Top Related Projects
TypeScript is a superset of JavaScript that compiles to clean JavaScript output.
TypeScript Compiler API wrapper for static analysis and programmatic code changes.
:sparkles: Monorepo for all the tooling which enables ESLint to support TypeScript
A Jest transformer with source map support that lets you use Jest to test projects written in TypeScript.
:books: The definitive guide to TypeScript and possibly the best TypeScript book :book:. Free and Open Source 🌹
TypeScript execution and REPL for node.js
Quick Overview
Pretty TS Errors is a Visual Studio Code extension that enhances TypeScript error messages. It transforms complex and hard-to-read TypeScript errors into more user-friendly, formatted, and easier-to-understand messages, improving the developer experience when working with TypeScript.
Pros
- Significantly improves readability of TypeScript error messages
- Provides color-coded and formatted error output
- Includes links to relevant TypeScript documentation
- Supports custom themes and configurations
Cons
- Only available for Visual Studio Code
- May not cover all possible TypeScript error scenarios
- Requires additional setup and configuration
- Could potentially slow down the IDE for very large projects
Getting Started
- Install the "Pretty TypeScript Errors" extension from the Visual Studio Code marketplace.
- Open a TypeScript project in VS Code.
- When you encounter a TypeScript error, the extension will automatically format and display the enhanced error message.
To customize the extension:
- Open VS Code settings (File > Preferences > Settings)
- Search for "Pretty TypeScript Errors"
- Adjust settings such as color theme, font size, and display options according to your preferences.
Competitor Comparisons
TypeScript is a superset of JavaScript that compiles to clean JavaScript output.
Pros of TypeScript
- Comprehensive language implementation with full compiler and tooling support
- Extensive documentation and large community backing
- Regular updates and improvements to the core language features
Cons of TypeScript
- Error messages can be complex and difficult to understand for beginners
- Large codebase and extensive features may be overwhelming for simple projects
- Steeper learning curve compared to vanilla JavaScript
Code Comparison
TypeScript (error output):
let x: number = "hello";
// Type 'string' is not assignable to type 'number'.
pretty-ts-errors (enhanced error output):
let x: number = "hello";
// ❌ Type 'string' is not assignable to type 'number'.
//
// The type 'string' cannot be assigned to 'number'.
// Consider using a type assertion or type guard to convert the value.
//
// Example:
// let x: number = parseInt("hello");
Summary
While TypeScript provides a robust and feature-rich implementation of the language, pretty-ts-errors focuses on improving the readability and understandability of TypeScript error messages. TypeScript offers comprehensive tooling and language support, but its error messages can be complex. pretty-ts-errors addresses this issue by providing more user-friendly and informative error outputs, making it easier for developers, especially beginners, to understand and resolve TypeScript errors.
TypeScript Compiler API wrapper for static analysis and programmatic code changes.
Pros of ts-morph
- More comprehensive TypeScript manipulation capabilities
- Provides a powerful API for analyzing and transforming TypeScript code
- Suitable for complex TypeScript tooling and refactoring tasks
Cons of ts-morph
- Steeper learning curve due to its extensive API
- May be overkill for simple error formatting tasks
- Larger package size and potential performance overhead
Code Comparison
ts-morph:
import { Project } from "ts-morph";
const project = new Project();
const sourceFile = project.createSourceFile("file.ts", "let x: string = 5;");
const diagnostics = sourceFile.getPreEmitDiagnostics();
pretty-ts-errors:
import { prettyTSErrors } from "pretty-ts-errors";
const errorMessage = "TS2322: Type 'number' is not assignable to type 'string'.";
const formattedError = prettyTSErrors(errorMessage);
Summary
ts-morph is a powerful library for TypeScript code analysis and manipulation, offering extensive capabilities for complex tasks. However, it may be excessive for simple error formatting. pretty-ts-errors, on the other hand, focuses specifically on improving TypeScript error readability, making it more suitable for straightforward error presentation tasks.
:sparkles: Monorepo for all the tooling which enables ESLint to support TypeScript
Pros of typescript-eslint
- Comprehensive linting and static analysis for TypeScript
- Highly configurable with numerous rules and plugins
- Integrates seamlessly with ESLint ecosystem
Cons of typescript-eslint
- More complex setup and configuration
- Can be resource-intensive for large projects
- Requires additional dependencies and maintenance
Code Comparison
typescript-eslint configuration:
{
"parser": "@typescript-eslint/parser",
"plugins": ["@typescript-eslint"],
"extends": ["eslint:recommended", "plugin:@typescript-eslint/recommended"]
}
pretty-ts-errors usage:
import { prettyTSErrors } from 'pretty-ts-errors';
prettyTSErrors(tsErrors);
Summary
typescript-eslint is a powerful and flexible tool for linting and analyzing TypeScript code, offering extensive customization options and integration with the ESLint ecosystem. It provides a comprehensive set of rules and plugins to enforce coding standards and catch potential issues.
On the other hand, pretty-ts-errors focuses specifically on improving the readability and presentation of TypeScript compiler errors. It's a lightweight solution that enhances error messages without the need for complex configuration.
While typescript-eslint offers more features and customization, it comes with a steeper learning curve and higher resource requirements. pretty-ts-errors provides a simpler, more focused solution for improving TypeScript error messages without the overhead of a full linting setup.
A Jest transformer with source map support that lets you use Jest to test projects written in TypeScript.
Pros of ts-jest
- Integrates TypeScript with Jest, allowing for seamless testing of TypeScript projects
- Provides type checking during test execution, enhancing code quality
- Supports various TypeScript configurations and custom transformers
Cons of ts-jest
- Focuses solely on testing, not on improving TypeScript error messages
- May have a steeper learning curve for those unfamiliar with Jest
- Can potentially slow down test execution due to type checking
Code Comparison
ts-jest configuration:
{
"jest": {
"preset": "ts-jest",
"testEnvironment": "node"
}
}
pretty-ts-errors usage:
import { prettyTsErrors } from 'pretty-ts-errors';
prettyTsErrors(tsErrors);
Summary
ts-jest is a powerful tool for integrating TypeScript with Jest for testing purposes, while pretty-ts-errors focuses on improving the readability of TypeScript error messages. ts-jest offers robust testing capabilities and type checking during test execution, but it may require more setup and potentially slow down tests. pretty-ts-errors, on the other hand, is specifically designed to enhance error messages, making it easier for developers to understand and fix TypeScript errors quickly. The choice between these tools depends on whether the primary need is for TypeScript testing integration or improved error message formatting.
:books: The definitive guide to TypeScript and possibly the best TypeScript book :book:. Free and Open Source 🌹
Pros of typescript-book
- Comprehensive guide covering a wide range of TypeScript topics
- In-depth explanations and examples for better understanding
- Regularly updated with new TypeScript features and best practices
Cons of typescript-book
- Not focused on error handling or improving error messages
- Requires more time investment to find specific error-related information
- May be overwhelming for beginners looking for quick error solutions
Code comparison
typescript-book example (basic type annotation):
let isDone: boolean = false;
let decimal: number = 6;
let color: string = "blue";
pretty-ts-errors example (error message enhancement):
// No direct code comparison available
// pretty-ts-errors focuses on enhancing TypeScript error messages
// rather than providing code examples
Summary
typescript-book is a comprehensive resource for learning TypeScript, offering in-depth explanations and examples across various topics. It's regularly updated but may require more time investment to find specific error-related information. pretty-ts-errors, on the other hand, focuses specifically on enhancing TypeScript error messages, making it more suitable for quick error resolution but less comprehensive in overall TypeScript education.
TypeScript execution and REPL for node.js
Pros of ts-node
- Provides a complete runtime environment for executing TypeScript directly
- Offers seamless integration with Node.js ecosystem and packages
- Supports various configuration options and customization
Cons of ts-node
- Heavier and more complex setup compared to pretty-ts-errors
- May introduce performance overhead due to runtime compilation
- Not specifically focused on error presentation and formatting
Code Comparison
ts-node usage:
// Execute TypeScript directly
ts-node script.ts
// Use with Node.js REPL
ts-node
// Register ts-node for on-the-fly compilation
require('ts-node').register();
require('./script.ts');
pretty-ts-errors usage:
// No direct execution, used as a VS Code extension
// Automatically formats TypeScript errors in the editor
ts-node is a comprehensive solution for running TypeScript in Node.js environments, offering direct execution and integration with the Node.js ecosystem. It provides a full runtime environment but may introduce some performance overhead.
pretty-ts-errors, on the other hand, is a lightweight VS Code extension focused solely on improving the presentation of TypeScript errors in the editor. It doesn't provide execution capabilities but excels in making error messages more readable and user-friendly.
While ts-node is ideal for development and running TypeScript applications, pretty-ts-errors enhances the development experience by improving error visibility and comprehension within the VS Code environment.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Pretty TypeScript
Errors
Make TypeScript errors prettier and human-readable in VSCode.
TypeScript errors become messier as the complexity of types increases. At some point, TypeScript will throw on you a shitty heap of parentheses and "..."
.
This extension will help you understand what's going on. For example, in this relatively simple error:
Watch this

and others from: Web Dev Simplified, Josh tried coding, trash dev, and more
Features
- Syntax highlighting with your theme colors for types in error messages, supporting both light and dark themes
- A button that leads you to the relevant type declaration next to the type in the error message
- A button that navigates you to the error at typescript.tv, where you can find a detailed explanation, sometimes with a video
- A button that navigates you to ts-error-translator, where you can read the error in plain English
Supports
- Node and Deno TypeScript error reporters (in
.ts
files) - JSDoc type errors (in
.js
and.jsx
files) - React, Solid and Qwik errors (in
.tsx
and.mdx
files) - Astro, Svelte and Vue files when TypeScript is enabled (in
.astro
,.svelte
and.vue
files) - Ember and Glimmer TypeScript errors and template issues reported by Glint (in
.hbs
,.gjs
, and.gts
files)
Installation
code --install-extension yoavbls.pretty-ts-errors
Or simply by searching for pretty-ts-errors
in the VSCode marketplace
How to hide the original errors and make the types copyable
Follow the instructions there. unfortunately, this hack is required because of VSCode limitations.
Why isn't it trivial
- TypeScript errors contain types that are not valid in TypeScript.
Yes, these types include things like... more ...
,{ ... }
, etc in an inconsistent manner. Some are also cutting in the middle because they're too long. - Types can't be syntax highlighted in code blocks because the part of
type X = ...
is missing, so I needed to create a new TextMate grammar, a superset of TypeScript grammar calledtype
. - VSCode markdown blocks all styling options, so I had to find hacks to style the error messages. e.g., there isn't an inlined code block on VSCode markdown, so I used a code block inside a codicon icon, which is the only thing that can be inlined. That's why it can't be copied. but it isn't a problem because you can still hover on the error and copy things from the original error pane.
Hype section
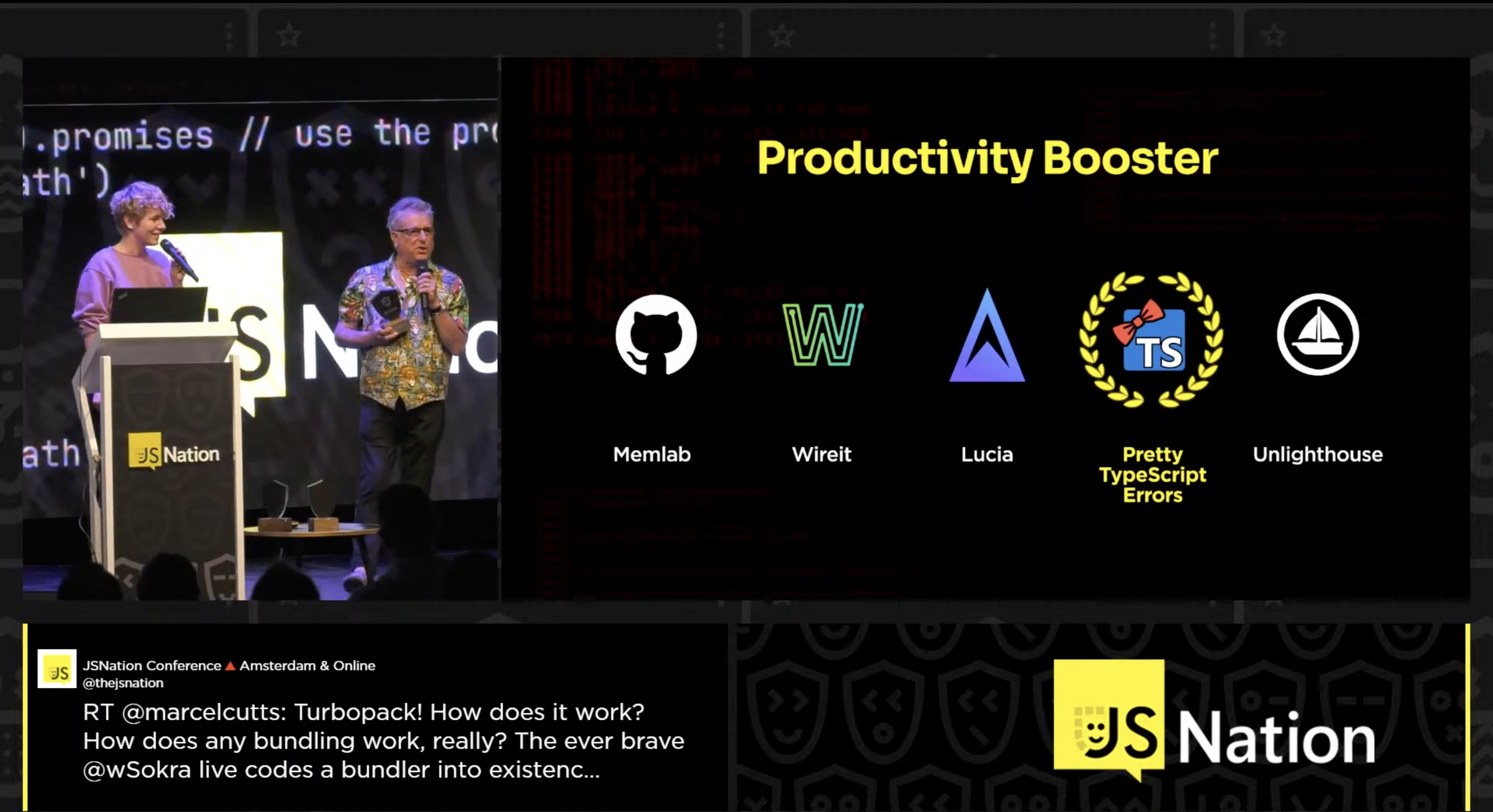



Stars from stars
![]() Kent C. Dodds |
![]() Matt Pocock |
![]() Alex / KATT |
![]() Tanner Linsley |
![]() Theo Browne |
Sponsorship
Every penny will be invested in other contributors to the project, especially ones that work
on things that I can't be doing myself like adding support to the extension for other IDEs ð«
Contribution
Help by upvoting or commenting on issues we need to be resolved here
Any other contribution is welcome. Feel free to open any issue / PR you think.
Top Related Projects
TypeScript is a superset of JavaScript that compiles to clean JavaScript output.
TypeScript Compiler API wrapper for static analysis and programmatic code changes.
:sparkles: Monorepo for all the tooling which enables ESLint to support TypeScript
A Jest transformer with source map support that lets you use Jest to test projects written in TypeScript.
:books: The definitive guide to TypeScript and possibly the best TypeScript book :book:. Free and Open Source 🌹
TypeScript execution and REPL for node.js
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot