react-modern-calendar-datepicker
A modern, beautiful, customizable date picker for React
Top Related Projects
A simple and reusable datepicker component for React
An easily internationalizable, mobile-friendly datepicker library for the web
Date & Time pickers for Material UI (support from v1 to v4)
DayPicker is a customizable date picker component for React. Add date pickers, calendars, and date inputs to your web applications.
React Calendar
Quick Overview
React Modern Calendar Datepicker is a lightweight, customizable date picker component for React applications. It provides a modern and responsive interface for selecting dates, with support for both single date and date range selection.
Pros
- Highly customizable with various theming options
- Supports both single date and date range selection
- Lightweight and performant
- Responsive design that works well on mobile devices
Cons
- Limited documentation for advanced use cases
- Lacks some advanced features like time selection
- Not as widely adopted as some other date picker libraries
- May require additional styling for seamless integration with some design systems
Code Examples
- Basic usage for single date selection:
import React, { useState } from 'react';
import DatePicker from 'react-modern-calendar-datepicker';
const App = () => {
const [selectedDay, setSelectedDay] = useState(null);
return (
<DatePicker
value={selectedDay}
onChange={setSelectedDay}
/>
);
};
- Date range selection:
import React, { useState } from 'react';
import DatePicker from 'react-modern-calendar-datepicker';
const App = () => {
const [selectedDayRange, setSelectedDayRange] = useState({
from: null,
to: null
});
return (
<DatePicker
value={selectedDayRange}
onChange={setSelectedDayRange}
/>
);
};
- Customizing the appearance:
import React, { useState } from 'react';
import DatePicker from 'react-modern-calendar-datepicker';
const App = () => {
const [selectedDay, setSelectedDay] = useState(null);
return (
<DatePicker
value={selectedDay}
onChange={setSelectedDay}
colorPrimary="#0fbcf9"
colorPrimaryLight="rgba(15, 188, 249, 0.2)"
calendarClassName="custom-calendar"
calendarTodayClassName="custom-today-day"
/>
);
};
Getting Started
-
Install the package:
npm install react-modern-calendar-datepicker
-
Import the component and styles:
import DatePicker from 'react-modern-calendar-datepicker'; import 'react-modern-calendar-datepicker/lib/DatePicker.css';
-
Use the component in your React application:
const [selectedDay, setSelectedDay] = useState(null); return ( <DatePicker value={selectedDay} onChange={setSelectedDay} /> );
Competitor Comparisons
A simple and reusable datepicker component for React
Pros of react-datepicker
- More comprehensive feature set, including time selection and custom input components
- Larger community and more frequent updates (20k+ stars, regular releases)
- Extensive documentation and examples
Cons of react-datepicker
- Larger bundle size due to more features
- Steeper learning curve for basic implementations
- Less modern default styling compared to react-modern-calendar-datepicker
Code Comparison
react-datepicker:
import DatePicker from "react-datepicker";
<DatePicker
selected={startDate}
onChange={(date) => setStartDate(date)}
showTimeSelect
dateFormat="Pp"
/>
react-modern-calendar-datepicker:
import DatePicker from "react-modern-calendar-datepicker";
<DatePicker
value={selectedDay}
onChange={setSelectedDay}
inputPlaceholder="Select a day"
/>
The code comparison shows that react-datepicker offers more built-in options like time selection, while react-modern-calendar-datepicker has a simpler API for basic date picking. react-datepicker uses a Date object for its value, whereas react-modern-calendar-datepicker uses a custom object structure.
An easily internationalizable, mobile-friendly datepicker library for the web
Pros of react-dates
- More comprehensive and feature-rich, offering a wide range of customization options
- Better documentation and extensive examples for various use cases
- Larger community support and more frequent updates
Cons of react-dates
- Larger bundle size due to its extensive feature set
- Steeper learning curve for beginners due to its complexity
- Requires additional styling and configuration for a modern look
Code Comparison
react-dates:
import { DateRangePicker } from 'react-dates';
<DateRangePicker
startDate={this.state.startDate}
startDateId="start_date_id"
endDate={this.state.endDate}
endDateId="end_date_id"
onDatesChange={({ startDate, endDate }) => this.setState({ startDate, endDate })}
focusedInput={this.state.focusedInput}
onFocusChange={focusedInput => this.setState({ focusedInput })}
/>
react-modern-calendar-datepicker:
import DatePicker from 'react-modern-calendar-datepicker';
<DatePicker
value={selectedDay}
onChange={setSelectedDay}
inputPlaceholder="Select a day"
shouldHighlightWeekends
/>
The react-dates example shows more complex configuration for a date range picker, while react-modern-calendar-datepicker offers a simpler API for a single date picker. react-dates requires more setup but provides greater flexibility, whereas react-modern-calendar-datepicker is more straightforward to implement but may have fewer customization options.
Date & Time pickers for Material UI (support from v1 to v4)
Pros of material-ui-pickers
- Integrated with Material-UI design system, providing consistent styling
- Offers a wider range of date/time picker components (e.g., time, date-time, month)
- Better accessibility support and keyboard navigation
Cons of material-ui-pickers
- Larger bundle size due to Material-UI dependencies
- Less customizable in terms of styling and layout
- Steeper learning curve for developers not familiar with Material-UI
Code Comparison
react-modern-calendar-datepicker:
import DatePicker from 'react-modern-calendar-datepicker';
<DatePicker
value={selectedDay}
onChange={setSelectedDay}
inputPlaceholder="Select a day"
/>
material-ui-pickers:
import { DatePicker } from '@material-ui/pickers';
<DatePicker
value={selectedDate}
onChange={handleDateChange}
label="Select a date"
/>
Both libraries offer simple APIs for basic date picking functionality. However, material-ui-pickers provides more options for customization and integration with Material-UI components, while react-modern-calendar-datepicker focuses on a lightweight and standalone solution.
DayPicker is a customizable date picker component for React. Add date pickers, calendars, and date inputs to your web applications.
Pros of react-day-picker
- More customizable and flexible, allowing for advanced use cases
- Extensive documentation and examples
- Larger community and more frequent updates
Cons of react-day-picker
- Steeper learning curve due to its flexibility
- Requires more setup for basic use cases
Code Comparison
react-day-picker:
import { DayPicker } from 'react-day-picker';
function MyDatePicker() {
return <DayPicker mode="single" />;
}
react-modern-calendar-datepicker:
import DatePicker from 'react-modern-calendar-datepicker';
function MyDatePicker() {
return <DatePicker />;
}
Summary
react-day-picker offers more flexibility and customization options, making it suitable for complex date-picking scenarios. It has a larger community and more frequent updates. However, it may require more setup for basic use cases and has a steeper learning curve.
react-modern-calendar-datepicker provides a simpler API and is easier to set up for basic date-picking needs. It may be more suitable for projects that don't require extensive customization.
The code comparison shows that both libraries offer a straightforward implementation for basic date picking, with react-modern-calendar-datepicker requiring slightly less code for a default setup.
React Calendar
Pros of calendar
- More comprehensive and feature-rich, offering a wider range of calendar-related functionalities
- Better documentation and examples, making it easier for developers to implement and customize
- Larger community and more frequent updates, ensuring better long-term support and maintenance
Cons of calendar
- Steeper learning curve due to its extensive feature set and configuration options
- Larger bundle size, which may impact performance for simpler use cases
- Less focused on modern design aesthetics compared to react-modern-calendar-datepicker
Code Comparison
react-modern-calendar-datepicker:
<Calendar
value={selectedDay}
onChange={setSelectedDay}
shouldHighlightWeekends
/>
calendar:
<Calendar
value={value}
onSelect={onSelect}
onPanelChange={onPanelChange}
fullscreen={false}
headerRender={({ value, type, onChange, onTypeChange }) => {
// Custom header rendering
}}
/>
The code comparison shows that calendar offers more customization options and flexibility in its implementation, while react-modern-calendar-datepicker provides a simpler and more straightforward approach for basic date picking functionality.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
react-modern-calendar-datepicker
A modern, beautiful, customizable date picker for React.
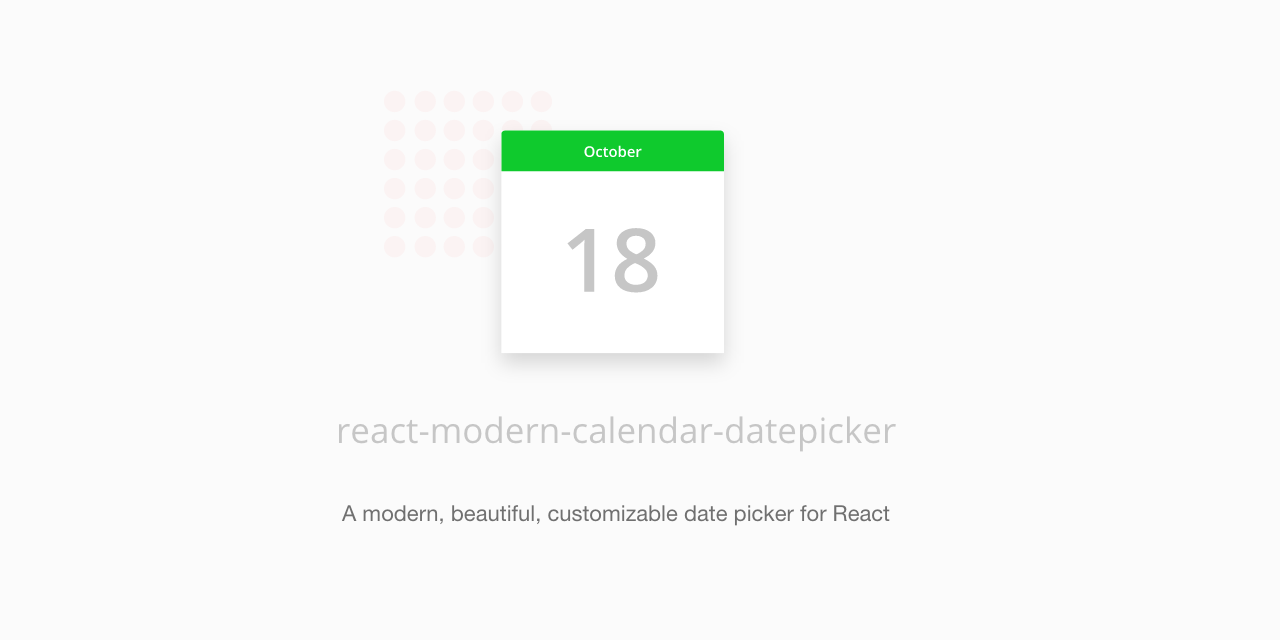
Installation ð
npm i react-modern-calendar-datepicker
# or if you prefer Yarn:
yarn add react-modern-calendar-datepicker
Documentation ð
You can find documentation on the website.
The documentation is divided into several sections:
- Getting Started
- Core Concepts
- Default Values
- Minimum & Maximum Date
- Disabled Days
- Customization
- Responsive Guide
- Utilities
- Different Locales
- TypeScript
Contributors â¨
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!
LICENSE
Top Related Projects
A simple and reusable datepicker component for React
An easily internationalizable, mobile-friendly datepicker library for the web
Date & Time pickers for Material UI (support from v1 to v4)
DayPicker is a customizable date picker component for React. Add date pickers, calendars, and date inputs to your web applications.
React Calendar
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot