Top Related Projects
๐ฑ๐ ๐งฉ Cross Device & High Performance Normal Form/Dynamic(JSON Schema) Form/Form Builder -- Support React/React Native/Vue 2/Vue 3
๐ React Hooks for form state management and validation (Web + React Native)
Build forms in React, without the tears ๐ญ
๐ High performance subscription-based form state management for React
Performance-focused API for React forms ๐
Quick Overview
Designable is an open-source low-code development platform created by Alibaba. It provides a drag-and-drop interface for building web applications, allowing developers and non-developers to create complex UIs without extensive coding knowledge. The platform is highly extensible and customizable, making it suitable for a wide range of projects.
Pros
- User-friendly drag-and-drop interface for rapid UI development
- Highly extensible and customizable, allowing for the creation of custom components
- Supports multiple frameworks, including React and Vue
- Generates clean, readable code that can be easily maintained
Cons
- Learning curve for advanced customization and component creation
- Documentation is primarily in Chinese, which may be challenging for non-Chinese speakers
- Limited community support compared to more established low-code platforms
- May not be suitable for highly complex or specialized applications
Code Examples
- Creating a simple form using Designable:
import React from 'react'
import { Designer, DesignerToolsWidget, ViewToolsWidget, Workspace } from '@designable/react'
import { createDesigner, GlobalRegistry } from '@designable/core'
const App = () => {
const engine = createDesigner()
return (
<Designer engine={engine}>
<DesignerToolsWidget />
<ViewToolsWidget />
<Workspace />
</Designer>
)
}
export default App
- Registering a custom component:
import { GlobalRegistry } from '@designable/core'
GlobalRegistry.registerDesignerLocales({
'zh-CN': {
sources: {
CustomComponent: '่ชๅฎไน็ปไปถ',
},
},
'en-US': {
sources: {
CustomComponent: 'Custom Component',
},
},
})
GlobalRegistry.registerDesignerProps({
CustomComponent: {
// Component properties
},
})
- Using the generated schema:
import React from 'react'
import { createForm } from '@formily/core'
import { createSchemaField } from '@formily/react'
import { Form } from '@formily/antd'
const SchemaField = createSchemaField({
components: {
// Your components
},
})
const App = () => {
const form = createForm()
return (
<Form form={form}>
<SchemaField schema={yourGeneratedSchema} />
</Form>
)
}
export default App
Getting Started
- Install Designable:
npm install @designable/react @designable/core @formily/react @formily/antd
- Create a new React component:
import React from 'react'
import { Designer, DesignerToolsWidget, ViewToolsWidget, Workspace } from '@designable/react'
import { createDesigner } from '@designable/core'
const DesignableApp = () => {
const engine = createDesigner()
return (
<Designer engine={engine}>
<DesignerToolsWidget />
<ViewToolsWidget />
<Workspace />
</Designer>
)
}
export default DesignableApp
- Use the component in your application:
import React from 'react'
import DesignableApp from './DesignableApp'
const App = () => {
return (
<div>
<h1>My Designable App</h1>
<DesignableApp />
</div>
)
}
export default App
Competitor Comparisons
๐ฑ๐ ๐งฉ Cross Device & High Performance Normal Form/Dynamic(JSON Schema) Form/Form Builder -- Support React/React Native/Vue 2/Vue 3
Pros of Formily
- More comprehensive form solution with advanced features like schema-driven forms and complex validation
- Better documentation and wider community adoption
- Supports multiple UI frameworks including Ant Design, Fusion Design, and Element
Cons of Formily
- Steeper learning curve due to its complexity and extensive API
- May be overkill for simple form scenarios
- Less focus on visual form design compared to Designable
Code Comparison
Formily:
import { createForm } from '@formily/core'
import { Field } from '@formily/react'
const form = createForm()
<Field name="username" component="Input" />
Designable:
import { Designer } from 'designable-core'
import { DesignerEngine } from 'designable-react'
const engine = new Designer()
<DesignerEngine engine={engine}>
{/* Form components */}
</DesignerEngine>
Key Differences
- Formily focuses on form management and validation, while Designable emphasizes visual form design
- Formily has a more extensive ecosystem and integrations
- Designable offers a drag-and-drop interface for form creation, making it more user-friendly for non-developers
Use Cases
- Choose Formily for complex form scenarios with advanced validation and schema requirements
- Opt for Designable when visual form design and rapid prototyping are priorities
๐ React Hooks for form state management and validation (Web + React Native)
Pros of react-hook-form
- Lightweight and performant, with minimal re-renders
- Easy integration with existing React projects
- Extensive documentation and community support
Cons of react-hook-form
- Limited to form handling, not a complete UI builder
- Requires more manual setup for complex form layouts
Code Comparison
react-hook-form:
import { useForm } from "react-hook-form";
const { register, handleSubmit } = useForm();
const onSubmit = data => console.log(data);
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register("firstName")} />
<input type="submit" />
</form>
Designable:
import { Designer } from "@designable/react";
import { DesignableField } from "@designable/react-settings-form";
<Designer>
<DesignableField
name="firstName"
title="First Name"
x-component="Input"
/>
</Designer>
Summary
react-hook-form excels in form handling with minimal overhead, making it ideal for developers who need a lightweight solution for form management in React applications. It offers excellent performance and easy integration but is limited to form functionality.
Designable, on the other hand, provides a more comprehensive UI building experience, allowing for visual form design and a wider range of UI components. However, it may have a steeper learning curve and potentially more overhead for simpler form scenarios.
The choice between the two depends on the specific needs of the project, with react-hook-form being more suitable for focused form handling and Designable offering a broader UI design capability.
Build forms in React, without the tears ๐ญ
Pros of Formik
- Lightweight and focused on form management
- Extensive documentation and large community support
- Seamless integration with React and TypeScript
Cons of Formik
- Limited visual design capabilities
- Requires more manual coding for complex form layouts
- Less suitable for non-technical users or rapid prototyping
Code Comparison
Formik:
import { Formik, Form, Field } from 'formik';
<Formik initialValues={{ name: '' }} onSubmit={handleSubmit}>
<Form>
<Field name="name" />
<button type="submit">Submit</button>
</Form>
</Formik>
Designable:
import { Designer } from '@designable/react';
import { DesignableField } from '@designable/formily-antd';
<Designer>
<DesignableField
name="name"
title="Name"
x-component="Input"
/>
</Designer>
Formik focuses on form state management and validation, while Designable provides a visual drag-and-drop interface for form creation. Formik requires more manual coding but offers greater flexibility, whereas Designable emphasizes rapid prototyping and visual design at the cost of some customization options.
๐ High performance subscription-based form state management for React
Pros of react-final-form
- Lightweight and focused on form state management
- Extensive documentation and community support
- Easy integration with existing React projects
Cons of react-final-form
- Limited UI customization options out-of-the-box
- Requires additional libraries for complex form layouts
- Less suitable for drag-and-drop form building
Code Comparison
react-final-form:
import { Form, Field } from 'react-final-form'
<Form
onSubmit={onSubmit}
render={({ handleSubmit }) => (
<form onSubmit={handleSubmit}>
<Field name="firstName" component="input" placeholder="First Name" />
<button type="submit">Submit</button>
</form>
)}
/>
designable:
import { Designer } from 'designable-react'
import { DesignableField } from 'designable-react-settings-form'
<Designer>
<DesignableField
name="firstName"
title="First Name"
x-component="Input"
/>
</Designer>
Summary
react-final-form excels in form state management and integration with React projects, while designable offers more robust visual form building capabilities. react-final-form is better suited for developers who need fine-grained control over form logic, whereas designable is ideal for creating complex, visually customizable forms with drag-and-drop functionality.
Performance-focused API for React forms ๐
Pros of Unform
- Lightweight and flexible form library for React
- Seamless integration with popular form validation libraries
- Supports both uncontrolled and controlled components
Cons of Unform
- Less visual design capabilities compared to Designable
- Primarily focused on form handling, not a complete low-code solution
- Steeper learning curve for complex form scenarios
Code Comparison
Unform:
import { Form } from '@unform/web';
import Input from './components/Input';
function MyForm() {
function handleSubmit(data) {
console.log(data);
}
return (
<Form onSubmit={handleSubmit}>
<Input name="email" />
<Input name="password" type="password" />
<button type="submit">Submit</button>
</Form>
);
}
Designable:
import { Designer } from '@designable/react';
import { DesignableField } from '@designable/formily-antd';
const App = () => (
<Designer>
<DesignableField
name="email"
title="Email"
x-component="Input"
/>
<DesignableField
name="password"
title="Password"
x-component="Password"
/>
</Designer>
);
Summary
Unform is a lightweight form library for React, offering flexibility and easy integration with validation libraries. It's ideal for developers who need a simple yet powerful form solution. Designable, on the other hand, provides a more comprehensive low-code platform with visual design capabilities, making it suitable for creating complex forms and interfaces with less code. The choice between the two depends on the specific project requirements and the level of visual design needed.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Introduction
If you are worrying about something builder, Such as form builder/table builder/chart builder/app builder etc. Designable is your perfect choice.
Screenshot
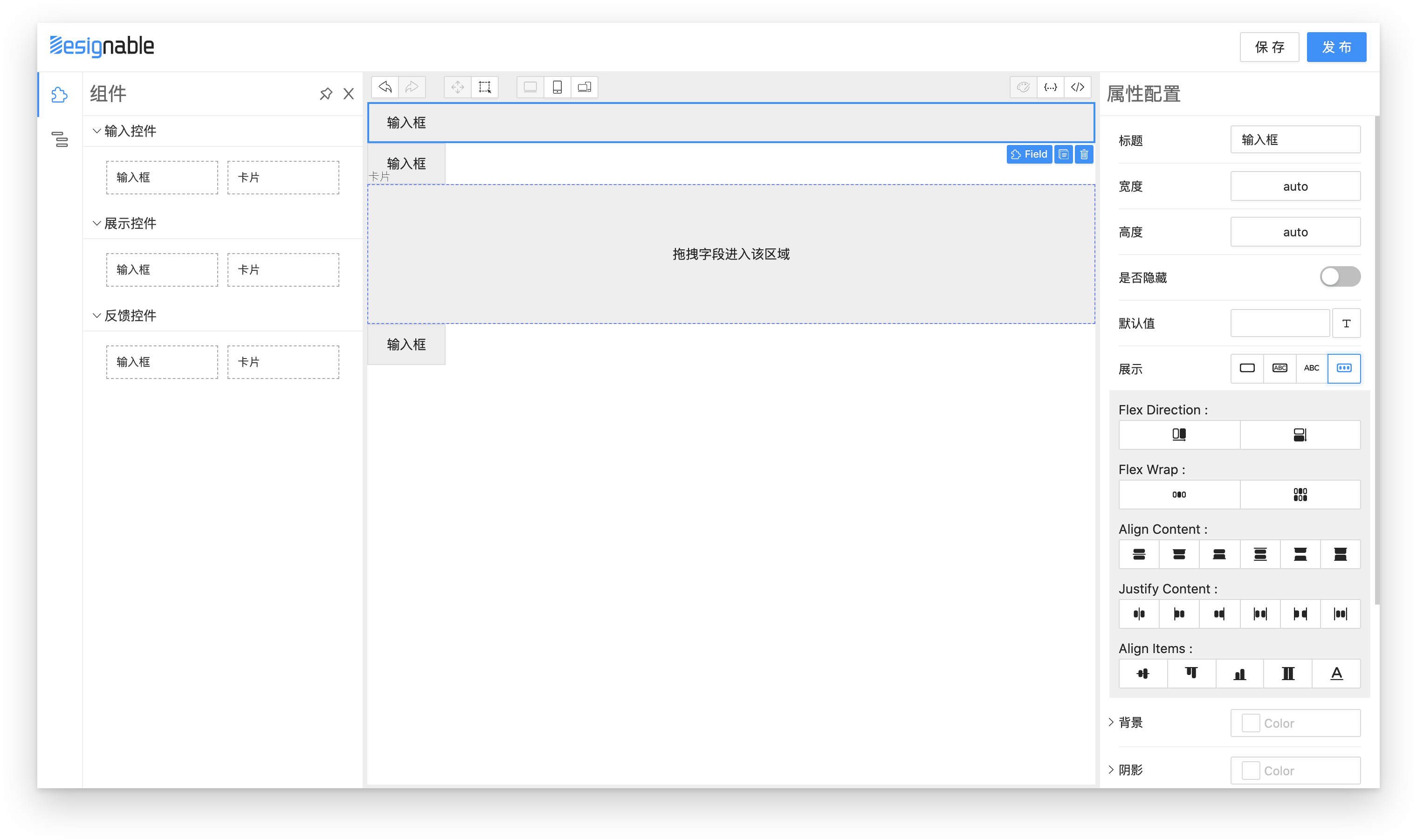
Features
- รฐยยย High performance, Smooth and beautiful drag and drop experience
- รฐยยยก Full scene coverage
- รฐยยยจ Support Low Code and No Code
- รฐยยย Strong scalability
Website
Contributors
This project exists thanks to all the people who contribute.
Top Related Projects
๐ฑ๐ ๐งฉ Cross Device & High Performance Normal Form/Dynamic(JSON Schema) Form/Form Builder -- Support React/React Native/Vue 2/Vue 3
๐ React Hooks for form state management and validation (Web + React Native)
Build forms in React, without the tears ๐ญ
๐ High performance subscription-based form state management for React
Performance-focused API for React forms ๐
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot