richeditor-android
RichEditor for Android is a beautiful Rich Text WYSIWYG Editor for Android.
Top Related Projects
Quick Overview
RichEditor for Android is a rich text editor library for Android applications. It provides a customizable WYSIWYG editor that allows users to format text, insert images, and perform various editing operations within Android apps.
Pros
- Easy integration into Android projects
- Customizable appearance and functionality
- Supports a wide range of text formatting options
- Lightweight and efficient
Cons
- Limited documentation and examples
- Some reported issues with stability in certain scenarios
- May require additional customization for complex use cases
- Occasional rendering inconsistencies across different Android versions
Code Examples
- Basic initialization of the RichEditor:
val editor = findViewById<RichEditor>(R.id.editor)
editor.setEditorHeight(200)
editor.setEditorFontSize(22)
editor.setEditorFontColor(Color.BLACK)
editor.setPadding(10, 10, 10, 10)
editor.setPlaceholder("Insert text here...")
- Adding custom actions to the editor:
editor.setOnTextChangeListener { text ->
// Handle text changes
}
findViewById<View>(R.id.action_bold).setOnClickListener {
editor.setBold()
}
findViewById<View>(R.id.action_italic).setOnClickListener {
editor.setItalic()
}
- Inserting an image into the editor:
editor.insertImage("https://example.com/image.jpg", "Image description")
Getting Started
To use RichEditor in your Android project:
- Add the dependency to your app's
build.gradle
file:
dependencies {
implementation 'jp.wasabeef:richeditor-android:2.0.0'
}
- Add the RichEditor view to your layout XML:
<jp.wasabeef.richeditor.RichEditor
android:id="@+id/editor"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
- Initialize and customize the editor in your Activity or Fragment:
val editor = findViewById<RichEditor>(R.id.editor)
editor.setEditorHeight(200)
editor.setPlaceholder("Start typing...")
// Add custom actions
findViewById<View>(R.id.action_bold).setOnClickListener {
editor.setBold()
}
This setup provides a basic rich text editor with a bold formatting option. You can further customize the editor by adding more formatting options and adjusting its appearance to fit your app's needs.
Competitor Comparisons
Android平台下的富文本解析器,支持Html和Markdown
Pros of RichText
- Lightweight and easy to integrate, focusing on rendering rich text rather than editing
- Supports a wider range of HTML tags and attributes out of the box
- Better performance for displaying complex rich text content
Cons of RichText
- Lacks built-in editing capabilities, primarily focused on rendering
- May require additional libraries or custom implementation for text editing features
- Less extensive documentation and community support compared to richeditor-android
Code Comparison
RichText usage:
RichText.fromHtml(htmlString)
.into(textView);
richeditor-android usage:
mEditor = (RichEditor) findViewById(R.id.editor);
mEditor.setEditorHeight(200);
mEditor.setEditorFontSize(22);
mEditor.setEditorFontColor(Color.RED);
mEditor.setPadding(10, 10, 10, 10);
RichText is more straightforward for rendering rich text, while richeditor-android provides more customization options for editing. RichText is ideal for applications that primarily display rich content, whereas richeditor-android is better suited for apps requiring rich text editing capabilities.
Knife is a rich text editor component for writing documents in Android.
Pros of Knife
- Lightweight and simple to use, with a focus on basic text editing features
- Easier to integrate into existing projects due to its minimalistic approach
- Faster performance for basic text editing tasks
Cons of Knife
- Limited rich text editing capabilities compared to richeditor-android
- Less active development and community support
- Fewer customization options for advanced text formatting
Code Comparison
Knife:
KnifeText knife = (KnifeText) findViewById(R.id.knife);
knife.bold();
knife.italic();
knife.underline();
knife.strikethrough();
richeditor-android:
RichEditor mEditor = (RichEditor) findViewById(R.id.editor);
mEditor.setEditorHeight(200);
mEditor.setEditorFontSize(22);
mEditor.setEditorFontColor(Color.RED);
mEditor.setPadding(10, 10, 10, 10);
The code comparison shows that Knife focuses on basic text formatting methods, while richeditor-android offers more advanced customization options for the editor's appearance and behavior.
一个Android富文本类库,支持图文混排,支持编辑和预览,支持插入和删除图片。
Pros of XRichText
- Simpler implementation with fewer dependencies
- Better support for image handling and insertion
- More active development and recent updates
Cons of XRichText
- Less comprehensive formatting options compared to richeditor-android
- Limited documentation and examples available
- Smaller community and fewer contributions
Code Comparison
XRichText:
RichTextEditor editor = findViewById(R.id.rich_text_editor);
editor.insertImage(imagePath);
editor.setBold();
editor.setItalic();
editor.setUnderline();
richeditor-android:
RichEditor mEditor = (RichEditor) findViewById(R.id.editor);
mEditor.setEditorHeight(200);
mEditor.setEditorFontSize(22);
mEditor.setEditorFontColor(Color.RED);
mEditor.setPadding(10, 10, 10, 10);
XRichText offers a more straightforward API for basic text formatting and image insertion, while richeditor-android provides more detailed customization options for the editor's appearance and behavior. The choice between the two depends on the specific requirements of your project, with XRichText being better suited for simpler implementations and richeditor-android offering more advanced features at the cost of complexity.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
RichEditor for Android
is a beautiful Rich Text WYSIWYG Editor
for Android
.
- Looking for iOS? Check out cjwirth/RichEditorView
Supported Functions
- Bold
- Italic
- Subscript
- Superscript
- Strikethrough
- Underline
- Justify Left
- Justify Center
- Justify Right
- Blockquote
- Heading 1
- Heading 2
- Heading 3
- Heading 4
- Heading 5
- Heading 6
- Undo
- Redo
- Indent
- Outdent
- Insert Image
- Insert Youtube
- Insert Video
- Insert Audio
- Insert Link
- Checkbox
- Text Color
- Text Background Color
- Text Font Size
- Unordered List (Bullets)
- Ordered List (Numbers)
Attribute change of editor
- Font Size
- Background Color
- Width
- Height
- Placeholder
- Load CSS
- State Callback
Milestone
- Font Family
Demo
How do I use it?
Setup
Gradle
repositories {
mavenCentral()
}
dependencies {
implementation 'jp.wasabeef:richeditor-android:2.0.0'
}
Default Setting for Editor
Height
editor.setEditorHeight(200);
Font
editor.setEditorFontSize(22);
editor.setEditorFontColor(Color.RED);
Background
editor.setEditorBackgroundColor(Color.BLUE);
editor.setBackgroundColor(Color.BLUE);
editor.setBackgroundResource(R.drawable.bg);
editor.setBackground("https://raw.githubusercontent.com/wasabeef/art/master/chip.jpg");
Padding
editor.setPadding(10, 10, 10, 10);
Placeholder
editor.setPlaceholder("Insert text here...");
Others
Please refer the samples for usage.
Functions for ContentEditable
Bold
editor.setBold();
Italic
editor.setItalic();
Insert Image
editor.insertImage("https://raw.githubusercontent.com/wasabeef/art/master/twitter.png","twitter");
Text Change Listener
RichEditor editor = (RichEditor) findViewById(R.id.editor);
editor. setOnTextChangeListener(new RichEditor.OnTextChangeListener() {
@Override
public void onTextChange(String text) {
// Do Something
Log.d("RichEditor", "Preview " + text);
}
});
Others
Please refer the samples for usage.
Requirements
Android 4+
Applications using RichEditor for Android
Please ping me or send a pull request if you would like to be added here.
Icon | Application |
---|---|
Ameba Ownd | |
ScorePal |
Developed By
Daichi Furiya (Wasabeef) - dadadada.chop@gmail.com
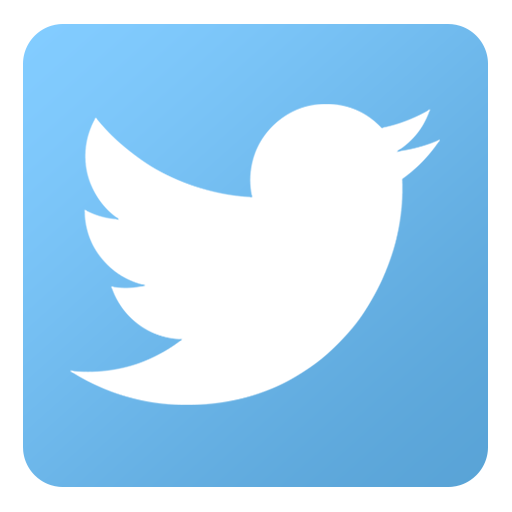
Thanks
- Inspired by
ZSSRichTextEditor
in nnhubbard.
License
Copyright (C) 2020 Wasabeef
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
Top Related Projects
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot