paper-onboarding-android
:octocat: PaperOnboarding is a material design slider made by @Ramotion
Top Related Projects
Inspired by Heinrich Reimer Material Intro and developed with love from scratch
Make a cool intro for your Android app.
A simple material design app intro with cool animations and a fluent API.
A collaborative list of awesome Swift libraries and resources. Feel free to contribute!
Quick Overview
Paper Onboarding for Android is a material design UI library created by Ramotion. It provides a sleek and interactive way to introduce users to an app's features through a series of animated screens. The library offers customizable onboarding elements with smooth transitions and engaging visuals.
Pros
- Easy integration with existing Android projects
- Highly customizable UI elements and animations
- Smooth and visually appealing transitions between onboarding screens
- Follows Material Design guidelines for a modern look and feel
Cons
- Limited to onboarding functionality, not a comprehensive UI toolkit
- May require additional customization for complex onboarding flows
- Documentation could be more extensive for advanced use cases
- Dependency on other libraries may increase app size
Code Examples
- Adding the Paper Onboarding view to your layout:
<com.ramotion.paperonboarding.PaperOnboardingView
android:id="@+id/onboardingView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
- Creating onboarding items programmatically:
val onBoardingPage1 = PaperOnboardingPage(
"Hotels",
"All hotels and hostels are sorted by hospitality rating",
Color.parseColor("#678FB4"),
R.drawable.hotels,
R.drawable.key
)
val onBoardingPage2 = PaperOnboardingPage(
"Banks",
"We carefully verify all banks before add them into the app",
Color.parseColor("#65B0B4"),
R.drawable.banks,
R.drawable.wallet
)
val elements = listOf(onBoardingPage1, onBoardingPage2)
- Setting up the onboarding view:
val onboardingView = findViewById<PaperOnboardingView>(R.id.onboardingView)
onboardingView.setOnRightOutListener { /* Handle completion */ }
onboardingView.setElements(elements)
Getting Started
- Add the dependency to your
build.gradle
file:
dependencies {
implementation 'com.ramotion.paperonboarding:paper-onboarding:1.1.3'
}
- Add the
PaperOnboardingView
to your layout XML:
<com.ramotion.paperonboarding.PaperOnboardingView
android:id="@+id/onboardingView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
- Create onboarding pages and set them to the view in your Activity or Fragment:
val pages = listOf(
PaperOnboardingPage("Title 1", "Description 1", Color.parseColor("#678FB4"), R.drawable.icon1, R.drawable.icon1_bg),
PaperOnboardingPage("Title 2", "Description 2", Color.parseColor("#65B0B4"), R.drawable.icon2, R.drawable.icon2_bg)
)
findViewById<PaperOnboardingView>(R.id.onboardingView).setElements(pages)
Competitor Comparisons
Inspired by Heinrich Reimer Material Intro and developed with love from scratch
Pros of material-intro-screen
- More customizable with a wider range of options for slide content and behavior
- Supports parallax effect for a more dynamic and engaging user experience
- Includes built-in permission handling for Android 6.0+
Cons of material-intro-screen
- Less visually polished out-of-the-box compared to paper-onboarding-android
- May require more setup and configuration to achieve desired results
- Last updated in 2017, potentially outdated for newer Android versions
Code Comparison
material-intro-screen:
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
introScreen(this) {
page(R.layout.intro_layout) {
title = "Welcome"
description = "This is a sample intro screen"
}
}
}
paper-onboarding-android:
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.onboarding_main)
val onBoardingView = findViewById<PaperOnboardingView>(R.id.onboardingView)
onBoardingView.setOnRightOutListener { /* Handle completion */ }
}
Both libraries offer simple implementation, but material-intro-screen provides more granular control over individual pages, while paper-onboarding-android focuses on a streamlined, visually appealing experience with less customization required.
Make a cool intro for your Android app.
Pros of AppIntro
- More comprehensive feature set, including customizable layouts and animations
- Larger community and more frequent updates
- Better documentation and examples
Cons of AppIntro
- Steeper learning curve due to more complex API
- Larger library size, which may impact app size
Code Comparison
AppIntro:
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setImmersiveMode()
addSlide(AppIntroFragment.newInstance(
title = "Welcome",
description = "This is a sample slide"
))
}
paper-onboarding-android:
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.onboarding_main)
val onBoardingView = findViewById<PaperOnboardingView>(R.id.onboardingView)
onBoardingView.setOnChangeListener(this)
}
AppIntro offers a more declarative approach to creating onboarding screens, while paper-onboarding-android requires more manual setup. AppIntro's API provides more built-in options for customization, but paper-onboarding-android's simpler structure may be easier for beginners to understand and implement quickly.
A simple material design app intro with cool animations and a fluent API.
Pros of material-intro
- More customizable with additional features like permissions handling and custom fragments
- Supports both ViewPager and ViewPager2
- Actively maintained with recent updates
Cons of material-intro
- Less visually appealing animations compared to paper-onboarding-android
- Steeper learning curve due to more complex API
- Larger library size due to additional features
Code Comparison
material-intro:
MaterialIntroActivity.Builder(this)
.addSlide(SimpleSlide.Builder()
.title("Title")
.description("Description")
.image(R.drawable.image)
.background(R.color.background)
.build())
.build()
paper-onboarding-android:
val onBoardingItems = listOf(
PaperOnboardingPage("Title", "Description", Color.parseColor("#color"), R.drawable.image, R.drawable.icon),
// Add more pages...
)
PaperOnboardingFragment.newInstance(onBoardingItems)
material-intro offers more flexibility in customization and additional features, making it suitable for complex onboarding flows. However, paper-onboarding-android provides a simpler API and more visually appealing animations out of the box. The choice between the two depends on the specific requirements of the project, with material-intro being better for feature-rich onboarding experiences and paper-onboarding-android for quick, visually appealing implementations.
A collaborative list of awesome Swift libraries and resources. Feel free to contribute!
Pros of awesome-swift
- Comprehensive resource for Swift developers, covering a wide range of topics and libraries
- Regularly updated with community contributions, ensuring up-to-date information
- Provides a curated list of high-quality Swift resources, saving developers time in research
Cons of awesome-swift
- Not a specific UI component or library, unlike paper-onboarding-android
- Requires more effort to implement specific features, as it's a collection of resources rather than a ready-to-use component
- May overwhelm beginners with the sheer amount of information provided
Code comparison
As awesome-swift is a curated list of resources and not a specific library, a direct code comparison is not applicable. However, here's an example of how you might use a library listed in awesome-swift for onboarding, compared to paper-onboarding-android:
awesome-swift (using SwiftyOnboard):
let swiftyOnboard = SwiftyOnboard(frame: view.frame)
view.addSubview(swiftyOnboard)
swiftyOnboard.style = .light
swiftyOnboard.delegate = self
paper-onboarding-android:
val onBoardingView = PaperOnboardingView(this)
setContentView(onBoardingView)
onBoardingView.setOnRightOutListener { /* Handle right out */ }
onBoardingView.setOnLeftOutListener { /* Handle left out */ }
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME

PAPER ONBOARDING
Android library Paper Onboarding is a material design UI slider written on Java
We specialize in the designing and coding of custom UI for Mobile Apps and Websites.

Stay tuned for the latest updates:

Requirements
â
- Android 4.0.3 IceCreamSandwich (API lvl 15) or greater
- Your favorite IDE
Installation
â Just download the package from here and add it to your project classpath, or just use the maven repo: â Gradle:
'com.ramotion.paperonboarding:paper-onboarding:1.1.3'
SBT:
libraryDependencies += "com.ramotion.paperonboarding" % "paper-onboarding" % "1.1.3"
Maven:
<dependency>
<groupId>com.ramotion.paperonboarding</groupId>
<artifactId>paper-onboarding</artifactId>
<version>1.1.3</version>
<type>aar</type>
</dependency>
Basic usage
Paper Onboarding is a simple and easy to use onboarding slider for your app. You just need to provide content for each slider page - a main icon, text, and small round icon for the bottom.
1 Use PaperOnboardingPage
to prepare your data for slider:
PaperOnboardingPage scr1 = new PaperOnboardingPage("Hotels",
"All hotels and hostels are sorted by hospitality rating",
Color.parseColor("#678FB4"), R.drawable.hotels, R.drawable.key);
PaperOnboardingPage scr2 = new PaperOnboardingPage("Banks",
"We carefully verify all banks before add them into the app",
Color.parseColor("#65B0B4"), R.drawable.banks, R.drawable.wallet);
PaperOnboardingPage scr3 = new PaperOnboardingPage("Stores",
"All local stores are categorized for your convenience",
Color.parseColor("#9B90BC"), R.drawable.stores, R.drawable.shopping_cart);
ArrayList<PaperOnboardingPage> elements = new ArrayList<>();
elements.add(scr1);
elements.add(scr2);
elements.add(scr3);
2 Create a fragment from PaperOnboardingFragment
and provide your data.
PaperOnboardingFragment onBoardingFragment = PaperOnboardingFragment.newInstance(elements);
3 Done! Now you can use this fragment as you want in your activity, for example :
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.add(R.id.fragment_container, onBoardingFragment);
fragmentTransaction.commit();
4 Extra step : You can add event listeners to fragments with your logic, like replacing this fragment to another when the user swipes next from the last screen:
onBoardingFragment.setOnRightOutListener(new PaperOnboardingOnRightOutListener() {
@Override
public void onRightOut() {
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
Fragment bf = new BlankFragment();
fragmentTransaction.replace(R.id.fragment_container, bf);
fragmentTransaction.commit();
}
});
Currently, there are three listeners that cover all events - onRightOut, onLeftOut and onChange; see code examples and usage in the repo.
ð Check this library on other language:

ð License
Paper Onboarding Android is released under the MIT license. See LICENSE for details.
This library is a part of a selection of our best UI open-source projects
If you use the open-source library in your project, please make sure to credit and backlink to www.ramotion.com
ð± Get the Showroom App for Android to give it a try
Try this UI component and more like this in our Android app. Contact us if interested.
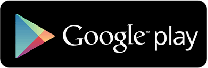

Top Related Projects
Inspired by Heinrich Reimer Material Intro and developed with love from scratch
Make a cool intro for your Android app.
A simple material design app intro with cool animations and a fluent API.
A collaborative list of awesome Swift libraries and resources. Feel free to contribute!
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot