Top Related Projects
The content behind MDN Web Docs
Material UI: Comprehensive React component library that implements Google's Material Design. Free forever.
A utility-first CSS framework for rapid UI development.
🐉 Vue Component Framework
An enterprise-class UI design language and React UI library
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Quick Overview
Angular Material is a UI component library for Angular applications, providing a set of reusable, well-tested, and accessible components based on Google's Material Design specification. It offers a comprehensive suite of pre-built components that can be easily integrated into Angular projects, allowing developers to create modern and consistent user interfaces.
Pros
- Extensive set of pre-built, customizable components
- Seamless integration with Angular framework
- Consistent design language following Material Design principles
- Strong focus on accessibility and responsive design
Cons
- Learning curve for developers new to Material Design concepts
- Limited customization options for some components without extensive overrides
- Can lead to similar-looking applications if not customized properly
- Large bundle size if importing all components
Code Examples
- Using a Material button:
import { MatButtonModule } from '@angular/material/button';
@Component({
selector: 'app-button-example',
template: '<button mat-raised-button color="primary">Click me!</button>',
standalone: true,
imports: [MatButtonModule],
})
export class ButtonExampleComponent {}
- Implementing a Material form field with input:
import { MatInputModule } from '@angular/material/input';
import { MatFormFieldModule } from '@angular/material/form-field';
@Component({
selector: 'app-input-example',
template: `
<mat-form-field>
<mat-label>Enter your name</mat-label>
<input matInput placeholder="John Doe">
</mat-form-field>
`,
standalone: true,
imports: [MatInputModule, MatFormFieldModule],
})
export class InputExampleComponent {}
- Creating a Material dialog:
import { MatDialogModule } from '@angular/material/dialog';
@Component({
selector: 'app-dialog-example',
template: '<button (click)="openDialog()">Open Dialog</button>',
standalone: true,
imports: [MatDialogModule],
})
export class DialogExampleComponent {
constructor(public dialog: MatDialog) {}
openDialog() {
this.dialog.open(DialogContentComponent);
}
}
@Component({
selector: 'app-dialog-content',
template: '<h2 mat-dialog-title>Dialog Title</h2><p>Dialog content goes here.</p>',
standalone: true,
imports: [MatDialogModule],
})
export class DialogContentComponent {}
Getting Started
To get started with Angular Material:
-
Install Angular Material in your project:
ng add @angular/material
-
Import the desired modules in your component:
import { MatButtonModule } from '@angular/material/button'; import { MatInputModule } from '@angular/material/input';
-
Add the modules to your component's imports:
@Component({ // ... standalone: true, imports: [MatButtonModule, MatInputModule], }) export class MyComponent {}
-
Use Material components in your template:
<button mat-raised-button color="primary">Click me!</button> <mat-form-field> <input matInput placeholder="Enter text"> </mat-form-field>
Competitor Comparisons
The content behind MDN Web Docs
Pros of content
- Comprehensive web development documentation covering a wide range of topics
- Open-source and community-driven, allowing for contributions from developers worldwide
- Regularly updated to reflect the latest web standards and best practices
Cons of content
- Primarily focused on documentation rather than providing ready-to-use UI components
- May require more effort to implement specific UI elements compared to Material
- Less opinionated in terms of design and styling
Code Comparison
content (HTML example):
<article>
<h1>Main Heading</h1>
<p>This is a paragraph of text.</p>
<ul>
<li>List item 1</li>
<li>List item 2</li>
</ul>
</article>
Material (Angular component example):
@Component({
selector: 'example-component',
template: `
<mat-card>
<mat-card-title>Card Title</mat-card-title>
<mat-card-content>Card content goes here.</mat-card-content>
</mat-card>
`
})
export class ExampleComponent { }
The content repository focuses on providing documentation and examples of standard web technologies, while Material offers pre-built Angular components for rapid UI development.
Material UI: Comprehensive React component library that implements Google's Material Design. Free forever.
Pros of Material-UI
- Larger community and more frequent updates
- More comprehensive component library
- Better documentation and examples
Cons of Material-UI
- Steeper learning curve for React beginners
- Larger bundle size
- Less opinionated, requiring more configuration
Code Comparison
Material-UI (React):
import React from 'react';
import Button from '@mui/material/Button';
function App() {
return <Button variant="contained">Hello World</Button>;
}
Material (Angular):
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '<button mat-raised-button>Hello World</button>'
})
export class AppComponent { }
Both libraries provide similar functionality, but Material-UI uses React's component-based approach, while Material integrates with Angular's template syntax. Material-UI offers more flexibility in styling and customization, while Material provides a more standardized look and feel that aligns closely with Angular's ecosystem.
Material-UI is generally preferred for React projects due to its extensive component library and active community. However, for Angular projects, Material is the natural choice as it's specifically designed to work seamlessly with the Angular framework.
A utility-first CSS framework for rapid UI development.
Pros of Tailwind CSS
- Highly customizable and flexible, allowing for rapid UI development
- Smaller bundle size and better performance due to its utility-first approach
- Framework-agnostic, can be used with any JavaScript library or framework
Cons of Tailwind CSS
- Steeper learning curve for developers accustomed to traditional CSS frameworks
- Can lead to longer class names and potentially cluttered HTML markup
- Requires additional configuration for optimal purging of unused styles
Code Comparison
Material (Angular):
<mat-card>
<mat-card-title>Card Title</mat-card-title>
<mat-card-content>Card content goes here</mat-card-content>
</mat-card>
Tailwind CSS:
<div class="bg-white rounded-lg shadow-md p-6">
<h2 class="text-xl font-bold mb-4">Card Title</h2>
<p class="text-gray-700">Card content goes here</p>
</div>
The Material example uses predefined components with encapsulated styles, while Tailwind CSS utilizes utility classes to style elements directly in the HTML. Tailwind's approach offers more flexibility but requires more class management, whereas Material provides a more structured component-based system with less customization out of the box.
🐉 Vue Component Framework
Pros of Vuetify
- Larger ecosystem with more components and pre-built layouts
- More frequent updates and active community support
- Better documentation with interactive examples and playground
Cons of Vuetify
- Steeper learning curve due to more complex API
- Larger bundle size, which may impact initial load times
- Less flexibility for custom styling compared to Material
Code Comparison
Material (Angular):
<mat-button-toggle-group name="fontStyle" aria-label="Font Style">
<mat-button-toggle value="bold">Bold</mat-button-toggle>
<mat-button-toggle value="italic">Italic</mat-button-toggle>
<mat-button-toggle value="underline">Underline</mat-button-toggle>
</mat-button-toggle-group>
Vuetify:
<v-btn-toggle v-model="toggle_exclusive" mandatory>
<v-btn>
<v-icon>mdi-format-align-left</v-icon>
</v-btn>
<v-btn>
<v-icon>mdi-format-align-center</v-icon>
</v-btn>
<v-btn>
<v-icon>mdi-format-align-right</v-icon>
</v-btn>
<v-btn>
<v-icon>mdi-format-align-justify</v-icon>
</v-btn>
</v-btn-toggle>
Both libraries offer comprehensive UI component sets for their respective frameworks. Material provides a more streamlined experience for Angular developers, while Vuetify offers a wider range of components and customization options for Vue.js projects. The choice between them often depends on the specific framework being used and the project requirements.
An enterprise-class UI design language and React UI library
Pros of Ant Design
- Larger ecosystem with more components and tools
- Better internationalization support
- More flexible and customizable theming system
Cons of Ant Design
- Steeper learning curve due to more complex API
- Larger bundle size, which may impact performance
- Less seamless integration with Angular projects
Code Comparison
Ant Design (React):
import { Button } from 'antd';
const MyComponent = () => (
<Button type="primary">Click me</Button>
);
Angular Material:
import { MatButtonModule } from '@angular/material/button';
@Component({
template: '<button mat-raised-button color="primary">Click me</button>'
})
export class MyComponent {}
Summary
Ant Design offers a more comprehensive set of components and better internationalization support, making it suitable for large-scale applications. However, it may have a steeper learning curve and larger bundle size compared to Angular Material. Angular Material provides tighter integration with Angular projects and a simpler API, but with fewer components and less flexibility in theming. The choice between the two depends on the specific project requirements, team expertise, and performance considerations.
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Pros of Ionic Framework
- Cross-platform development: Build for iOS, Android, and web from a single codebase
- Extensive UI component library with native-like look and feel
- Strong focus on performance and optimizations for mobile devices
Cons of Ionic Framework
- Steeper learning curve, especially for developers new to hybrid app development
- May have limitations for highly complex or performance-intensive applications
- Dependency on Cordova for native functionality can introduce additional complexity
Code Comparison
Ionic Framework:
import { Component } from '@angular/core';
import { IonicModule } from '@ionic/angular';
@Component({
selector: 'app-home',
template: '<ion-button>Click me</ion-button>',
standalone: true,
imports: [IonicModule],
})
export class HomePage {}
Angular Material:
import { Component } from '@angular/core';
import { MatButtonModule } from '@angular/material/button';
@Component({
selector: 'app-home',
template: '<button mat-button>Click me</button>',
standalone: true,
imports: [MatButtonModule],
})
export class HomePage {}
Both frameworks provide component-based architectures and use similar Angular syntax. However, Ionic Framework focuses on mobile-first design with native-like components, while Angular Material offers a more general-purpose UI library following Material Design principles.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
Material Design for AngularJS Apps
Material Design is a specification for a unified system of visual, motion, and interaction design that adapts across different devices. Our goal is to deliver a lean, lightweight set of AngularJS-native UI elements that implement the material design specification for use in AngularJS single-page applications (SPAs).
AngularJS Material is an implementation of Google's Material Design Specification (2014-2017) for AngularJS (v1.x) developers.
For an implementation of the Material Design Specification (2018+), please see the Angular Material project which is built for Angular (v2+) developers.
End-of-Life
AngularJS Material support has officially ended as of January 2022. See what ending support means and read the end of life announcement. Visit material.angular.io for the actively supported Angular Material.
Find details on reporting security issues here.
AngularJS Material includes a rich set of reusable, well-tested, and accessible UI components.
Quick Links:
Please note that using the latest version of AngularJS Material requires the use of AngularJS 1.7.2 or higher.
AngularJS Material supports the browser versions defined in the browserslist
field
of our package.json. Find out more on our
docs site.
AngularJS Material supports the screen reader versions listed here.
Online Documentation and Demos
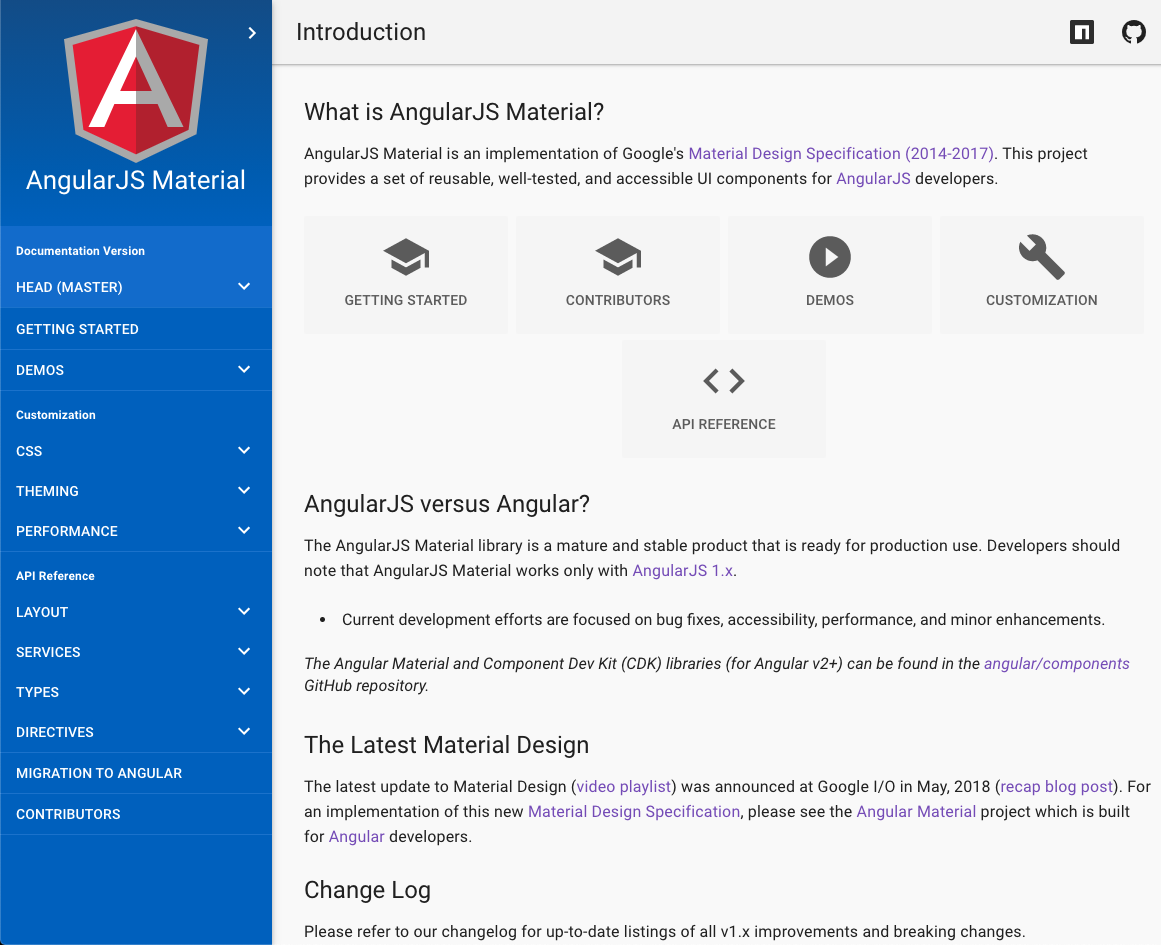
- Visit material.angularjs.org online to review the API, see the components in action via live demos, and to read our detailed guides which include the layout system, theming system, typography, and more.
- Or you can build the documentation and demos locally; see Build Docs & Demos for details.
Building
Developers can build AngularJS Material using NPM and gulp.
First install or update your local project's npm dependencies:
npm install
Install Gulp v3 globally:
npm install -g gulp@3
Then run the gulp tasks:
# To build `angular-material.js/.css` and `Theme` files in the `/dist` directory
gulp build
# To build the AngularJS Material Docs and Demos in `/dist/docs` directory
gulp docs
For development, use the docs:watch
NPM script to run in dev mode:
# To build the AngularJS Material Source, Docs, and Demos in watch mode
npm run docs:watch
For more details on how the build process works and additional commands (available for testing and debugging) developers should read the Build Guide.
Installing Build (Distribution Files)
NPM
For developers not interested in building the AngularJS Material library... use NPM to install and use the AngularJS Material distribution files.
Change to your project's root directory.
# To get the latest stable version, use NPM from the command line.
npm install angular-material --save
# To get the most recent, latest committed-to-master version use:
npm install http://github.com/angular/bower-material#master --save
Other Dependency Managers
Visit our distribution repository for more details on how to install and use the AngularJS Material distribution files within your local project.
CDN
CDN versions of AngularJS Material are available.
With the Google CDN, you will not need to download local copies of the distribution files. Instead, simply reference the CDN urls to easily use those remote library files. This is especially useful when using online tools such as CodePen or Plunker.
<head>
<!-- AngularJS Material CSS now available via Google CDN; version 1.2.1 used here -->
<link rel="stylesheet" href="https://ajax.googleapis.com/ajax/libs/angular_material/1.2.1/angular-material.min.css">
</head>
<body>
<!-- AngularJS Material Dependencies -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular-animate.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular-aria.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular-messages.min.js"></script>
<!-- AngularJS Material Javascript now available via Google CDN; version 1.2.1 used here -->
<script src="https://ajax.googleapis.com/ajax/libs/angular_material/1.2.1/angular-material.min.js"></script>
</body>
Developers seeking the latest, most-current build versions can use GitCDN.xyz to pull directly from our distribution repository:
<head>
<!-- AngularJS Material CSS using GitCDN to load directly from `bower-material/master` -->
<link rel="stylesheet" href="https://gitcdn.xyz/cdn/angular/bower-material/master/angular-material.css">
</head>
<body>
<!-- AngularJS Material Dependencies -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular-animate.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular-aria.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular-messages.min.js"></script>
<!-- AngularJS Material Javascript using GitCDN to load directly from `bower-material/master` -->
<script src="https://gitcdn.xyz/cdn/angular/bower-material/master/angular-material.js"></script>
</body>
Once you have all the necessary assets installed, add ngMaterial
and ngMessages
as dependencies for your
app:
angular.module('myApp', ['ngMaterial', 'ngMessages']);
Top Related Projects
The content behind MDN Web Docs
Material UI: Comprehensive React component library that implements Google's Material Design. Free forever.
A utility-first CSS framework for rapid UI development.
🐉 Vue Component Framework
An enterprise-class UI design language and React UI library
A powerful cross-platform UI toolkit for building native-quality iOS, Android, and Progressive Web Apps with HTML, CSS, and JavaScript.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot