Top Related Projects
Simple HTML5 Charts using the <canvas> tag
📊 Vue 2 component for ApexCharts
Vue.js component for Apache ECharts™.
Quick Overview
vue-chartjs is a wrapper for Chart.js in Vue.js. It provides easy-to-use components for creating various types of charts in Vue applications, allowing developers to leverage the power of Chart.js with the simplicity of Vue's component system.
Pros
- Seamless integration with Vue.js components
- Easy to use and implement in Vue projects
- Supports all Chart.js chart types
- Reactive data updates without the need for manual chart redraws
Cons
- Limited to Chart.js functionality; custom chart types may be challenging
- Learning curve for those unfamiliar with Chart.js
- May have performance issues with large datasets
- Documentation could be more comprehensive for advanced use cases
Code Examples
- Creating a simple line chart:
<template>
<LineChart :chart-data="chartData" />
</template>
<script>
import { LineChart } from 'vue-chartjs'
export default {
components: { LineChart },
data() {
return {
chartData: {
labels: ['January', 'February', 'March'],
datasets: [
{
label: 'Data One',
data: [40, 20, 12]
}
]
}
}
}
}
</script>
- Using mixins for chart options:
<script>
import { Bar } from 'vue-chartjs'
import { Chart as ChartJS, Title, Tooltip, Legend, BarElement, CategoryScale, LinearScale } from 'chart.js'
ChartJS.register(Title, Tooltip, Legend, BarElement, CategoryScale, LinearScale)
export default {
extends: Bar,
props: ['chartData'],
mounted() {
this.renderChart(this.chartData, {
responsive: true,
maintainAspectRatio: false
})
}
}
</script>
- Reactive chart updates:
<template>
<div>
<button @click="addData">Add Data</button>
<LineChart :chart-data="chartData" :options="chartOptions" />
</div>
</template>
<script>
import { LineChart } from 'vue-chartjs'
export default {
components: { LineChart },
data() {
return {
chartData: {
labels: ['January', 'February', 'March'],
datasets: [{ data: [10, 20, 30] }]
},
chartOptions: {
responsive: true
}
}
},
methods: {
addData() {
this.chartData.labels.push('April')
this.chartData.datasets[0].data.push(40)
this.$set(this.chartData, 'labels', this.chartData.labels)
this.$set(this.chartData.datasets[0], 'data', this.chartData.datasets[0].data)
}
}
}
</script>
Getting Started
-
Install the package:
npm install vue-chartjs chart.js
-
Import and use in your Vue component:
<template> <LineChart :chart-data="chartData" /> </template> <script> import { Line as LineChart } from 'vue-chartjs' import { Chart as ChartJS, Title, Tooltip, Legend, LineElement, LinearScale, CategoryScale, PointElement } from 'chart.js' ChartJS.register(Title, Tooltip, Legend, LineElement, LinearScale, CategoryScale, PointElement) export default { components: { LineChart }, data() { return { chartData: { labels: ['January', 'February', 'March'], datasets: [{ data: [40, 20, 12] }] } } } } </script>
Competitor Comparisons
Simple HTML5 Charts using the <canvas> tag
Pros of Chart.js
- More flexible and can be used with various frameworks or vanilla JavaScript
- Larger community and ecosystem, leading to more resources and third-party plugins
- Direct access to Chart.js features and updates without waiting for wrapper maintenance
Cons of Chart.js
- Requires more setup and configuration when used with Vue.js
- Less Vue-specific optimizations and integrations
- May require additional work to make it reactive with Vue's data management
Code Comparison
Chart.js (vanilla JavaScript):
const ctx = document.getElementById('myChart').getContext('2d');
const chart = new Chart(ctx, {
type: 'bar',
data: chartData,
options: chartOptions
});
vue-chartjs:
<bar-chart :chart-data="chartData" :options="chartOptions"></bar-chart>
import { Bar } from 'vue-chartjs'
export default {
extends: Bar,
mounted() {
this.renderChart(this.chartData, this.chartOptions)
}
}
vue-chartjs is a wrapper for Chart.js specifically designed for Vue.js applications. It provides a more Vue-centric approach to using Chart.js, with better integration into Vue's component system and reactivity. However, Chart.js offers more flexibility and can be used in a wider range of projects beyond Vue.js. The choice between the two depends on the specific needs of your project and your preference for Vue-specific tooling versus a more general-purpose charting library.
📊 Vue 2 component for ApexCharts
Pros of vue-apexcharts
- More feature-rich with a wider variety of chart types and customization options
- Better performance for large datasets and real-time updates
- Active development with frequent updates and improvements
Cons of vue-apexcharts
- Steeper learning curve due to more complex API and configuration options
- Larger bundle size, which may impact initial load times for smaller projects
Code Comparison
vue-chartjs:
<script>
import { Bar } from 'vue-chartjs'
export default {
extends: Bar,
mounted() {
this.renderChart(this.chartData, this.options)
}
}
</script>
vue-apexcharts:
<template>
<apexchart type="bar" :options="chartOptions" :series="series"></apexchart>
</template>
<script>
export default {
data: () => ({
chartOptions: { ... },
series: [ ... ]
})
}
</script>
vue-chartjs provides a simpler API with less boilerplate, while vue-apexcharts offers more granular control over chart configuration. vue-apexcharts uses a template-based approach, whereas vue-chartjs extends the chart component directly in the script section.
Both libraries have their strengths, with vue-chartjs being easier to get started with and vue-apexcharts offering more advanced features and customization options. The choice between them depends on the specific requirements of your project and the level of chart complexity needed.
Vue.js component for Apache ECharts™.
Pros of vue-echarts
- More comprehensive and feature-rich, offering a wider range of chart types and customization options
- Better performance for large datasets and complex visualizations
- Stronger community support and more frequent updates
Cons of vue-echarts
- Steeper learning curve due to its extensive API and configuration options
- Larger bundle size, which may impact initial load times for smaller applications
Code Comparison
vue-echarts:
<template>
<v-chart :option="option" />
</template>
<script>
import { use } from "echarts/core";
import { CanvasRenderer } from "echarts/renderers";
import { PieChart } from "echarts/charts";
import VChart from "vue-echarts";
use([CanvasRenderer, PieChart]);
export default {
components: { VChart },
data() {
return {
option: {
series: [{
type: 'pie',
data: [/* ... */]
}]
}
};
}
};
</script>
vue-chartjs:
<template>
<Pie :chart-data="chartData" />
</template>
<script>
import { Pie } from 'vue-chartjs';
export default {
components: { Pie },
data() {
return {
chartData: {
labels: [/* ... */],
datasets: [{
data: [/* ... */]
}]
}
};
}
};
</script>
Both libraries offer Vue.js components for creating charts, but vue-echarts provides more flexibility and power at the cost of complexity, while vue-chartjs offers a simpler API for basic charting needs.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
vue-chartjs
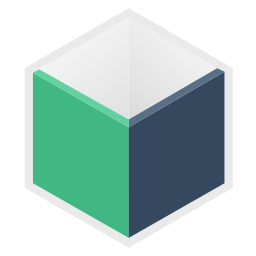
vue-chartjs is a wrapper for Chart.js in Vue. You can easily create reuseable chart components.
Supports Chart.js v4.
QuickStart ⢠Docs ⢠Stack Overflow
Quickstart
Install this library with peer dependencies:
pnpm add vue-chartjs chart.js
# or
yarn add vue-chartjs chart.js
# or
npm i vue-chartjs chart.js
Then, import and use individual components:
<template>
<Bar :data="data" :options="options" />
</template>
<script lang="ts">
import {
Chart as ChartJS,
Title,
Tooltip,
Legend,
BarElement,
CategoryScale,
LinearScale
} from 'chart.js'
import { Bar } from 'vue-chartjs'
ChartJS.register(CategoryScale, LinearScale, BarElement, Title, Tooltip, Legend)
export default {
name: 'App',
components: {
Bar
},
data() {
return {
data: {
labels: ['January', 'February', 'March'],
datasets: [{ data: [40, 20, 12] }]
},
options: {
responsive: true
}
}
}
}
</script>
Need an API to fetch data? Consider Cube, an open-source API for data apps.
Docs
- Reactivity
- Access to Chart instance
- Accessibility
- Migration from v4 to v5
- Migration from vue-chart-3
- API
- Examples
Build Setup
# install dependencies
pnpm install
# build for production with minification
pnpm build
# run unit tests
pnpm test:unit
# run all tests
pnpm test
Contributing
- Fork it ( https://github.com/apertureless/vue-chartjs/fork )
- Create your feature branch (
git checkout -b my-new-feature
) - Commit your changes (
git commit -am 'Add some feature'
) - Push to the branch (
git push origin my-new-feature
) - Create a new Pull Request
License
This software is distributed under MIT license.
Top Related Projects
Simple HTML5 Charts using the <canvas> tag
📊 Vue 2 component for ApexCharts
Vue.js component for Apache ECharts™.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot