Top Related Projects
Complete Ethereum library and wallet implementation in JavaScript.
Collection of comprehensive TypeScript libraries for Interaction with the Ethereum JSON RPC API and utility functions.
:warning: The Truffle Suite is being sunset. For information on ongoing support, migration options and FAQs, visit the Consensys blog. Thank you for all the support over the years.
Hardhat is a development environment to compile, deploy, test, and debug your Ethereum software.
Quick Overview
FuelLabs/fuels-ts is a TypeScript SDK for interacting with the Fuel blockchain. It provides a comprehensive set of tools and utilities for developers to build decentralized applications (dApps) on the Fuel network, offering seamless integration with Fuel's unique features and capabilities.
Pros
- Strongly typed and well-documented, enhancing developer experience and reducing errors
- Supports both browser and Node.js environments, allowing for versatile application development
- Includes a wide range of utilities for common blockchain operations, such as wallet management and contract interactions
- Actively maintained and regularly updated, ensuring compatibility with the latest Fuel network features
Cons
- Specific to the Fuel blockchain, limiting its use for developers working on other networks
- Learning curve may be steep for developers new to Fuel's unique architecture
- Documentation, while comprehensive, may be overwhelming for beginners
- Limited ecosystem compared to more established blockchain platforms
Code Examples
- Connecting to a Fuel node:
import { Wallet } from 'fuels';
const wallet = Wallet.fromPrivateKey('0x...your-private-key-here...');
console.log('Address:', wallet.address.toString());
- Deploying a contract:
import { Wallet, Contract, ContractFactory } from 'fuels';
const wallet = Wallet.fromPrivateKey('0x...your-private-key-here...');
const factory = new ContractFactory(contractABI, contractBytecode, wallet);
const contract = await factory.deployContract();
console.log('Contract deployed at:', contract.id.toString());
- Calling a contract function:
import { Wallet, Contract, BN } from 'fuels';
const wallet = Wallet.fromPrivateKey('0x...your-private-key-here...');
const contract = new Contract(contractAddress, contractABI, wallet);
const result = await contract.functions.transfer(recipientAddress, new BN(100)).call();
console.log('Transfer result:', result);
Getting Started
To start using fuels-ts in your project:
-
Install the package:
npm install fuels
-
Import and use in your TypeScript/JavaScript project:
import { Wallet } from 'fuels'; const wallet = Wallet.generate(); console.log('New wallet address:', wallet.address.toString());
-
Refer to the official documentation for more detailed usage instructions and examples.
Competitor Comparisons
Complete Ethereum library and wallet implementation in JavaScript.
Pros of ethers.js
- Mature and widely adopted library with extensive documentation
- Supports multiple Ethereum-compatible networks out of the box
- Large community and ecosystem of tools and extensions
Cons of ethers.js
- Limited to Ethereum and EVM-compatible chains
- May have performance limitations for high-frequency transactions
- Requires additional libraries for advanced cryptographic operations
Code Comparison
ethers.js:
const provider = new ethers.providers.JsonRpcProvider();
const signer = provider.getSigner();
const contract = new ethers.Contract(address, abi, signer);
const tx = await contract.transfer(recipient, amount);
await tx.wait();
fuels-ts:
const wallet = Wallet.fromPrivateKey(privateKey);
const contract = new Contract(address, abi, wallet);
const { transactionId, transactionResponse } = await contract.functions
.transfer(recipient, amount)
.call();
The code snippets demonstrate basic contract interaction. ethers.js uses a provider and signer model, while fuels-ts utilizes a wallet-based approach. fuels-ts offers a more streamlined syntax for function calls and transaction handling, potentially simplifying the development process for Fuel-specific applications.
Collection of comprehensive TypeScript libraries for Interaction with the Ethereum JSON RPC API and utility functions.
Pros of web3.js
- Mature and widely adopted library for Ethereum development
- Extensive documentation and community support
- Supports a broad range of Ethereum-related functionalities
Cons of web3.js
- Larger bundle size, which can impact application performance
- Primarily focused on Ethereum, limiting its use for other blockchain platforms
- Some users report issues with TypeScript support and type definitions
Code Comparison
web3.js:
const Web3 = require('web3');
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR-PROJECT-ID');
const balance = await web3.eth.getBalance('0x1234567890123456789012345678901234567890');
console.log('Balance:', web3.utils.fromWei(balance, 'ether'), 'ETH');
fuels-ts:
import { Wallet } from 'fuels';
const wallet = Wallet.fromPrivateKey('0x...');
const balance = await wallet.getBalance();
console.log('Balance:', balance.format());
The code examples demonstrate basic balance retrieval operations. web3.js requires more setup and uses utility functions for unit conversion, while fuels-ts provides a more streamlined API with built-in formatting.
:warning: The Truffle Suite is being sunset. For information on ongoing support, migration options and FAQs, visit the Consensys blog. Thank you for all the support over the years.
Pros of Truffle
- Mature ecosystem with extensive documentation and community support
- Comprehensive suite of tools for smart contract development, testing, and deployment
- Integrates well with other Ethereum development tools and frameworks
Cons of Truffle
- Primarily focused on Ethereum, limiting its use for other blockchain platforms
- Can be complex for beginners due to its extensive feature set
- Slower to adopt new Ethereum features compared to some newer alternatives
Code Comparison
Truffle (JavaScript):
const MyContract = artifacts.require("MyContract");
module.exports = function(deployer) {
deployer.deploy(MyContract);
};
Fuels-ts (TypeScript):
import { Wallet } from "fuels";
import { MyContract__factory } from "./contracts";
const wallet = Wallet.fromPrivateKey("...");
const contract = MyContract__factory.connect(contractId, wallet);
Key Differences
- Truffle is designed for Ethereum development, while Fuels-ts is specifically for the Fuel blockchain
- Truffle provides a full development environment, whereas Fuels-ts focuses on SDK functionality
- Truffle uses JavaScript, while Fuels-ts is built with TypeScript, offering stronger type safety
Use Cases
- Choose Truffle for comprehensive Ethereum development projects
- Opt for Fuels-ts when building applications on the Fuel blockchain
Both tools serve different purposes and ecosystems, making direct comparison challenging. The choice depends on the target blockchain and specific project requirements.
Hardhat is a development environment to compile, deploy, test, and debug your Ethereum software.
Pros of Hardhat
- Mature ecosystem with extensive documentation and community support
- Robust testing framework with built-in Ethereum network simulation
- Flexible plugin system for extending functionality
Cons of Hardhat
- Primarily focused on Ethereum development, limiting cross-chain compatibility
- Steeper learning curve for beginners compared to Fuels-ts
- Requires more configuration for advanced features
Code Comparison
Hardhat (JavaScript):
const { ethers } = require("hardhat");
async function main() {
const Contract = await ethers.getContractFactory("MyContract");
const contract = await Contract.deploy();
await contract.deployed();
}
Fuels-ts (TypeScript):
import { Wallet } from "fuels";
async function main() {
const wallet = Wallet.generate();
const contract = await Contract.deployContract("MyContract", wallet);
console.log("Contract deployed:", contract.id);
}
Both frameworks provide similar functionality for deploying smart contracts, but Fuels-ts offers a more streamlined approach with built-in wallet integration. Hardhat's example demonstrates its tight integration with the ethers.js library, while Fuels-ts showcases its native TypeScript support and simplified deployment process.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
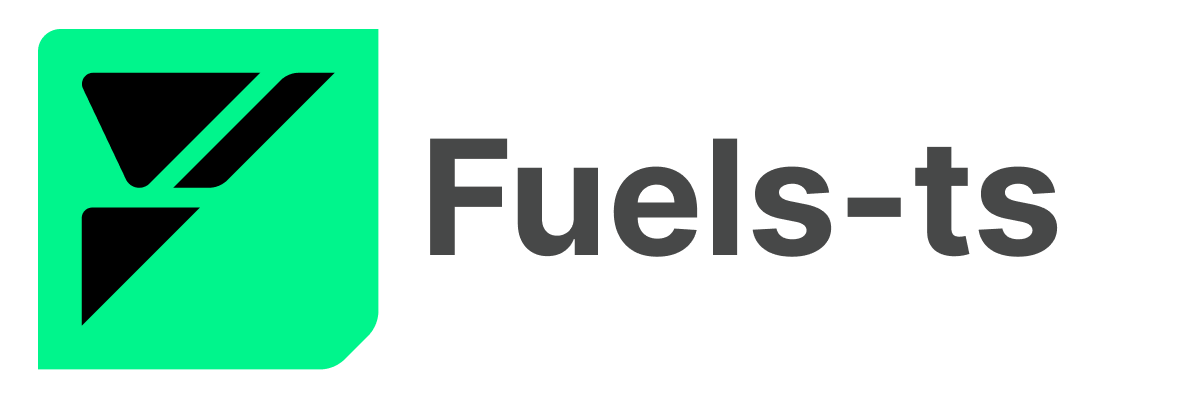
fuels-ts is a library for interacting with Fuel v2.
Resources
The documentation site is your main stop for resources.
Install
npm install fuels --save
If you are a Windows user, you will need to be running Windows Subsystem for Linux (WSL) to install and use the Fuel toolchain, including the TypeScript SDK. We don't support Windows natively at this time.
Import
Simple example usages.
import { Wallet } from "fuels";
// Random Wallet
console.log(Wallet.generate());
// Using privateKey Wallet
console.log(new Wallet("0x0000...0000"));
CLI
Fuels include some utility commands via built-in CLI tool.
Check the docs for more info.
$ npm add fuels
$ npx fuels --help
Usage: fuels [options] [command]
Options:
-D, --debug Enables verbose logging (default: false)
-S, --silent Omit output messages (default: false)
-v, --version Output the version number
-h, --help Display help
Commands:
init [options] Create a sample `fuel.config.ts` file
node [options] Start a Fuel node
dev [options] Start a Fuel node and run build + deploy on every file change
build [options] Build Sway programs and generate Typescript for them
deploy [options] Deploy contracts to the Fuel network
typegen [options] Generate Typescript from Sway ABI JSON files
versions Check for version incompatibilities
help [command] Display help for command
The Fuel Ecosystem
Learn more about the Fuel Ecosystem.
- ð´ Sway â The new language, empowering everyone to build reliable and efficient smart contracts
- ð§° Forc â The Fuel toolbox: Build, deploy and manage your sway projects
- âï¸ Fuel Core â The new FuelVM, a blazingly fast blockchain VM
- ð Fuel Specs â The Fuel protocol specifications
- ð¼ Fuels Wallet â The Official Fuels Wallet
- ð¦ Rust SDK â A robust SDK in rust
- â¡ Fuel Network â The project
- ð The Fuel Forum â Ask questions, get updates, and contribute to a modular future
License
The primary license for this repo is Apache 2.0
, see LICENSE
.
Top Related Projects
Complete Ethereum library and wallet implementation in JavaScript.
Collection of comprehensive TypeScript libraries for Interaction with the Ethereum JSON RPC API and utility functions.
:warning: The Truffle Suite is being sunset. For information on ongoing support, migration options and FAQs, visit the Consensys blog. Thank you for all the support over the years.
Hardhat is a development environment to compile, deploy, test, and debug your Ethereum software.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot