material-react-table
A fully featured Material UI V5 implementation of TanStack React Table V8, written from the ground up in TypeScript
Top Related Projects
🤖 Headless UI for building powerful tables & datagrids for TS/JS - React-Table, Vue-Table, Solid-Table, Svelte-Table
The best JavaScript Data Table for building Enterprise Applications. Supports React / Angular / Vue / Plain JavaScript.
Material UI: Comprehensive React component library that implements Google's Material Design. Free forever.
Next Generation of react-bootstrap-table
Datatables for React using Material-UI
A responsive table library with built-in sorting, pagination, selection, expandable rows, and customizable styling.
Quick Overview
Material React Table is a fully featured data table component for React, built on top of Material-UI (MUI) and TanStack Table v8. It offers a comprehensive set of features for displaying, sorting, filtering, and editing tabular data, with a focus on performance and customization.
Pros
- Extensive feature set including sorting, filtering, pagination, and row selection
- Built on top of popular and well-maintained libraries (Material-UI and TanStack Table)
- Highly customizable with many configuration options
- Excellent TypeScript support
Cons
- Learning curve can be steep due to the large number of features and options
- Large bundle size due to dependencies and feature-rich nature
- May be overkill for simple table needs
- Styling customization can be complex due to Material-UI integration
Code Examples
- Basic table setup:
import MaterialReactTable from 'material-react-table';
const columns = [
{ accessorKey: 'name', header: 'Name' },
{ accessorKey: 'age', header: 'Age' },
];
const data = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 },
];
const MyTable = () => <MaterialReactTable columns={columns} data={data} />;
- Enabling features:
<MaterialReactTable
columns={columns}
data={data}
enableColumnFilters
enablePagination
enableRowSelection
/>
- Custom cell rendering:
const columns = [
{
accessorKey: 'status',
header: 'Status',
Cell: ({ cell }) => (
<Chip
label={cell.getValue()}
color={cell.getValue() === 'Active' ? 'success' : 'error'}
/>
),
},
];
Getting Started
-
Install the package:
npm install material-react-table @mui/material @emotion/react @emotion/styled
-
Import and use in your React component:
import MaterialReactTable from 'material-react-table'; const columns = [ { accessorKey: 'name', header: 'Name' }, { accessorKey: 'age', header: 'Age' }, ]; const data = [ { name: 'John', age: 30 }, { name: 'Jane', age: 25 }, ]; const MyComponent = () => ( <MaterialReactTable columns={columns} data={data} /> );
-
Customize as needed using props and options provided by the library.
Competitor Comparisons
🤖 Headless UI for building powerful tables & datagrids for TS/JS - React-Table, Vue-Table, Solid-Table, Svelte-Table
Pros of TanStack Table
- Framework-agnostic, supporting React, Vue, Solid, and more
- Highly flexible and customizable with a headless approach
- Extensive documentation and community support
Cons of TanStack Table
- Steeper learning curve due to its flexibility
- Requires more setup and configuration for basic use cases
- Less out-of-the-box styling and UI components
Code Comparison
Material React Table:
import MaterialReactTable from 'material-react-table';
const MyTable = () => (
<MaterialReactTable
columns={columns}
data={data}
enableColumnFilters
/>
);
TanStack Table:
import { useReactTable, getCoreRowModel } from '@tanstack/react-table';
const table = useReactTable({
data,
columns,
getCoreRowModel: getCoreRowModel(),
});
Material React Table provides a more opinionated and ready-to-use solution with Material-UI integration, while TanStack Table offers a flexible foundation for building custom table experiences across various frameworks. The choice between them depends on project requirements, desired customization level, and development preferences.
The best JavaScript Data Table for building Enterprise Applications. Supports React / Angular / Vue / Plain JavaScript.
Pros of ag-grid
- More comprehensive feature set, including advanced filtering, sorting, and grouping
- Better performance with large datasets due to virtualization
- Extensive documentation and community support
Cons of ag-grid
- Steeper learning curve due to its complexity
- Requires a commercial license for enterprise features
- Larger bundle size, which may impact initial load times
Code Comparison
material-react-table:
<MaterialReactTable
columns={columns}
data={data}
enableColumnFilters
enableSorting
/>
ag-grid:
<AgGridReact
columnDefs={columnDefs}
rowData={rowData}
defaultColDef={{
filter: true,
sortable: true
}}
/>
Both libraries offer similar basic functionality, but ag-grid provides more granular control over column definitions and grid behavior. material-react-table focuses on simplicity and ease of use, while ag-grid offers more advanced features and customization options.
material-react-table is better suited for projects that require a quick setup and integration with Material-UI, while ag-grid is ideal for complex enterprise applications that need advanced data grid capabilities and can justify the commercial licensing costs.
Material UI: Comprehensive React component library that implements Google's Material Design. Free forever.
Pros of Material-UI
- Comprehensive UI component library with a wide range of pre-built components
- Extensive documentation and community support
- Highly customizable with theming capabilities
Cons of Material-UI
- Steeper learning curve due to its extensive API and features
- Larger bundle size, which may impact initial load times
- More complex setup for specific use cases like data tables
Code Comparison
Material-UI (basic button):
import Button from '@mui/material/Button';
<Button variant="contained" color="primary">
Click me
</Button>
Material React Table (basic table):
import MaterialReactTable from 'material-react-table';
<MaterialReactTable
columns={columns}
data={data}
/>
Summary
Material-UI is a comprehensive UI library offering a wide range of components, while Material React Table focuses specifically on data table functionality. Material-UI provides more flexibility for general UI development, but Material React Table offers a more streamlined solution for complex table requirements. The choice between the two depends on the specific needs of your project, with Material-UI being better suited for full application UI development and Material React Table excelling in data-heavy table implementations.
Next Generation of react-bootstrap-table
Pros of react-bootstrap-table2
- Simpler API and easier to get started for basic table functionality
- Built-in support for Bootstrap styling, making it a good fit for Bootstrap-based projects
- Lightweight and focused specifically on table functionality
Cons of react-bootstrap-table2
- Less feature-rich compared to material-react-table, with fewer advanced features out of the box
- Limited customization options for complex table layouts and interactions
- Less active development and community support
Code Comparison
react-bootstrap-table2:
import BootstrapTable from 'react-bootstrap-table-next';
const columns = [{
dataField: 'id',
text: 'Product ID'
}, {
dataField: 'name',
text: 'Product Name'
}];
<BootstrapTable keyField='id' data={ products } columns={ columns } />
material-react-table:
import { MaterialReactTable } from 'material-react-table';
const columns = [
{ accessorKey: 'id', header: 'Product ID' },
{ accessorKey: 'name', header: 'Product Name' },
];
<MaterialReactTable columns={columns} data={products} />
Both libraries offer a straightforward way to create tables, but material-react-table provides a more modern and feature-rich API with better TypeScript support and integration with Material-UI components.
Datatables for React using Material-UI
Pros of mui-datatables
- More mature and established project with a larger user base
- Offers built-in server-side pagination and filtering
- Provides a simpler API for basic use cases
Cons of mui-datatables
- Less flexible for advanced customization scenarios
- Fewer features for complex data manipulation and editing
- Limited support for nested data structures
Code Comparison
mui-datatables:
import MUIDataTable from "mui-datatables";
const columns = ["Name", "Company", "City", "State"];
const data = [
["Joe James", "Test Corp", "Yonkers", "NY"],
["John Walsh", "Test Corp", "Hartford", "CT"],
];
<MUIDataTable
title={"Employee List"}
data={data}
columns={columns}
options={options}
/>
material-react-table:
import MaterialReactTable from 'material-react-table';
const columns = [
{ accessorKey: 'name', header: 'Name' },
{ accessorKey: 'company', header: 'Company' },
{ accessorKey: 'city', header: 'City' },
{ accessorKey: 'state', header: 'State' },
];
<MaterialReactTable
columns={columns}
data={data}
enableRowSelection
enableColumnFilters
/>
Both libraries offer easy-to-use APIs for creating data tables, but material-react-table provides more granular control over features and styling out of the box.
A responsive table library with built-in sorting, pagination, selection, expandable rows, and customizable styling.
Pros of react-data-table-component
- Lightweight and minimal dependencies, making it easier to integrate into existing projects
- Highly customizable with a wide range of styling options and theming support
- Better performance for large datasets due to its simpler architecture
Cons of react-data-table-component
- Less comprehensive feature set compared to material-react-table
- Limited built-in support for advanced functionalities like row grouping or tree data
- Fewer pre-built components for complex operations like filtering and sorting
Code Comparison
material-react-table:
import { MaterialReactTable } from 'material-react-table';
const MyTable = () => (
<MaterialReactTable
columns={columns}
data={data}
enableColumnFilters
enableGlobalFilter
/>
);
react-data-table-component:
import DataTable from 'react-data-table-component';
const MyTable = () => (
<DataTable
columns={columns}
data={data}
pagination
highlightOnHover
/>
);
Both libraries offer a declarative approach to creating data tables, but material-react-table provides more built-in features out of the box, while react-data-table-component focuses on simplicity and customization. The choice between them depends on the specific requirements of your project and the level of complexity you need in your data tables.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Material React Table V3
View Documentation
About
Quickly Create React Data Tables with Material Design
Built with Material UI V6 and TanStack Table V8
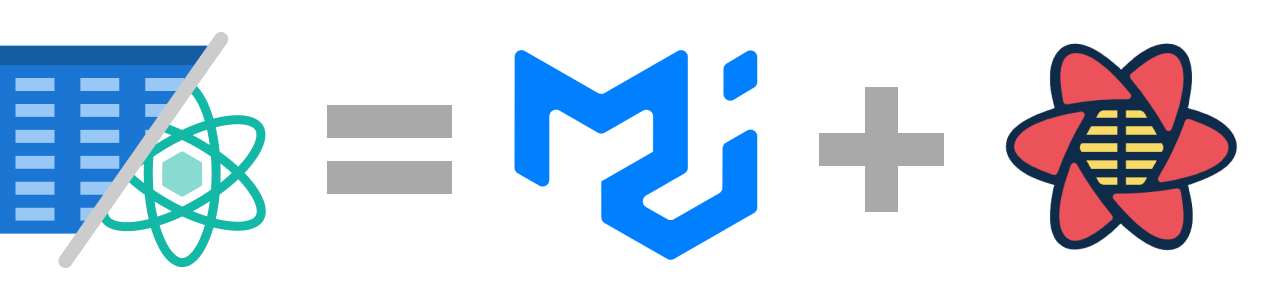
Want to use Mantine instead of Material UI? Check out Mantine React Table
Learn More
- Join the Discord server to join in on the development discussion or ask questions
- View the Docs Website
- See all Props, Options, APIs, Components, and Hooks
Quick Examples
- Basic Table (See Default Features)
- Minimal Table (Turn off Features like Pagination, Sorting, Filtering, and Toolbars)
- Advanced Table (See some of the Advanced Features)
- Custom Headless Table (Build your own table markup)
- Dragging / Ordering Examples (Drag and Drop)
- Editing (CRUD) Examples (Create, Edit, and Delete Rows)
- Expanding / Grouping Examples (Sum, Average, Count, etc.)
- Filtering Examples (Faceted Values, Switching Filters, etc.)
- Sticky Pinning Examples (Sticky Headers, Sticky Columns, Sticky Rows, etc.)
- Remote Data Fetching Examples (Server-side Pagination, Sorting, and Filtering)
- Virtualized Examples (10,000 rows at once!)
- Infinite Scrolling (Fetch data as you scroll)
- Localization (i18n) (Over a dozen languages built-in)
View additional storybook examples
Features
All features can easily be enabled/disabled
Fully Fleshed out Docs are available for all features
- 30-56kb gzipped - Bundlephobia
- Advanced TypeScript Generics Support (TypeScript Optional)
- Aggregation and Grouping (Sum, Average, Count, etc.)
- Cell Actions (Right-click Context Menu)
- Click To Copy Cell Values
- Column Action Dropdown Menu
- Column Hiding
- Column Ordering via Drag'n'Drop
- Column Pinning (Freeze Columns)
- Column Resizing
- Customize Icons
- Customize Styling of internal Mui Components
- Data Editing and Creating (5 different editing modes)
- Density Toggle
- Detail Panels (Expansion)
- Faceted Value Generation for Filter Options
- Filtering (supports client-side and server-side)
- Filter Match Highlighting
- Full Screen Mode
- Global Filtering (Search across all columns, rank by best match)
- Header Groups & Footers
- Localization (i18n) support
- Manage your own state or let the table manage it internally for you
- Pagination (supports client-side and server-side)
- Row Actions (Your Custom Action Buttons)
- Row Numbers
- Row Ordering via Drag'n'Drop
- Row Pinning
- Row Selection (Checkboxes)
- SSR compatible
- Sorting (supports client-side and server-side)
- Theming (Respects your Material UI Theme)
- Toolbars (Add your own action buttons)
- Tree Data / Expanding Sub-rows
- Virtualization (@tanstack/react-virtual)
Getting Started
Installation
View the full Installation Docs
-
Ensure that you have React 18 or later installed
-
Install Peer Dependencies (Material UI V6)
npm install @mui/material @mui/x-date-pickers @mui/icons-material @emotion/react @emotion/styled
- Install material-react-table
npm install material-react-table
@tanstack/react-table
,@tanstack/react-virtual
, and@tanstack/match-sorter-utils
are internal dependencies, so you do NOT need to install them yourself.
Usage
Read the full usage docs here
import { useMemo, useState, useEffect } from 'react';
import {
MaterialReactTable,
useMaterialReactTable,
} from 'material-react-table';
//data must be stable reference (useState, useMemo, useQuery, defined outside of component, etc.)
const data = [
{
name: 'John',
age: 30,
},
{
name: 'Sara',
age: 25,
},
];
export default function App() {
const columns = useMemo(
() => [
{
accessorKey: 'name', //simple recommended way to define a column
header: 'Name',
muiTableHeadCellProps: { sx: { color: 'green' } }, //optional custom props
Cell: ({ cell }) => <span>{cell.getValue()}</span>, //optional custom cell render
},
{
accessorFn: (row) => row.age, //alternate way
id: 'age', //id required if you use accessorFn instead of accessorKey
header: 'Age',
Header: () => <i>Age</i>, //optional custom header render
},
],
[],
);
//optionally, you can manage any/all of the table state yourself
const [rowSelection, setRowSelection] = useState({});
useEffect(() => {
//do something when the row selection changes
}, [rowSelection]);
const table = useMaterialReactTable({
columns,
data,
enableColumnOrdering: true, //enable some features
enableRowSelection: true,
enablePagination: false, //disable a default feature
onRowSelectionChange: setRowSelection, //hoist internal state to your own state (optional)
state: { rowSelection }, //manage your own state, pass it back to the table (optional)
});
const someEventHandler = () => {
//read the table state during an event from the table instance
console.log(table.getState().sorting);
};
return (
<MaterialReactTable table={table} /> //other more lightweight MRT sub components also available
);
}
Open in Code Sandbox
Contributors
PRs are Welcome, but please discuss in GitHub Discussions or the Discord Server first if it is a large change!
Read the Contributing Guide to learn how to run this project locally.
Top Related Projects
🤖 Headless UI for building powerful tables & datagrids for TS/JS - React-Table, Vue-Table, Solid-Table, Svelte-Table
The best JavaScript Data Table for building Enterprise Applications. Supports React / Angular / Vue / Plain JavaScript.
Material UI: Comprehensive React component library that implements Google's Material Design. Free forever.
Next Generation of react-bootstrap-table
Datatables for React using Material-UI
A responsive table library with built-in sorting, pagination, selection, expandable rows, and customizable styling.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot