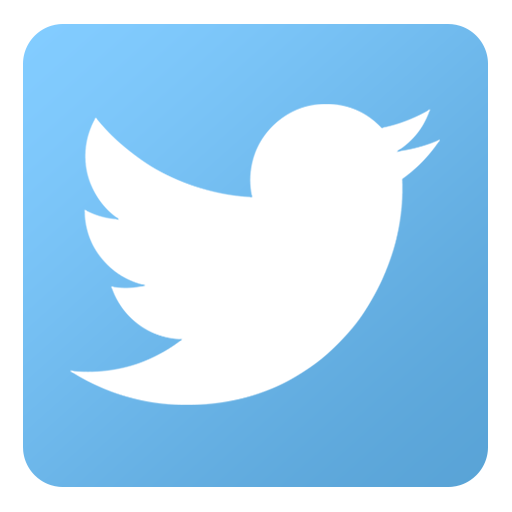
Contributions
Any contributions are welcome!
Contributers
Thanks
- Inspired by
AndroidViewAnimations
in daimajia.
License
Copyright 2020 Daichi Furiya / Wasabeef
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
Top Related Projects
BRVAH:Powerful and flexible RecyclerAdapter
An Android library which provides simple Item animations to RecyclerView items
一款支持上下拉刷新、越界回弹、二级刷新、横向刷新、拉伸回弹、平滑滚动、嵌套滚动的多功能刷新控件
:octocat: ≡ GarlandView seamlessly transitions between multiple lists of content. Made by @Ramotion
Android Carousel LayoutManager for RecyclerView
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot