ChatGPT-wechat-bot
ChatGPT for wechat https://github.com/AutumnWhj/ChatGPT-wechat-bot
Top Related Projects
🤖一个基于 WeChaty 结合 DeepSeek / ChatGPT / Kimi / 讯飞等Ai服务实现的微信机器人 ,可以用来帮助你自动回复微信消息,或者管理微信群/好友,检测僵尸粉等...
Use ChatGPT On Wechat via wechaty
基于大模型搭建的聊天机器人,同时支持 微信公众号、企业微信应用、飞书、钉钉 等接入,可选择GPT4.1/GPT-4o/GPT-o1/ DeepSeek/Claude/文心一言/讯飞星火/通义千问/ Gemini/GLM-4/Kimi/LinkAI,能处理文本、语音和图片,访问操作系统和互联网,支持基于自有知识库进行定制企业智能客服。
Quick Overview
ChatGPT-wechat-bot is a project that integrates ChatGPT with WeChat, allowing users to interact with ChatGPT through their WeChat accounts. It provides a bridge between the popular messaging platform and OpenAI's language model, enabling automated responses and conversations within WeChat.
Pros
- Seamless integration of ChatGPT with WeChat, a widely used messaging platform
- Supports multiple ChatGPT API providers, including OpenAI and Azure
- Customizable conversation settings and user management
- Easy deployment using Docker
Cons
- Limited documentation, which may make setup and customization challenging for some users
- Potential issues with WeChat's anti-bot measures, requiring careful configuration
- Relies on third-party libraries that may need regular updates for compatibility
- May require additional costs for API usage depending on the chosen provider
Code Examples
- Setting up the ChatGPT configuration:
chatgpt_config = {
"api_key": "your-api-key",
"model": "gpt-3.5-turbo",
"temperature": 0.7,
"max_tokens": 1000,
"top_p": 1,
"frequency_penalty": 0,
"presence_penalty": 0,
}
- Initializing the WeChat bot:
from wechat_bot import WeChatBot
bot = WeChatBot(chatgpt_config)
bot.run()
- Customizing response handling:
@bot.handler
def on_message(message):
if message.type == 'text':
response = bot.get_chatgpt_response(message.content)
message.reply(response)
Getting Started
-
Clone the repository:
git clone https://github.com/AutumnWhj/ChatGPT-wechat-bot.git cd ChatGPT-wechat-bot
-
Install dependencies:
pip install -r requirements.txt
-
Configure the bot:
- Copy
config.yaml.example
toconfig.yaml
- Edit
config.yaml
with your API keys and settings
- Copy
-
Run the bot:
python main.py
-
Scan the QR code with your WeChat account to log in.
Competitor Comparisons
🤖一个基于 WeChaty 结合 DeepSeek / ChatGPT / Kimi / 讯飞等Ai服务实现的微信机器人 ,可以用来帮助你自动回复微信消息,或者管理微信群/好友,检测僵尸粉等...
Pros of wechat-bot
- More comprehensive documentation, including detailed setup instructions and usage examples
- Supports multiple AI models (ChatGPT, Claude, Stable Diffusion) for diverse functionality
- Includes features like image generation and voice message processing
Cons of wechat-bot
- More complex setup process due to additional dependencies and configurations
- Potentially higher resource usage due to multiple AI model integrations
- Less focused on a single use case (ChatGPT integration) compared to ChatGPT-wechat-bot
Code Comparison
ChatGPT-wechat-bot:
const { WechatyBuilder } = require('wechaty');
const qrcodeTerminal = require('qrcode-terminal');
const ChatGPTBot = require('./bot');
wechat-bot:
import { WechatyBuilder } from 'wechaty';
import { FileBox } from 'file-box';
import { ChatGPTBot } from './bot.js';
import { config } from './config.js';
Both projects use Wechaty for WeChat integration, but wechat-bot imports additional modules for enhanced functionality. ChatGPT-wechat-bot uses CommonJS module syntax, while wechat-bot uses ES6 import statements.
Use ChatGPT On Wechat via wechaty
Pros of wechat-chatgpt
- More active development with frequent updates and contributions
- Supports multiple AI models including ChatGPT, GPT-3.5-turbo, and Claude
- Offers Docker support for easier deployment
Cons of wechat-chatgpt
- More complex setup process with additional dependencies
- Lacks some features like customizable welcome messages and multi-user support
- May have higher resource requirements due to additional functionalities
Code Comparison
ChatGPT-wechat-bot:
const { WechatyBuilder } = require('wechaty');
const qrcodeTerminal = require('qrcode-terminal');
const ChatGPTBot = require('./bot');
const bot = new ChatGPTBot();
wechat-chatgpt:
import { WechatyBuilder } from 'wechaty';
import { ChatGPTBot } from './chatgpt';
import { Config } from './config';
const chatGPTBot = new ChatGPTBot();
Both projects use Wechaty for WeChat integration, but wechat-chatgpt employs TypeScript and has a more modular structure. ChatGPT-wechat-bot uses JavaScript and has a simpler implementation.
While ChatGPT-wechat-bot focuses solely on ChatGPT integration, wechat-chatgpt offers more flexibility with multiple AI model support. However, this comes at the cost of increased complexity in setup and configuration.
ChatGPT-wechat-bot may be more suitable for users seeking a straightforward ChatGPT integration, while wechat-chatgpt caters to those requiring advanced features and multiple AI model options.
基于大模型搭建的聊天机器人,同时支持 微信公众号、企业微信应用、飞书、钉钉 等接入,可选择GPT4.1/GPT-4o/GPT-o1/ DeepSeek/Claude/文心一言/讯飞星火/通义千问/ Gemini/GLM-4/Kimi/LinkAI,能处理文本、语音和图片,访问操作系统和互联网,支持基于自有知识库进行定制企业智能客服。
Pros of chatgpt-on-wechat
- More comprehensive documentation, including detailed setup instructions and configuration options
- Supports multiple AI platforms (OpenAI, Azure, Claude) and chat modes (e.g., continuous dialogue, image generation)
- Active development with frequent updates and a larger community (3.7k+ stars)
Cons of chatgpt-on-wechat
- More complex setup process due to additional features and dependencies
- Potentially higher resource usage and slower response times in some scenarios
- Steeper learning curve for users unfamiliar with Python and AI integrations
Code Comparison
ChatGPT-wechat-bot (main.py):
@itchat.msg_register(itchat.content.TEXT)
def reply_text(msg):
message = msg['Text']
response = chatgpt.get_chat_response(message)
return response
chatgpt-on-wechat (channel/wechat/wechat_channel.py):
def handle_text(self, msg):
context = Context(channel_type=ChannelType.WECHAT)
context.message = msg.content
context.ctype = ContextType.TEXT
reply = super().build_reply_content(context)
return reply
The chatgpt-on-wechat code demonstrates a more modular approach with better separation of concerns, while ChatGPT-wechat-bot offers a simpler implementation.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
ChatGPT-wechat-botð¤
å æ¥å³å¯è·å¾ä¸ä¸ªåºäº ChatGPT ç微信æºå¨äºº ð¤ã English | ä¸æææ¡£
Support
- æ¯æä¸ä¸æè¯å¢ç对è¯ã
- æ¯æéç½®ä¸ä¸æè¯å¢ï¼éè¿å ³é®è¯(reset)é置对è¯ä¸ä¸æè¯å¢ã
- æ¯æå¨ç¾¤è@ä½ çæºå¨äºº ð¤ï¼@æºå¨äººå³å¯æ¶å°åå¤ã
- æ¯æéè¿å ³é®è¯å¤éä½ çæºå¨äººï¼å¦å½å¨ç¾¤ç»ä¸åéâ@æºå¨äºº hello xxxxâæ¶æä¼æ¶å°åå¤ã
- å ¶ä»
åä½
é»è®¤é ç½®
{
// å¡«å
¥ä½ çOPENAI_API_KEY
OPENAI_API_KEY: "",
// åå代çå°åï¼ç®å说就æ¯ä½ çå¨å½å¤æå¡å¨å°åï¼å¦ä½è·åçREADME
reverseProxyUrl: "",
// å¨ç¾¤ç»ä¸è®¾ç½®å¤é微信æºå¨äººçå
³é®è¯
groupKey: "",
// å¨ç§èä¸è®¾ç½®å¤é微信æºå¨äººçå
³é®è¯
privateKey: "",
// éç½®ä¸ä¸æçå
³é®è¯ï¼å¦å¯è®¾ç½®ä¸ºreset
resetKey: "reset",
// æ¯å¦å¨ç¾¤èä¸å¸¦ä¸æé®çé®é¢
groupReplyMode: true,
// æ¯å¦å¨ç§èä¸å¸¦ä¸æé®çé®é¢
privateReplyMode: false,
}
å¼å§è®¾ç½®æºå¨äºº ð¤
- é¦å ï¼éè¦æç §ä»¥ä¸æ¥éª¤è·ä½ ç ChatGPT ç OPENAI_API_KEY.
è·åä½ ç OPENAI_API_KEY:
- æå¼ https://platform.openai.com/overview å¹¶ç»å½æ³¨åï¼è¿å ¥ç½é¡µã
-
æ OPENAI_API_KEY å¡«å ¥ç®å½
src/config.ts
ä¸çOPENAI_API_KEY
ä¸ -
æ reverseProxyUrl å¡«å ¥ç®å½
src/config.ts
ä¸çreverseProxyUrl
ä¸ï¼å¦ä½è®¾ç½®å¯çä¸é¢ä»ç»ã
å½ç¶ä¹å¯ä»¥éæ©ç½å«å±±æèå¸ç代çå°åï¼
https://ai.devtool.tech/proxy/v1/chat/completions
ï¼å¯ä»¥å ³æ³¨ä»ç项ç®
- ç¶åå¨ç»ç«¯è¿è¡ä»¥ä¸å½ä»¤ã妿éè¦ï¼è¯·å¨
src/config.ts
ä¸é ç½®å ¶å®é ç½®åéã
// å®è£
ä¾èµ
npm i
npm run dev
// ä¹å¯ä»¥ä½¿ç¨pnpm
npm i -g pnpm
pnpm i
pnpm run dev
-
æ§è¡å®ä¹åï¼å¯ä»¥çå°ç»ç«¯æ§å¶å°è¾åºä¸ä¸ä¿¡æ¯ï¼æ«ç ç»å½å³å¯.
-
ç»å½æåï¼ç¨å¦å¤ä¸ä¸ªå¾®ä¿¡å¾ä½ æ«ç ç»å½çå¾®ä¿¡åæ¶æ¯ï¼ä½ å°ä¼æ¶å°æ¥èª ChatGPT çåå¤ã
设置åå代çå°å
ChatGPT API 代çhttps://hub.docker.com/r/mirrors2/chatgpt-api-proxy
chatgpt api 代ç,å·²éªè¯ OpenCat,AssisChat,AMA(é®å¤©),chathub
å¯é 置好 OPENAI_API_KEY å享代çå°åç»ä»äººç¨.
å¿«éå¼å§
docker run -d -p 80:80 --name chatgpt-api-proxy mirrors2/chatgpt-api-proxy
# å¯é -e OPENAI_API_KEY={nide_api_key}
docker è·èµ·æ¥ä¹åä½ ç代çå°åå°±çæäºï¼
宿¹çï¼https://api.openai.com/v1/chat/completions
ä½ çï¼ ä½ çåå/v1/chat/completions
æè
ä½ çæå¡å¨ipå端å£/v1/chat/completions
QA
-
å¾®ä¿¡æ æ³åæ¶ç»å½é®é¢ å¯ä»¥ç´æ¥å é¤
WechatEveryDay.memory-card
æä»¶ï¼éæ°è·ç¨åº -
æ¯æç node çæ¬: Node.js >= 16.8
-
å 为 ChatGPT çé¿åº¦éå¶ï¼å¦æå夿¶æ¯ä¸å®æ´ï¼å¯ä»¥å¯¹å®è¯´"请继ç»" æè "请继ç»åå®"ã
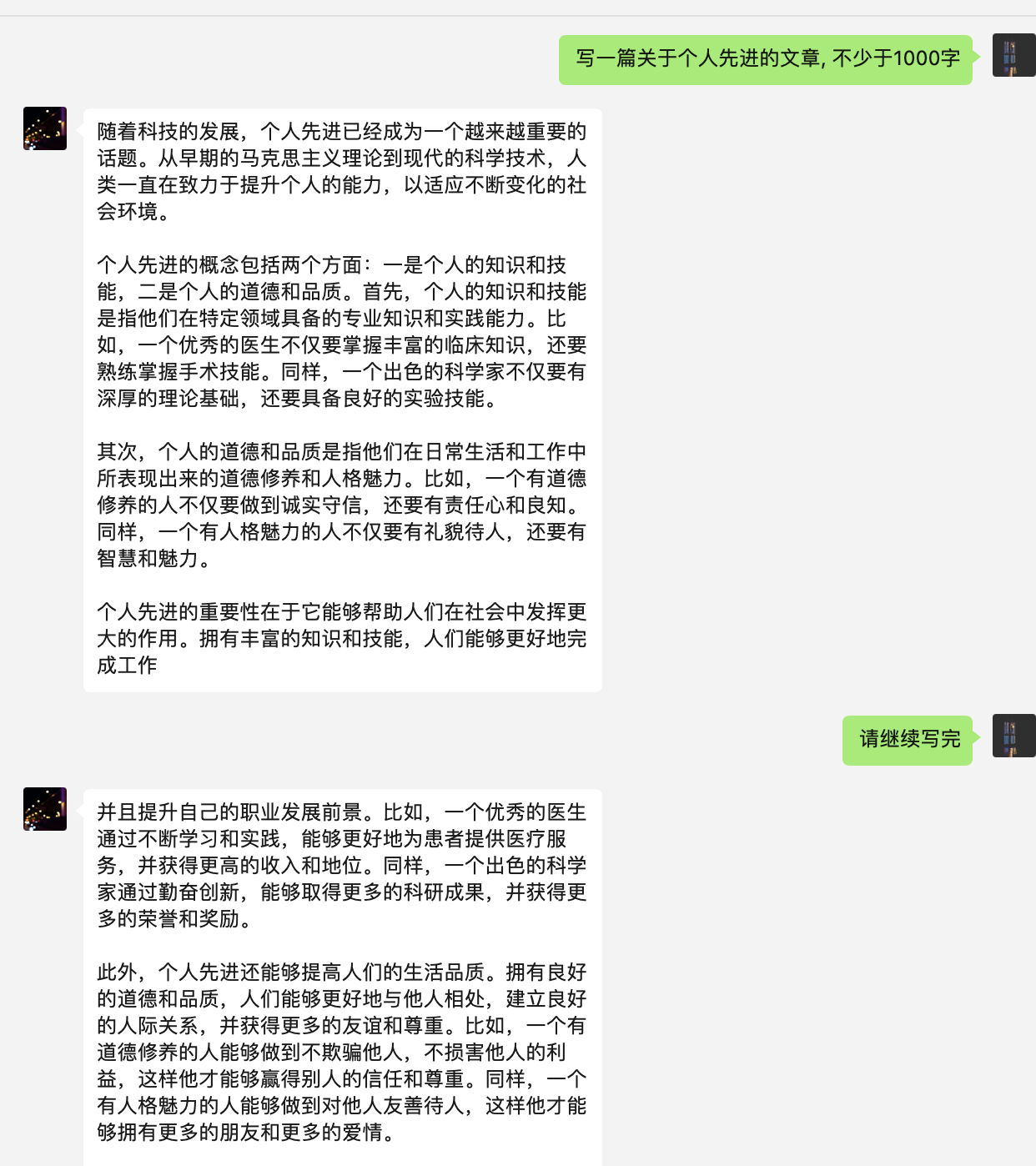
- Error: Failed to launch the browser process puppeteer refer to https://github.com/puppeteer/puppeteer/blob/main/docs/troubleshooting.md#chrome-headless-doesnt-launch-on-unix
# ubuntu
sudo apt-get install chromium-browser
sudo apt-get install ca-certificates fonts-liberation libasound2 libatk-bridge2.0-0 libatk1.0-0 libc6 libcairo2 libcups2 libdbus-1-3 libexpat1 libfontconfig1 libgbm1 libgcc1 libglib2.0-0 libgtk-3-0 libnspr4 libnss3 libpango-1.0-0 libpangocairo-1.0-0 libstdc++6 libx11-6 libx11-xcb1 libxcb1 libxcomposite1 libxcursor1 libxdamage1 libxext6 libxfixes3 libxi6 libxrandr2 libxrender1 libxss1 libxtst6 lsb-release wget xdg-utils
ðð» æ¬¢è¿ä¸èµ·å ±å»º
欢è¿è´¡ç®ä½ ç代ç ä»¥åæ³æ³ ðµã
Top Related Projects
🤖一个基于 WeChaty 结合 DeepSeek / ChatGPT / Kimi / 讯飞等Ai服务实现的微信机器人 ,可以用来帮助你自动回复微信消息,或者管理微信群/好友,检测僵尸粉等...
Use ChatGPT On Wechat via wechaty
基于大模型搭建的聊天机器人,同时支持 微信公众号、企业微信应用、飞书、钉钉 等接入,可选择GPT4.1/GPT-4o/GPT-o1/ DeepSeek/Claude/文心一言/讯飞星火/通义千问/ Gemini/GLM-4/Kimi/LinkAI,能处理文本、语音和图片,访问操作系统和互联网,支持基于自有知识库进行定制企业智能客服。
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot