Top Related Projects
A scriptable music downloader for Qobuz, Tidal, SoundCloud, and Deezer
Quick Overview
Tidal-Media-Downloader is an open-source tool for downloading music and videos from the Tidal streaming service. It allows users to download tracks, albums, playlists, and music videos in various quality formats, including lossless audio.
Pros
- Supports high-quality audio downloads, including MQA and FLAC formats
- Offers a command-line interface and a graphical user interface for different user preferences
- Allows batch downloading of entire albums, playlists, and artist discographies
- Includes features like automatic metadata tagging and cover art downloading
Cons
- Requires a Tidal account and may violate Tidal's terms of service
- Depends on Tidal's API, which may change and break functionality
- Limited to Tidal content only, not usable with other streaming services
- May face potential legal issues due to copyright concerns
Code Examples
# Initialize the Tidal client
from tidal_dl import TidalClient
client = TidalClient()
client.login(username="your_username", password="your_password")
# Download a single track
track_id = "12345678"
client.download_track(track_id)
# Download an entire album
album_id = "87654321"
client.download_album(album_id)
# Download a playlist
playlist_id = "11223344"
client.download_playlist(playlist_id)
Getting Started
-
Install the package:
pip install tidal-dl
-
Run the CLI:
tidal-dl
-
Log in with your Tidal account credentials when prompted.
-
Use commands like
track <ID>
,album <ID>
, orplaylist <ID>
to download content.
For the GUI version, run:
tidal-gui
Note: Always ensure you have the right to download and use the content according to your Tidal subscription and local copyright laws.
Competitor Comparisons
A scriptable music downloader for Qobuz, Tidal, SoundCloud, and Deezer
Pros of streamrip
- Supports multiple streaming services (Tidal, Qobuz, Deezer, SoundCloud)
- Offers a command-line interface for easier automation and scripting
- Provides more advanced features like playlist syncing and metadata editing
Cons of streamrip
- May have a steeper learning curve due to its command-line nature
- Potentially less user-friendly for those who prefer graphical interfaces
- Requires more setup and configuration compared to Tidal-Media-Downloader
Code Comparison
Tidal-Media-Downloader:
def start(argv=None):
args = parse_args(argv)
create_logfile(args.logfile)
SETTINGS.read(args.config)
run(args)
streamrip:
def main():
args = parse_args()
config = load_config()
setup_logging(args.verbose)
run_command(args, config)
Both projects use similar structures for their main entry points, parsing arguments and loading configurations. However, streamrip's code appears to be more modular and follows a more modern Python style. Tidal-Media-Downloader's code is more focused on Tidal-specific functionality, while streamrip's design allows for multiple service integrations.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
[GUI-REPOSITORY]
«Tidal-Media-Downloader» is an application that lets you download videos and tracks from Tidal. It supports two version: tidal-dl and tidal-gui. (This repository only contains tidal-dl, and the release isn't the newest gui version.)
Download |
Documentation |
ä¸æææ¡£ |
ðº Installation
pip3 install tidal-dl --upgrade
USE | FUNCTION |
---|---|
tidal-dl | Show interactive interface |
tidal-dl -h | Show help-message |
tidal-dl -l "https://tidal.com/browse/track/70973230" | Download link |
tidal-dl -g | Show simple-gui |
If you are using windows system, you can use tidal-pro
Nightly Builds
Download nightly builds from continuous integration: |
---|
ð¤ Features
-
Download album \ track \ video \ playlist \ artist-albums
-
Add metadata to songs
-
Selectable video resolution and track quality
ð½ User Interface
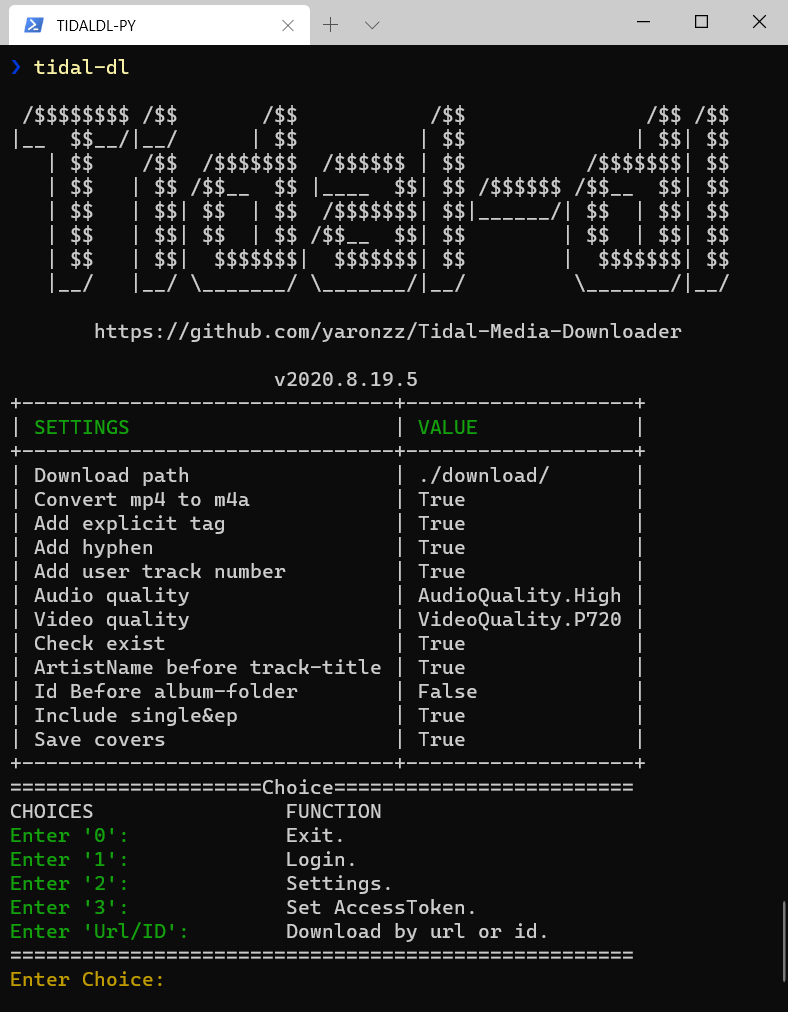
Settings - Possible Tags
Album
Tag | Example value |
---|---|
{ArtistName} | The Beatles |
{AlbumArtistName} | The Beatles |
{Flag} | M/A/E (Master/Dolby Atmos/Explicit) |
{AlbumID} | 55163243 |
{AlbumYear} | 1963 |
{AlbumTitle} | Please Please Me (Remastered) |
{AudioQuality} | LOSSLESS |
{DurationSeconds} | 1919 |
{Duration} | 31:59 |
{NumberOfTracks} | 14 |
{NumberOfVideos} | 0 |
{NumberOfVolumes} | 1 |
{ReleaseDate} | 1963-03-22 |
{RecordType} | ALBUM |
{None} |
Track
Tag | Example Value |
---|---|
{TrackNumber} | 01 |
{ArtistName} | The Beatles |
{ArtistsName} | The Beatles |
{TrackTitle} | I Saw Her Standing There (Remastered 2009) |
{ExplicitFlag} | (Explicit) |
{AlbumYear} | 1963 |
{AlbumTitle} | Please Please Me (Remastered) |
{AudioQuality} | LOSSLESS |
{DurationSeconds} | 173 |
{Duration} | 02:53 |
{TrackID} | 55163244 |
Video
Tag | Example Value |
---|---|
{VideoNumber} | 00 |
{ArtistName} | DMX |
{ArtistsName} | DMX, Westside Gunn |
{VideoTitle} | Hood Blues |
{ExplicitFlag} | (Explicit) |
{VideoYear} | 2021 |
{TrackID} | 188932980 |
â Support
If you really like my projects and want to support me, you can buy me a coffee and star this project.
ð Contributors
This project exists thanks to all the people who contribute.
ð¨ Libraries and reference
ð Disclaimer
- Private use only.
- Need a Tidal-HIFI subscription.
- You should not use this method to distribute or pirate music.
- It may be illegal to use this in your country, so be informed.
Developing
pip3 uninstall tidal-dl
pip3 install -r requirements.txt --user
python3 setup.py install
Top Related Projects
A scriptable music downloader for Qobuz, Tidal, SoundCloud, and Deezer
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot