Top Related Projects
Quick Overview
Element is a popular Vue.js 2.0 UI toolkit for building responsive, mobile-first web interfaces. It provides a rich set of customizable components and is widely used in both personal and enterprise-level projects. Element aims to improve development efficiency and create a consistent user experience across applications.
Pros
- Comprehensive component library with a wide range of UI elements
- Extensive documentation and active community support
- Customizable themes and styles to match brand requirements
- Internationalization support for multiple languages
Cons
- Primarily designed for Vue.js 2.0, which may not be ideal for newer Vue 3 projects
- Large bundle size if importing the entire library, which can impact performance
- Some components may have limited customization options
- Learning curve for developers new to Vue.js or component-based UI libraries
Code Examples
- Basic Button Component:
<template>
<el-button type="primary" @click="handleClick">Click me</el-button>
</template>
<script>
export default {
methods: {
handleClick() {
console.log('Button clicked!');
}
}
}
</script>
- Form with Validation:
<template>
<el-form :model="form" :rules="rules" ref="form">
<el-form-item label="Name" prop="name">
<el-input v-model="form.name"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm">Submit</el-button>
</el-form-item>
</el-form>
</template>
<script>
export default {
data() {
return {
form: { name: '' },
rules: {
name: [{ required: true, message: 'Please input your name', trigger: 'blur' }]
}
}
},
methods: {
submitForm() {
this.$refs.form.validate((valid) => {
if (valid) {
console.log('Form submitted');
}
});
}
}
}
</script>
- Table with Pagination:
<template>
<el-table :data="tableData" style="width: 100%">
<el-table-column prop="name" label="Name"></el-table-column>
<el-table-column prop="age" label="Age"></el-table-column>
</el-table>
<el-pagination
@current-change="handleCurrentChange"
:current-page="currentPage"
:page-size="10"
:total="100">
</el-pagination>
</template>
<script>
export default {
data() {
return {
tableData: [
{ name: 'John', age: 20 },
{ name: 'Jane', age: 24 },
// ... more data
],
currentPage: 1
}
},
methods: {
handleCurrentChange(val) {
this.currentPage = val;
// Fetch data for the new page
}
}
}
</script>
Getting Started
- Install Element UI in your Vue.js project:
npm install element-ui
- Import and use Element UI in your main.js file:
import Vue from 'vue'
import ElementUI from 'element-ui'
import 'element-ui/lib/theme-chalk/index.css'
import App from './App.vue'
Vue.use(ElementUI)
new Vue({
el: '#app',
render: h => h(App)
})
- Start using Element components in your Vue templates:
<template>
<el-button>Hello Element UI</el-button>
</template>
Competitor Comparisons
🐉 Vue Component Framework
Pros of Vuetify
- More comprehensive component library with a wider range of UI elements
- Better support for material design principles and aesthetics
- More active community and frequent updates
Cons of Vuetify
- Larger bundle size, which may impact initial load times
- Steeper learning curve due to more complex API and configuration options
Code Comparison
Element:
<el-button type="primary">Primary Button</el-button>
<el-input v-model="input" placeholder="Please input"></el-input>
Vuetify:
<v-btn color="primary">Primary Button</v-btn>
<v-text-field v-model="input" label="Please input"></v-text-field>
Both Element and Vuetify are popular Vue.js UI component libraries, but they have different focuses and strengths. Element provides a clean and minimalistic design approach, while Vuetify offers a more feature-rich and material design-oriented solution. Element may be easier to get started with for beginners, but Vuetify provides more advanced customization options and a wider range of components. The choice between the two often depends on project requirements, design preferences, and development team familiarity.
Quasar Framework - Build high-performance VueJS user interfaces in record time
Pros of Quasar
- Cross-platform development: Supports web, mobile, and desktop from a single codebase
- Rich ecosystem: Includes CLI, dev tools, and extensive component library
- Performance-focused: Optimized for speed and minimal bundle size
Cons of Quasar
- Steeper learning curve: More complex due to its comprehensive nature
- Less widespread adoption: Smaller community compared to Element
- Opinionated structure: May be less flexible for some projects
Code Comparison
Element (Vue.js component):
<template>
<el-button type="primary" @click="handleClick">Click me</el-button>
</template>
<script>
export default {
methods: {
handleClick() {
console.log('Button clicked')
}
}
}
</script>
Quasar (Vue.js component):
<template>
<q-btn color="primary" @click="handleClick">Click me</q-btn>
</template>
<script>
export default {
methods: {
handleClick() {
console.log('Button clicked')
}
}
}
</script>
Both frameworks offer similar component-based structures, but Quasar's syntax is more concise and uses its own prefix (q-
) for components. Element follows a more traditional Vue.js approach with the el-
prefix. Quasar's components often have built-in features that may require additional configuration in Element.
Lightweight UI components for Vue.js based on Bulma
Pros of Buefy
- Lightweight and modular, with a smaller bundle size
- Closer integration with Bulma CSS framework
- Simpler API and easier learning curve for beginners
Cons of Buefy
- Fewer components and features compared to Element
- Less extensive documentation and community resources
- Not as widely adopted in large-scale enterprise projects
Code Comparison
Element component usage:
<el-button type="primary" @click="handleClick">
Click me
</el-button>
Buefy component usage:
<b-button type="is-primary" @click="handleClick">
Click me
</b-button>
Both libraries offer similar component structures, but Buefy follows Bulma's naming conventions more closely (e.g., is-primary
instead of just primary
).
Element provides more customization options out of the box, while Buefy relies more on Bulma's utility classes for styling. Element's ecosystem is larger, with more third-party components and plugins available. However, Buefy's simplicity and lightweight nature make it an attractive option for smaller projects or those already using Bulma.
Next Generation Vue UI Component Library
Pros of PrimeVue
- More comprehensive component library with 90+ UI components
- Better TypeScript support and integration
- Regular updates and active community support
Cons of PrimeVue
- Steeper learning curve due to more complex API
- Larger bundle size compared to Element
Code Comparison
PrimeVue:
<template>
<Button label="Submit" icon="pi pi-check" />
</template>
<script>
import Button from 'primevue/button';
export default {
components: { Button }
}
</script>
Element:
<template>
<el-button type="primary" icon="el-icon-check">Submit</el-button>
</template>
<script>
import { ElButton } from 'element-plus'
export default {
components: { ElButton }
}
</script>
Summary
PrimeVue offers a more extensive component library and better TypeScript support, making it suitable for large-scale applications. However, it has a steeper learning curve and larger bundle size. Element, on the other hand, provides a simpler API and smaller bundle size, making it easier to get started with but potentially limiting for complex projects. The choice between the two depends on project requirements and developer preferences.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
A Vue.js 2.0 UI Toolkit for Web.
Element will stay with Vue 2.x
For Vue 3.0, we recommend using Element Plus(Element Plus is a community develop project)
For MiniProgram development, we recommend using MorJS
Links
- Homepage and documentation
- awesome-element
- FAQ
- Vue.js 3.0 migration
- Customize theme
- Preview and generate theme online
- Element for React
- Element for Angular
- Atom helper
- Visual Studio Code helper
- Starter kit
- Design resources
- Gitter
Install
npm install element-ui -S
Quick Start
import Vue from 'vue'
import Element from 'element-ui'
Vue.use(Element)
// or
import {
Select,
Button
// ...
} from 'element-ui'
Vue.component(Select.name, Select)
Vue.component(Button.name, Button)
For more information, please refer to Quick Start in our documentation.
Browser Support
Modern browsers and Internet Explorer 10+.
Development
Skip this part if you just want to use Element.
For those who are interested in contributing to Element, please refer to our contributing guide (䏿 | English | Español | Français) to see how to run this project.
Changelog
Detailed changes for each release are documented in the release notes.
FAQ
We have collected some frequently asked questions. Before reporting an issue, please search if the FAQ has the answer to your problem.
Contribution
Please make sure to read the contributing guide (䏿 | English | Español | Français) before making a pull request.
Special Thanks
English documentation is brought to you by SwiftGG Translation Team:
Spanish documentation is made possible by these community developers:
- adavie1
- carmencitaqiu
- coderdiaz
- fedegar33
- Gonzalo2310
- lesterbx
- ProgramerGuy
- SantiagoGdaR
- sigfriedCub1990
- thechosenjuan
French documentation is made possible by these community developers:
Join Discussion Group
Scan the QR code using Dingtalk App to join in discussion group :
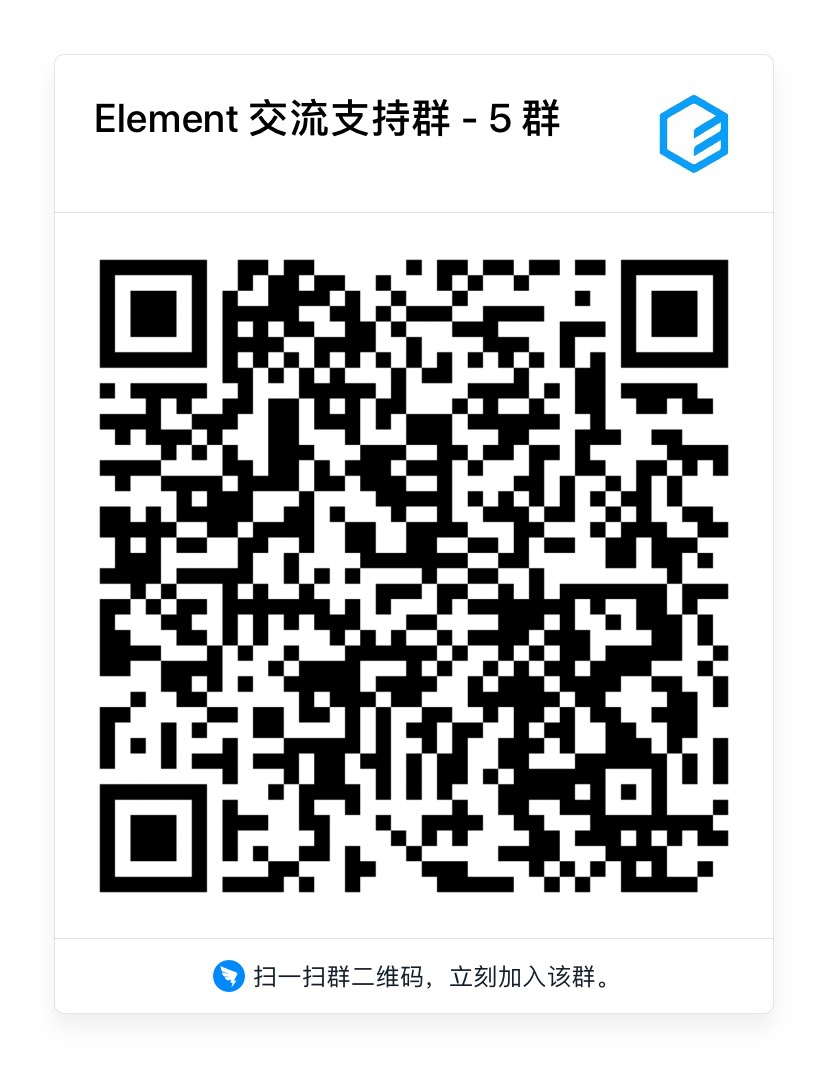
LICENSE
Top Related Projects
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot