Top Related Projects
:electron: Build cross-platform desktop apps with JavaScript, HTML, and CSS
Build smaller, faster, and more secure desktop and mobile applications with a web frontend.
Create beautiful applications using Go
Portable and lightweight cross-platform desktop application development framework
Tiny cross-platform webview library for C/C++. Uses WebKit (GTK/Cocoa) and Edge WebView2 (Windows).
Make any web page a desktop application
Quick Overview
Lorca is a lightweight Go library for building cross-platform desktop applications using HTML, CSS, and JavaScript. It leverages the locally installed Chrome browser as a UI layer, allowing developers to create native-feeling desktop apps using web technologies while keeping the binary size small.
Pros
- Small binary size (typically under 3MB) as it doesn't bundle a web engine
- Cross-platform support (Windows, macOS, Linux)
- Utilizes the user's installed Chrome browser, ensuring up-to-date web standards
- Simple API for Go developers to create desktop applications
Cons
- Requires Chrome to be installed on the user's system
- Limited to Chrome's capabilities and features
- Not suitable for applications requiring deep system integration
- May have security concerns due to using the user's browser instance
Code Examples
- Creating a simple window:
ui, _ := lorca.New("", "", 480, 320)
defer ui.Close()
// Bind Go function to JavaScript
ui.Bind("add", func(a, b int) int { return a + b })
// Load HTML
ui.Load("data:text/html,<html><body><h1>Hello World</h1></body></html>")
<-ui.Done()
- Executing JavaScript from Go:
ui, _ := lorca.New("", "", 480, 320)
defer ui.Close()
// Execute JavaScript
result := ui.Eval(`
const name = "Lorca";
console.log("Hello, " + name);
name;
`)
fmt.Println(result) // Prints: Lorca
- Binding Go functions to JavaScript:
ui, _ := lorca.New("", "", 480, 320)
defer ui.Close()
ui.Bind("greet", func(name string) string {
return fmt.Sprintf("Hello, %s!", name)
})
ui.Load("data:text/html,<html><body><script>alert(greet('World'))</script></body></html>")
<-ui.Done()
Getting Started
To use Lorca in your Go project, follow these steps:
-
Install Lorca:
go get github.com/zserge/lorca
-
Import Lorca in your Go file:
import "github.com/zserge/lorca"
-
Create a new UI instance:
ui, _ := lorca.New("", "", 800, 600) defer ui.Close()
-
Load your HTML content and start the application:
ui.Load("http://localhost:8080") <-ui.Done()
Make sure Chrome is installed on your system before running the application.
Competitor Comparisons
:electron: Build cross-platform desktop apps with JavaScript, HTML, and CSS
Pros of Electron
- Extensive ecosystem and community support
- Cross-platform compatibility (Windows, macOS, Linux)
- Rich set of APIs for native OS integration
Cons of Electron
- Large application size due to bundled Chromium and Node.js
- Higher memory usage compared to native applications
- Potential security concerns due to full Node.js access
Code Comparison
Electron:
const { app, BrowserWindow } = require('electron')
function createWindow () {
const win = new BrowserWindow({ width: 800, height: 600 })
win.loadFile('index.html')
}
app.whenReady().then(createWindow)
Lorca:
ui, _ := lorca.New("", "", 480, 320)
defer ui.Close()
ui.Bind("add", func(a, b int) int { return a + b })
ui.Load("data:text/html,<html><body><h1>Hello World</h1></body></html>")
<-ui.Done()
Key Differences
- Lorca uses the system-installed Chrome/Chromium browser, resulting in smaller app sizes
- Electron provides a more comprehensive set of APIs for desktop integration
- Lorca is Go-based, while Electron uses JavaScript/Node.js
- Electron has a larger learning curve but offers more flexibility for complex applications
- Lorca is lightweight and suitable for simpler applications with minimal native integration needs
Build smaller, faster, and more secure desktop and mobile applications with a web frontend.
Pros of Tauri
- Multi-platform support (Windows, macOS, Linux) out of the box
- Smaller bundle sizes due to native system webviews
- Robust security features and customizable permissions
Cons of Tauri
- Steeper learning curve, especially for developers new to Rust
- Slower development cycle compared to Lorca's simplicity
- Limited to using system webviews, which may have inconsistencies across platforms
Code Comparison
Lorca (Go):
ui, _ := lorca.New("", "", 480, 320)
defer ui.Close()
ui.Bind("add", add)
<-ui.Done()
Tauri (Rust):
fn main() {
tauri::Builder::default()
.setup(|app| {
// Setup code here
Ok(())
})
.run(tauri::generate_context!())
.expect("error while running tauri application");
}
Both Lorca and Tauri aim to create desktop applications using web technologies, but they differ in approach and features. Lorca focuses on simplicity and ease of use, leveraging Go and Chrome/Chromium. Tauri offers a more comprehensive framework with Rust, emphasizing security and performance. While Lorca is quicker to get started with, Tauri provides more robust features for production-ready applications.
Create beautiful applications using Go
Pros of Wails
- Supports multiple programming languages (Go, JavaScript, TypeScript)
- Offers a more comprehensive framework with built-in tooling and CLI
- Provides native OS dialogs and menus integration
Cons of Wails
- Larger footprint and more dependencies
- Steeper learning curve due to more complex architecture
- May have slower startup times compared to Lorca
Code Comparison
Lorca:
ui, _ := lorca.New("", "", 480, 320)
defer ui.Close()
ui.Bind("add", add)
ui.Load("index.html")
<-ui.Done()
Wails:
app := wails.CreateApp(&wails.AppConfig{
Width: 480,
Height: 320,
})
app.Bind(add)
err := app.Run()
Key Differences
- Lorca uses Chrome/Chromium installed on the system, while Wails bundles a WebView2 runtime
- Wails offers more extensive features and customization options
- Lorca is lighter and simpler, focusing on quick prototyping and small applications
- Wails provides better support for creating production-ready desktop applications
Both projects aim to simplify the creation of desktop applications using web technologies and Go, but they cater to different use cases and development preferences.
Portable and lightweight cross-platform desktop application development framework
Pros of Neutralinojs
- Cross-platform support for Windows, macOS, and Linux
- Built-in API for file system operations, OS-level functions, and more
- Smaller application size compared to Electron-based apps
Cons of Neutralinojs
- Requires separate installation of the Neutralino runtime
- Less mature ecosystem and community support than Go-based solutions
- Limited access to native OS features compared to Lorca
Code Comparison
Neutralinojs:
Neutralino.init();
Neutralino.window.setTitle("My App");
Neutralino.os.showMessageBox("Hello", "Welcome to Neutralino!");
Lorca:
ui, _ := lorca.New("", "", 480, 320)
defer ui.Close()
ui.Bind("hello", func() string { return "Hello, World!" })
<-ui.Done()
Neutralinojs uses JavaScript for both frontend and backend logic, while Lorca leverages Go for the backend and HTML/CSS/JavaScript for the frontend. Neutralinojs provides a more integrated API for common desktop application tasks, whereas Lorca offers tighter integration with the Go ecosystem and native OS capabilities through CGo.
Both frameworks aim to simplify cross-platform desktop app development, but they cater to different developer preferences and use cases. Neutralinojs is more suitable for web developers looking for a lightweight alternative to Electron, while Lorca is ideal for Go developers who want to create desktop UIs using web technologies.
Tiny cross-platform webview library for C/C++. Uses WebKit (GTK/Cocoa) and Edge WebView2 (Windows).
Pros of webview
- Supports multiple programming languages (C/C++, Go, Rust, Python, etc.)
- Provides a native OS window with web content
- Smaller binary size and lower memory usage
Cons of webview
- Less feature-rich compared to Lorca
- Requires more setup and configuration
- Limited JavaScript interop capabilities
Code Comparison
webview
package main
import "github.com/webview/webview"
func main() {
w := webview.New(true)
defer w.Destroy()
w.SetTitle("Webview Example")
w.SetSize(800, 600, webview.HintNone)
w.Navigate("https://example.com")
w.Run()
}
Lorca
package main
import "github.com/zserge/lorca"
func main() {
ui, _ := lorca.New("", "", 800, 600)
defer ui.Close()
ui.Load("https://example.com")
<-ui.Done()
}
Key Differences
- Lorca uses Chrome/Chromium as the rendering engine, while webview uses the native WebView component
- Lorca provides easier JavaScript interop and DOM manipulation
- webview offers broader language support and smaller footprint
- Lorca focuses on Go, while webview caters to multiple languages
- webview provides more control over the native window properties
Both libraries aim to create desktop applications with web technologies, but they differ in their approach, feature set, and target audience.
Make any web page a desktop application
Pros of Nativefier
- Cross-platform support for Windows, macOS, and Linux
- Extensive customization options for the generated app
- Ability to package any web application as a desktop app
Cons of Nativefier
- Larger app size due to bundling Electron
- Potentially higher resource usage compared to native apps
- Limited access to system-level APIs
Code Comparison
Nativefier (JavaScript):
nativefier('https://example.com', {
name: 'My App',
icon: 'path/to/icon.png',
width: 1280,
height: 800
});
Lorca (Go):
ui, _ := lorca.New("https://example.com", "", 800, 600)
defer ui.Close()
<-ui.Done()
Key Differences
Nativefier is a more feature-rich solution for creating desktop apps from web applications, offering extensive customization options and cross-platform support. However, it results in larger app sizes and potentially higher resource usage due to its reliance on Electron.
Lorca, on the other hand, provides a lightweight alternative using the system's installed browser, resulting in smaller app sizes and potentially better performance. However, it offers fewer customization options and may have limited cross-platform compatibility compared to Nativefier.
Choose Nativefier for a more robust, customizable solution with broader platform support, or Lorca for a lightweight, performance-focused approach that leverages the system's existing browser.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Lorca
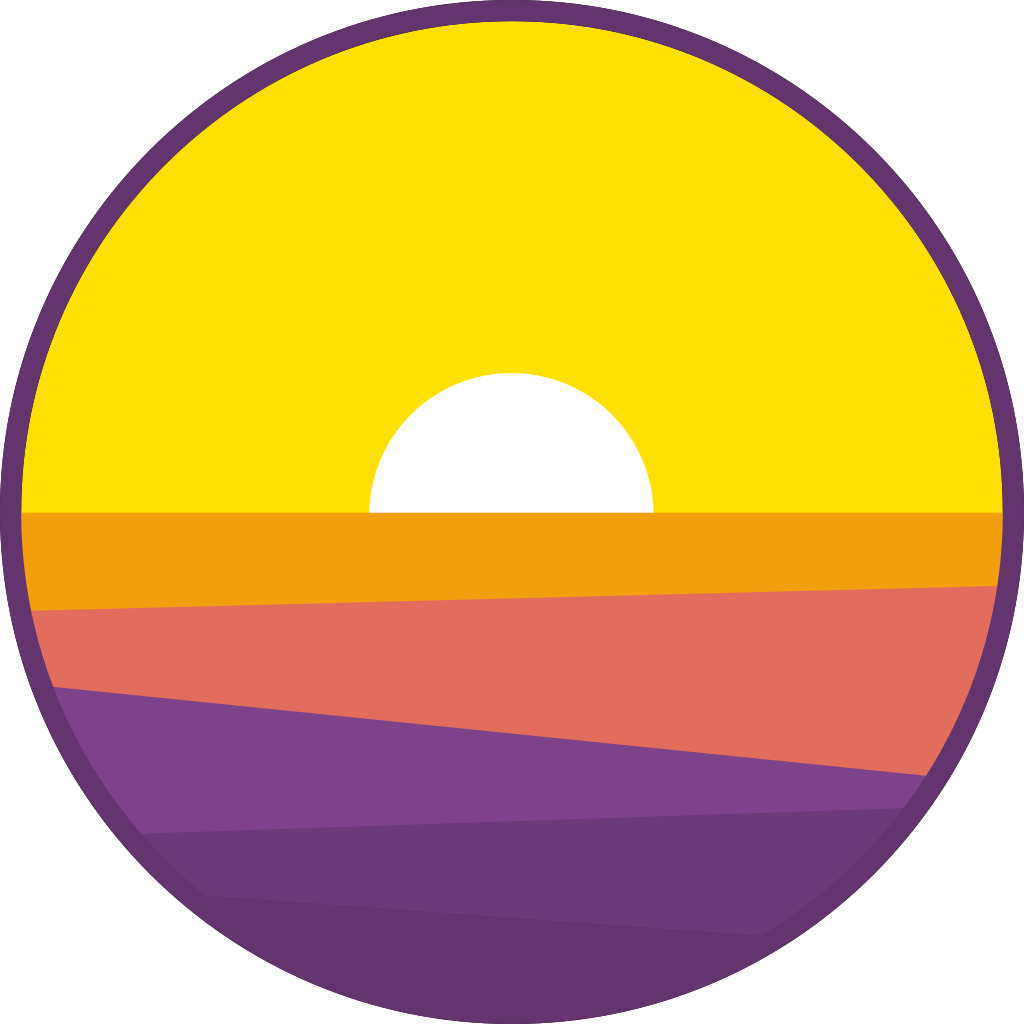
A very small library to build modern HTML5 desktop apps in Go. It uses Chrome browser as a UI layer. Unlike Electron it doesn't bundle Chrome into the app package, but rather reuses the one that is already installed. Lorca establishes a connection to the browser window and allows calling Go code from the UI and manipulating UI from Go in a seamless manner.
Features
- Pure Go library (no cgo) with a very simple API
- Small application size (normally 5-10MB)
- Best of both worlds - the whole power of HTML/CSS to make your UI look good, combined with Go performance and ease of development
- Expose Go functions/methods and call them from JavaScript
- Call arbitrary JavaScript code from Go
- Asynchronous flow between UI and main app in both languages (async/await and Goroutines)
- Supports loading web UI from the local web server or via data URL
- Supports testing your app with the UI in the headless mode
- Supports multiple app windows
- Supports packaging and branding (e.g. custom app icons). Packaging for all three OS can be done on a single machine using GOOS and GOARCH variables.
Also, limitations by design:
- Requires Chrome/Chromium >= 70 to be installed.
- No control over the Chrome window yet (e.g. you can't remove border, make it transparent, control position or size).
- No window menu (tray menus and native OS dialogs are still possible via 3rd-party libraries)
If you want to have more control of the browser window - consider using webview library with a similar API, so migration would be smooth.
Example
ui, _ := lorca.New("", "", 480, 320)
defer ui.Close()
// Bind Go function to be available in JS. Go function may be long-running and
// blocking - in JS it's represented with a Promise.
ui.Bind("add", func(a, b int) int { return a + b })
// Call JS function from Go. Functions may be asynchronous, i.e. return promises
n := ui.Eval(`Math.random()`).Float()
fmt.Println(n)
// Call JS that calls Go and so on and so on...
m := ui.Eval(`add(2, 3)`).Int()
fmt.Println(m)
// Wait for the browser window to be closed
<-ui.Done()
Also, see examples for more details about binding functions and packaging binaries.
Hello World
Here are the steps to run the hello world example.
cd examples/counter
go get
go run ./
How it works
Under the hood Lorca uses Chrome DevTools Protocol to instrument on a Chrome instance. First Lorca tries to locate your installed Chrome, starts a remote debugging instance binding to an ephemeral port and reads from stderr
for the actual WebSocket endpoint. Then Lorca opens a new client connection to the WebSocket server, and instruments Chrome by sending JSON messages of Chrome DevTools Protocol methods via WebSocket. JavaScript functions are evaluated in Chrome, while Go functions actually run in Go runtime and returned values are sent to Chrome.
What's in a name?
There is kind of a legend, that before his execution Garcia Lorca have seen a sunrise over the heads of the soldiers and he said "And yet, the sun rises...". Probably it was the beginning of a poem. (J. Brodsky)
Lorca is an anagram of Carlo, a project with a similar goal for Node.js.
License
Code is distributed under MIT license, feel free to use it in your proprietary projects as well.
Top Related Projects
:electron: Build cross-platform desktop apps with JavaScript, HTML, and CSS
Build smaller, faster, and more secure desktop and mobile applications with a web frontend.
Create beautiful applications using Go
Portable and lightweight cross-platform desktop application development framework
Tiny cross-platform webview library for C/C++. Uses WebKit (GTK/Cocoa) and Edge WebView2 (Windows).
Make any web page a desktop application
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot