Top Related Projects
Node graph editor framework focused on data processing using Unity UIElements and C# 4.6
A flexible and modular Node Editor Framework for creating node based displays and editors in Unity
Additional tools for Visual Effect Artists
Quick Overview
xNode is a node editor framework for Unity, allowing developers to create node-based visual scripting systems. It provides a flexible and customizable foundation for building node graphs within the Unity engine, making it easier to implement complex logic and workflows visually.
Pros
- Highly customizable and extensible node system
- Intuitive visual programming interface within Unity
- Supports both runtime and editor-time functionality
- Active community and ongoing development
Cons
- Learning curve for initial setup and custom node creation
- Limited built-in node types, requiring more custom development
- Performance may be impacted for very large node graphs
- Documentation could be more comprehensive
Code Examples
Creating a custom node:
[CreateNodeMenu("Custom/MyNode")]
public class MyNode : Node {
[Input] public float input;
[Output] public float output;
public override object GetValue(NodePort port) {
return input * 2; // Double the input value
}
}
Connecting nodes programmatically:
NodeGraph graph = ScriptableObject.CreateInstance<NodeGraph>();
MyNode node1 = graph.AddNode<MyNode>();
MyNode node2 = graph.AddNode<MyNode>();
node1.GetOutputPort("output").Connect(node2.GetInputPort("input"));
Accessing node values:
float result = (float)node2.GetInputPort("input").GetInputValue();
Debug.Log("Node input value: " + result);
Getting Started
- Install xNode in your Unity project (via Package Manager or manual import).
- Create a new script inheriting from
NodeGraph
:
using XNode;
[CreateAssetMenu(fileName = "MyNodeGraph", menuName = "MyNodeGraph")]
public class MyNodeGraph : NodeGraph { }
- Create custom node scripts inheriting from
Node
. - Add nodes to your graph in the Unity editor or programmatically.
- Connect nodes and access their values as needed in your game logic.
For more detailed instructions, refer to the xNode documentation and examples in the GitHub repository.
Competitor Comparisons
Node graph editor framework focused on data processing using Unity UIElements and C# 4.6
Pros of NodeGraphProcessor
- More advanced and feature-rich, with support for multi-threading and compute shaders
- Better performance for large-scale node graphs
- Includes a built-in node library and custom node creation tools
Cons of NodeGraphProcessor
- Steeper learning curve due to increased complexity
- Less lightweight and may be overkill for simpler projects
- Requires Unity 2019.3 or later, limiting compatibility with older projects
Code Comparison
NodeGraphProcessor:
[System.Serializable, NodeMenuItem("Custom/Add")]
public class AddNode : BaseNode
{
[Input(name = "A")] public float inputA;
[Input(name = "B")] public float inputB;
[Output(name = "Out")] public float output;
public override string name => "Add";
}
xNode:
[CreateNodeMenu("Math/Add")]
public class AddNode : Node
{
[Input] public float a;
[Input] public float b;
[Output] public float result;
public override object GetValue(NodePort port) {
return a + b;
}
}
Both repositories provide node-based graph systems for Unity, but NodeGraphProcessor offers more advanced features at the cost of increased complexity, while xNode is simpler and more lightweight. The code comparison shows similar node definitions, with NodeGraphProcessor using attributes for input/output declarations and xNode using a custom GetValue method.
A flexible and modular Node Editor Framework for creating node based displays and editors in Unity
Pros of Node_Editor_Framework
- More flexible and customizable node system
- Better support for complex graph structures
- Includes a runtime evaluation system
Cons of Node_Editor_Framework
- Less actively maintained (last update in 2019)
- Steeper learning curve due to increased complexity
- Limited documentation compared to xNode
Code Comparison
Node_Editor_Framework:
[Node(false, "Example Node")]
public class ExampleNode : Node
{
[ValueConnectionKnob("Input", Direction.In, "Float")]
public ValueConnectionKnob inputKnob;
[ValueConnectionKnob("Output", Direction.Out, "Float")]
public ValueConnectionKnob outputKnob;
}
xNode:
[CreateNodeMenu("Example Node")]
public class ExampleNode : Node
{
[Input] public float input;
[Output] public float output;
}
Both frameworks offer node-based editing capabilities for Unity, but they differ in their approach and features. Node_Editor_Framework provides more flexibility and support for complex graph structures, while xNode offers a simpler, more straightforward implementation with better documentation and active maintenance.
Additional tools for Visual Effect Artists
Pros of VFXToolbox
- Official Unity tool, ensuring compatibility and long-term support
- Focused on visual effects creation, offering specialized features for VFX artists
- Integrates seamlessly with Unity's Visual Effect Graph system
Cons of VFXToolbox
- Limited to visual effects workflows, less versatile for general node-based systems
- Steeper learning curve for users not familiar with Unity's VFX ecosystem
- Less community-driven development compared to xNode
Code Comparison
VFXToolbox (C#):
public class VFXOperator : VFXSlotContainerModel<VFXModel, VFXModel>
{
protected override void OnInvalidate(VFXModel model, InvalidationCause cause)
{
base.OnInvalidate(model, cause);
}
}
xNode (C#):
public abstract class Node : ScriptableObject
{
[SerializeField] private NodePort[] ports = new NodePort[0];
[NonSerialized] private NodeGraph graph;
public NodeGraph Graph { get { return graph; } set { graph = value; } }
}
Both repositories provide node-based systems, but VFXToolbox is tailored for Unity's visual effects workflow, while xNode offers a more general-purpose node graph solution. VFXToolbox benefits from official Unity support and integration, whereas xNode provides greater flexibility for various node-based applications beyond visual effects.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
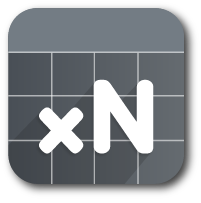
Downloads / Asset Store / Documentation
Support xNode on Ko-fi or Patreon
For full Odin support, consider using KAJed82's fork
xNode
Thinking of developing a node-based plugin? Then this is for you. You can download it as an archive and unpack to a new unity project, or connect it as git submodule.
xNode is super userfriendly, intuitive and will help you reap the benefits of node graphs in no time. With a minimal footprint, it is ideal as a base for custom state machines, dialogue systems, decision makers etc.
Key features
- Lightweight in runtime
- Very little boilerplate code
- Strong separation of editor and runtime code
- No runtime reflection (unless you need to edit/build node graphs at runtime. In this case, all reflection is cached.)
- Does not rely on any 3rd party plugins
- Custom node inspector code is very similar to regular custom inspector code
- Supported from Unity 5.3 and up
Wiki
- Getting started - create your very first node node and graph
- Examples branch - look at other small projects
Installation
Instructions
Installing with Unity Package Manager
Via Git URL (Requires Unity version 2018.3.0b7 or above)
To install this project as a Git dependency using the Unity Package Manager,
add the following line to your project's manifest.json
:
"com.github.siccity.xnode": "https://github.com/siccity/xNode.git"
You will need to have Git installed and available in your system's PATH.
If you are using Assembly Definitions in your project, you will need to add XNode
and/or XNodeEditor
as Assembly Definition References.
Via OpenUPM
The package is available on the openupm registry. It's recommended to install it via openupm-cli.
openupm add com.github.siccity.xnode
Installing with git
Via Git Submodule
To add xNode as a submodule in your existing git project, run the following git command from your project root:
git submodule add git@github.com:Siccity/xNode.git Assets/Submodules/xNode
Installing 'the old way'
If no source control or package manager is available to you, you can simply copy/paste the source files into your assets folder.
Node example:
// public classes deriving from Node are registered as nodes for use within a graph
public class MathNode : Node {
// Adding [Input] or [Output] is all you need to do to register a field as a valid port on your node
[Input] public float a;
[Input] public float b;
// The value of an output node field is not used for anything, but could be used for caching output results
[Output] public float result;
[Output] public float sum;
// The value of 'mathType' will be displayed on the node in an editable format, similar to the inspector
public MathType mathType = MathType.Add;
public enum MathType { Add, Subtract, Multiply, Divide}
// GetValue should be overridden to return a value for any specified output port
public override object GetValue(NodePort port) {
// Get new a and b values from input connections. Fallback to field values if input is not connected
float a = GetInputValue<float>("a", this.a);
float b = GetInputValue<float>("b", this.b);
// After you've gotten your input values, you can perform your calculations and return a value
if (port.fieldName == "result")
switch(mathType) {
case MathType.Add: default: return a + b;
case MathType.Subtract: return a - b;
case MathType.Multiply: return a * b;
case MathType.Divide: return a / b;
}
else if (port.fieldName == "sum") return a + b;
else return 0f;
}
}
Plugins
Plugins are repositories that add functionality to xNode
- xNodeGroups: adds resizable groups
Community
Join the Discord server to leave feedback or get support. Feel free to also leave suggestions/requests in the issues page.
Top Related Projects
Node graph editor framework focused on data processing using Unity UIElements and C# 4.6
A flexible and modular Node Editor Framework for creating node based displays and editors in Unity
Additional tools for Visual Effect Artists
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot