Top Related Projects
The library for web and native user interfaces.
This is the repo for Vue 2. For Vue 3, go to https://github.com/vuejs/core
web development for the rest of us
Deliver web apps with confidence 🚀
A declarative, efficient, and flexible JavaScript library for building user interfaces.
⚛️ Fast 3kB React alternative with the same modern API. Components & Virtual DOM.
Quick Overview
Yew is a modern web framework for creating multi-threaded front-end web applications using Rust. It leverages WebAssembly for high performance and provides a React-like declarative model for building user interfaces.
Pros
- High performance due to Rust and WebAssembly
- Strong type safety and memory safety inherited from Rust
- Seamless JavaScript interoperability
- Component-based architecture similar to React
Cons
- Steeper learning curve for developers not familiar with Rust
- Smaller ecosystem compared to more established JavaScript frameworks
- Potentially larger initial bundle size due to WebAssembly
- Limited browser support for older versions
Code Examples
- Creating a simple component:
use yew::prelude::*;
#[function_component]
fn App() -> Html {
html! {
<div>
<h1>{ "Hello, Yew!" }</h1>
</div>
}
}
- Handling state with hooks:
use yew::prelude::*;
#[function_component]
fn Counter() -> Html {
let counter = use_state(|| 0);
let onclick = {
let counter = counter.clone();
Callback::from(move |_| counter.set(*counter + 1))
};
html! {
<div>
<p>{ "Current count: " }{ *counter }</p>
<button {onclick}>{ "Increment" }</button>
</div>
}
}
- Using properties in a component:
use yew::prelude::*;
#[derive(Properties, PartialEq)]
pub struct NameProps {
pub name: String,
}
#[function_component]
fn NameDisplay(props: &NameProps) -> Html {
html! {
<p>{ format!("Hello, {}!", props.name) }</p>
}
}
Getting Started
To start using Yew, follow these steps:
- Install Rust and cargo: https://www.rust-lang.org/tools/install
- Install
trunk
for building and serving Yew applications:cargo install trunk
- Create a new Yew project:
cargo new yew-app cd yew-app
- Add Yew to your
Cargo.toml
:[dependencies] yew = "0.20"
- Replace
src/main.rs
with a basic Yew app:use yew::prelude::*; #[function_component] fn App() -> Html { html! { <h1>{ "Hello, Yew!" }</h1> } } fn main() { yew::Renderer::<App>::new().render(); }
- Run your app with
trunk serve
Competitor Comparisons
The library for web and native user interfaces.
Pros of React
- Larger ecosystem and community support
- More mature and battle-tested in production environments
- Extensive documentation and learning resources
Cons of React
- Larger bundle size and potential performance overhead
- Steeper learning curve for beginners
- Requires additional tools for state management in complex applications
Code Comparison
React:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
const element = <Welcome name="Sara" />;
Yew:
use yew::prelude::*;
#[function_component(Welcome)]
fn welcome(props: &Props) -> Html {
html! { <h1>{ format!("Hello, {}", props.name) }</h1> }
}
html! { <Welcome name="Sara" /> }
Both frameworks use a component-based architecture and support JSX-like syntax. React uses JavaScript, while Yew leverages Rust's strong typing and performance benefits. Yew's syntax is more verbose due to Rust's stricter type system, but it offers better performance and smaller bundle sizes. React's ecosystem is more extensive, making it easier to find solutions and third-party libraries. Yew, being newer and Rust-based, has a smaller community but is growing rapidly, especially for WebAssembly applications.
This is the repo for Vue 2. For Vue 3, go to https://github.com/vuejs/core
Pros of Vue
- Larger ecosystem and community support
- More mature and battle-tested in production environments
- Extensive documentation and learning resources
Cons of Vue
- Larger bundle size compared to Yew
- Potentially slower performance due to JavaScript runtime
- Less type safety compared to Rust-based Yew
Code Comparison
Vue component:
<template>
<div>{{ message }}</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello, Vue!'
}
}
}
</script>
Yew component:
use yew::prelude::*;
#[function_component]
fn App() -> Html {
let message = "Hello, Yew!";
html! { <div>{ message }</div> }
}
Vue uses a template-based approach with separate script and template sections, while Yew leverages Rust's type system and macros for a more concise and type-safe component definition. Vue's syntax may be more familiar to web developers, but Yew offers stronger compile-time guarantees and potentially better performance due to its Rust foundation.
web development for the rest of us
Pros of Svelte
- Smaller bundle sizes due to compile-time optimization
- Simpler syntax with less boilerplate code
- Faster runtime performance, especially for complex UIs
Cons of Svelte
- Smaller ecosystem and community compared to Yew
- Limited support for server-side rendering
- Steeper learning curve for developers familiar with traditional frameworks
Code Comparison
Svelte component:
<script>
let count = 0;
const increment = () => count += 1;
</script>
<button on:click={increment}>
Clicks: {count}
</button>
Yew component:
use yew::prelude::*;
pub struct Counter {
count: i32,
}
impl Component for Counter {
type Message = ();
type Properties = ();
fn create(_ctx: &Context<Self>) -> Self {
Self { count: 0 }
}
fn update(&mut self, _ctx: &Context<Self>, _msg: Self::Message) -> bool {
self.count += 1;
true
}
fn view(&self, ctx: &Context<Self>) -> Html {
html! {
<button onclick={ctx.link().callback(|_| ())}>
{ format!("Clicks: {}", self.count) }
</button>
}
}
}
Deliver web apps with confidence 🚀
Pros of Angular
- Mature ecosystem with extensive documentation and community support
- Comprehensive framework with built-in features like routing and dependency injection
- Powerful CLI for scaffolding and development tasks
Cons of Angular
- Steeper learning curve due to its complexity and TypeScript requirement
- Larger bundle size, potentially impacting initial load times
- More opinionated structure, which may limit flexibility in some cases
Code Comparison
Angular component:
@Component({
selector: 'app-hello',
template: '<h1>Hello, {{name}}!</h1>'
})
export class HelloComponent {
name: string = 'World';
}
Yew component:
use yew::prelude::*;
#[function_component(Hello)]
pub fn hello() -> Html {
let name = "World";
html! { <h1>{ format!("Hello, {}!", name) }</h1> }
}
Summary
Angular is a mature, feature-rich framework with strong tooling support, ideal for large-scale applications. Yew, being newer and Rust-based, offers a lighter-weight alternative with potential performance benefits. Angular's TypeScript foundation provides robust type checking, while Yew leverages Rust's safety features. The choice between them depends on project requirements, team expertise, and performance considerations.
A declarative, efficient, and flexible JavaScript library for building user interfaces.
Pros of Solid
- Smaller bundle size and faster runtime performance
- Fine-grained reactivity without a virtual DOM
- Simpler mental model with fewer abstractions
Cons of Solid
- Smaller ecosystem and community compared to Yew
- Less mature, with potential for more frequent breaking changes
- Limited native mobile support
Code Comparison
Yew:
use yew::prelude::*;
#[function_component(App)]
fn app() -> Html {
let counter = use_state(|| 0);
let onclick = {
let counter = counter.clone();
Callback::from(move |_| counter.set(*counter + 1))
};
html! {
<div>
<button {onclick}>{ "+1" }</button>
<p>{ *counter }</p>
</div>
}
}
Solid:
import { createSignal } from "solid-js";
function App() {
const [count, setCount] = createSignal(0);
return (
<div>
<button onClick={() => setCount(count() + 1)}>+1</button>
<p>{count()}</p>
</div>
);
}
⚛️ Fast 3kB React alternative with the same modern API. Components & Virtual DOM.
Pros of Preact
- Smaller bundle size and faster performance
- Easier learning curve for developers familiar with React
- Extensive ecosystem and community support
Cons of Preact
- Less type safety compared to Yew's Rust-based approach
- Limited built-in state management solutions
- Potentially more challenging for developers without JavaScript experience
Code Comparison
Preact:
import { h, render } from 'preact';
const App = () => <h1>Hello, World!</h1>;
render(<App />, document.body);
Yew:
use yew::prelude::*;
#[function_component(App)]
fn app() -> Html {
html! { <h1>{"Hello, World!"}</h1> }
}
fn main() {
yew::start_app::<App>();
}
Both Yew and Preact are popular choices for building web applications, but they cater to different developer preferences and project requirements. Preact offers a lightweight alternative to React with familiar syntax, while Yew leverages Rust's strong typing and performance benefits. The choice between them often depends on factors such as team expertise, project scale, and specific performance needs.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
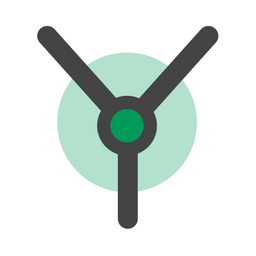
Yew
Rust / Wasm client web app framework
Documentation (stable) | Documentation (latest) | Examples | Changelog | Roadmap | ç®ä½ä¸æææ¡£ | ç¹é«ä¸æææª | ããã¥ã¡ã³ã
About
Yew is a modern Rust framework for creating multi-threaded front-end web apps with WebAssembly.
- Features a macro for declaring interactive HTML with Rust expressions. Developers who have experience using JSX in React should feel quite at home when using Yew.
- Achieves high performance by minimizing DOM API calls for each page render and by making it easy to offload processing to background web workers.
- Supports JavaScript interoperability, allowing developers to leverage NPM packages and integrate with existing JavaScript applications.
Note: Yew is not 1.0 yet. Be prepared to do major refactoring due to breaking API changes.
Contributing
Yew is a community effort and we welcome all kinds of contributions, big or small, from developers of all backgrounds. We want the Yew community to be a fun and friendly place, so please review our Code of Conduct to learn what behavior will not be tolerated.
ð¤ New to Yew?
Start learning about the framework by helping us improve our documentation. Pull requests which improve test coverage are also very welcome.
ð Looking for inspiration?
Check out the community curated list of awesome things related to Yew / WebAssembly at jetli/awesome-yew.
ð¤ Confused about something?
Feel free to drop into our Discord chatroom or open a new "Question" issue to get help from contributors. Often questions lead to improvements to the ergonomics of the framework, better documentation, and even new features!
ð Ready to dive into the code?
After reviewing the Contribution Guide, check out the "Good First Issues" (they are eager for attention!). Once you find one that interests you, feel free to assign yourself to an issue and don't hesitate to reach out for guidance, the issues vary in complexity.
ð± Found a bug?
Please report all bugs! We are happy to help support developers fix the bugs they find if they are interested and have the time.
ð¤ Want to help translate?
Translations can be submitted on the Yew GitLocalize Repo. If you are interested in being the official moderator for a language, please reach out on Discord.
Contributors
Code Contributors
This project exists thanks to all the people who contribute.
Financial Contributors
Become a financial contributor and help us sustain our community. [Contribute]
Individuals
Organizations
Support this project with your organization. Your logo will show up here with a link to your website. [Contribute]
Top Related Projects
The library for web and native user interfaces.
This is the repo for Vue 2. For Vue 3, go to https://github.com/vuejs/core
web development for the rest of us
Deliver web apps with confidence 🚀
A declarative, efficient, and flexible JavaScript library for building user interfaces.
⚛️ Fast 3kB React alternative with the same modern API. Components & Virtual DOM.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot