Top Related Projects
Cheatsheets for experienced React developers getting started with TypeScript
Code from the React tutorial.
A collection of awesome things regarding React ecosystem
📋 React Hooks for form state management and validation (Web + React Native)
🔥 A highly scalable, offline-first foundation with the best developer experience and a focus on performance and best practices.
Quick Overview
The arkency/reactjs_koans
repository is a collection of exercises designed to help developers learn and understand the fundamentals of React.js, a popular JavaScript library for building user interfaces. These "koans" (a term borrowed from Zen Buddhism) are a series of small, focused exercises that guide the learner through various React concepts and best practices.
Pros
- Hands-on Learning: The koans provide a practical, interactive way to learn React, allowing developers to write and test code directly within the exercises.
- Comprehensive Coverage: The repository covers a wide range of React topics, from basic component rendering to more advanced concepts like state management and lifecycle methods.
- Structured Approach: The koans are organized in a logical progression, making it easier for learners to build their understanding step-by-step.
- Community Support: The project has a dedicated community of contributors and users, providing support and feedback for those working through the exercises.
Cons
- Outdated Dependencies: The project uses older versions of React and other dependencies, which may not reflect the latest best practices and features.
- Limited Flexibility: The koans follow a predetermined structure, which may not align with the learning preferences or prior knowledge of all developers.
- Lack of Guidance: While the repository provides the exercises, it may not offer detailed explanations or supplementary resources for those who need more comprehensive guidance.
- Potential Complexity: For beginners, the koans may present a steep learning curve, as they assume a certain level of familiarity with JavaScript and web development concepts.
Getting Started
To get started with the arkency/reactjs_koans
repository, follow these steps:
- Clone the repository to your local machine:
git clone https://github.com/arkency/reactjs_koans.git
- Navigate to the project directory:
cd reactjs_koans
- Install the required dependencies:
npm install
- Start the development server:
npm start
-
Open your web browser and navigate to
http://localhost:3000
. You should see the koans interface, where you can work through the exercises. -
To run a specific koan, open the corresponding file (e.g.,
01_hello_world.js
) in thesrc/koans
directory and follow the instructions within the file. -
As you complete each koan, the test suite will provide feedback on your progress, helping you identify areas for improvement.
Remember, the arkency/reactjs_koans
repository is designed to be a learning tool, so don't hesitate to experiment, ask questions, and seek out additional resources to deepen your understanding of React.js.
Competitor Comparisons
Cheatsheets for experienced React developers getting started with TypeScript
Pros of react
- Comprehensive TypeScript-focused cheatsheets for React development
- Regularly updated with the latest React and TypeScript best practices
- Large community contribution and maintenance
Cons of react
- May be overwhelming for beginners due to its extensive content
- Focuses primarily on TypeScript, which might not be suitable for pure JavaScript developers
Code Comparison
reactjs_koans:
assert.deepEqual(
yourCode.transformCountriesData(countriesData),
expectedOutput,
"The transformed data doesn't match the expected output"
);
react:
interface Props {
name: string;
age: number;
}
const MyComponent: React.FC<Props> = ({ name, age }) => {
return <div>{`Hello, ${name}! You are ${age} years old.`}</div>;
};
Summary
While reactjs_koans provides hands-on exercises for learning React with JavaScript, react offers comprehensive TypeScript-focused cheatsheets for React development. reactjs_koans may be more suitable for beginners and those focusing on JavaScript, while react caters to developers looking to leverage TypeScript in their React projects. The code comparison highlights the difference in focus, with reactjs_koans emphasizing testing and react demonstrating TypeScript usage in React components.
Code from the React tutorial.
Pros of react-tutorial
- Official tutorial maintained by the React team, ensuring up-to-date content
- Focuses on building a practical, interactive application (tic-tac-toe game)
- Provides a comprehensive introduction to React concepts and best practices
Cons of react-tutorial
- Limited in scope, covering only basic React concepts
- Less hands-on coding exercises compared to reactjs_koans
- May not cover advanced topics or edge cases in React development
Code Comparison
react-tutorial:
class Square extends React.Component {
render() {
return (
<button className="square" onClick={() => this.props.onClick()}>
{this.props.value}
</button>
);
}
}
reactjs_koans:
var TodoList = React.createClass({
render: function() {
return (
<ul>
<li>Learn React</li>
<li>Build an awesome app</li>
</ul>
);
}
});
The react-tutorial example uses modern class syntax and demonstrates component props and event handling, while the reactjs_koans example uses the older createClass
method and focuses on basic JSX rendering.
A collection of awesome things regarding React ecosystem
Pros of awesome-react
- Comprehensive collection of React resources, tools, and libraries
- Regularly updated with new content and community contributions
- Covers a wide range of React-related topics, from basics to advanced concepts
Cons of awesome-react
- Lacks hands-on learning exercises or interactive components
- May be overwhelming for beginners due to the sheer volume of information
- Does not provide structured learning path or progression
Code comparison
reactjs_koans focuses on interactive learning through exercises:
var React = require("react");
module.exports = React.createClass({
render: function() {
return (
<div>
Hello World!
</div>
);
}
});
awesome-react primarily provides links and descriptions:
## React
### React General Resources
* [React Official Website](https://reactjs.org/)
* [React Documentation](https://reactjs.org/docs)
* [React GitHub](https://github.com/facebook/react)
Summary
reactjs_koans is designed for hands-on learning through interactive exercises, making it ideal for beginners and those who prefer a structured approach. awesome-react serves as a comprehensive resource hub, offering a vast collection of React-related materials suitable for developers at all levels. While reactjs_koans provides a more focused learning experience, awesome-react offers a broader overview of the React ecosystem and its various tools and libraries.
📋 React Hooks for form state management and validation (Web + React Native)
Pros of react-hook-form
- More focused on form handling and validation
- Actively maintained with frequent updates
- Comprehensive documentation and examples
Cons of react-hook-form
- Steeper learning curve for beginners
- Less emphasis on general React concepts
- May be overkill for simple form implementations
Code Comparison
reactjs_koans:
var TodoList = React.createClass({
render: function() {
return (
<ul>
{this.props.items.map(function(item) {
return <li key={item.id}>{item.text}</li>;
})}
</ul>
);
}
});
react-hook-form:
import { useForm } from "react-hook-form";
function App() {
const { register, handleSubmit } = useForm();
const onSubmit = data => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register("firstName")} />
<input {...register("lastName")} />
<input type="submit" />
</form>
);
}
Summary
reactjs_koans is designed as a learning tool for React beginners, focusing on core concepts through interactive exercises. react-hook-form, on the other hand, is a production-ready library specifically for handling forms in React applications. While reactjs_koans provides a broader introduction to React, react-hook-form offers more advanced and specialized functionality for form management.
🔥 A highly scalable, offline-first foundation with the best developer experience and a focus on performance and best practices.
Pros of react-boilerplate
- More comprehensive and production-ready setup
- Includes advanced features like code splitting and offline-first functionality
- Actively maintained with frequent updates
Cons of react-boilerplate
- Steeper learning curve due to its complexity
- May be overkill for smaller projects or beginners
- Opinionated structure might not suit all project needs
Code Comparison
reactjs_koans:
var ProductsList = React.createClass({
render: function() {
return (
<ul>
<li>Product 1</li>
<li>Product 2</li>
</ul>
);
}
});
react-boilerplate:
import React from 'react';
import styled from 'styled-components';
const List = styled.ul`
list-style: none;
margin: 0;
padding: 0;
`;
export function ProductsList() {
return (
<List>
<li>Product 1</li>
<li>Product 2</li>
</List>
);
}
The react-boilerplate example showcases modern React practices with functional components, styled-components for CSS-in-JS, and ES6 module syntax. In contrast, reactjs_koans uses older React.createClass syntax, which is more suitable for learning basic concepts but less relevant for current React development.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual CopilotREADME
React.js Koans
If you want to learn React.js you came to the right place. We prepared a set of practical exercises that will help you learn React.js from square one. The only thing you need to know is JavaScript. Here we are using ECMAScript 2015 standard.
The Koans are a set of tasks to complete. Prepared tests checks if they are done correctly.
Installation
Make sure you have Node.js and Python 2 installed.
git clone https://github.com/arkency/reactjs_koans.git
cd reactjs_koans
npm run setup
Koans structure
- Edit the files found in the
exercises
directory. - The
koans
directory contains the source of all the exercises.test
contains the tests. src
contains files compiled fromexercises
.build
contains sources launched in the web browser version of Koans.
Start a local web server (optional)
You can run a webserver and see your changes live in your web browser:
- Run command
npm run start
- Visit http://localhost:8080/
Instructions
- Remember about running the setup script before you start working on Koans!
- Work on the code found in the
exercises
directory and run the tests to see if you did everything right. - You need to do the exercises in the given order.
- Try to not peek at the test files! They contain spoilers.
- To run the tests, use
npm run test
. To automatically run tests when your code changes, usenpm run watch
.
Video walkthrough
More than just Koans
Blog
If you want to read more about React.js and modern JavaScript applications, check out our React Kung Fu blog.
The book
For people who finished Koans, we prepared something to go continue: the React by example book. In this book, we explain how to create common widgets like password-strength meter or credit card input.
For the price of the ebook, you get:
- Over 140 pages of React content. From fast introduction to React to example Todo app;
- 11 practical real-world examples;
- Repositories with code for most of the examples;
It's an early version of the book. It means some wording in book may change and there will be more examples later. All updates for the book are free.
You can use special 20% discount code: REACTKOANS. Grab your copy today or download a free chapter
Additional resources
- React docs - it's a great source of in-depth information about React.
- Why keys are important in React - great reading explaining the reason for React's keys.
- Reactiflux. User group on Slack. You can meet a lot of people using React there. There's a channel for beginners
needhelp
.
About
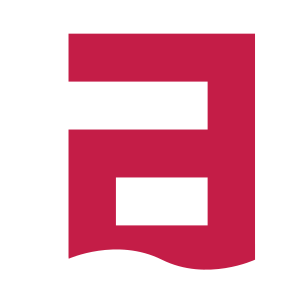
React Koans is funded and maintained by Arkency. Check out our other open-source projects.
You can also hire us or read our blog.
Top Related Projects
Cheatsheets for experienced React developers getting started with TypeScript
Code from the React tutorial.
A collection of awesome things regarding React ecosystem
📋 React Hooks for form state management and validation (Web + React Native)
🔥 A highly scalable, offline-first foundation with the best developer experience and a focus on performance and best practices.
Convert
designs to code with AI
Introducing Visual Copilot: A new AI model to turn Figma designs to high quality code using your components.
Try Visual Copilot